To take ownership of a file or folder in PowerShell, you can use the following command, which assigns the specified user as the owner of the item.
Takeown /f "C:\Path\To\Your\FileOrFolder" /r /d y
Understanding the `takeown` Command
The `takeown` utility is a powerful built-in command in Windows that allows users to take ownership of files and directories. When using PowerShell, this command can be executed directly to manage file permissions effectively. Ownership is an essential component of file security, permitting the owner to alter permissions and control access to the file.
Benefits of Using PowerShell for Taking Ownership
PowerShell provides several advantages over traditional methods of changing file ownership through the Windows GUI:
- Automation of Tasks: PowerShell scripts can automate repetitive tasks, making it easy to manage file ownership across multiple files and folders without manual intervention.
- Scriptable Solutions: For users dealing with bulk data or migrating files, the ability to write scripts can save time and reduce errors.
- Enhanced Control and Flexibility: PowerShell commands allow for more granular control and detailed modifications that might not be feasible through a graphical interface.
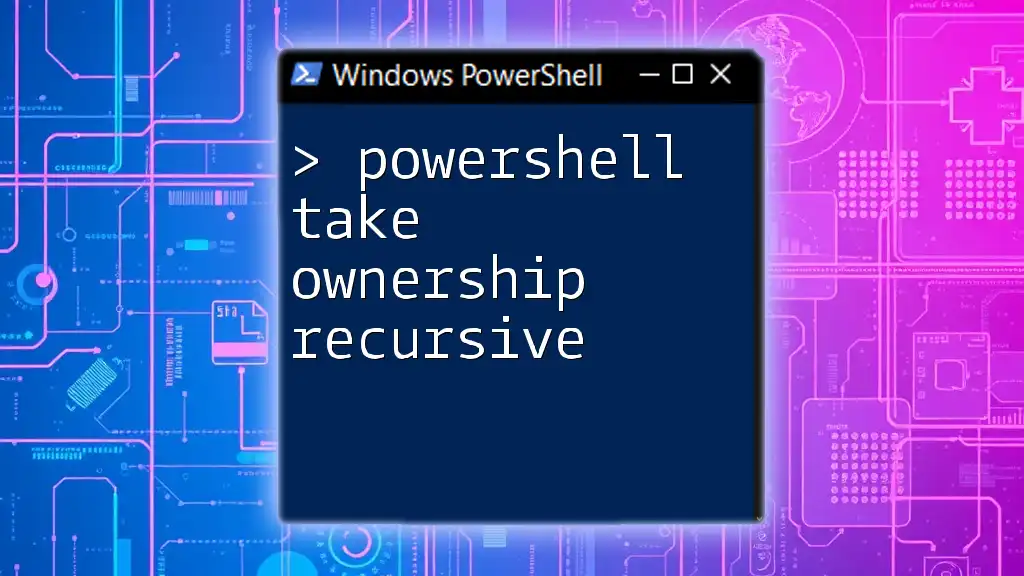
Preparing to Use PowerShell for File Ownership
Before diving into the command line, it’s crucial to prepare adequately:
Checking Your User Permissions
Understanding your current user permissions is essential before attempting to take ownership of files. You can check your permissions in PowerShell by executing the following command:
whoami /groups
This command will display a list of the groups you belong to, along with their associated permissions.
Final Considerations Before Modification
Before making changes to file ownership, consider the following:
- Backup Recommendations: Always ensure that important files are backed up before altering ownership, as accidental changes can result in data loss.
- Risks Associated: Be mindful that taking ownership of certain system files or folders might lead to unexpected behavior in the operating system.
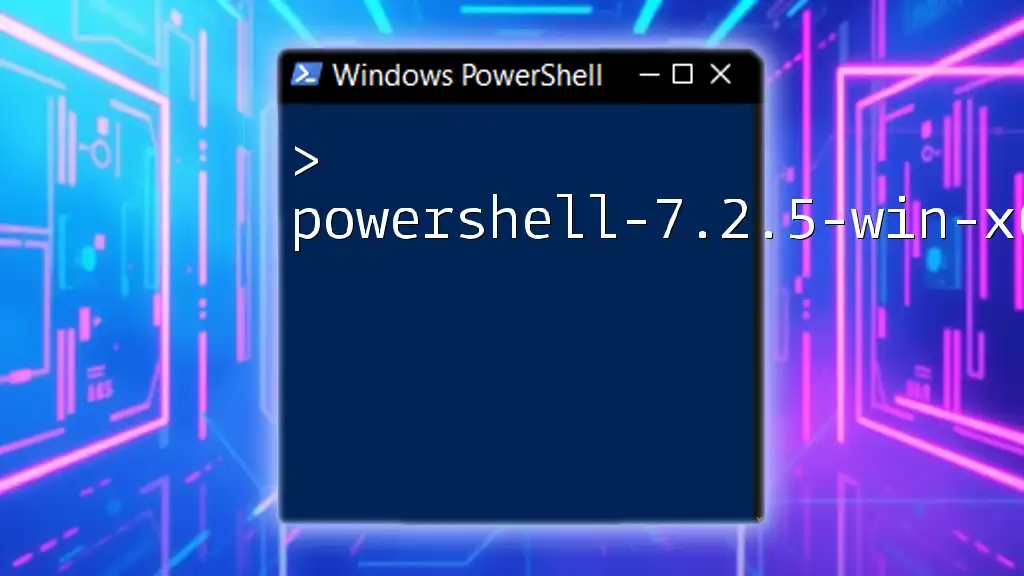
How to Take Ownership of a File Using PowerShell
Using the `takeown` Command
To take ownership of a single file, you can directly use the `takeown` command in PowerShell. Here’s a simple example:
takeown /f "C:\path\to\your\file.txt"
This command grants you ownership of `file.txt`.
Taking Ownership of a Directory
To take ownership of an entire directory, simply add the `/r` switch for a recursive ownership change:
takeown /f "C:\path\to\your\directory" /r
This command processes all files and subdirectories within the specified directory, ensuring comprehensive ownership.
Handling Access Denied Errors
During the ownership process, you may encounter access denied errors. If you do, consider the following troubleshooting steps:
- Run PowerShell as Administrator: Ensure that you are executing the PowerShell session with elevated privileges.
- Check Alternative Ownership Tasks: Sometimes, employing the GUI to change ownership could be an interim solution if PowerShell isn’t functioning as expected.
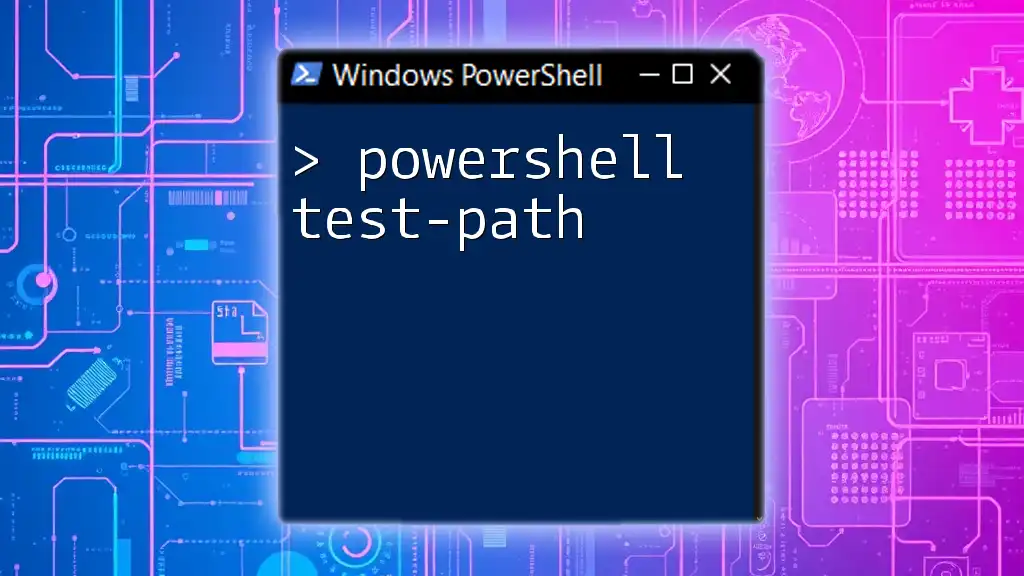
Using PowerShell Cmdlets for Advanced Ownership Management
Understanding `Get-Acl` and `Set-Acl`
Access Control Lists (ACLs) in Windows define the permissions attached to files. PowerShell provides cmdlets `Get-Acl` and `Set-Acl` to manage these permissions.
To view the current permissions of a file, utilize the following command:
Get-Acl "C:\path\to\your\file.txt"
Changing Ownership with `Set-Acl`
To change the ownership of a file using ACLs, you need to first obtain the current ACL and modify it. Here’s a step-by-step example:
-
Retrieve the current ACL:
$acl = Get-Acl "C:\path\to\your\file.txt"
-
Define the new owner. Replace `YourDomain\YourUsername` with the appropriate account name:
$principal = New-Object System.Security.Principal.NTAccount("YourDomain\YourUsername")
-
Set the new owner and apply the changes:
$acl.SetOwner($principal) Set-Acl "C:\path\to\your\file.txt" $acl
This method provides a more comprehensive way to manage ownership and permissions directly within PowerShell.
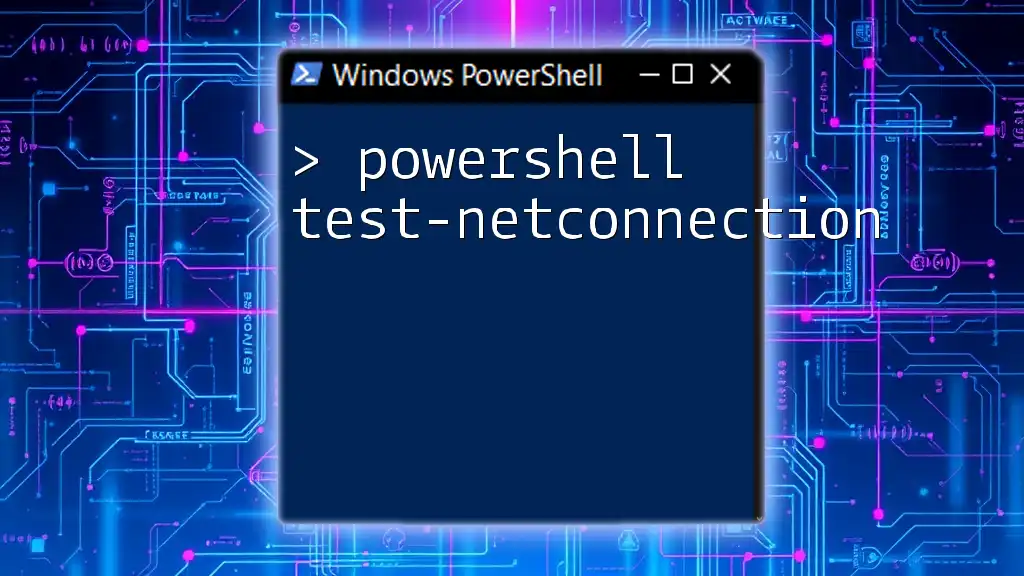
Batch Processing: Taking Ownership of Multiple Files
Using `ForEach-Object` for Bulk Operations
Taking ownership of multiple files can be streamlined using PowerShell's scripting capabilities. You can use the `ForEach-Object` cmdlet to automate this process. For instance, to take ownership of all files within a directory, you can use:
Get-ChildItem "C:\path\to\your\directory" | ForEach-Object { takeown /f $_.FullName }
Common Use Cases for Bulk Ownership Changes
Bulk ownership changes are particularly useful in scenarios like:
- Migrating data from one server to another where old ownership might cause access issues.
- Cleaning out old directories of outdated files, especially during system upgrades or clean sweeps.
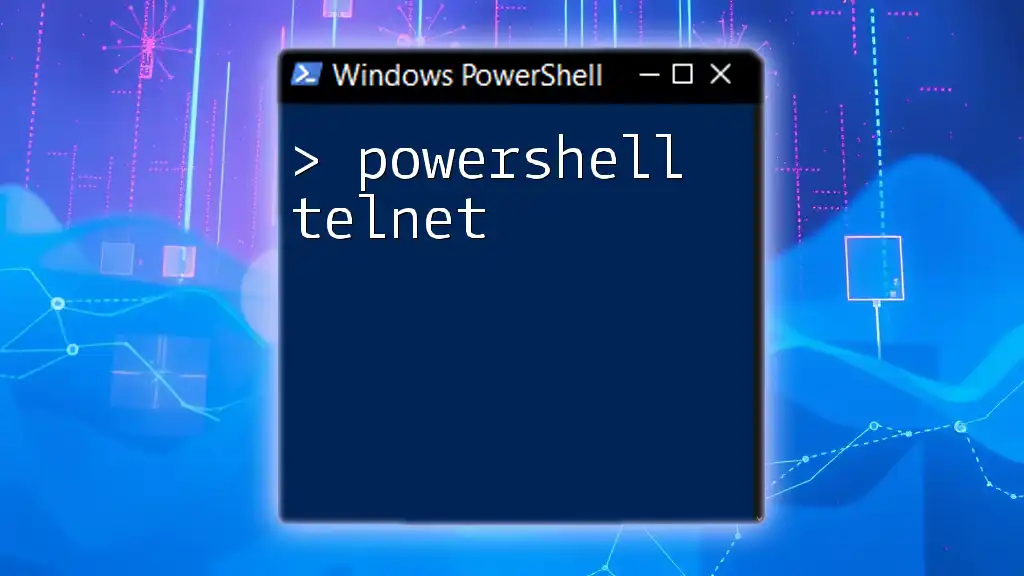
Best Practices for File Ownership Management
To ensure that file ownership management is not merely a one-time task, consider implementing these best practices:
- Regularly Check Ownership: Periodically auditing file and directory ownership can help maintain correct permissions, especially in collaborative environments.
- Use Scripts for Periodic Reviews: Create easy-to-execute scripts that assess and log ownership for important folders.
- Document Changes: Keeping a record of any ownership alterations assists in future audits and compliance verifications.
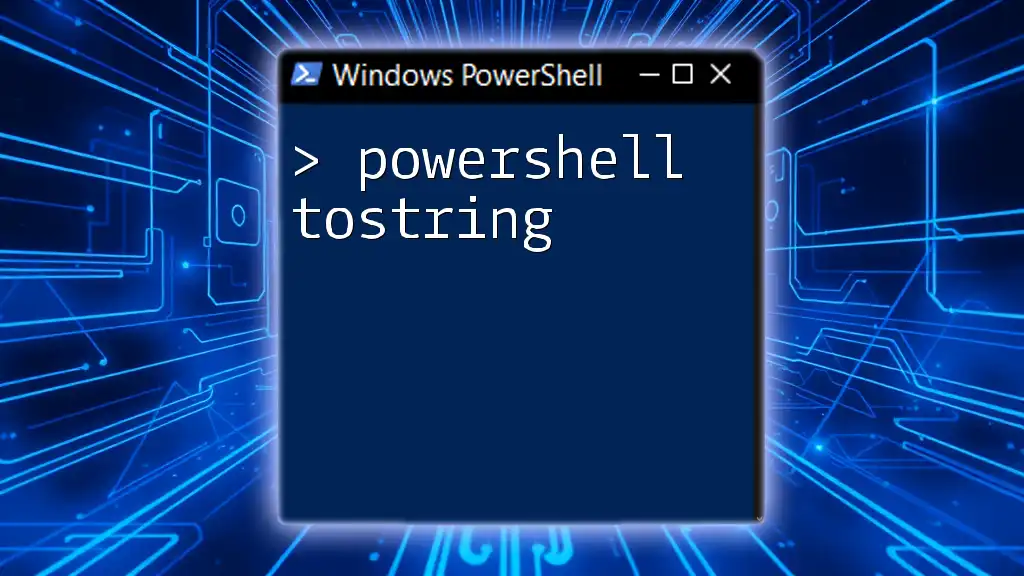
Conclusion
Understanding how to use PowerShell to take ownership of files and directories is an essential skill for managing file security on Windows systems. By utilizing commands like `takeown`, along with ACL manipulation through `Get-Acl` and `Set-Acl`, users can effectively manage file permissions and enhance their overall control over their data.
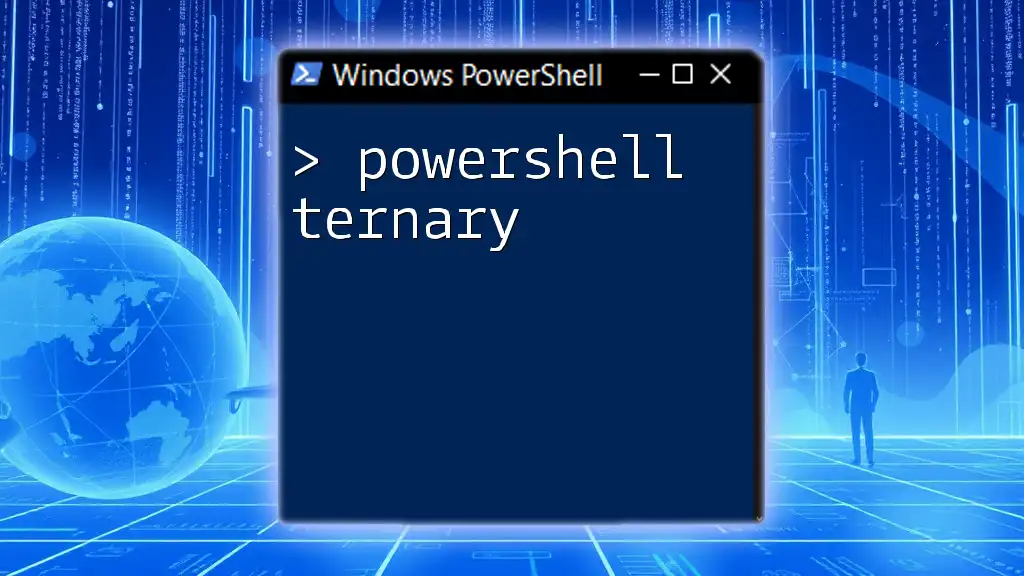
Additional Resources
To further enhance your understanding of file ownership and permissions management through PowerShell, consult the official Microsoft documentation. Community forums, tutorials, and additional reading materials can provide more insights and advanced techniques to streamline your PowerShell practices.