PowerShell symbols, such as `$`, represent variables within PowerShell commands, allowing users to store and manipulate data effectively.
Here's a basic code snippet that demonstrates the use of a variable symbol:
$greeting = 'Hello, World!'
Write-Host $greeting
Understanding PowerShell Symbols
What are Symbols in PowerShell?
In PowerShell, symbols refer to various characters and operators that serve specific functions within scripts and commands. They play a crucial role in defining the structure of the code, enabling the execution of commands, and facilitating logical operations. Understanding these symbols is essential for crafting effective and efficient scripts.
Types of Symbols in PowerShell
Special Characters
PowerShell utilizes a variety of special characters that enhance the functionality of commands. Each character carries particular significance:
-
`#`: This symbol marks the beginning of a comment. Any text following this symbol on the same line is ignored during execution, which makes it perfect for adding notes or explanations within your code.
# This function retrieves all running processes Get-Process
-
`;`: The semicolon acts as a command separator. It allows you to execute multiple commands in a single line, enhancing efficiency.
Get-Process; Get-Service
-
`"`: Double quotation marks are used to encapsulate strings. This is vital for defining text values or paths in your scripts.
Operators
Operators in PowerShell also serve as symbols, allowing users to perform various operations. These operators can be categorized into arithmetic, comparison, and logical types:
-
Arithmetic Operators
- These include basic math operations such as `+`, `-`, `*`, and `/`. They are essential for performing calculations within scripts.
Example of adding two numbers:
$result = 5 + 10 Write-Output $result # Output: 15
-
Comparison Operators
- Operators like `-eq`, `-ne`, `-lt`, and `-gt` allow you to compare values. These are crucial for controlling the flow of execution in your code based on conditions.
Example of a comparison:
if ($x -lt 10) { Write-Output "Less than 10" }
-
Logical Operators
- These include `-and`, `-or`, and `-not`. They are used to create compound conditions and to control logic branches in your scripts.
Escape Characters
Escape characters are used to include special characters in strings without interfering with the execution of the code. In PowerShell, the backtick (`` ` ``) acts as the escape character.
For example, to display a double quote within a string, use:
Write-Output "This is a double quote: `"`
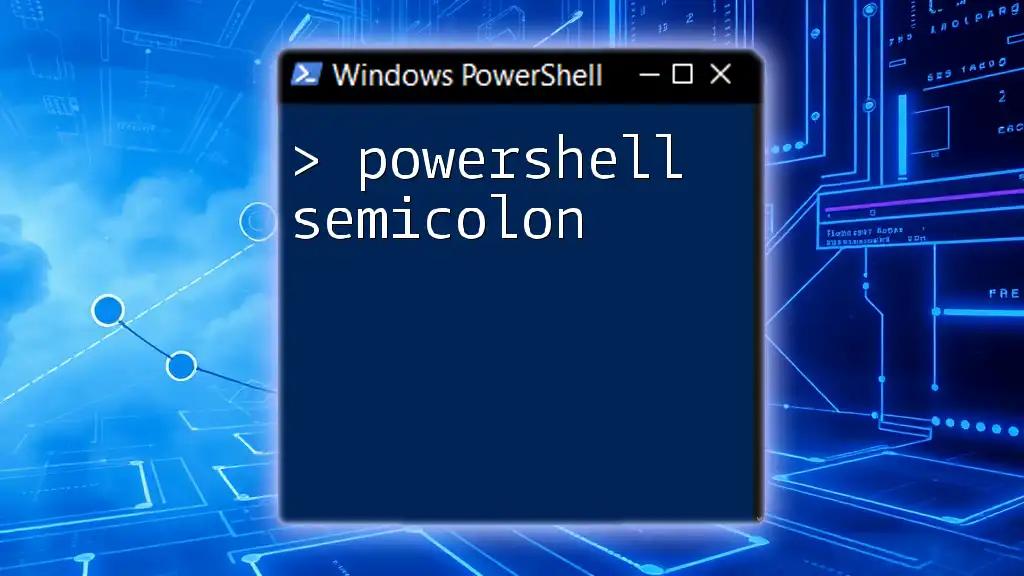
Practical Uses of PowerShell Symbols
Commenting in Scripts
Comments are vital for enhancing the readability and maintainability of your scripts. They allow developers to provide context or explanations for specific code sections.
By using the `#` symbol, you can write single-line comments effectively:
# This line retrieves the list of services
Get-Service
For multiline comments, you can alternatively use `<#` and `#>` to encompass your notes:
<#
This section retrieves all running processes
and outputs their names and statuses.
#>
Get-Process | Select-Object Name, Status
Combining Commands
The ability to combine multiple commands concisely is one of PowerShell's strengths. The semicolon (`;`) plays a key role here by allowing you to execute commands sequentially.
Get-Process; Get-Service
This execution will first retrieve the current processes and then the services without needing to separate them into different lines or files.
Working with Variables
In PowerShell, variables are denoted by a dollar sign (`$`). Understanding how to properly define and manipulate variables is fundamental for any script.
To create a variable, simply prefix your identifier with `$`:
$greeting = "Hello, World!"
Write-Output $greeting # Output: Hello, World!
This symbolization clearly differentiates variables from other types of data or commands.
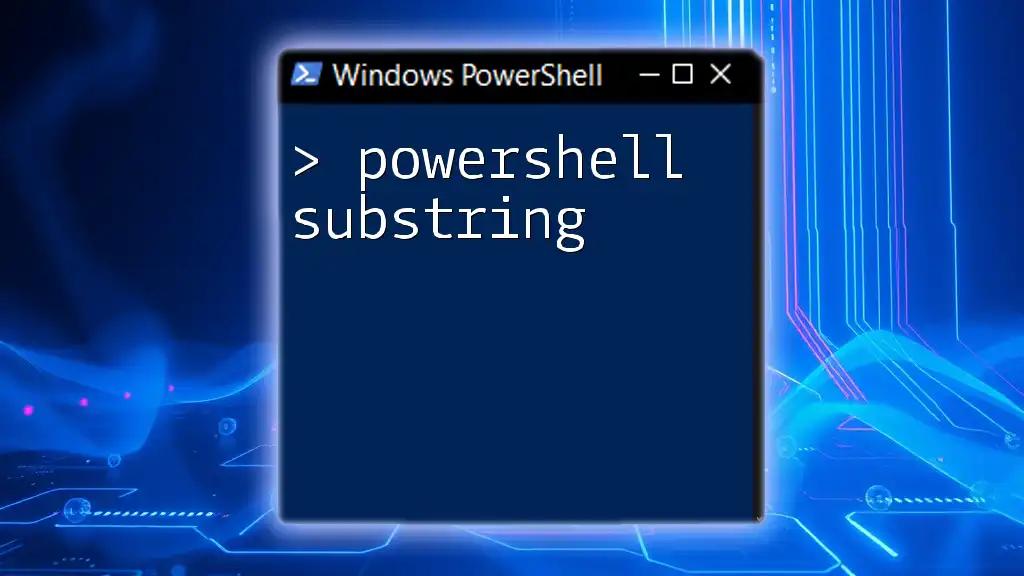
Advanced Symbol Use Cases
PowerShell Piping (`|`)
The pipe symbol (`|`) is a powerful feature in PowerShell that allows you to pass the output of one command directly into another command as input. This facilitates data manipulation seamlessly.
For example, the following command retrieves all running services and pipes that output to filter specific information:
Get-Service | Where-Object { $_.Status -eq "Running" }
In this case, `$_` represents the current object in the pipeline, making it a valuable tool for refining outputs without intermediate variables.
Using Parentheses for Control Flow
Parentheses (`(` `)`) are instrumental in controlling the order of operations within your commands. They ensure specific expressions are evaluated first.
For example:
if ($x -gt 10) { Write-Output "Greater than 10" }
Here, the condition inside the parentheses is evaluated before the action block is executed.
Chaining Commands with the `&&` Operator
The logical AND operator (`&&`) allows you to execute a second command only if the first command succeeds. This is particularly useful for creating dependencies between processes.
Test-Path "C:\example.txt" && Get-Content "C:\example.txt"
In this example, the second command to get the content of the file will only run if the first command confirms that the file exists.
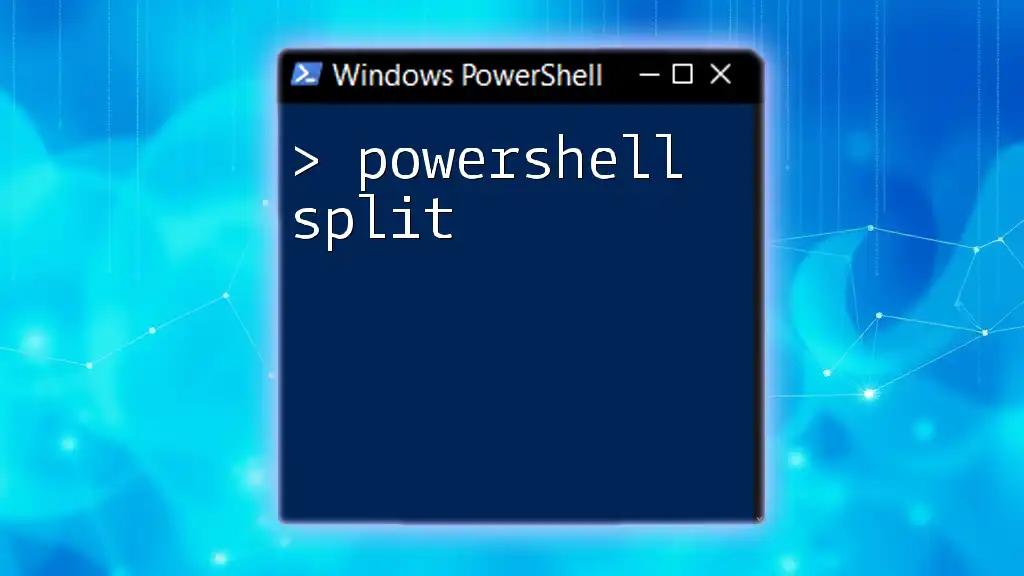
Best Practices for Using Symbols in PowerShell
Clarity and Readability
To ensure that your PowerShell scripts are straightforward and easy to understand, adhere to best practices concerning the use of symbols. Consistent and logical use of comments, formatting, and spacing will greatly enhance readability. Pay special attention to symbols like `#` for comments, using clear and descriptive variable names, and maintaining proper indentation for blocks of code.
Debugging Common Symbol Issues
Errors involving symbols can often lead to frustrating debugging sessions. Common mistakes might include:
- Misplaced symbols or missing operators, which can lead to syntax errors.
- Improper use of parentheses, affecting command precedence.
- Neglecting the escape character when special characters are needed in strings.
To troubleshoot effectively:
- Carefully review your code to ensure all symbols are used correctly.
- Use the PowerShell Integrated Scripting Environment (ISE) or PowerShell console to test sections of your code incrementally.
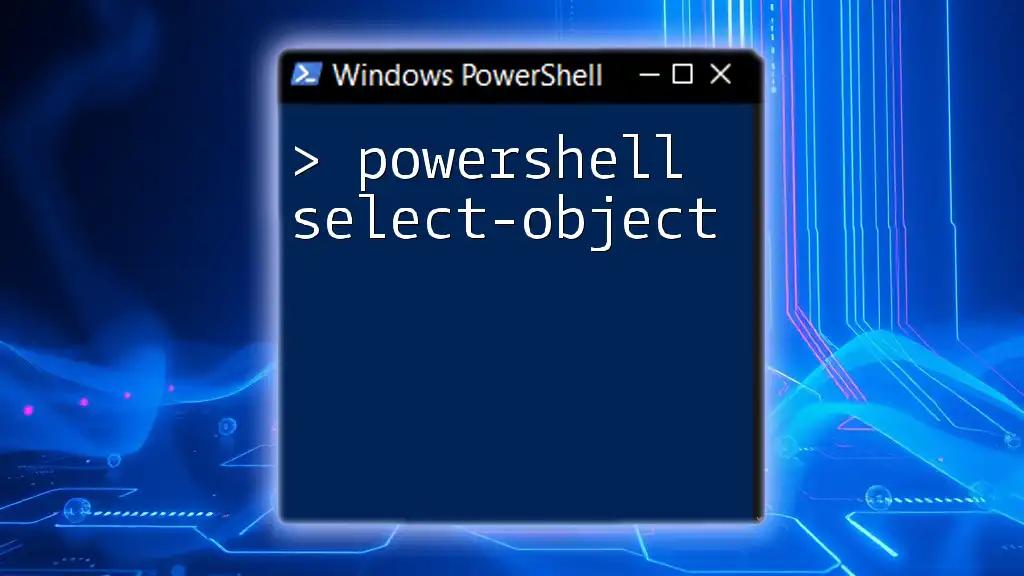
Conclusion
In conclusion, a strong grasp of PowerShell symbols is essential for anyone looking to harness the full power of PowerShell scripting. From defining variables to combining commands and creating complex workflows, symbols play a pivotal role. By practicing their usage and understanding their functionalities, you can greatly enhance your scripting capabilities and efficiency.
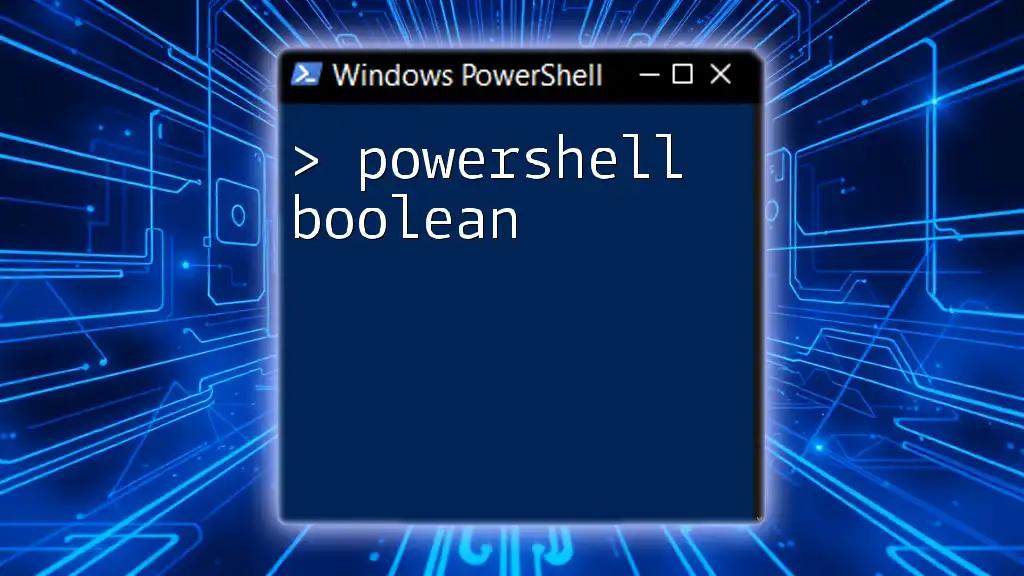
Additional Resources
For further learning, explore additional resources such as online courses, PowerShell documentation, and tutorials that delve into more advanced topics. Take advantage of community forums and discussion boards to engage with other PowerShell users and enhance your learning experience.
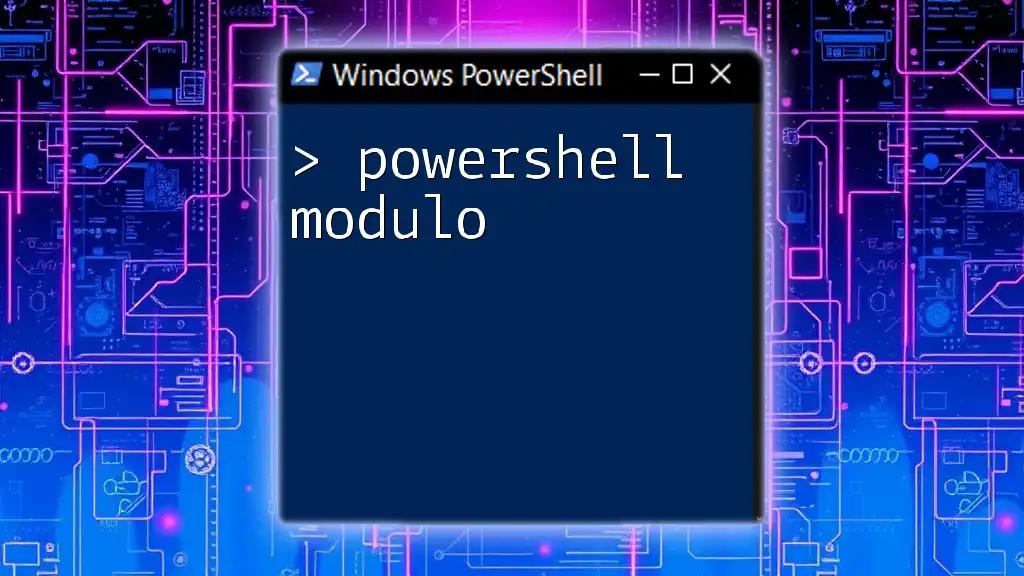
Call to Action
Feel free to leave comments or questions regarding PowerShell symbols. We encourage you to practice writing your own scripts and leverage the symbols discussed to become proficient in PowerShell scripting.