PowerShell can be effectively utilized to interact with PostgreSQL databases, allowing users to execute commands for managing data directly from the PowerShell console.
Here’s a simple code snippet to connect to a PostgreSQL database and execute a query:
# Load PostgreSQL .NET library
Add-Type -AssemblyName "Npgsql"
# Connection string
$connectionString = "Host=your_server;Username=your_user;Password=your_password;Database=your_database"
# Create a connection to the database
$connection = New-Object Npgsql.NpgsqlConnection($connectionString)
$connection.Open()
# Execute a simple SQL query
$command = $connection.CreateCommand()
$command.CommandText = "SELECT * FROM your_table"
$reader = $command.ExecuteReader()
# Read and display results
while ($reader.Read()) {
Write-Host $reader[0] # Adjust index according to the desired column
}
# Clean up
$reader.Close()
$connection.Close()
Setting Up Your Environment
Installing PostgreSQL
Before integrating PowerShell with PostgreSQL, the first step is to ensure PostgreSQL is correctly installed on your machine. You can download the latest version of PostgreSQL from the official website. Follow the installation wizard, making sure to select the appropriate components, including the PostgreSQL Server and pgAdmin.
Compatibility Considerations:
For Windows users, ensure that you select the appropriate architecture (32-bit or 64-bit) based on your operating system. Additionally, remember to configure the PostgreSQL server to start automatically with Windows, ensuring it is always available for your queries.
Installing PowerShell
PowerShell is essential for scripting and automation based tasks. You may be using Windows PowerShell or PowerShell Core, depending on your system and needs. To verify if PowerShell is installed, simply search for it in the start menu.
Updating to the Latest Version:
If you are using PowerShell prior to Version 7, consider upgrading to the latest version. Visit the official PowerShell GitHub page for installation packages.
Installing PostgreSQL PowerShell Module
To ease the integration between PowerShell and PostgreSQL, it's highly recommended to use a dedicated module, such as Npgsql. This .NET data provider for PostgreSQL allows you to use PostgreSQL features in your PowerShell scripts.
Command to Install:
Install-Package Npgsql -Source NuGet
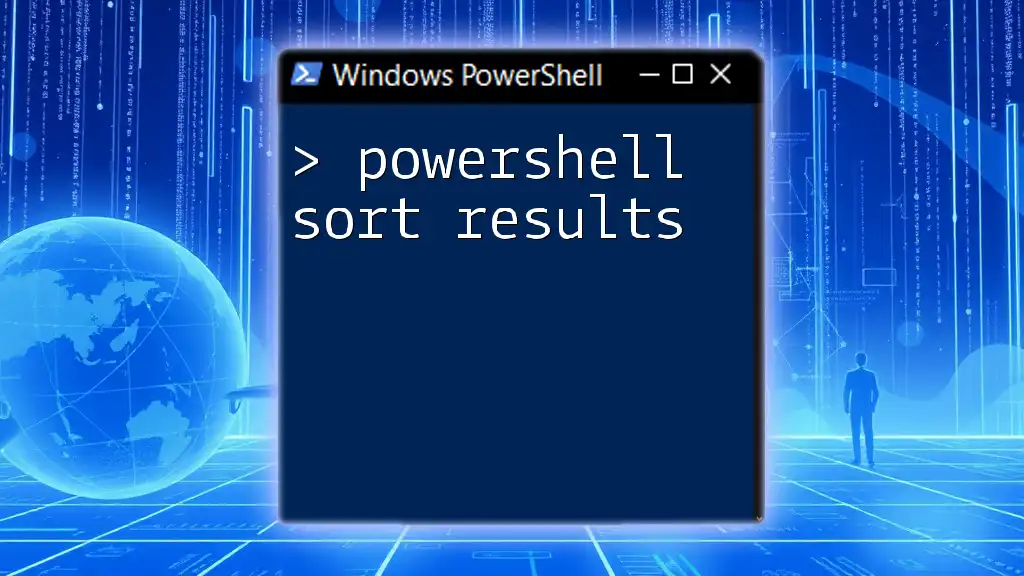
Connecting PowerShell to PostgreSQL
Configuring Connection Strings
A connection string is crucial for establishing a connection between PowerShell and your PostgreSQL database. A typical connection string contains essential details, such as host address, port, username, password, and database name.
Establishing a Connection
Once you've configured your connection string, you can establish a connection to your PostgreSQL database using PowerShell. Here’s an example:
$connectionString = "Host=localhost;Port=5432;Username=myusername;Password=mypassword;Database=mydatabase;"
$connection = New-Object Npgsql.NpgsqlConnection($connectionString)
$connection.Open()
In this command:
- Host specifies the server where PostgreSQL runs (localhost in this case).
- Port is the PostgreSQL default port (5432).
- Username and Password are your database credentials.
- Database is the name of the PostgreSQL database you wish to connect to.
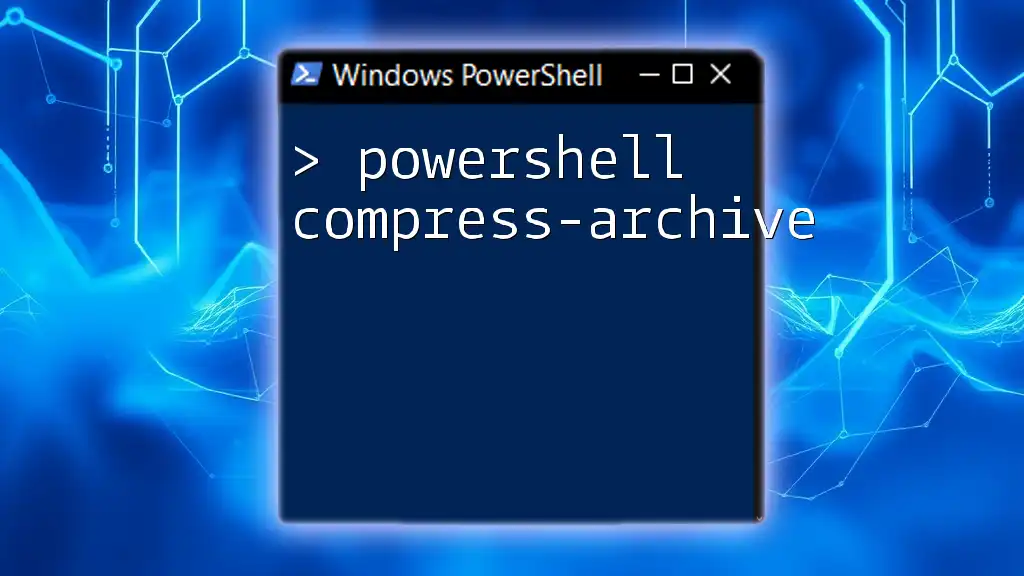
Executing SQL Commands via PowerShell
Running Basic SQL Queries
With a successful connection, you can execute SQL commands directly from PowerShell. For example, to run a simple SELECT query, you can create a command as shown below:
$command = $connection.CreateCommand()
$command.CommandText = "SELECT * FROM mytable;"
$reader = $command.ExecuteReader()
Once the command is executed, you can iterate through the results using the following snippet:
while ($reader.Read()) {
Write-Output $reader["column1"]
}
This allows you to access and display the data retrieved from your specified table.
Inserting Data
To add new rows to your table, you can use an INSERT command in PowerShell. Here’s how you can do it:
$command.CommandText = "INSERT INTO mytable (column1, column2) VALUES ('value1', 'value2');"
$command.ExecuteNonQuery()
This command inserts values into the specified columns of your table. Always ensure the data types match those defined in your PostgreSQL table schema.
Updating Data
If you need to modify existing data, an UPDATE command can be utilized. Here’s an example:
$command.CommandText = "UPDATE mytable SET column1 = 'newvalue' WHERE condition;"
$command.ExecuteNonQuery()
This command updates the values in `column1` based on the specified condition.
Deleting Data
To remove records from your table, you can use a DELETE command as follows:
$command.CommandText = "DELETE FROM mytable WHERE condition;"
$command.ExecuteNonQuery()
This will delete all rows that meet the specified condition.
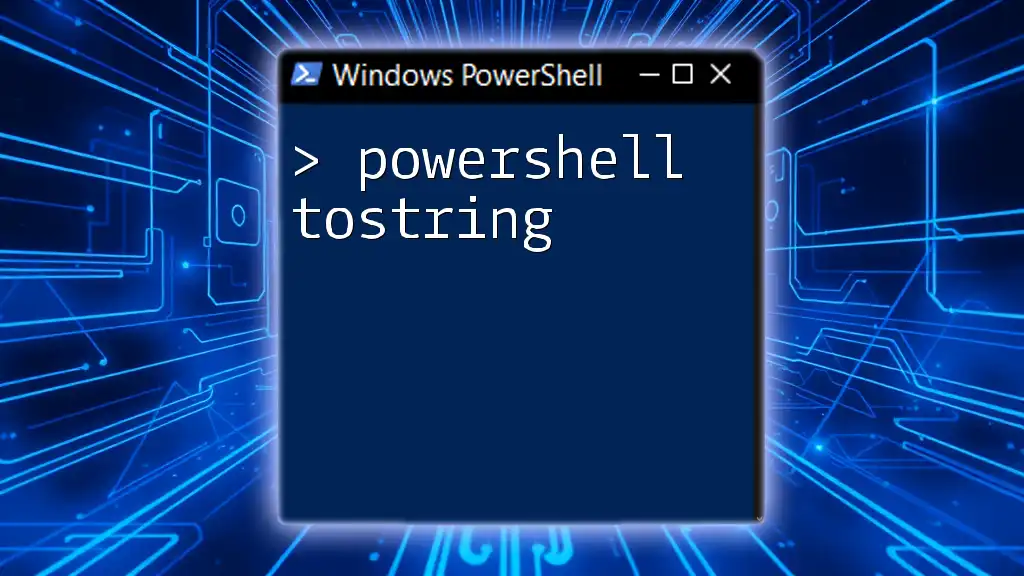
Handling Transactions
Understanding Transactions
In database management, a transaction ensures that a set of commands either fully complete or leave the database intact in case of an error. This prevents any partial updates that could cause data inconsistency.
Implementing Transactions in PowerShell
Using transactions is straightforward in PowerShell. Here's an example of how to use them effectively:
$transaction = $connection.BeginTransaction()
$command.Transaction = $transaction
try {
# Execute several commands
$command.ExecuteNonQuery()
$transaction.Commit()
} catch {
$transaction.Rollback()
}
In this code, a transaction is initiated, and if all commands execute successfully, a commit is performed. If there’s any error, a rollback restores the database to its previous state.
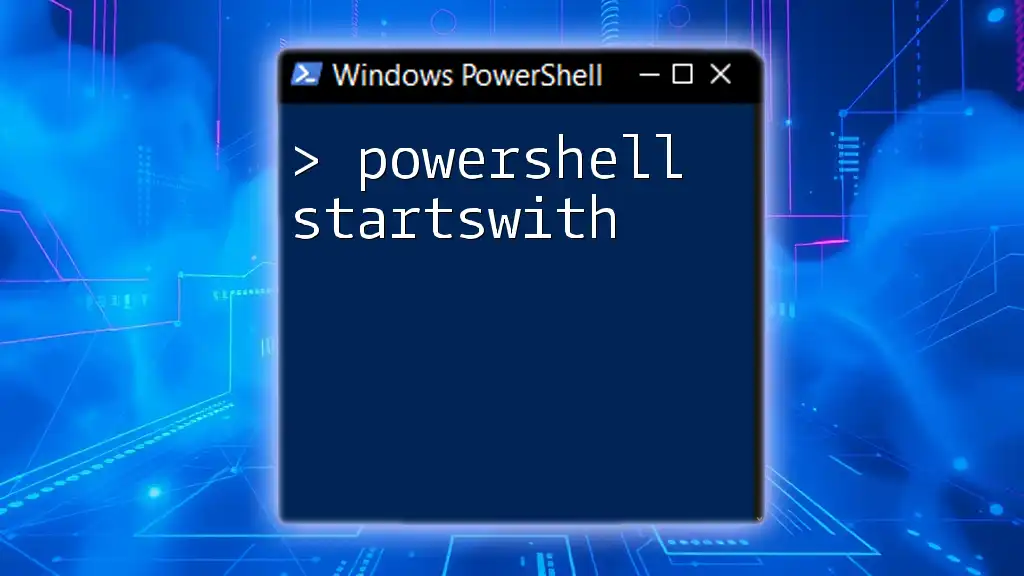
Exporting Data from PostgreSQL to Files
Using PowerShell to Export Data
Exporting data from PostgreSQL can be done conveniently using PowerShell. One effective way is utilizing the COPY command to export data to a CSV file:
$exportCommand = "COPY (SELECT * FROM mytable) TO '/path/to/file.csv' DELIMITER ',' CSV HEADER;"
$command.CommandText = $exportCommand
$command.ExecuteNonQuery()
This command extracts all records from `mytable` and saves them in a specified CSV file with headers included.
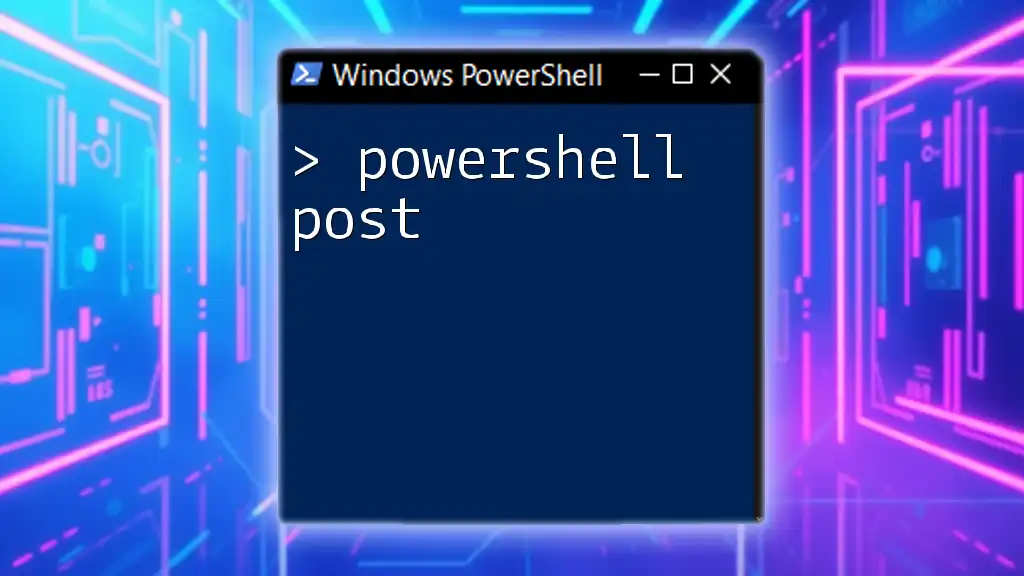
Importing Data into PostgreSQL from Files
Importing Data using PowerShell
Similarly, you can import data into PostgreSQL using the COPY command. Here’s how you can do it:
$importCommand = "COPY mytable FROM '/path/to/file.csv' DELIMITER ',' CSV HEADER;"
$command.CommandText = $importCommand
$command.ExecuteNonQuery()
Ensure that the CSV file adheres to the data types defined in your database schema to avoid any import errors.
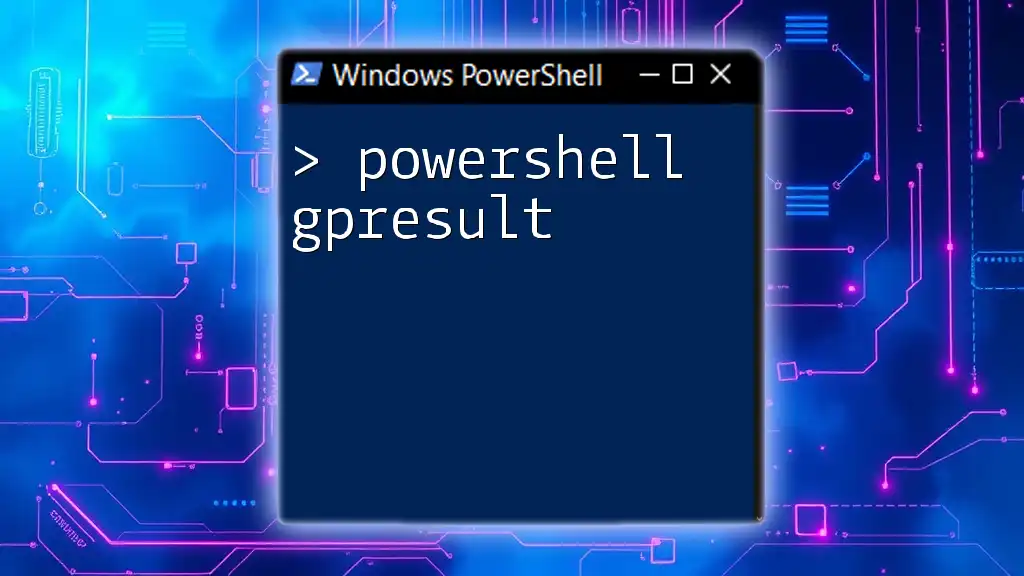
Troubleshooting Common Issues
Best Practices in PowerShell and PostgreSQL
Working with databases often leads to a variety of issues. It’s crucial to take certain precautions:
- Connection Issues: Double-check your connection string and ensure PostgreSQL service is running.
- Data Type Mismatches: Be attentive to the data types specified in your table to avoid runtime errors.
- Permissions Errors: Ensure your PostgreSQL user has the appropriate permissions for the operations you intend to execute.
Debugging Techniques
To effectively troubleshoot issues, utilize PowerShell’s verbose logging feature:
$VerbosePreference = "Continue"
This command will allow you to see detailed error messages, helping you pinpoint the problem area when the script runs.
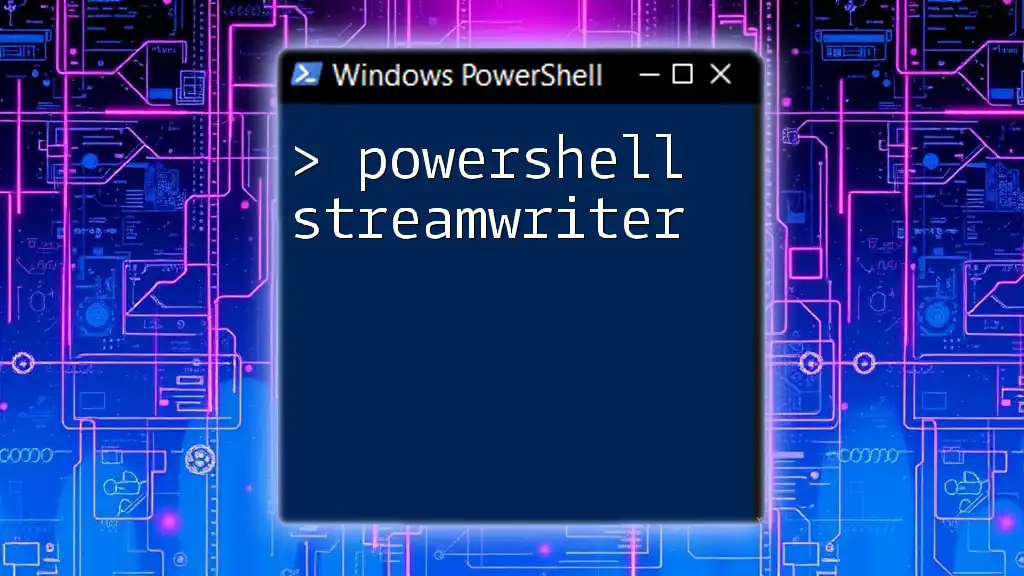
Conclusion
Integrating PowerShell with PostgreSQL opens up a wide array of possibilities for database management. It enables efficient execution of commands, transaction management, and data handling, significantly enhancing your workflow. By following the steps outlined in this guide, you can now harness the power of PowerShell in your PostgreSQL endeavors.
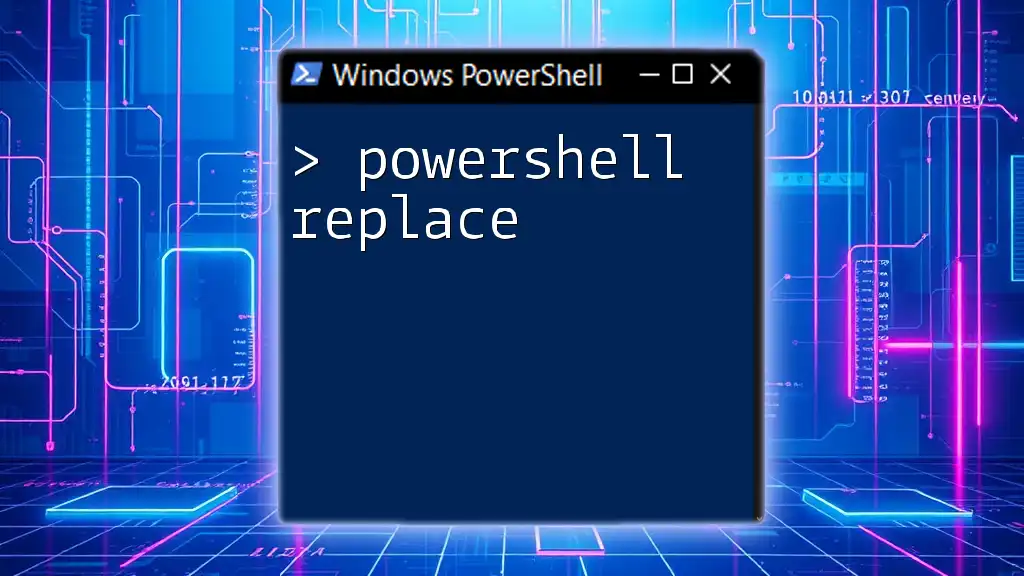
Additional Resources
For more information and guidance, consider exploring the official documentation for both PowerShell and PostgreSQL. Engaging with community forums and support channels can also provide additional insight and shared experiences from other users, further enriching your knowledge.