To retrieve the owner of a specific file using PowerShell, you can use the `Get-Acl` cmdlet followed by the `Owner` property, as shown in the code snippet below:
(Get-Acl "C:\Path\To\Your\File.txt").Owner
Understanding File Ownership
What is File Ownership?
File ownership refers to the control and permissions assigned to files and folders within an operating system. Each file has an owner, usually the user who created it, and this ownership dictates who can access, modify, or delete that file. Knowing file ownership is crucial for managing security and ensuring that sensitive files are protected properly.
Why Know the File Owner?
Understanding file ownership is vital for several reasons:
-
Security: Identifying file owners helps pinpoint potential vulnerabilities or unauthorized access attempts. If a file that should be restricted is owned by a user without the need for access, it could pose a risk.
-
File Management: Effective file management relies on knowing who owns what. If files are mismanaged, it could lead to confusion, duplication, and loss of data integrity.
-
Auditing and Compliance: Organizations often need to conduct audits for compliance with regulations. Knowing file ownership helps provide transparency in managing sensitive information.
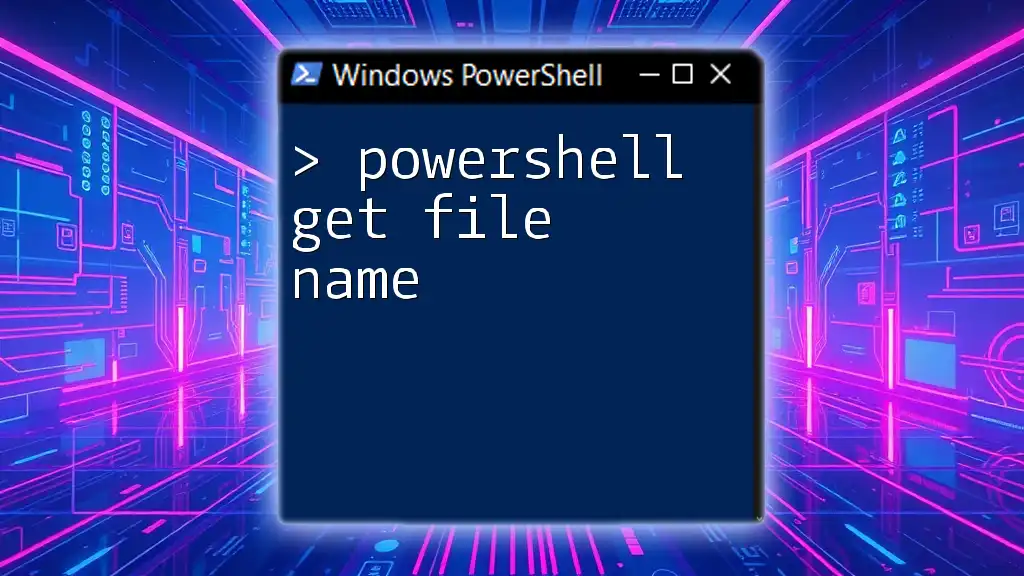
Introduction to PowerShell
What is PowerShell?
PowerShell is a powerful task automation and configuration management framework built on the .NET framework. It enables users to carry out a multitude of tasks such as managing system configurations, automating repetitive tasks, and retrieving detailed system information. Unlike traditional command-line interfaces, PowerShell operates primarily with cmdlets, which are specialized functions built into the shell.
Why Use PowerShell for File Management?
PowerShell simplifies file management with its versatility and strong scripting capabilities. Here are a few scenarios where using PowerShell can prove beneficial:
-
Automation: Tasks that would typically take several manual steps can be executed with a single command or script, saving time and reducing the chance for human error.
-
Scripting: Complex file management tasks can be scripted, enabling repeatable processes across varied environments, which is particularly useful for IT administration.
-
Remote Management: PowerShell allows users to manage files and systems remotely, providing a level of convenience that is critical for system administrators.
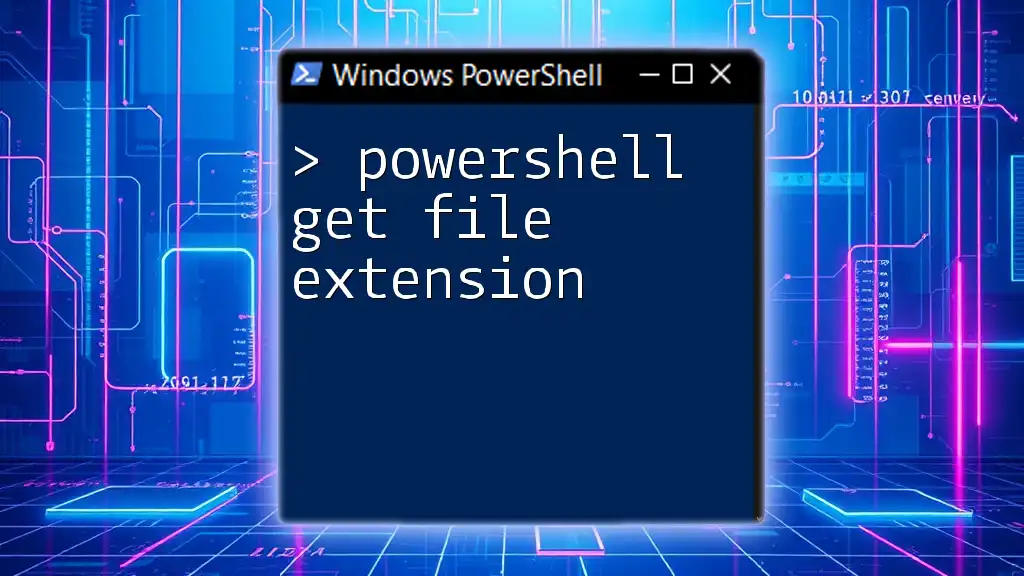
Retrieving File Owner Information
Key PowerShell Cmdlets for File Owner
To get file ownership information, you will primarily use the following PowerShell cmdlets:
-
`Get-Acl`: Retrieves the Access Control List (ACL) for a file, which contains the owner’s information and other permission-related details.
-
`Get-Item`: Retrieves an item within the file system, which could be a file or a directory.
Understanding Access Control Lists (ACLs)
Access Control Lists (ACLs) play a vital role in the world of file ownership. An ACL specifies which users or groups have access to a particular file and what permissions they hold (read, write, modify, delete). The structure of an ACL typically includes two critical components:
-
Owner: The user who has ownership of the file and thus the ultimate control.
-
Group: The user group that may have various permissions associated with the file.
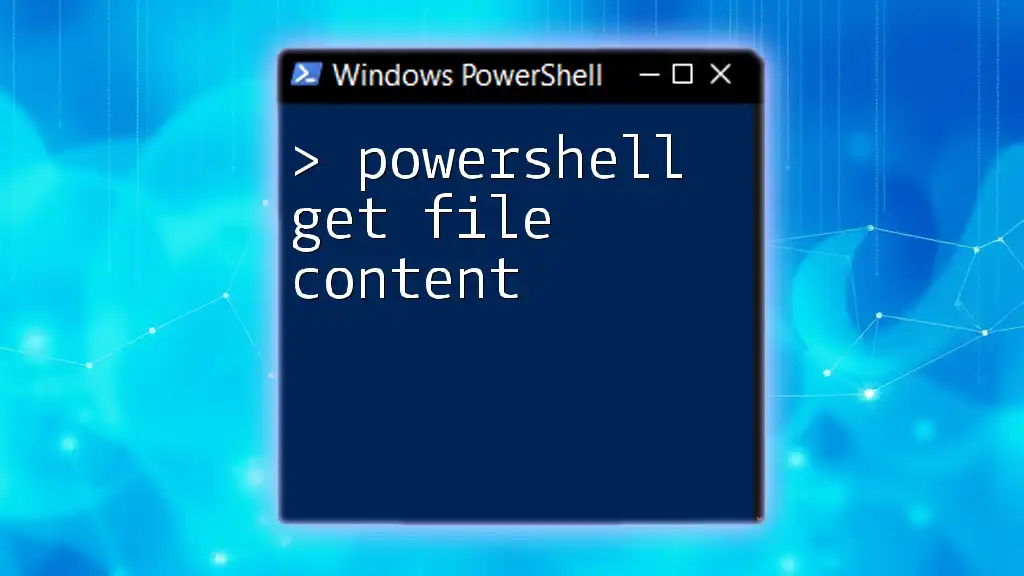
How to Use PowerShell to Get File Owner
Basic Command to Retrieve Owner
To retrieve the owner of a specific file, you can execute one of the simplest commands in PowerShell:
Get-Acl "C:\Path\To\Your\File.txt" | Select-Object -ExpandProperty Owner
In this command, `Get-Acl` fetches the ACL for the specified file, while `Select-Object -ExpandProperty Owner` extracts just the owner information. This straightforward command is your first step in understanding how to navigate file ownership through PowerShell.
Detailed Example: Retrieving Owner of Multiple Files
If you aim to retrieve the owners of multiple files, you can extend this command with a loop. Examine the following example:
Get-ChildItem "C:\Path\To\Your\Files\*" | ForEach-Object { Get-Acl $_.FullName | Select-Object -Property @{Name='File';Expression={$_.PSPath}}, @{Name='Owner';Expression={$_.Owner}} }
This command begins with `Get-ChildItem` to list all files in the specified directory. Then, it pipes each file through `ForEach-Object`, retrieving the ACL and selecting both the file path and the owner for each file. This way, you can analyze ownership for multiple files in one command.
Customizing Output
Formatting Results
To make the output more readable, you can format it using PowerShell’s `Format-Table` or `Format-List`. Here’s how to enhance the output format using `Format-Table`:
Get-ChildItem "C:\Path\To\Your\Files\*" | ForEach-Object { Get-Acl $_.FullName | Select-Object @{Name='File';Expression={$_.PSPath}}, @{Name='Owner';Expression={$_.Owner}} } | Format-Table -AutoSize
This modification optimizes the display, allowing you to see the file path alongside the owner neatly arranged in a tabular format.
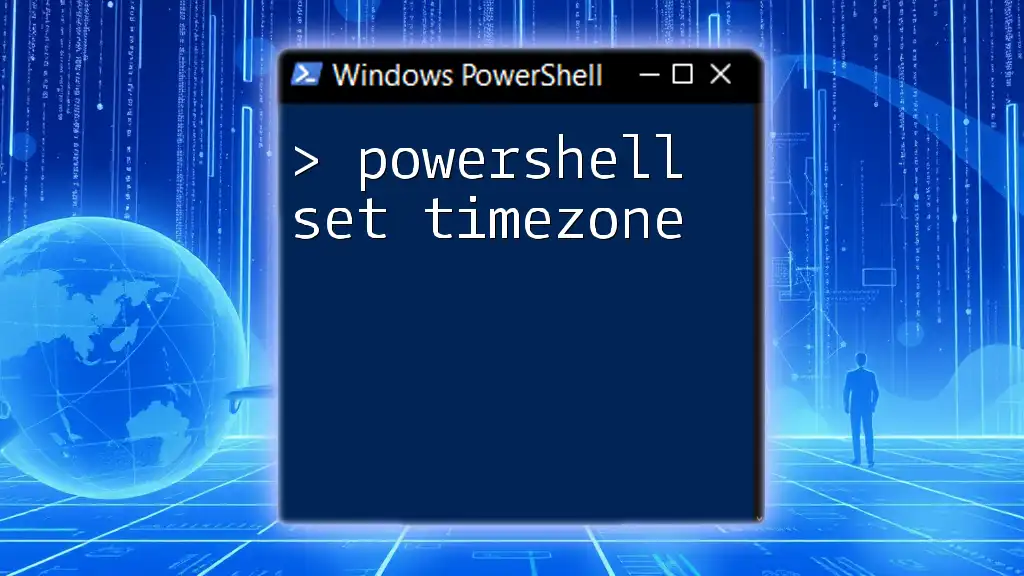
Advanced Techniques
Getting Owners of Files Recursively
If you need to fetch owners for files located in subdirectories, you can implement recursion with the `-Recurse` parameter:
Get-ChildItem "C:\Path\To\Your\Files\" -Recurse | ForEach-Object { Get-Acl $_.FullName | Select-Object @{Name='File';Expression={$_.PSPath}}, @{Name='Owner';Expression={$_.Owner}} }
Using the `-Recurse` option allows PowerShell to search through all nested directories, giving you comprehensive ownership information for all files beneath the specified path.
Filtering by Owner
Finding Specific Owner
If you need to find files owned by a specific user, leveraging conditional logic in PowerShell is an effective approach. Here’s how you can filter the results based on ownership:
Get-ChildItem "C:\Path\To\Your\Files\" | ForEach-Object { if ((Get-Acl $_.FullName).Owner -eq "DOMAIN\User") { $_.FullName } }
In this command, replace `"DOMAIN\User"` with the actual user for whom you wish to search. This command streamlines the process of identifying files owned by specified users, crucial for audits or security assessments.
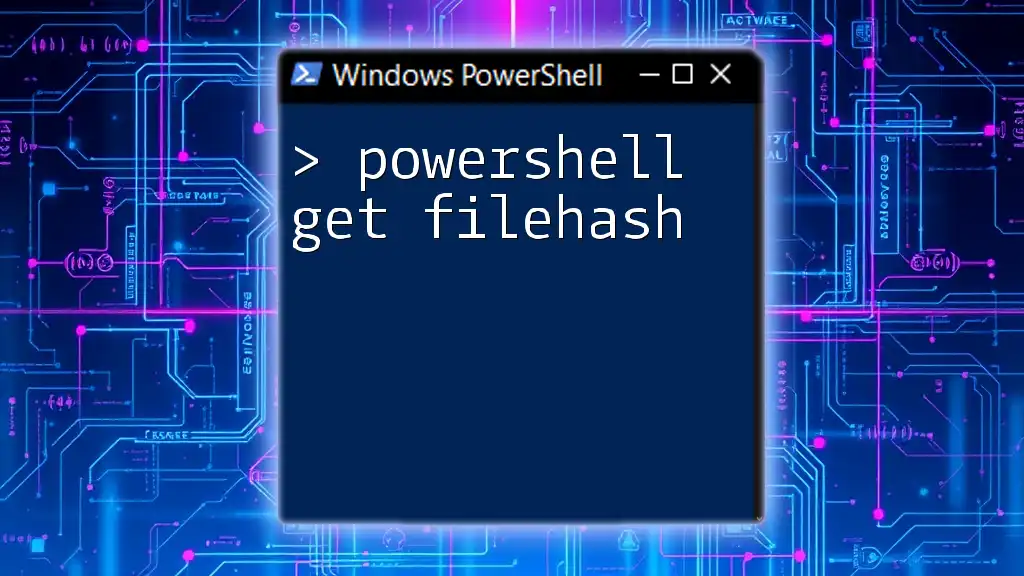
Common Issues and Troubleshooting
Access Denied Errors
While retrieving file ownership information, you may encounter "Access Denied" errors. This can happen due to insufficient permissions. To address this issue, ensure that you are running PowerShell with elevated permissions (Run as Administrator). If the problem persists, you may need to adjust user access levels or contact your system administrator for assistance.
Performance Considerations
When executing commands against a large number of files, performance can become a concern. To optimize the execution time, consider narrowing your search scope by specifying a more precise directory or limiting the types of files processed. This helps avoid unnecessary overhead.
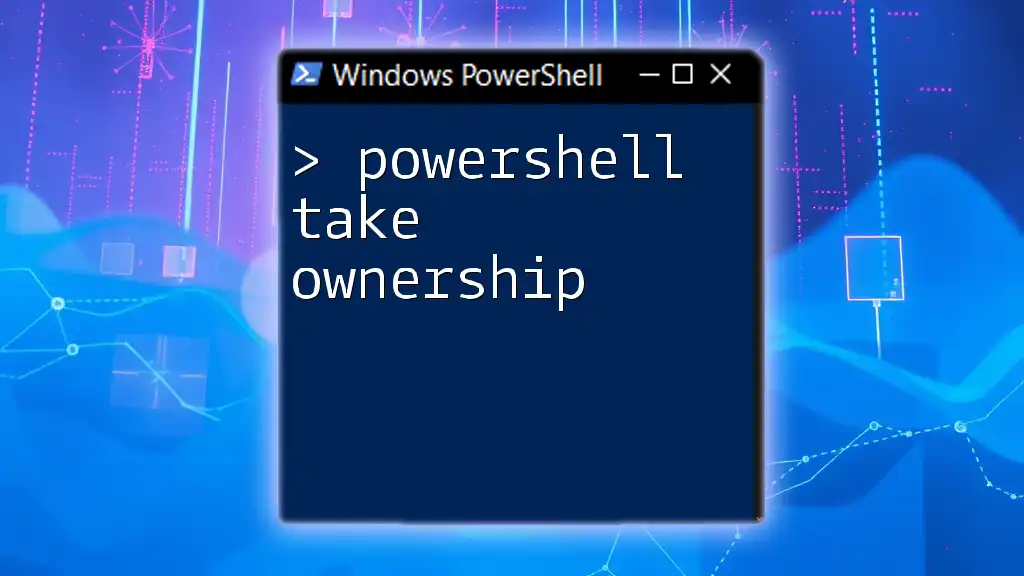
Conclusion
Knowing how to efficiently retrieve file ownership information using PowerShell is a critical skill for system administrators and IT professionals. By leveraging PowerShell commands, you can simplify complex tasks related to file management, enhancing your ability to maintain security and compliance within your organization. Take the time to practice these commands and explore additional functionalities – the learning curve will pay off handsomely in efficiency and control over your file systems.
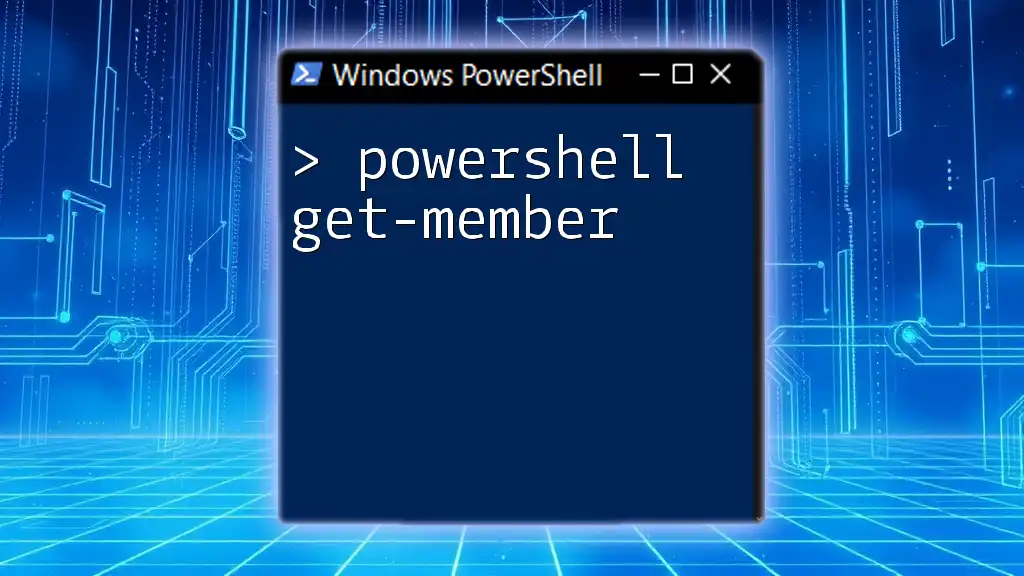
Additional Resources
Further Reading and Learning
For a deeper understanding of PowerShell, consider exploring official PowerShell documentation, available at [Microsoft Docs](https://docs.microsoft.com/powershell/). Additionally, various online courses and books offer expanded learning opportunities.
Community and Support
Engaging with the PowerShell community can provide invaluable support. Consider joining forums such as PowerShell.org or Reddit's r/PowerShell, where you can ask questions, share knowledge, and learn from other PowerShell users.