The `ToString()` method in PowerShell is used to convert an object to its string representation, which can be particularly useful for displaying complex objects in a readable format.
$number = 123
$stringRepresentation = $number.ToString()
Write-Host $stringRepresentation
What is `ConvertTo-String`?
`ConvertTo-String` is a command in PowerShell that is used to convert objects into their string representations. This command plays a vital role in scripting, primarily because it allows developers to take complex objects, such as arrays or custom objects, and represent them in a human-readable format. Understanding the difference between string representations and the original object is crucial; while the original object retains its structure and properties, the string representation simplifies this data for easier interpretation.
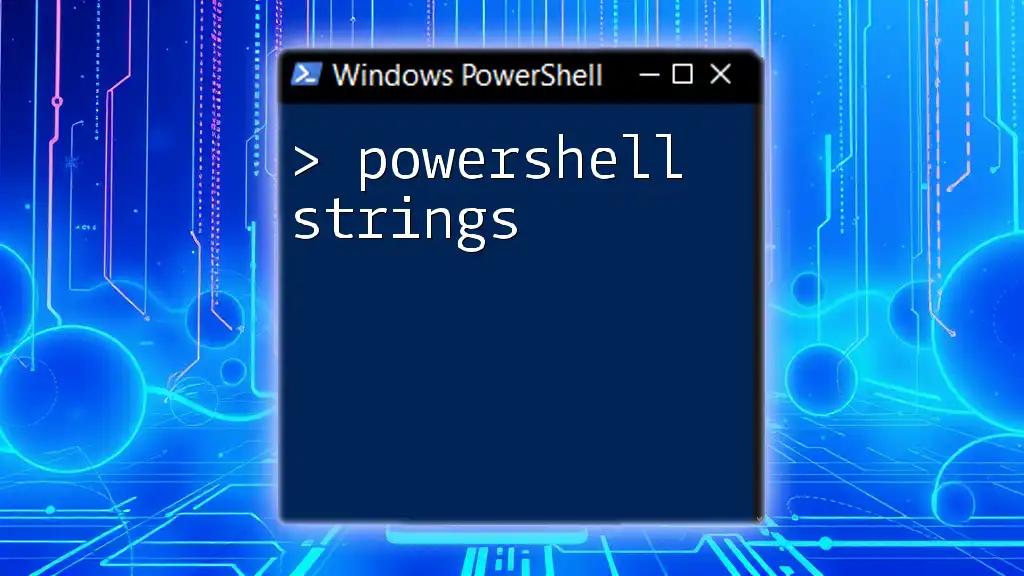
Why Use `ConvertTo-String`?
Benefits of Converting Objects to Strings
Easy Readability: Converting objects to strings enhances the readability of the output. For instance, if you're working with an object that contains multiple properties, turning that object into a string can clarify its contents and avoid overwhelming details.
Data Handling: Strings are often more manageable for data storage and transfer. Whether you're writing to a log file or passing strings between functions, the conversion process simplifies interactions with data.
Debugging Aid: During troubleshooting, visualizing the current state or value of an object as a string can provide instant insight. When a script produces unexpected results, `ConvertTo-String` can be a powerful tool for debugging.
Practical Applications
Logging: Strings are essential in logging mechanisms. When logging events or errors, converting objects to strings allows for smoother logging, where the logs can be human-readable and easily searchable.
Exporting Data: If you need to export data to formats like CSV or JSON, converting your objects into strings first can ensure formatting is uniform and precise. This minimizes errors during the export process, leading to a smoother workflow.
Formatting Output: When running scripts or commands interactively, presenting output as strings makes it easier to read and understand the command results. This transformation is vital for enhancing user interfaces in command-line tools.
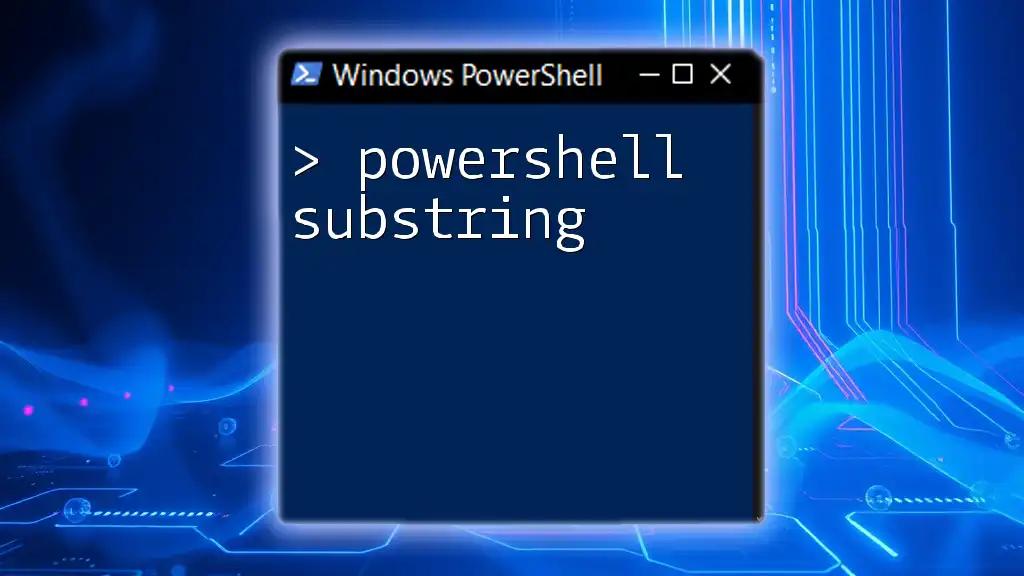
Syntax of `ConvertTo-String`
Basic Syntax
The basic syntax for `ConvertTo-String` is as follows:
ConvertTo-String [-InputObject] <PSObject> [[-Stream] <String[]>] [-Separator <String>] [<CommonParameters>]
Parameters Explained
-
InputObject: This parameter is essential, as it specifies the object you wish to convert to a string. For example, you might input an array or any other structure without worrying about its complexity.
-
Stream: This optional parameter allows you to define streams for the output. You might implement different streams to categorize parts of your output, particularly in more complex scripts.
-
Separator: Control how the output is formatted by using the separator parameter. Specifying a custom string as a separator can help format the output for better readability.
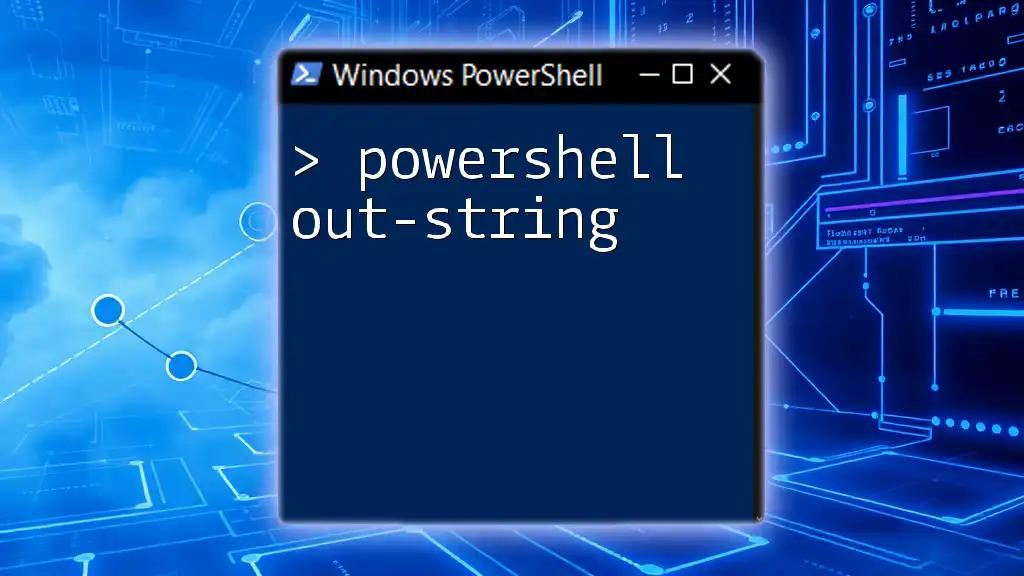
How to Use `ConvertTo-String`
Basic Example
A straightforward example of converting a basic string is as follows:
"Hello, World!" | ConvertTo-String
Converting Complex Objects
In the case of more complex objects, such as an array, the conversion can still be seamless:
$array = @("Item1", "Item2", "Item3")
$array | ConvertTo-String
This command takes the array and converts it into a string representation, outputting "Item1 Item2 Item3" (based on the default format).
Using Streams
To demonstrate the power of streams, consider the following example:
$object = Get-Process
$object | ConvertTo-String -Stream "Process Details"
In this scenario, `ConvertTo-String` is used on the output of `Get-Process`, categorizing parts of the output with a defined stream name.
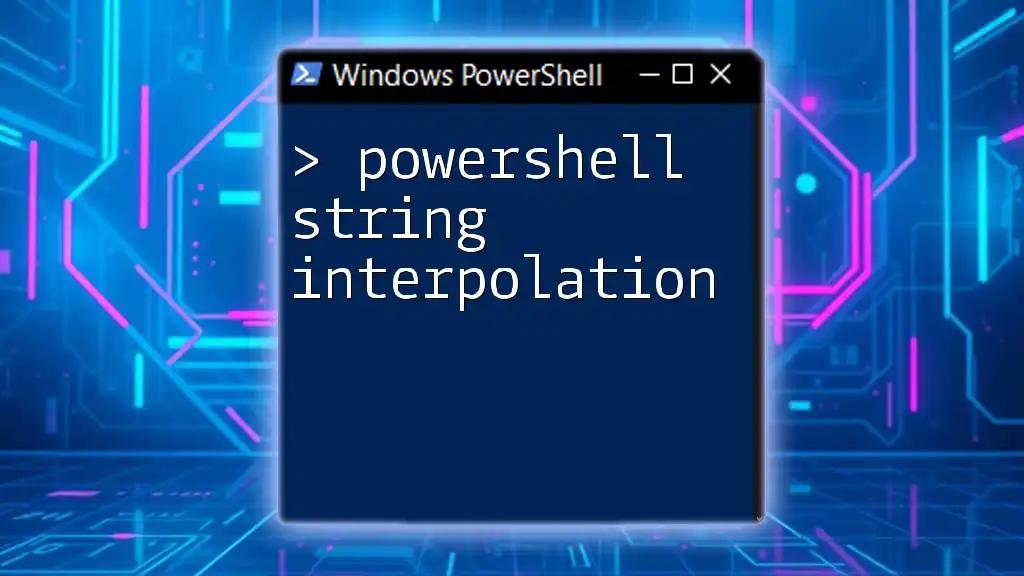
Tips for Effective Usage of `ConvertTo-String`
Best Practices
-
Clarity in Naming Streams: When using streams, clear and descriptive names make it easier to understand the purpose of the output. This makes your scripts more maintainable and understandable for other users.
-
Output Formatting: When you employ separators or streams, ensure that you're using them to enhance clarity. Avoid overcomplicating the output; the goal is to make it easier to interpret.
Common Pitfalls
While using `ConvertTo-String`, one common mistake involves data loss during conversion. This can occur when users expect a complex object to retain its structure after conversion.
Here’s a brief look at an example of what not to do:
$array = @(1, 2, 3)
$string = $array | ConvertTo-String -Separator ';'
In this situation, while you’re attempting to join the numbers with a semicolon, the resulting output may not meet your formatting expectations. Be cautious with complex object conversions, as it’s important to verify the results.

Comparison with Other Conversion Methods
`Out-String`
Unlike `ConvertTo-String`, which focuses on creating string representations for further utilization, `Out-String` directs the output to the console or another stream. For example, if you want to display the contents of an array in the console without converting it into a string format, `Out-String` may be more suitable.
`ToString()` Method
When it comes to using the `ToString()` method, this is useful for a specific single object. For instance:
$object = Get-Process | Select-Object -First 1
$object.ToString()
This will output the string representation of that specific object but won't provide the broader functionality available in `ConvertTo-String`.

Real-World Scenarios
Example Scenario: Logging User Actions
In a scenario where you need to log user actions, utilizing `ConvertTo-String` can streamline the process. Here's a hypothetical snippet:
$userAction = @{ User = "john_doe"; Action = "Logged In"; Time = Get-Date }
$userAction | ConvertTo-String -Separator ': '
The resulting log string can then be written directly to a log file, making record-keeping clear and efficient.
Example Scenario: Generating Reports
When generating reports from complex objects, string conversion becomes a powerful ally. For instance, you could convert a report from various user details into a clear string format for easier visualization or export:
$users = Get-Content "users.txt"
$users | ConvertTo-String -Separator ", "
The formatted string output can be utilized in generating reports, where the results are both organized and presentable.

Conclusion
In summary, using `ConvertTo-String` in PowerShell is a fundamental skill for anyone looking to manage and manipulate data effectively. By converting objects to their string representations, you can enhance readability, troubleshoot issues, and manage data more efficiently. Practicing with this command in your scripts will not only develop your skills but also improve the overall quality of your scripting outputs.
Engage with your scripts and share your experiences with converting objects; understanding these concepts will elevate your PowerShell proficiency tremendously.

Additional Resources
For more in-depth exploration, consult the official PowerShell documentation, and consider enrolling in online courses or reading technical books that focus on PowerShell scripting and best practices.

FAQs
What objects can be converted using `ConvertTo-String`?
`ConvertTo-String` supports a wide variety of objects, including but not limited to strings, arrays, lists, and custom objects. As long as the object can be represented as a string, you will find that this command applies effectively.
How do I handle errors when using `ConvertTo-String`?
To troubleshoot common errors during conversion, ensure your input object is not null and that it exists in the context where you're running the command. A simple check before conversion can significantly reduce potential pitfalls.
Can I customize the output format when using `ConvertTo-String`?
Yes, you can customize the output using the `-Separator` parameter. By defining how different elements in your object are separated within the final string, you can tailor the format to meet your requirements effectively.