In PowerShell, you can create a timestamp using the `Get-Date` cmdlet, which retrieves the current date and time.
Get-Date -Format "yyyy-MM-dd HH:mm:ss"
What is a Timestamp?
A timestamp is a sequence of characters or encoded information that represents the date and time at which an event occurs. Timestamps play a critical role in various aspects of computing, such as logging events, tracking changes, and ensuring data integrity. They offer a precise way to record when certain actions were performed, making them essential for tasks ranging from data management to audit logging.
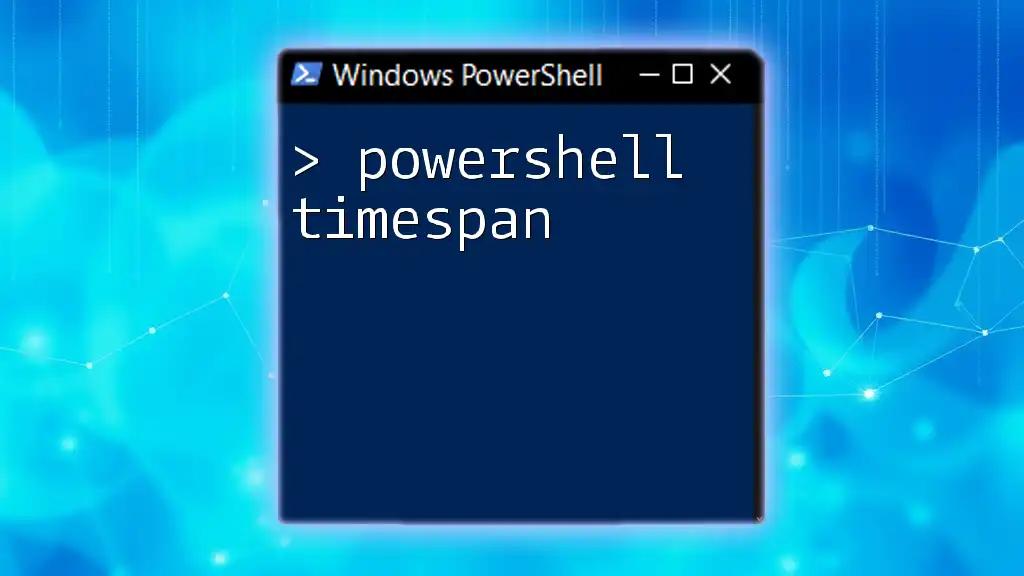
Why Use Timestamps in PowerShell?
In PowerShell, timestamps are widely utilized for:
- Automation: When running automated scripts, you often want to log the time an event occurred to facilitate troubleshooting and monitoring the execution timeline.
- File Management: Including timestamps in filenames helps avoid conflicts and ensures that files are easily identifiable by their creation time.
- Data Integrity: Recording timestamps when data is modified helps maintain a history of changes, useful for auditing and compliance.
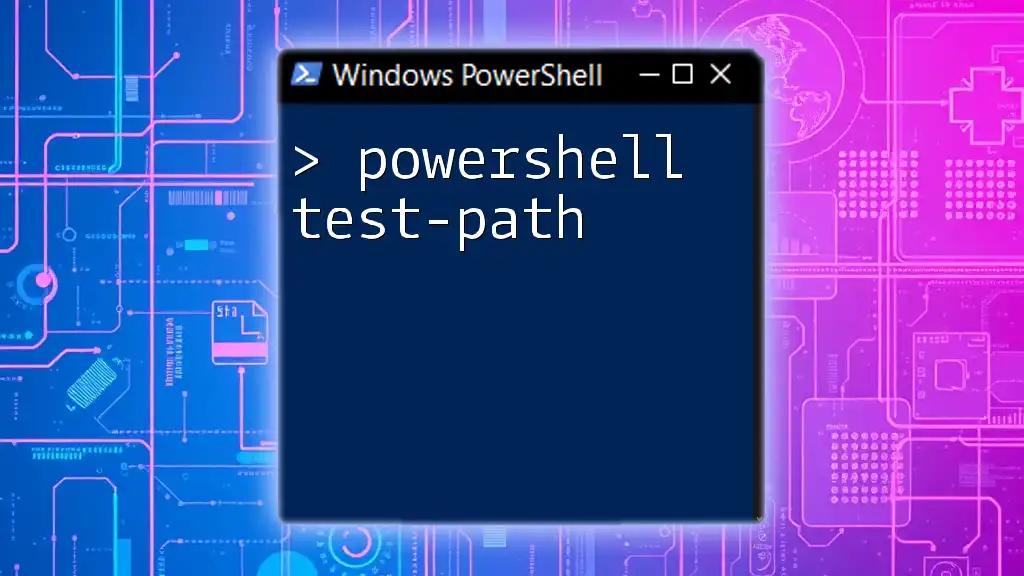
Getting Current Timestamps
Using Get-Date Cmdlet
One of the simplest ways to retrieve the current timestamp in PowerShell is through the `Get-Date` cmdlet. This cmdlet returns the current date and time based on the system clock.
Basic Syntax:
Get-Date
This command outputs the current date and time in the default format.
Custom Formatting
PowerShell allows you to customize the output format of the timestamp using the `-Format` parameter. This flexibility makes it easier to display the date and time in a way that suits your needs.
Example:
Get-Date -Format "yyyy-MM-dd HH:mm:ss"
This format provides a clear and easily understandable representation of the date and time, which can be useful for logging and reporting purposes. You can use various format specifiers to adjust how the output looks. For instance, using `MM` displays the month in two digits, while `dd` shows the day.
Timezone and Culture Considerations
Adjusting for Timezones
When working with timestamps, it’s important to account for potential timezone differences, especially in scripts that may report events across various regions.
Example:
Get-Date -Format "yyyy-MM-dd HH:mm:ss zzz" -TimeZone "Pacific Standard Time"
This snippet gives you the current date and time while explicitly formatting the timezone, allowing scripts to operate correctly regardless of location.
Localization in PowerShell
Localization is key when dealing with timestamps in an international environment. Different cultures have unique date and time formats, and PowerShell can accommodate these by setting the desired culture.
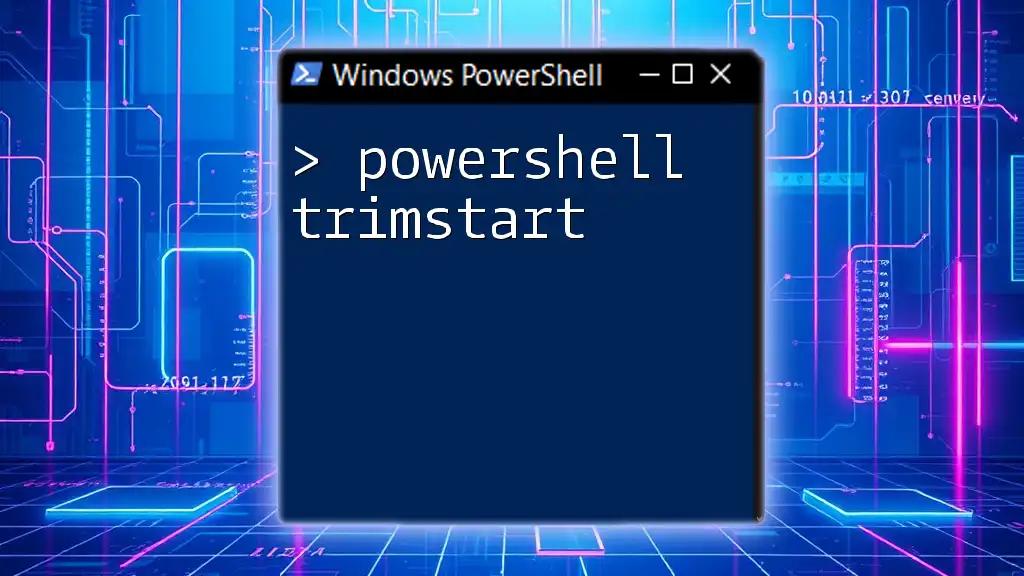
Creating Custom Timestamps
Building Your Own Timestamp
Creating custom timestamps can help format the information to better suit your requirements. For example, you might want to create a timestamp for logging activities in a standardized format.
Example:
$timestamp = (Get-Date).ToString("yyyyMMdd_HHmmss")
This command generates a timestamp that can be easily included in filenames or logs, ensuring they remain unique and organized.
Using Timestamps in Filenames
When creating files programmatically, you often want to include timestamps to prevent overwrites and to maintain historical records.
Example:
$filename = "Log_" + $timestamp + ".txt"
This results in a filename like `Log_20231101_150000.txt`, making it clear when the log was created.
Avoiding Common Pitfalls
When using timestamps in filenames, it's crucial to avoid illegal characters. Many systems do not allow the use of colons (`:`) in filenames because they are reserved for denoting time in the format. Instead, consider using underscores or hyphens to separate date and time components, promoting clarity.
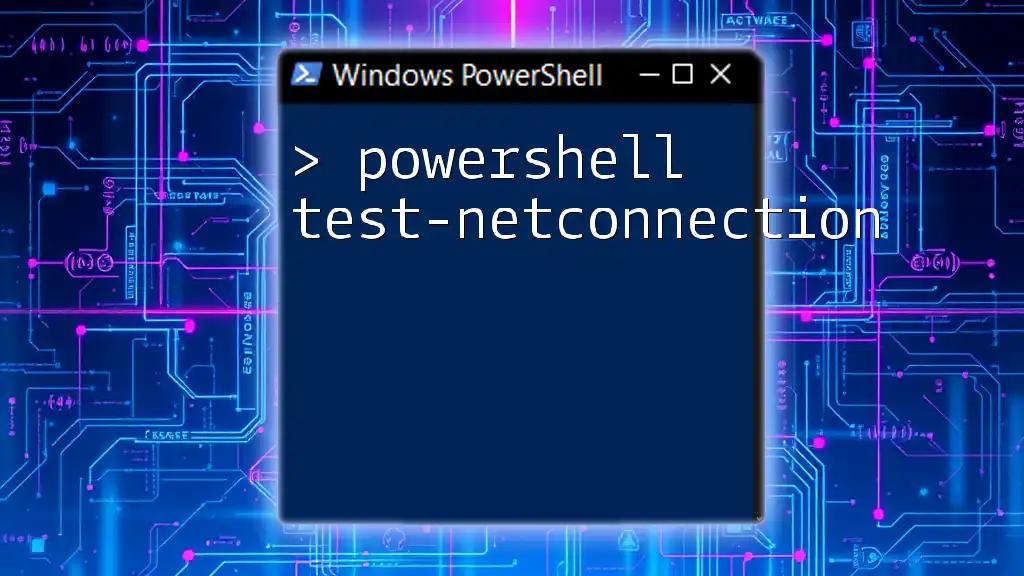
Manipulating Timestamps
Adding and Subtracting Time
PowerShell provides various methods to manipulate timestamps, allowing you to calculate future or past events seamlessly.
Using Add-Methods
You can easily add or subtract time using the `Add` methods available on the DateTime object.
Example:
$futureDate = (Get-Date).AddDays(5)
$pastDate = (Get-Date).AddHours(-3)
The first command computes a date five days in the future, while the second takes three hours off the current time, making it easy to plan or log events accurately.
Comparing Timestamps
Comparisons between timestamps can inform decisions or trigger conditions within scripts.
Example:
if ((Get-Date) -gt $pastDate) { "It's in the future!" }
In this example, PowerShell evaluates whether the current date and time are greater than a previously saved timestamp, demonstrating the utility of comparison operators in various automation scenarios.
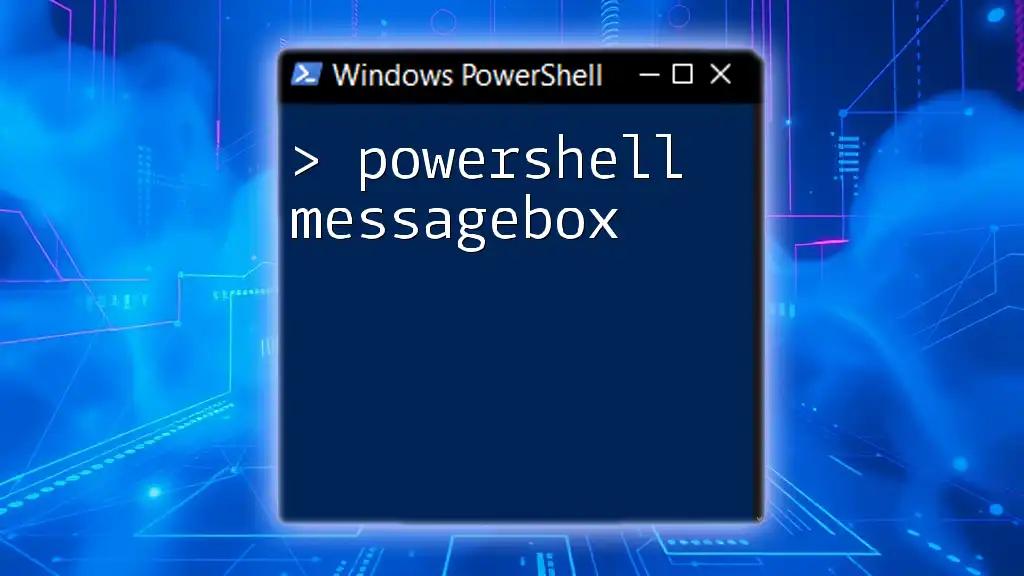
Formatting Timestamps for Output
Different Formatting Methods
When generating outputs from commands in PowerShell, formatting your timestamps enhances readability.
Using Format-Table for Output:
Get-Process | Select-Object Name, @{Name='StartTime';Expression={($_.StartTime).ToString('yyyy-MM-dd HH:mm:ss')}} | Format-Table
This command retrieves a list of running processes and formats their start times into an easily digestible structure.
Exporting Timestamps to Files
Timestamps can also be used when exporting data to files, ensuring that logs or reports are labeled correctly.
Example:
Get-Process | Export-Csv -Path "Processes_$timestamp.csv"
This ensures each exported process log is uniquely identified by the timestamp embedded in the filename, facilitating better organization.

Advanced Timestamp Techniques
Using Timestamps in Logs
Creating logs is one of the most critical aspects of scripting for monitoring and debugging purposes. Timestamps can establish exactly when each entry was made.
Example of a Simple Logging Function:
function Log-Event {
param (
[string]$message
)
$timestamp = Get-Date -Format "yyyy-MM-dd HH:mm:ss"
Add-Content -Path "eventlog.txt" -Value "$timestamp : $message"
}
This function records the message along with a timestamp, logging activity in a straightforward manner that aids in diagnosing issues later.
Timestamping Events
Automating event logging further enhances script functionality. This includes using timestamps to note a range of activities like start and end of processes, failures, or status changes.

Common Use Cases
Automation Scripts: In automated scripts, timestamps often serve as markers for task execution. By logging timestamps, you can review when each task completed, helping to diagnose performance bottlenecks.
Backup and Restore Functions: When performing backups, applying timestamps ensures you can create versioned copies. This practice not only protects against data loss but also facilitates easy restoration to a specific point in time.
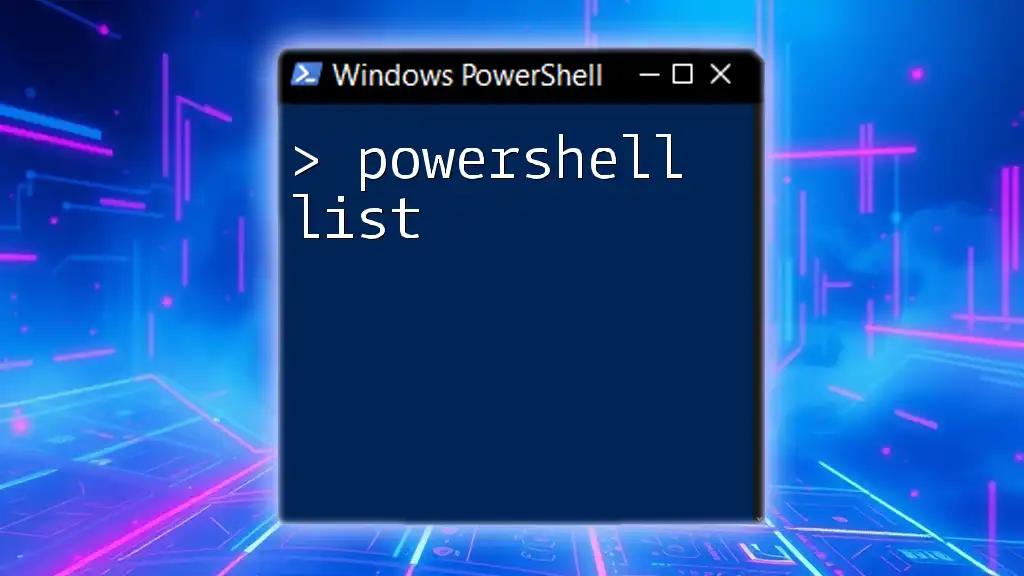
Troubleshooting Common Timestamp Issues
Handling Timezone Confusion
Many common issues arise from server or script execution in different time zones. To avoid timezone-related errors, always explicitly specify the timezone if your script interacts with users or systems across multiple regions.
Date Formats and Localization Problems
Improper date formatting can lead to unexpected behaviors in scripts or incorrect log data. Always prefer using the standard formats, and consider localizations if your script may be used in different cultures.
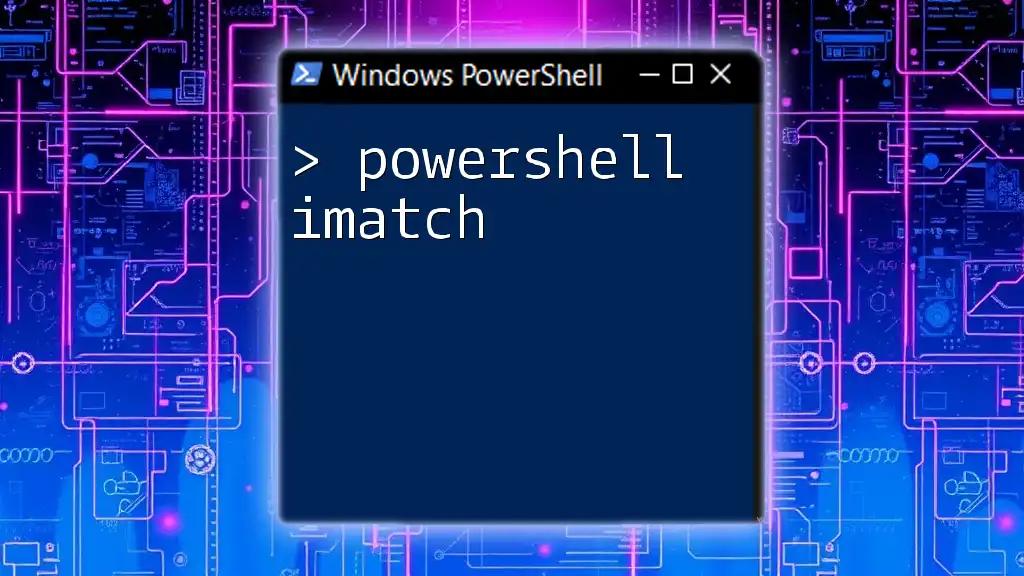
Recap of Key Points
Utilizing timestamps in PowerShell not only enhances your scripting capabilities but also improves the maintainability and debuggability of your scripts. With their versatility in automation, file management, and logging, timestamps are an indispensable feature in any PowerShell toolkit.
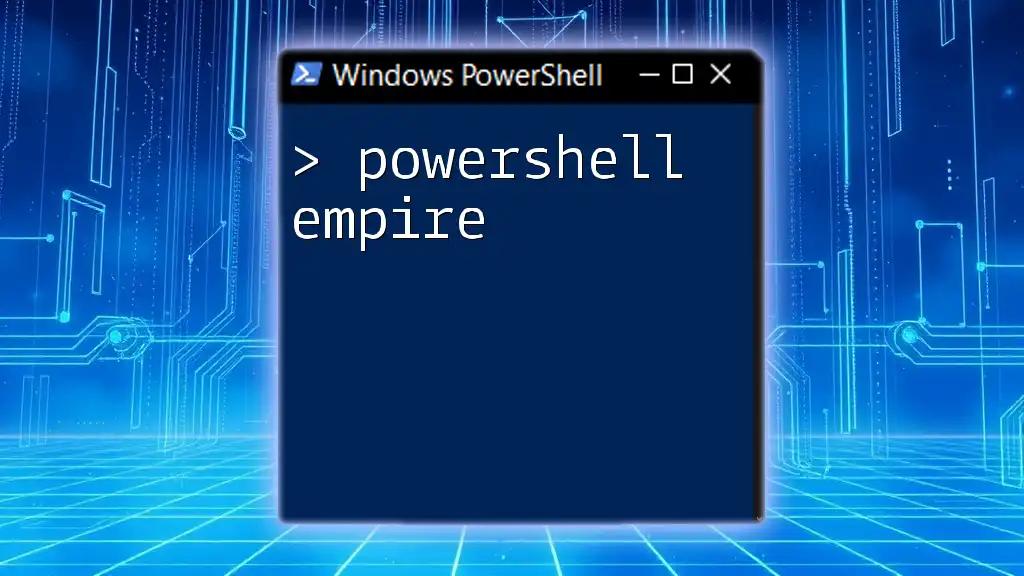
Call to Action
Practice using timestamps in your PowerShell scripts today! Experiment with the provided examples and think creatively about how timestamps can improve your scripts. Explore further scenarios in real-world applications to master the use of timestamps effectively.
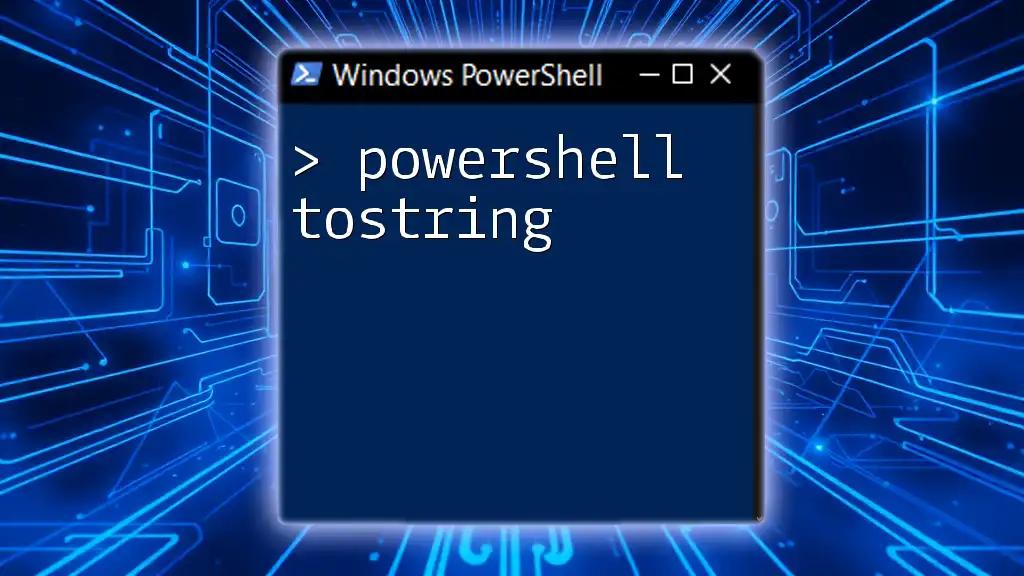
Additional Resources
For those looking to delve deeper into PowerShell timestamps, consider referring to Microsoft's official PowerShell documentation or community forums. These resources provide a wealth of information that can expand your knowledge even further.

FAQs
Q1: What if I want to display only the date part of a timestamp?
You can easily achieve this by using the `-Format` parameter of the `Get-Date` cmdlet:
Get-Date -Format "yyyy-MM-dd"
Q2: How do I read timestamps from a file?
Reading timestamps from files typically requires parsing the content where timestamps are stored. You can use `Get-Content` to read the file and then process it accordingly in your script. For example:
Get-Content "eventlog.txt" | ForEach-Object {
$parts = $_ -split " : "
$timestamp = $parts[0]
$message = $parts[1]
# Do something with the timestamp and message
}
Incorporating these techniques into your PowerShell scripts will greatly enhance your coding efficiency and effectiveness.