In PowerShell, you can sort a hashtable by its keys or values using the `Sort-Object` cmdlet in conjunction with `GetEnumerator()`, as demonstrated in the following code snippet:
$hashtable = @{ "b" = 2; "a" = 1; "c" = 3 }
$sortedByKey = $hashtable.GetEnumerator() | Sort-Object Key
$sortedByValue = $hashtable.GetEnumerator() | Sort-Object Value
This code creates a hashtable, sorts it by keys, and sorts it by values, allowing easy access to organized data.
Understanding Hashtables
What is a Hashtable?
A hashtable is a versatile collection in PowerShell that stores data in key-value pairs. Each key is unique, and it works like an index, allowing you to quickly access the associated value. Hashtables are useful for scenarios where you need to map distinct entries to specific values, making them ideal for settings, configurations, and lookups.
Creating a Hashtable
Creating a hashtable in PowerShell is straightforward. You can initialize an empty hashtable using the following syntax:
$myHashtable = @{}
Once you have your hashtable initialized, you can easily add key-value pairs. For example:
$myHashtable["Key1"] = "Value1"
$myHashtable["Key2"] = "Value2"
This method allows you to define your hashtable dynamically, adding entries as needed.
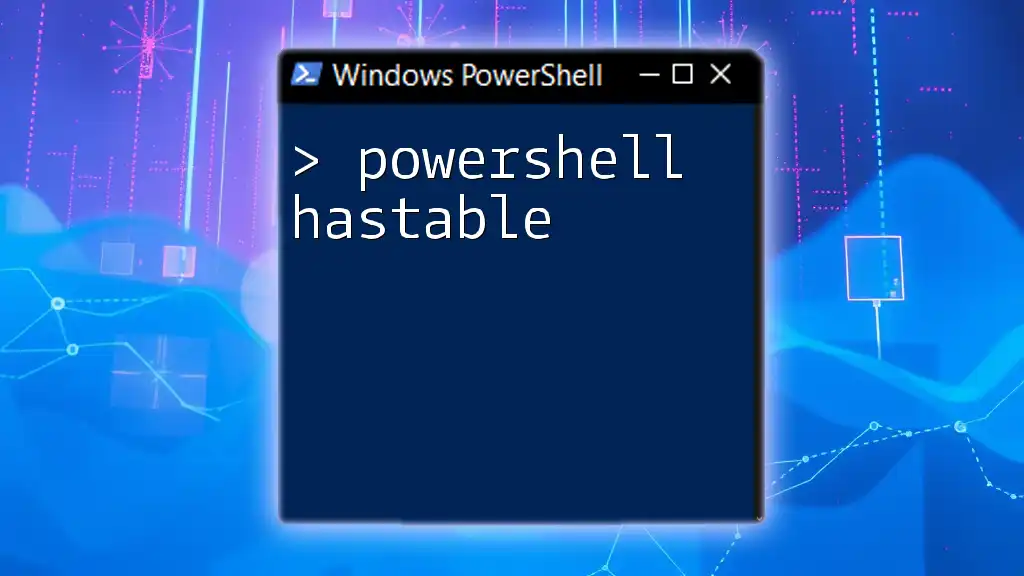
Sorting Hashtables
Why Sort a Hashtable?
Sorting hashtables is crucial for enhancing readability and facilitating efficient data processing. When you're dealing with large data sets or configurations, a sorted hashtable provides an easier way to locate information quickly. Additionally, sorted data is often more visually appealing, making reports or outputs easier to understand.
Methods to Sort a Hashtable
Sorting by Keys
One of the simplest methods to sort a hashtable is by its keys. By utilizing the `Sort-Object` cmdlet, you can effectively order your keys as follows:
$sortedKeys = $myHashtable.Keys | Sort-Object
After executing this code, `sortedKeys` will contain all the keys sorted in ascending order. This separation of keys is fundamental when you want to organize data based on its identifier.
Sorting by Values
In some scenarios, you may want to sort the hashtable based on its values rather than its keys. To sort by values, you can once again employ the `Sort-Object` cmdlet as demonstrated below:
$sortedValues = $myHashtable.GetEnumerator() | Sort-Object Value
This line will provide you with a sorted enumeration of the key-value pairs, organized by their respective values. Understanding how to retrieve sorted outputs based on value is essential for analyzing data contextually.
Displaying Sorted Hashtable
Creating Sorted Hashtable from Keys
If you want to construct a new hashtable based on sorted keys, you can do so with the following code:
$sortedHashtable = @{}
foreach ($key in $sortedKeys) {
$sortedHashtable[$key] = $myHashtable[$key]
}
This snippet initializes an empty hashtable and populates it using the sorted keys. The result is a new hashtable that retains the original key-value relationships, but in a sorted order.
Creating Sorted Hashtable from Values
Alternatively, if you wish to create a sorted hashtable based on values, consider the following example:
$sortedHashtableByValues = @{}
foreach ($pair in $sortedValues) {
$sortedHashtableByValues[$pair.Key] = $pair.Value
}
This method ensures that your new hashtable reflects the sorting applied to values, enabling you to present data in different contexts.
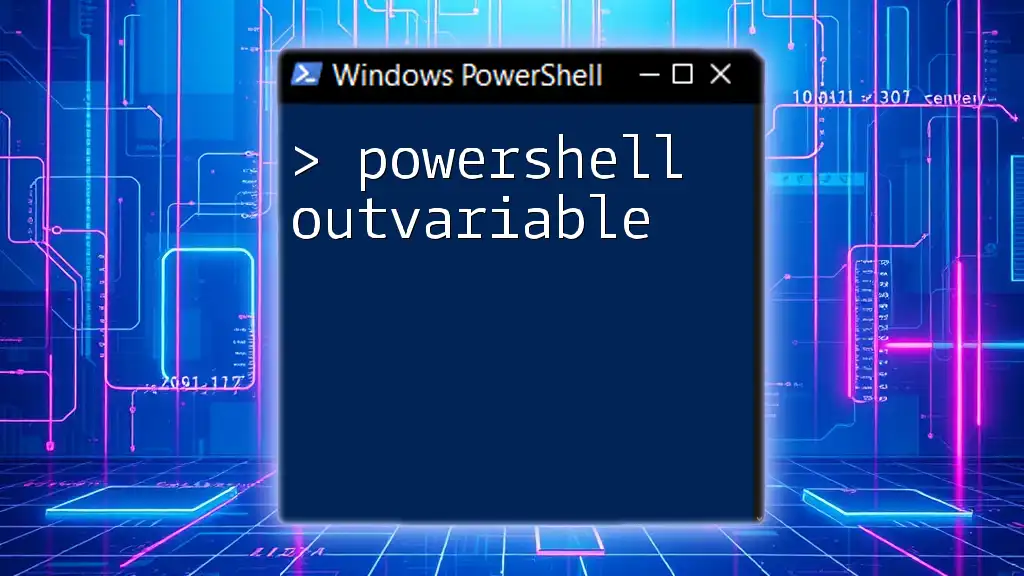
Practical Examples
Example 1: Sorting a Simple Hashtable
Let’s consider a simple hashtable and sort it. Here’s how you can define and sort it:
$simpleHashtable = @{
"Banana" = 2
"Apple" = 5
"Cherry" = 3
}
$sortedByFruit = $simpleHashtable.Keys | Sort-Object
This will yield a sorted list of the fruit names: Apple, Banana, Cherry. The sorted result can be highly beneficial when you want to present information in a specific order.
Example 2: Real-World Application
Sorting hashtables can play a significant role in practical situations, such as managing an inventory system. Below is an example:
$inventory = @{
"ItemA" = 50
"ItemB" = 75
"ItemC" = 30
}
$sortedInventory = $inventory.GetEnumerator() | Sort-Object Value
In this case, the `sortedInventory` will reflect the items organized by their stock levels, enabling you to quickly identify items in desirable order.
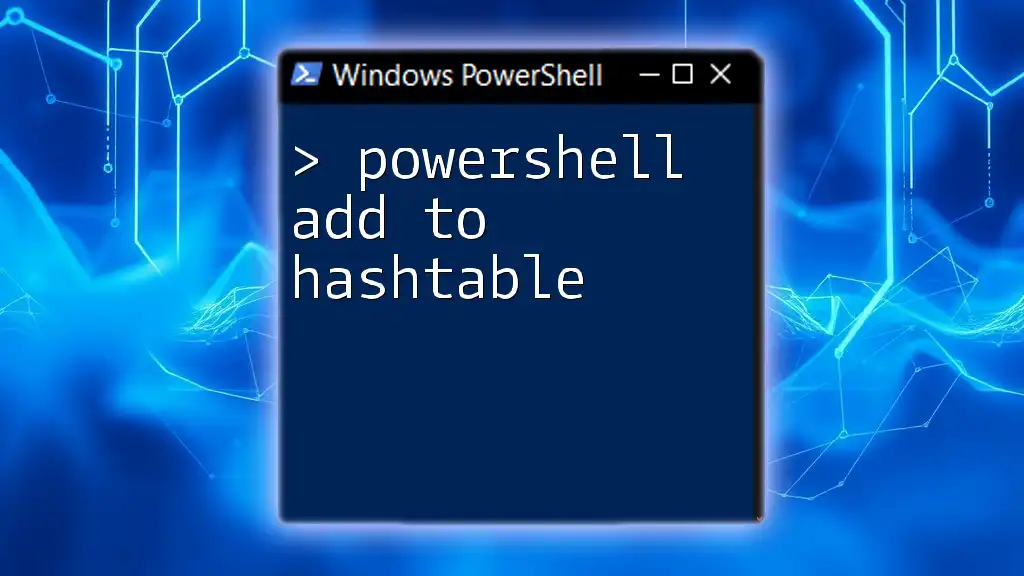
Advanced Sorting Techniques
Sorting with Custom Comparers
PowerShell allows for custom sorting based on your specific requirements. For instance, if you want to utilize a particular comparison technique, you can create a custom sort function as shown:
$customComparer = {
param($a, $b)
return [string]::Compare($a.Value, $b.Value)
}
$sortedCustom = $myHashtable.GetEnumerator() | Sort-Object -Property Value -Comparer $customComparer
This method provides flexibility by allowing you to sort according to custom criteria, which could be essential in specific data management scenarios.
Handling Duplicate Values
When sorting hashtables with duplicate values, it's essential to be mindful, as the nature of the keys remaining unique can lead to confusions in outputs. While duplicates are allowed in values, they pose a challenge when you need to present distinct entries based on those values. Therefore, considering the context of sorted data and the impact of duplicate values is key in your data handling strategy.
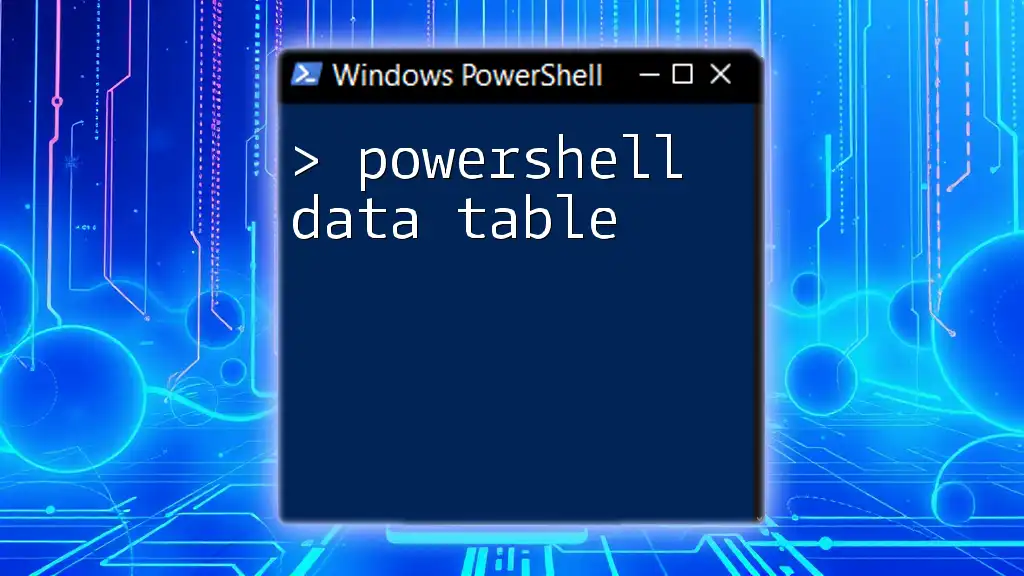
Conclusion
Understanding how to PowerShell sort hashtable lays the groundwork for efficiently managing data structures. With techniques for sorting by keys and values, as well as advanced methods for custom sorting, you’re now equipped to manipulate hashtables effectively. Practice and experimentation will allow you to apply these concepts in real-world scenarios, enhancing both your scripting skills and your ability to process data seamlessly.
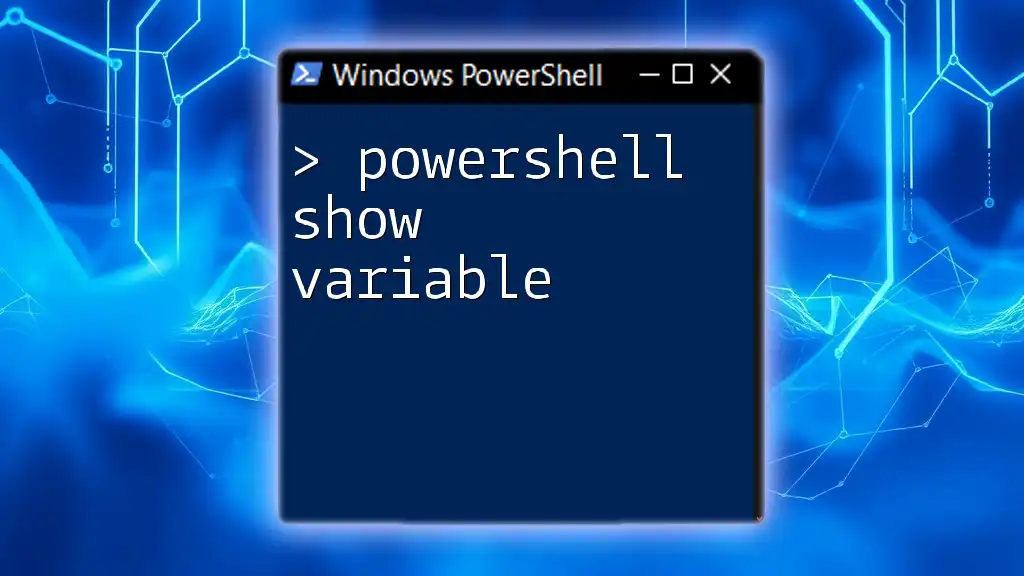
Additional Resources
To expand your knowledge on PowerShell and its functionalities, consider exploring recommended books, online courses, or authoritative documentation that dive deeper into PowerShell’s capabilities.
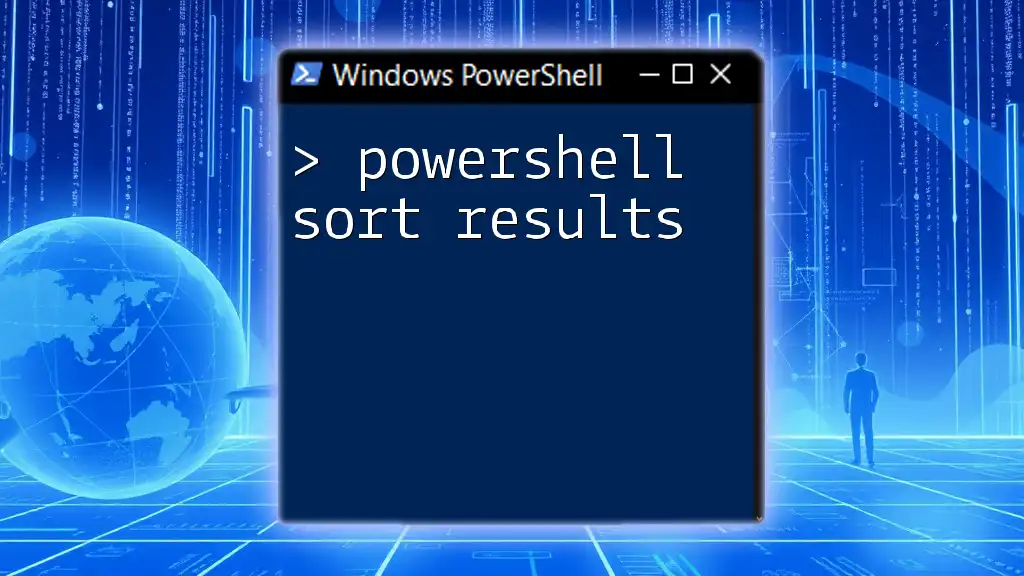
Call to Action
If you're eager to delve further into the world of PowerShell and learn practical skills, we invite you to sign up for our upcoming workshops. Join a community of learners dedicated to mastering PowerShell and enhancing their technical prowess!