The "Or" statement in PowerShell allows you to evaluate multiple conditions and returns True if at least one of the conditions is met.
if ($x -eq 10 -or $y -eq 20) {
Write-Host 'At least one condition is true!'
}
What is the "Or" Statement?
Definition
The PowerShell "Or" statement is a logical operator that allows you to evaluate multiple conditions, returning true if at least one of the conditions is true. In contrast to the "And" statement, which requires all conditions to be true, the "Or" statement provides more flexibility in conditional evaluations.
Syntax of the "Or" Statement
In PowerShell, the syntax for the "Or" statement primarily uses two operators: `-or` and `||`. While both serve the same basic purpose, they can differ based on the context in which they are used. Here’s a brief look at each:
- `-or`: A PowerShell-specific operator that evaluates logical conditions.
- `||`: A traditional programming operator that also functions as a logical "Or".

How to Use the "Or" Statement in PowerShell
Basic Example
To understand the "Or" statement's application, let’s consider a straightforward example. In this case, we can use two boolean variables to determine if at least one of them is true.
$a = $true
$b = $false
if ($a -or $b) {
"At least one condition is true"
}
In the code above, the output will indicate that at least one condition is true since `$a` is `$true`.
Combining Multiple Conditions
The "Or" statement shines when you're evaluating multiple conditions. You can easily combine them to form more complex logic. For instance, let’s evaluate whether a variable meets either of two numerical conditions:
$x = 5
if ($x -lt 10 -or $x -gt 20) {
"x is either less than 10 or greater than 20"
}
In this example, since `$x` equals 5, the condition “less than 10” is true, and you'll see the output indicating that at least one of the conditions has been met.
Using "Or" with Functions
You can also utilize "Or" statements within functions to enhance custom logic. Here’s an example where we check if a number is either 1 or 2:
function Test-Value {
param ($num)
if ($num -eq 1 -or $num -eq 2) {
"Value is either 1 or 2"
}
}
When you call `Test-Value` with arguments `1` or `2`, the function indicates that the value meets the specified conditions.
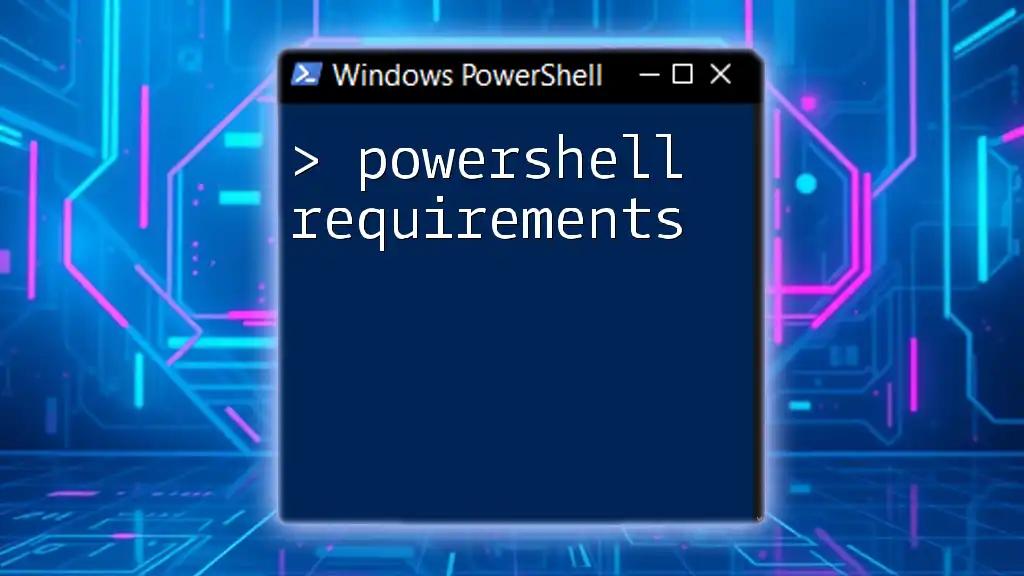
Practical Applications of the "Or" Statement
Filtering Outputs
One of the most practical uses of the "Or" statement is in filtering command outputs. For example, if you want to filter processes based on CPU usage or the number of handles, you can apply the "Or" logical operator:
Get-Process | Where-Object { $_.CPU -gt 100 -or $_.Handles -gt 200 }
This command filters the processes, allowing only those with CPU usage greater than 100 or handles greater than 200 to be displayed.
Error Handling with the "Or" Statement
PowerShell allows for robust error handling, and the "Or" statement can facilitate alternative paths. You can manage a try-catch scenario and use an "Or" statement to define what should happen if an error occurs:
try {
# Attempt some risky operation
}
catch {
"Error occurred but continuing with alternative action"
} -or {
# alternative operation
}
This setup enables a smooth continuation of script operation even if the initial try fails, ensuring you don’t lose flow in your script.

Best Practices for Using the "Or" Statement
Clarity and Readability
When using the "Or" statement, clarity and readability should be your top priorities. Always strive to structure your conditional logic in a way that is easily understandable. Group complex conditions logically and use parentheses to specify the order of evaluation, improving the clarity of your code.
Performance Considerations
While the "Or" statement is powerful, be cautious about using too many complex conditions. Evaluate the logic efficiently without unnecessary checks. For example, rewrite nested "Or" states to reduce the overall number of checks when possible.
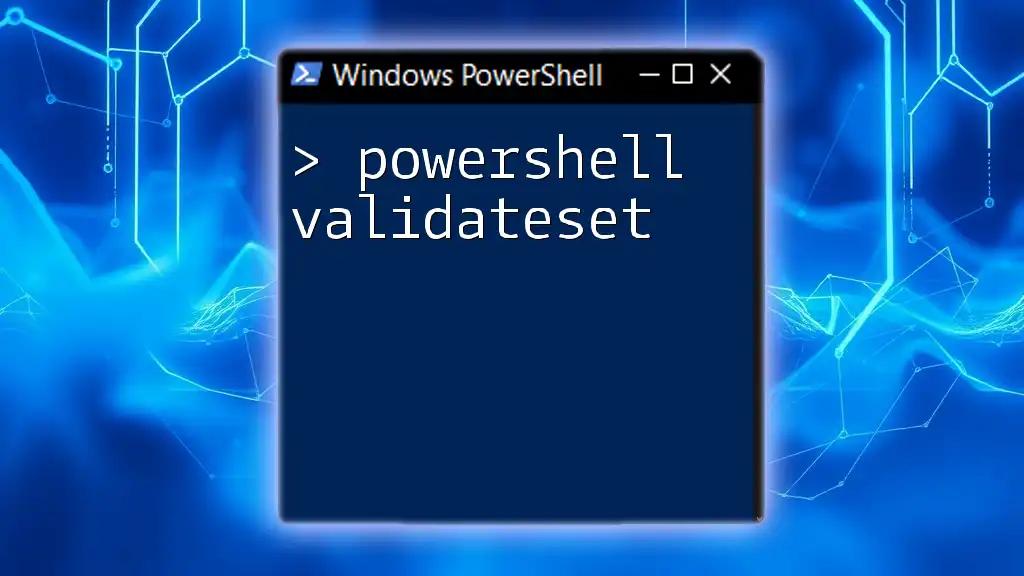
Common Mistakes When Using "Or"
Precedence Issues
Logical operators have a specific precedence, and the "Or" statement can lead to confusion if not properly managed. Always be mindful of how conditions are evaluated, especially in complex statements. When in doubt, use parentheses to ensure clarity regarding which conditions are evaluated together.
Overusing "Or" Statements
While "Or" statements are useful, they can affect readability if overused. Assess whether combining several conditions into an "Or" statement is necessary or if a clearer approach, such as restructuring your logic or using additional functions, might serve better.
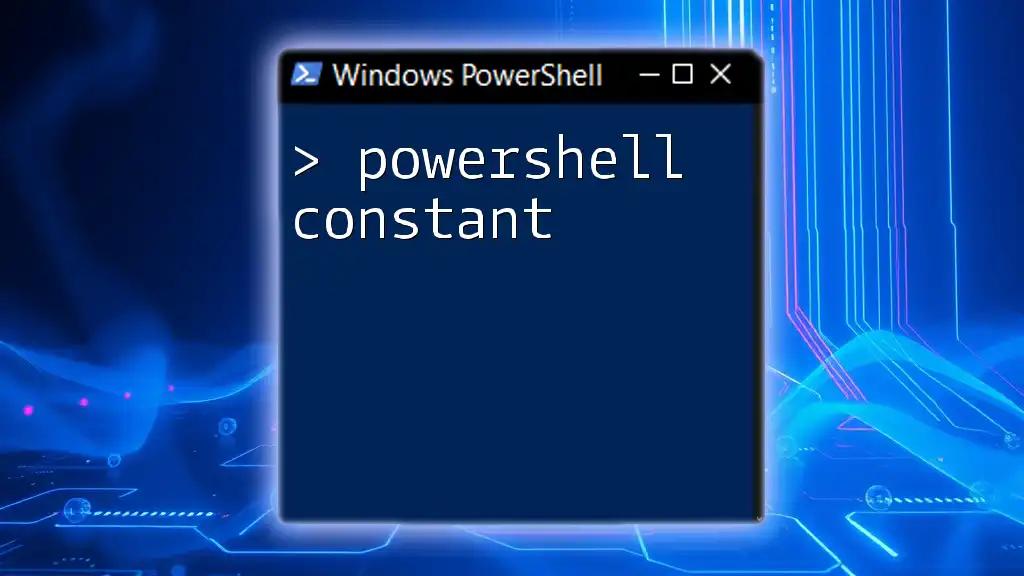
Conclusion
The PowerShell "Or" statement is an essential tool for executing conditional logic within your scripts. By mastering the "Or" statement, you will significantly enhance your ability to create dynamic, flexible scripts that can handle a variety of situations. Dive deeper into coding and practice with these logical operators, and you’ll see how they can improve your scripting capabilities.
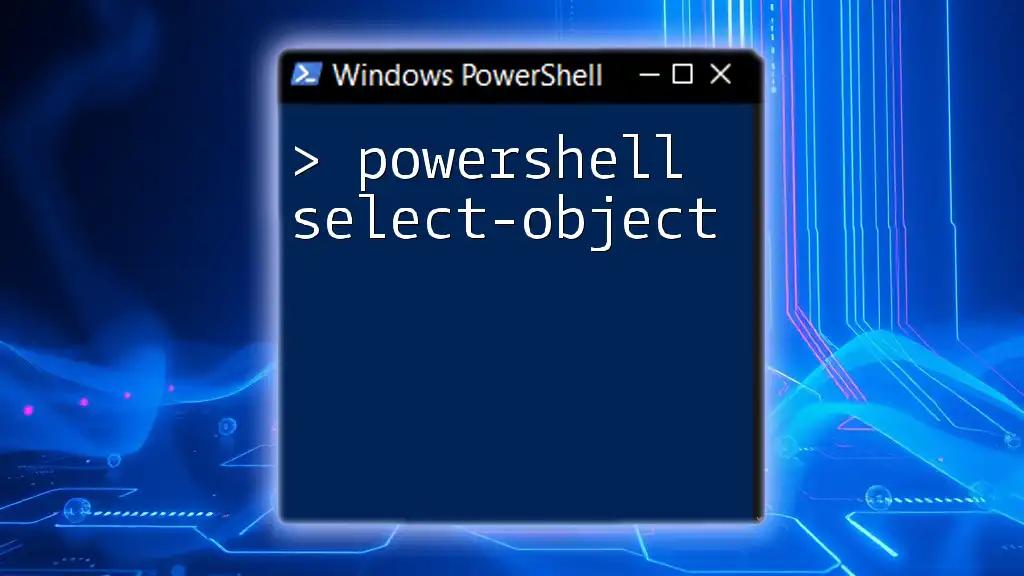
Additional Resources
For further reading, check the official PowerShell documentation, explore online forums, or engage in community-driven PowerShell courses to broaden your knowledge and skill set regarding scripting.
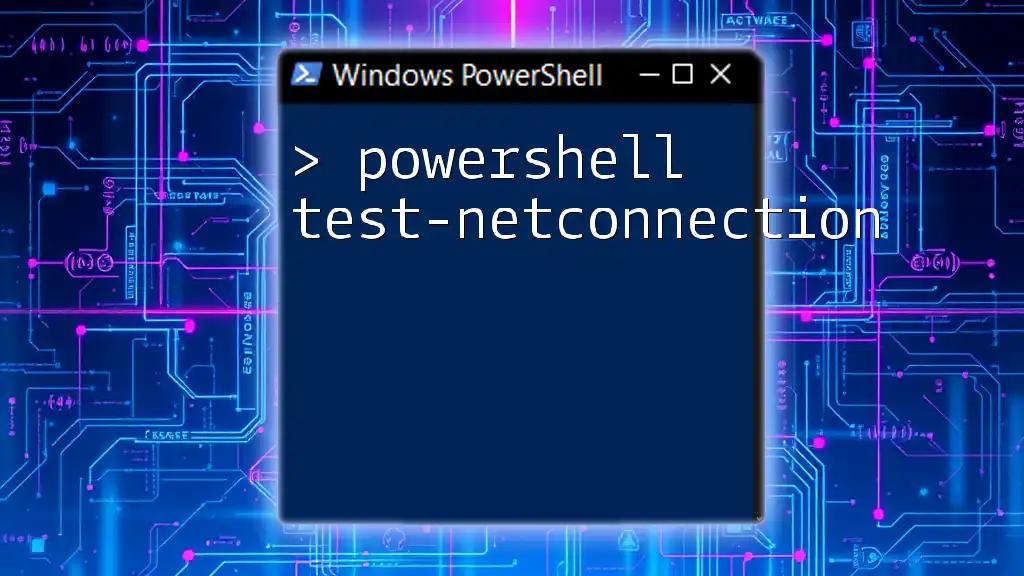
FAQs
What is the difference between `-or` and `||` in PowerShell?
Both operators serve as logical "Or" operators. The `-or` operator is specific to PowerShell and evaluates conditions based on PowerShell syntax. The `||` operator is taken from traditional programming languages and can sometimes lead to confusion if misapplied. As a general convention, use `-or` for PowerShell-specific conditions to maintain clarity.
Can I combine "Or" statements with other logical operators?
Yes, you can certainly combine "Or" with "And" statements. Just remember the operator precedence and structure your conditions using parentheses for clarity. This approach will help you formulate complex logical scenarios accurately.
Examples of outputs using the "Or" statement
You can expect varied outputs based on the conditions set in your "Or" statements. The functionality allows for versatile response handling that informs users about met conditions while validating overall script performance.
With practice and understanding, the "Or" statement can be a powerful ally in your PowerShell toolkit, improving both the functionality and efficiency of your scripts.