To take ownership of files and folders recursively in PowerShell, you can use the `takeown` command combined with the `-Recurse` parameter to ensure all sub-items are included.
Here's a code snippet to achieve that:
takeown /F "C:\Path\To\Your\Folder" /R /D Y
This command will grant ownership of the specified folder and its contents to the current user.
What is PowerShell Ownership?
Understanding Ownership
In the Windows operating system, ownership is a critical aspect of file and folder management. Ownership determines who has control over a file or folder, which affects both accessibility and the ability to modify contents. The user or group that owns a file can set permissions for others, allowing for granular control over access to sensitive data.
Why Take Ownership?
There are several scenarios where you might find it necessary to take ownership of files or folders. These include:
- Access Denied Errors: When trying to open or modify files and receiving permission errors.
- Migrating Files: During system upgrades or migrations, ownership might need to be reassigned.
- Restoring Deleted Files: Sometimes, ownership must be restored to regain control over documents or data.
By taking ownership, you can ensure that you have the rights necessary for the effective management of files and folders.
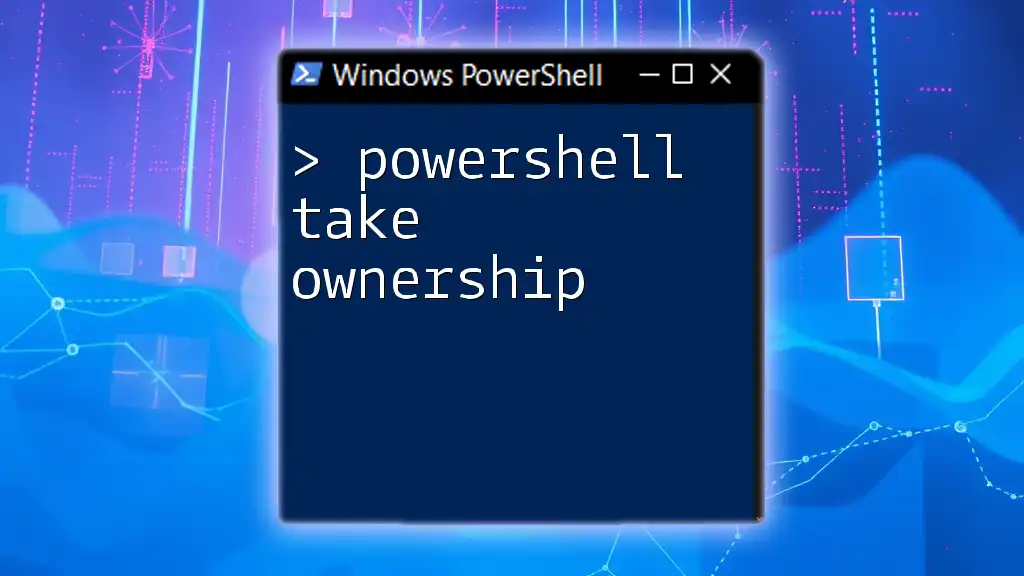
Basics of PowerShell
Introduction to PowerShell Commands
PowerShell is a powerful command-line interface that enables users to automate tasks and manage configurations. It utilizes cmdlets, which are specialized .NET classes built for specific functions, ensuring a streamlined management experience.
Getting Started with PowerShell
To begin using PowerShell:
- Open PowerShell: Click on the Start menu, type "PowerShell," and select the application from the results.
- Execution Policy: Before executing scripts, it’s essential to verify the execution policy using:
If needed, you can change it to allow script running by setting it to RemoteSigned or Unrestricted.Get-ExecutionPolicy
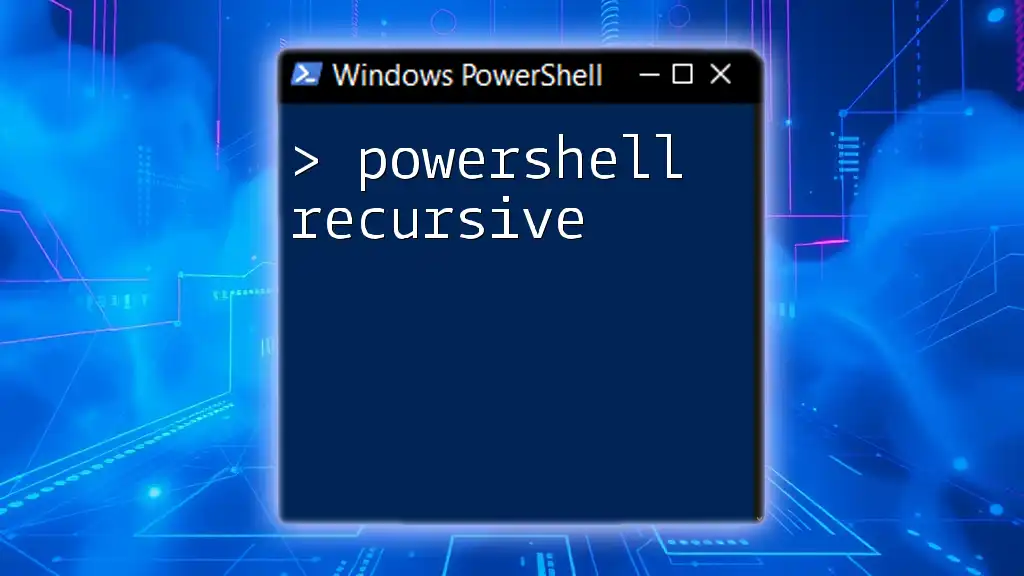
PowerShell Take Ownership Recursive
The `takeown` Command
The `takeown` command is a built-in Windows command-line utility that allows users to take ownership of files and folders. Its basic syntax is as follows:
takeown /f "Path\To\Folder" /r /d y
In this command:
- `/f` specifies the files or folders to take ownership of.
- `/r` indicates that the command should apply recursively to all subfolders and files.
- `/d y` automatically grants ownership without prompting for confirmation.
Using PowerShell to Take Ownership
PowerShell Cmdlet for Ownership Changes
In PowerShell, you can change ownership using the `Set-Acl` and `Get-Acl` cmdlets. These are more flexible than the `takeown` command and allow for scripting and automation.
Example of Using PowerShell to Take Ownership Recursively
To take ownership of a folder recursively, you can utilize the following PowerShell script:
$user = "DOMAIN\Username"
$path = "C:\Path\To\Folder"
$acl = Get-Acl $path
$acl.SetOwner([System.Security.Principal.NTAccount]$user)
Set-Acl $path $acl
In this example:
- You first set the `$user` variable to your desired username.
- The `$path` variable holds the folder path for which you're changing ownership.
- Get-Acl retrieves the current access control list (ACL), and with SetOwner, you modify the owner.
This script effectively changes the ownership of the specified folder.
Common Issues When Taking Ownership
Permissions Issues
When attempting to take ownership, you may encounter various permission issues. One common problem is running PowerShell without administrative privileges. To avoid this, always run PowerShell as an administrator by right-clicking the icon and selecting Run as Administrator.
Troubleshooting Common Take Ownership Errors
Common error messages might display when permissions do not allow ownership change. These can usually be resolved by ensuring you have administrative rights and that no other processes are using the files or directories you're attempting to modify.
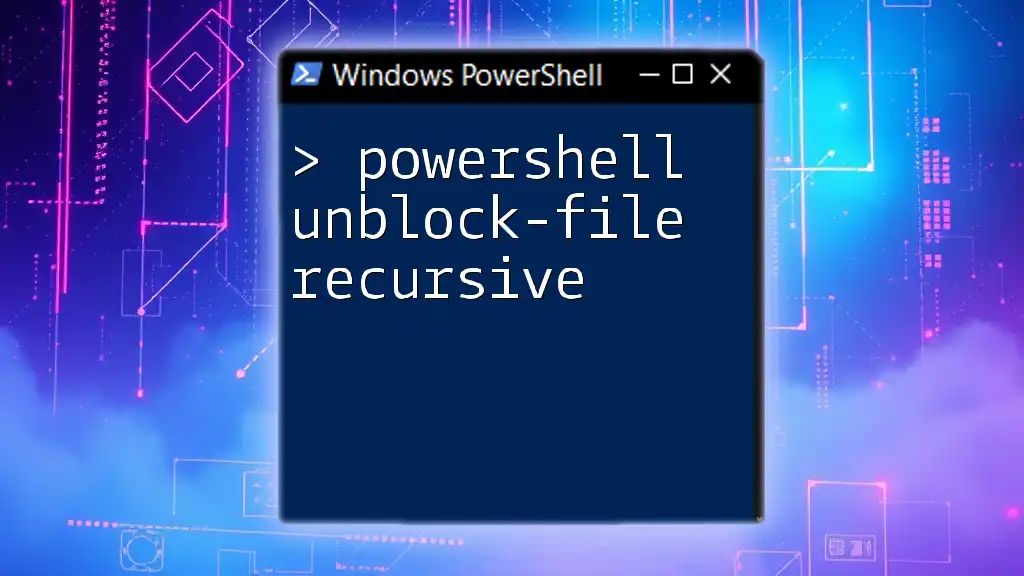
Changing Ownership Recursively
Overview of Recursion
Recursion in this context means that the ownership change applies not just to the main folder but to all its contents, including subfolders and files. This is critical for maintaining consistent access rights throughout an entire directory structure.
Changing Owner of Folder Recursively Using PowerShell
Writing a PowerShell Script for Recursion
Here’s a step-by-step guide for changing the owner of a folder recursively:
- Define the folder path where you want to change ownership.
- Use `Get-ChildItem` to retrieve all child items within that folder.
- Employ a loop to change the owner for each item.
Here’s how the complete script looks:
$folderPath = "C:\Path\To\Folder"
$newOwner = "DOMAIN\Username"
Get-ChildItem -Path $folderPath -Recurse | ForEach-Object {
$acl = Get-Acl $_.FullName
$acl.SetOwner([System.Security.Principal.NTAccount]$newOwner)
Set-Acl $_.FullName $acl
}
In this script:
- Get-ChildItem retrieves all files and folders under the defined `$folderPath` recursively.
- For each item, Get-Acl obtains the current ACL, and SetOwner updates the owner to `$newOwner`.
- Finally, Set-Acl applies the changes.
Best Practices for Changing Ownership
When engaging in ownership changes, adhere to these best practices:
- Backup Critical Data: Before making ownership changes, ensure that you have backups of any important data in case of unexpected issues.
- Document Changes: Keep a log of ownership modifications to track who has access to certain files or folders.
- Limit Administrative Use: Only use administrative rights when absolutely necessary, as this reduces the risk of unintended modifications.
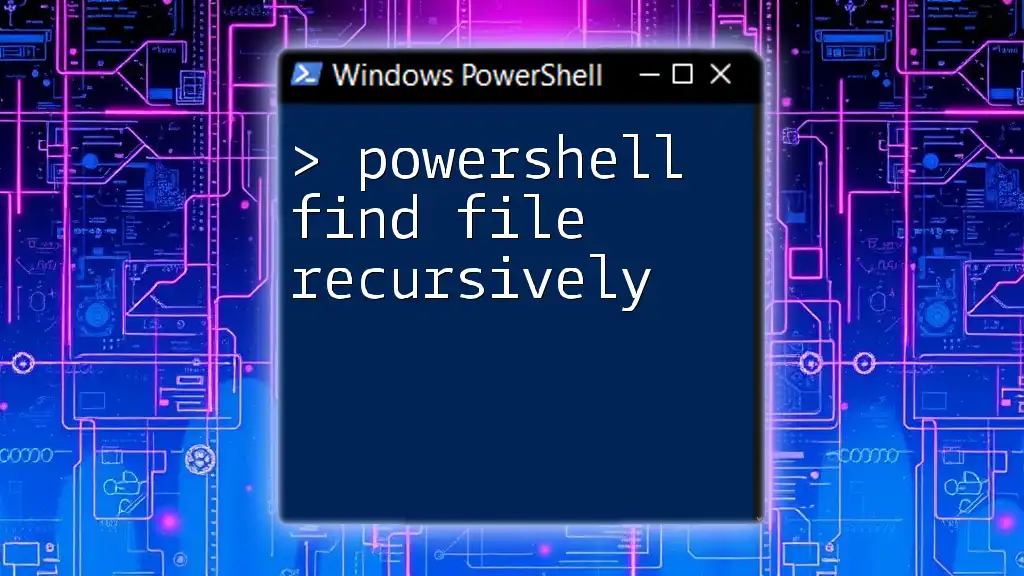
Conclusion
Taking ownership of files and folders using PowerShell is an essential skill for system administrators and power users alike. By understanding the methods described above—including the `takeown` command and the use of PowerShell's `Set-Acl` and `Get-Acl` cmdlets—you can efficiently manage file and folder permissions in a recursive manner.
Practice these commands and scripts in a controlled environment to reinforce your skills and ensure you're fully equipped to manage file ownership effectively. Start exploring more PowerShell resources to enhance your capabilities further!
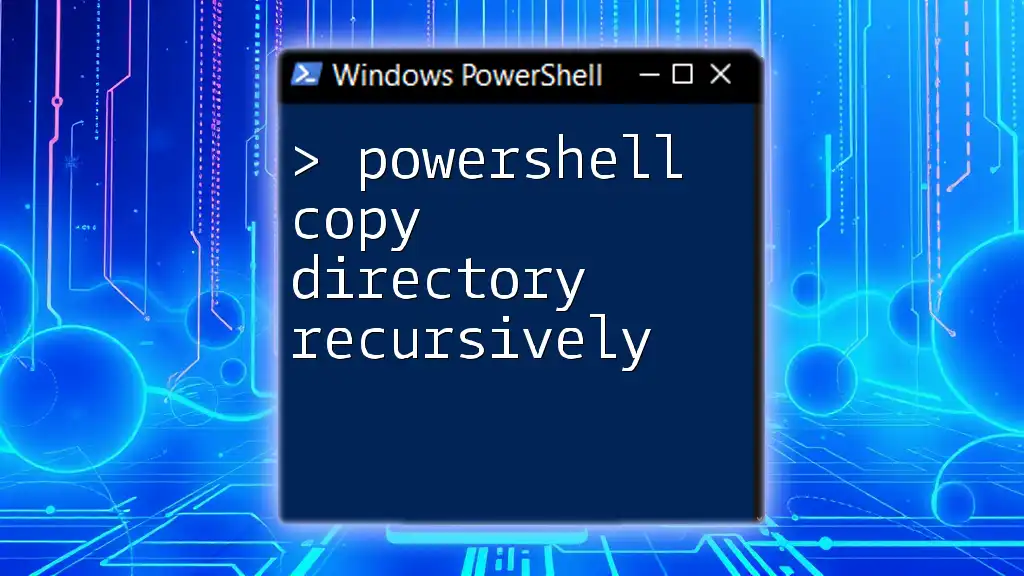
Additional Resources
Recommended PowerShell Resources
- Official Microsoft PowerShell Documentation
- PowerShell Books: "Learn Windows PowerShell in a Month of Lunches"
- Online courses on platforms like Coursera, Udemy, or Pluralsight
FAQs about PowerShell Ownership
Feel free to explore common questions relating to file ownership and how to manage these effectively using PowerShell commands!