Certainly! The PowerShell message box is a simple way to display a pop-up alert to users, using the `Windows.Forms` assembly to create interactive dialog boxes.
Here’s a code snippet in Markdown format:
Add-Type –AssemblyName System.Windows.Forms
[System.Windows.Forms.MessageBox]::Show("Hello, World!", "Greeting")
Understanding PowerShell and its User Interface
PowerShell is a powerful scripting language and command-line shell designed primarily for system administration. Its primary focus is on automating tasks and managing system configurations while allowing interactions with various programming elements. Part of its strength lies in enhancing user communications through a graphical user interface (GUI). This includes utilizing MessageBoxes to inform users or prompt them for decisions during script execution.
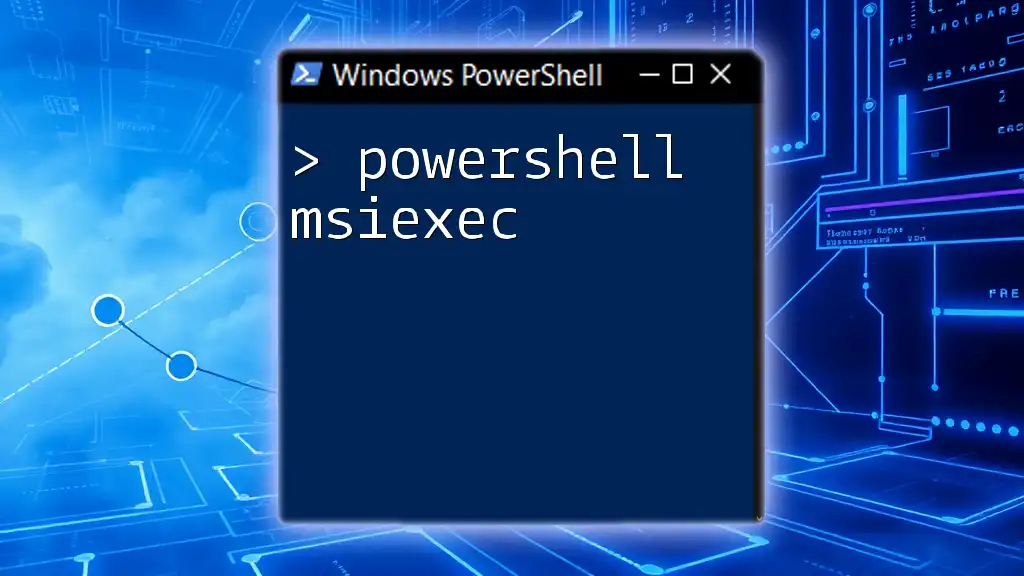
What is a PowerShell MessageBox?
A PowerShell MessageBox is a simple graphical dialog that can display messages and solicit user responses. It is particularly useful when you need to alert the user about a situation, for example, confirming actions or communicating errors.
Common use cases for PowerShell MessageBoxes include:
- Alerting users to an important status.
- Asking users to confirm risky operations.
- Displaying informational messages to guide users.
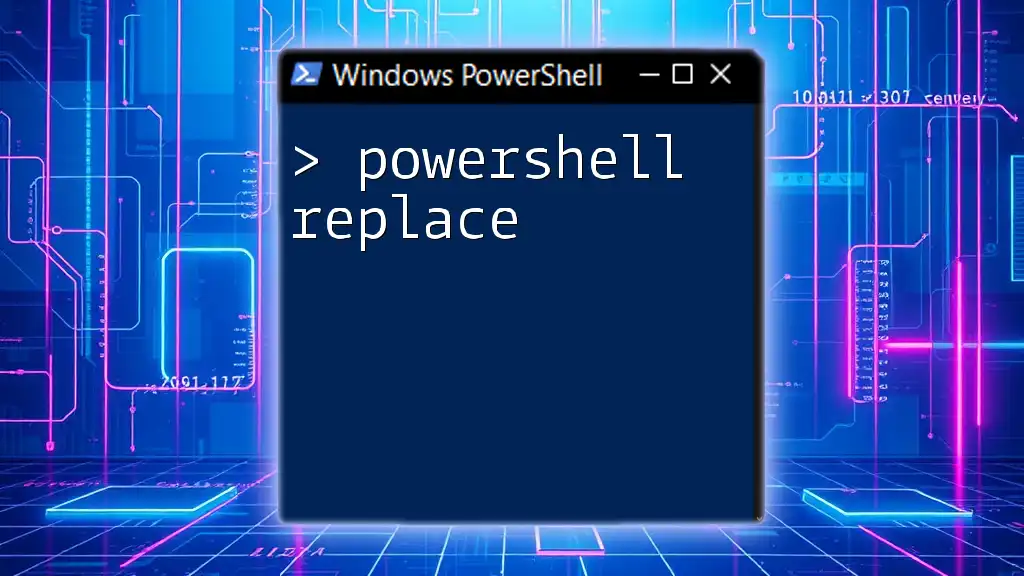
Creating a PowerShell MessageBox
Requirements for Using MessageBox in PowerShell
To use MessageBoxes in PowerShell, you'll need to incorporate the Windows Forms assembly, which is a part of the .NET Framework. Make sure you are using a compatible version of PowerShell and verify that Windows Forms is accessible in your script environment.
Basic Syntax for Creating a MessageBox
The basic syntax for creating a MessageBox is straightforward. You can display a simple message with a command indicating the message you wish to show. Here’s how to write a simple MessageBox command:
[System.Windows.Forms.MessageBox]::Show("Your message here")
This line of code will produce a basic MessageBox with the provided message. It's the simplest way to convey information to the user.
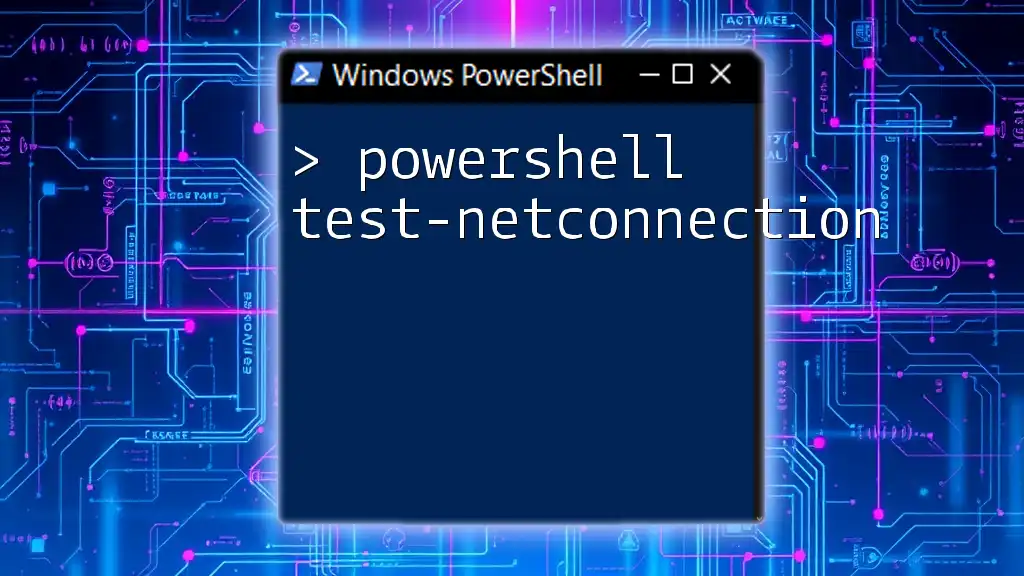
Customizing Your MessageBox
Adding Buttons to Your MessageBox
PowerShell MessageBoxes can be enhanced by including different buttons. You can customize the interaction by choosing from various button combinations. For example, you can prompt the user with OK and Cancel options:
[System.Windows.Forms.MessageBox]::Show("Your message here", "Title", [System.Windows.Forms.MessageBoxButtons]::OKCancel)
This command reflects a more interactive dialog, allowing users to either confirm or cancel an action.
Customizing Icons in MessageBoxes
Another way to enhance your MessageBox is by adding icons that reflect the nature of the message. PowerShell provides several built-in options for icons, such as Information, Warning, Error, etc. Here’s how to include an icon:
[System.Windows.Forms.MessageBox]::Show("Your message!", "Title", [System.Windows.Forms.MessageBoxButtons]::OK, [System.Windows.Forms.MessageBoxIcon]::Information)
Using an icon helps set the tone of the message and can immediately inform the user about the context.
Setting a Title for Your MessageBox
Setting a title for your MessageBox is crucial for providing users with context. It signifies what the message pertains to, guiding their understanding of the dialog's content. Here is an example of setting a title:
[System.Windows.Forms.MessageBox]::Show("Information Message", "Information", [System.Windows.Forms.MessageBoxButtons]::OK)
By doing this, you add clarity, making the user experience richer.
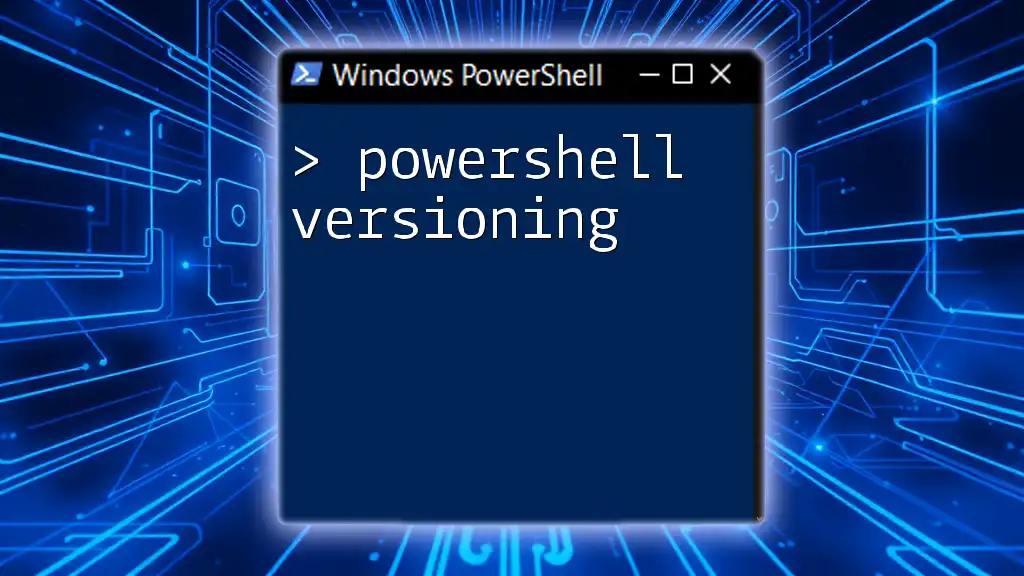
Handling User Responses from a MessageBox
One of the key features of PowerShell MessageBoxes is capturing user responses. This enables you to take different actions based on the user's decision. For instance, if you want to confirm whether the user wants to proceed with an operation, you can implement the following:
$result = [System.Windows.Forms.MessageBox]::Show("Do you want to continue?", "Confirmation", [System.Windows.Forms.MessageBoxButtons]::YesNo)
if ($result -eq [System.Windows.Forms.DialogResult]::Yes) {
# Action for Yes
} else {
# Action for No
}
In this example, based on the user's response, the script can proceed with specific actions, enhancing interactivity and decision-making.
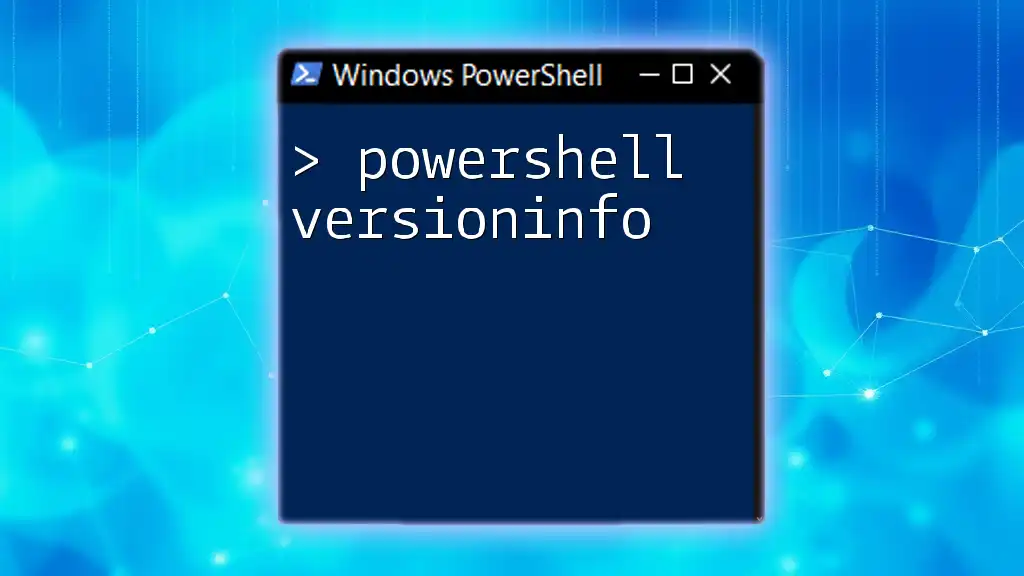
Real-world Use Cases for PowerShell MessageBoxes
User Confirmation Dialogs
A common application of PowerShell MessageBoxes is to present confirmation dialogs. For critical actions, such as deleting files or changing settings, utilizing a MessageBox to confirm can safeguard against inadvertently executing undesired operations.
Alerting Users to Errors or Important Information
PowerShell MessageBoxes are also effective for communicating errors or significant notices. For instance, if an unexpected issue arises during script execution, the user should be informed promptly. Here is a simple example:
[System.Windows.Forms.MessageBox]::Show("An error has occurred!", "Error", [System.Windows.Forms.MessageBoxButtons]::OK, [System.Windows.Forms.MessageBoxIcon]::Error)
This alert not only informs the user of an error but does so in a clear and engaging manner, allowing them to understand that prompt action may be needed.
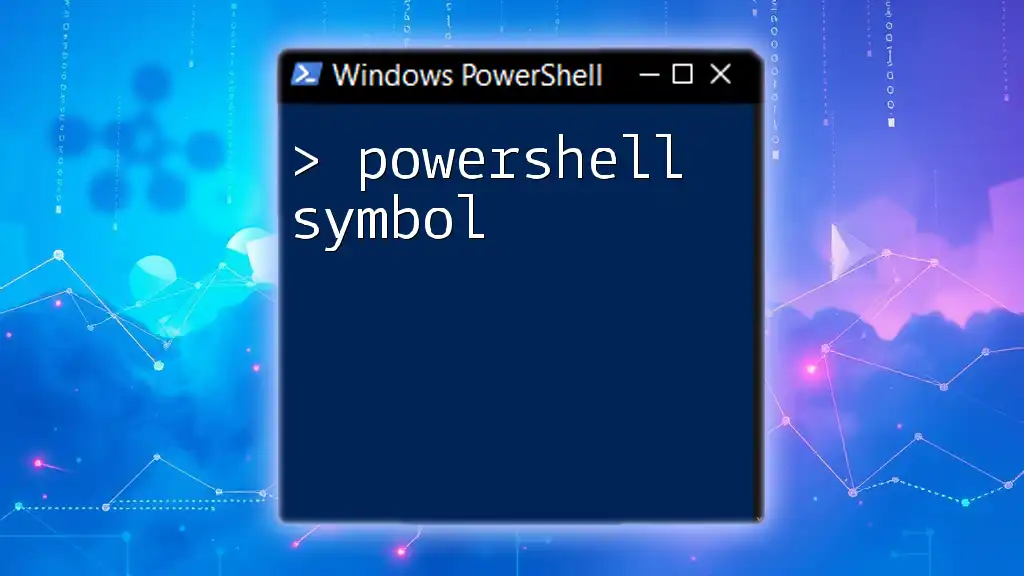
Best Practices for Using MessageBoxes
Keeping It Concise and Clear
MessageBoxes should convey messages that are concise and clear. Avoid overcrowding the MessageBox with unnecessary text. A well-constructed message can greatly improve user understanding. Aim to deliver succinct information while still providing necessary details.
Avoiding Overuse of MessageBoxes
While MessageBoxes can be immensely helpful, it's crucial to avoid overusing them. Excessive interruptions can frustrate users and detract from the script's usability. Consider integrating alternative forms of user communication, such as logging errors to a file or using the console output to reduce dependency on MessageBoxes.
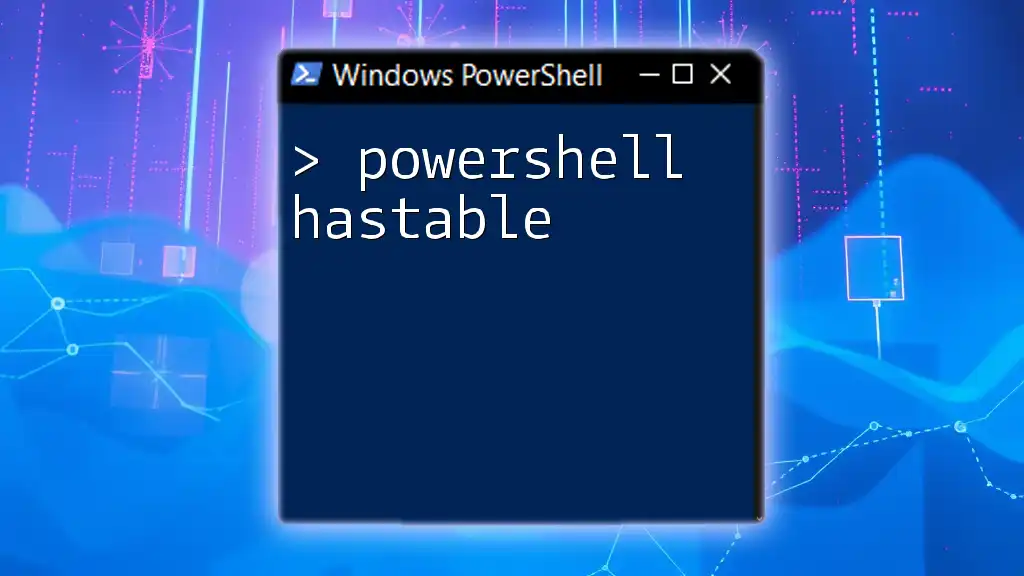
Conclusion
In summary, PowerShell MessageBoxes serve as a vital tool for improving user interaction within scripts. They help in conveying critical information, confirming actions, and alerting users to potential issues, thereby enhancing the overall user experience.
As you explore creating and customizing MessageBoxes in your own PowerShell scripts, remember to keep your messages clear, concise, and purposeful. Embrace this valuable feature, and elevate your scripts to new levels of interactivity and ease of use!