In PowerShell, you can sort results from a command by using the `Sort-Object` cmdlet, which organizes data in ascending or descending order based on specified properties.
Here's a simple code snippet to sort a list of numbers in ascending order:
1..10 | Sort-Object
Understanding Sorting in PowerShell
What is Sorting?
Sorting is the process of arranging data in a specific order based on certain criteria, which could be alphabetical, numerical, or based on other defining characteristics. In PowerShell, sorting enables users to organize output data for better readability and analysis. Whether you are working with system information, logs, or any dataset, employing sort operations can make it easier to extract meaningful insights.
Key Benefits of Sorting Results
Sorting results in PowerShell offers several advantages:
-
Improved Readability of Data: Organized data is easier to read and interpret. For instance, viewing a list of processes sorted by CPU usage can help you quickly identify which processes are consuming the most resources.
-
Efficient Data Management: When data is sorted, it can be more easily managed and manipulated for further analysis or reporting.
-
Enhanced Capability for Subsequent Analysis: Sorted data serves as a foundational aspect for functions like filtering or grouping, ensuring analyses are conducted on structured datasets.
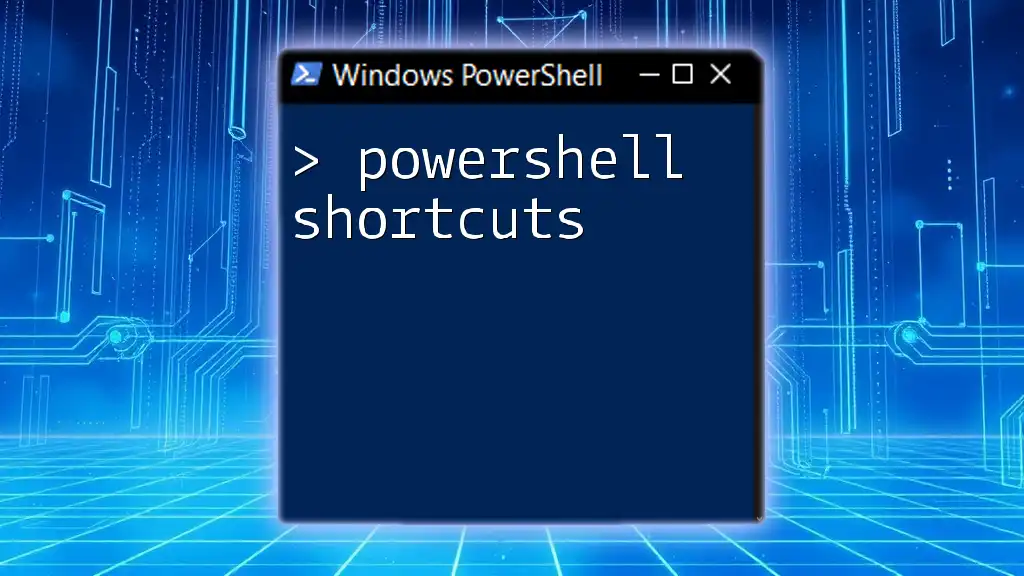
PowerShell Sorting Basics
The `Sort-Object` Cmdlet
The primary tool for sorting in PowerShell is the `Sort-Object` cmdlet. Its straightforward syntax allows users to sort collections of objects by one or more properties.
Here is the basic syntax:
Sort-Object [-Property] <string[]> [-Descending] [-InputObject <psobject>]
To illustrate, let’s consider a simple example that sorts running processes by CPU usage:
Get-Process | Sort-Object CPU
In this example, `Get-Process` retrieves a list of all currently running processes, and `Sort-Object CPU` arranges these processes in ascending order based on the CPU usage.
Sorting by Multiple Properties
You can also sort results by multiple columns, which is particularly useful when you want to sort data hierarchically.
For example, to sort services by their status and then by name:
Get-Service | Sort-Object Status, Name
In this command, services are first sorted by their `Status` (like Running or Stopped), and then within each status group, they are ordered alphabetically by `Name`.
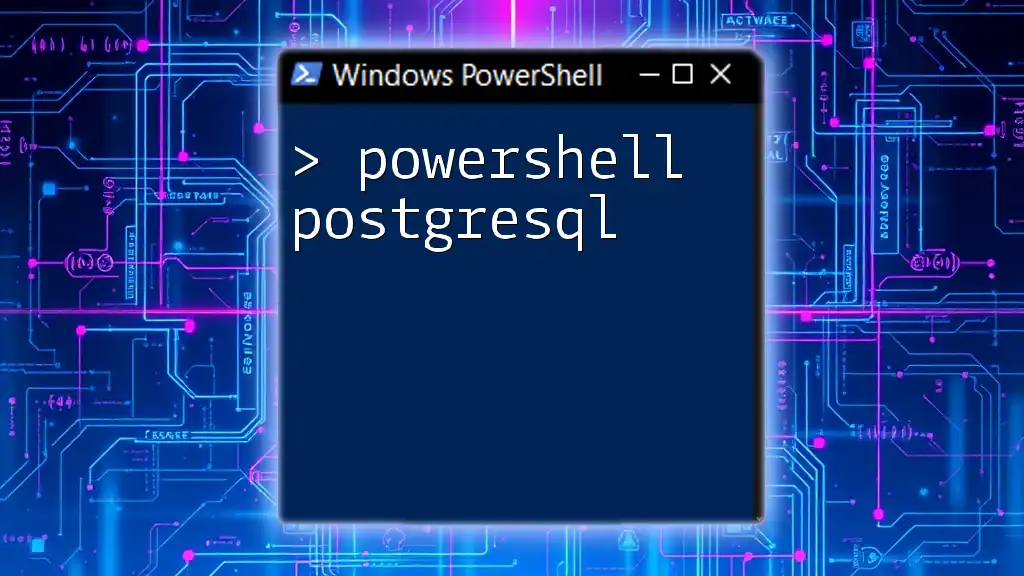
Advanced Sorting Techniques
Sorting in Descending Order
Sometimes, you may need to display results in descending order to highlight the top entries quickly. This can be achieved by adding the `-Descending` parameter.
Let’s consider an example that sorts processes by CPU usage in descending order:
Get-Process | Sort-Object CPU -Descending
Here, the processes consuming the most CPU resources will appear at the top of the list. Using descending sorting is particularly beneficial when working with large datasets, as it allows you to quickly identify significant data points.
Custom Sorting with ScriptBlocks
What are ScriptBlocks?
ScriptBlocks in PowerShell allow you to provide a custom sorting logic through a block of code. This is particularly powerful when the sorting criterion is not limited to straightforward properties.
Here's how you can sort files by the length of their names:
Get-ChildItem | Sort-Object { $_.Name.Length }
In this command, `Sort-Object` uses a ScriptBlock to determine the sorting order based on the length of each file's name. This flexibility enables highly customized sorting scenarios tailored to specific needs.
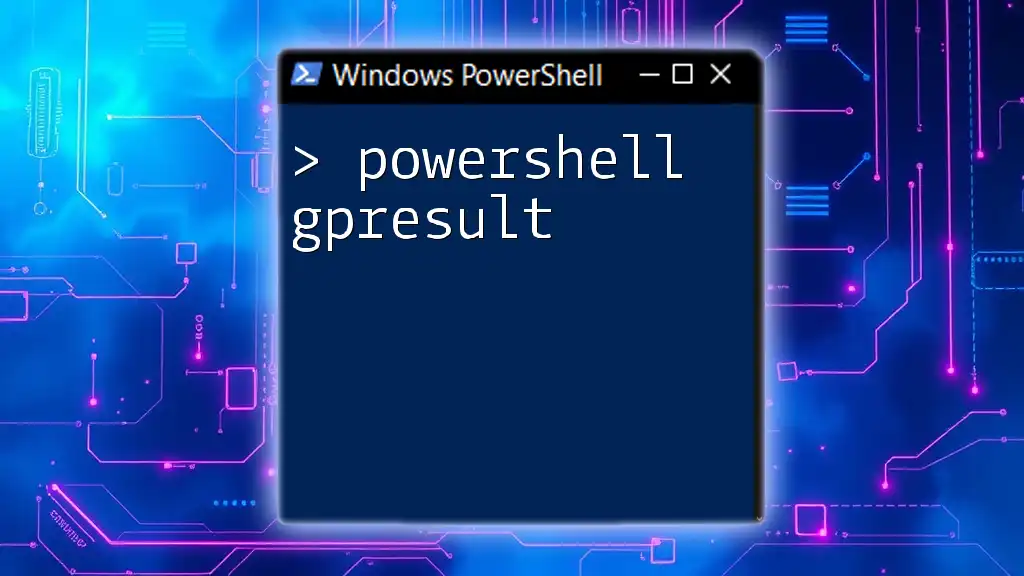
Practical Applications of Sorting
Sorting Data from CSV Files
Handling CSV files is a common practice when working with data in PowerShell. Sorting can enhance the insights derived from this data.
To import a CSV file and sort the entries by age, you could use:
Import-Csv "data.csv" | Sort-Object Age
This command imports data from "data.csv" and sorts it based on the `Age` column. This method is essential when analyzing datasets, as sorting helps identify patterns or outliers.
Sorting Network Information
For those managing network connections, sorting can be invaluable. You may want to sort connections for better visibility into the network's state.
For example:
Get-NetTCPConnection | Sort-Object LocalAddress, LocalPort
This command gathers TCP connection details and sorts by both `LocalAddress` and `LocalPort`, making it easier to assess active connections based on their endpoints.
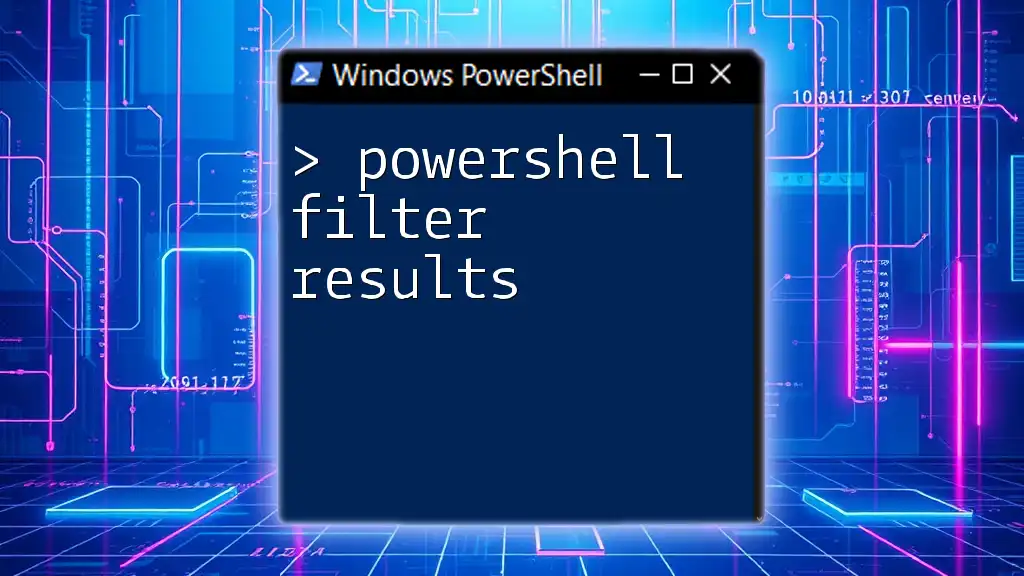
Optimizing Sorting Performance
Considerations for Large Datasets
Sorting can become resource-intensive, especially with large datasets. Considerations for performance include:
- Filtering Before Sorting: Always filter your dataset before sorting to reduce the number of objects being processed. For instance:
Get-EventLog -LogName Security | Where-Object { $_.EntryType -eq 'Failure' } | Sort-Object TimeGenerated
By filtering to include only failed entries, you minimize processing and improve sorting time, which is especially beneficial in environments with extensive logging.
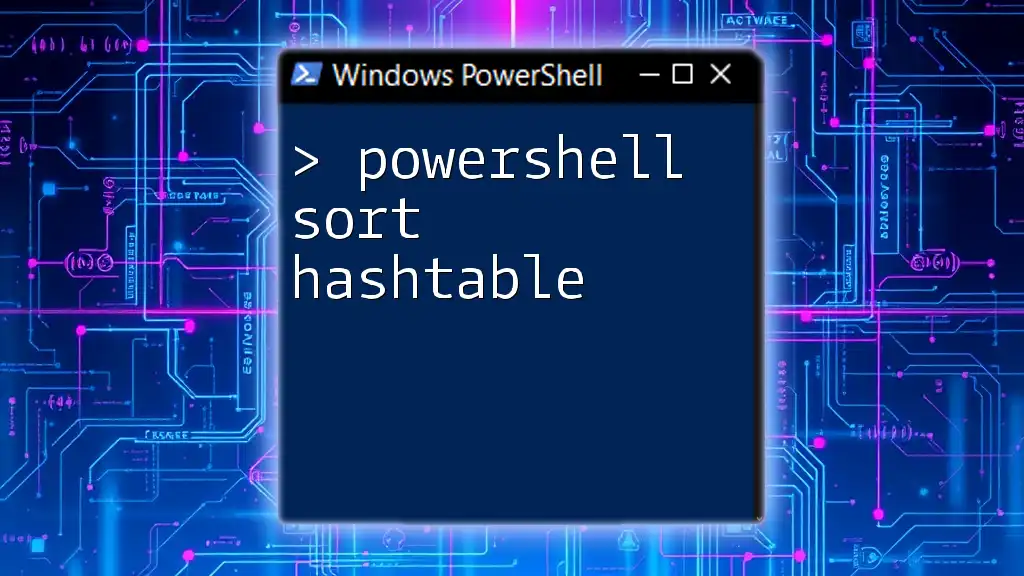
Troubleshooting Common Sorting Issues
Unexpected Sort Order
When sorting doesn't yield expected results, several factors could be at play. A common pitfall is the data type of the properties being sorted. Consult this example:
Get-Process | Sort-Object Name
If `Name` includes numeric values as strings, it could result in unexpected order (e.g., "1", "10", "2" instead of "1", "2", "10"). Ensure your data types are consistent or convert them as needed before sorting.
For example, you could convert the string representation of a property to a number using:
Get-Process | Sort-Object { [int]$_.Name }
This ensures proper numerical sorting rather than alphabetical.
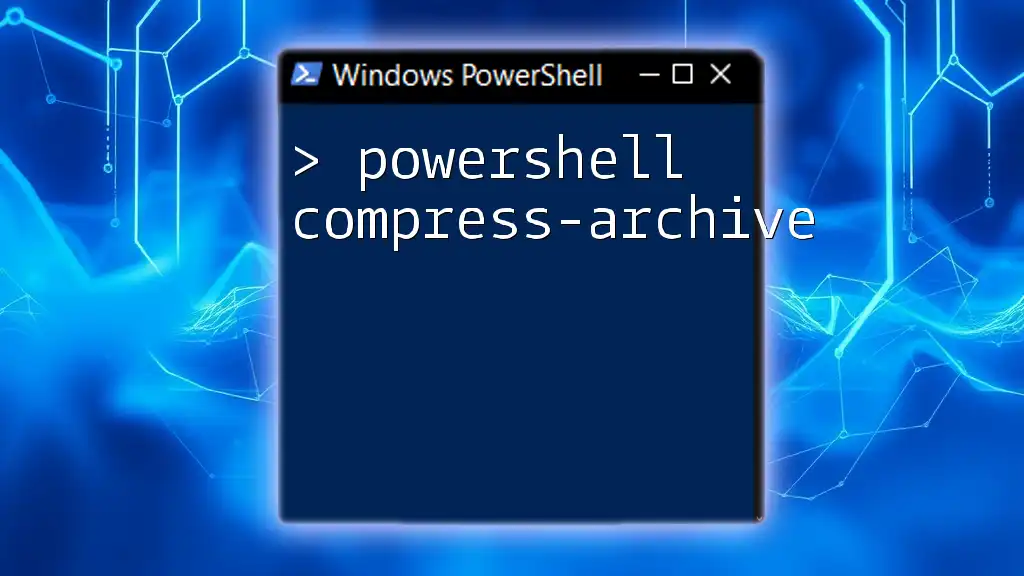
Conclusion
Sorting results in PowerShell is an invaluable skill that enhances data management, readability, and analysis efficiency. By understanding how to use the `Sort-Object` cmdlet effectively, as well as advanced techniques such as ScriptBlocks and filtering, users can optimize their scripting efforts and gain deeper insights from their data. Practice sorting with various datasets and commands to fully grasp these concepts and unlock the full potential of PowerShell in your workflows.
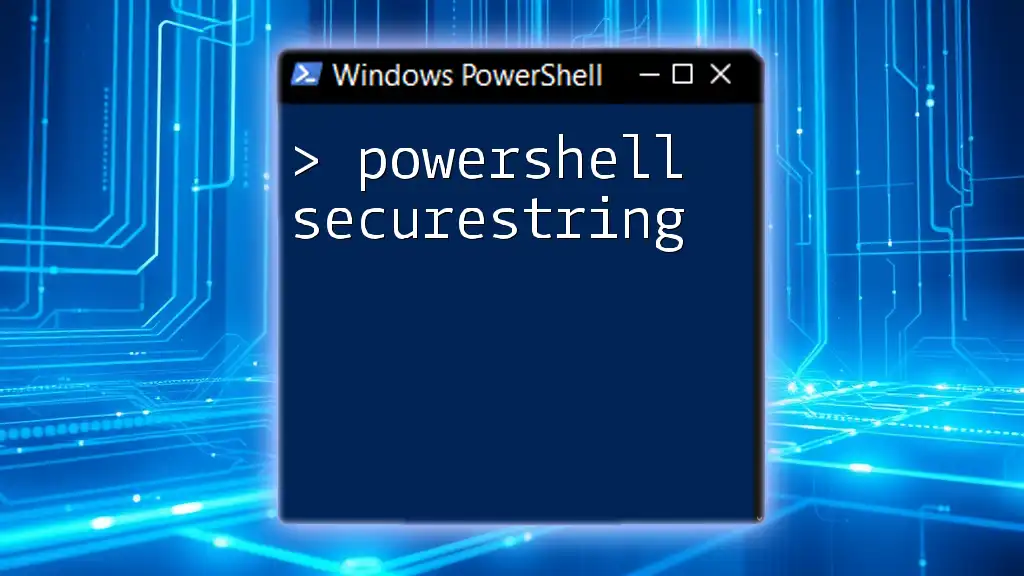
Additional Resources
Recommended Learning Resources
- Official PowerShell Documentation: Discover comprehensive guides on cmdlets and features.
- Online PowerShell Courses: Websites like Pluralsight or Udemy offer structured learning paths.
- PowerShell Community Forums: Engage with other PowerShell users to share insights and solutions.
FAQs
Common queries about PowerShell sorting can usually be answered by diving deeper into the topics covered here or consulting community resources for advanced guidance.