PowerShell modules, or "powershell mod," are reusable packages of PowerShell functions and commands that streamline complex tasks and extend functionality.
Here's an example of creating a simple PowerShell module:
# Create a new module called HelloWorld.psm1
function Say-Hello {
param (
[string]$Name = "World"
)
Write-Host "Hello, $Name!"
}
Export-ModuleMember -Function Say-Hello
Understanding PowerShell Mod
What is Mod in PowerShell?
In PowerShell, the term mod refers to the modulus operation, which calculates the remainder of a division between two numbers. This operation is crucial in many programming and scripting situations as it provides insight into the relationships between integers, especially regarding division and remainder results.
The significance of modulation in PowerShell cannot be underestimated; it allows for essential functionality such as conditional checks, data validation, and more sophisticated arithmetic and logical operations within scripts.
Why Use Modulus in PowerShell?
Utilizing the modulus operator in PowerShell can serve various purposes, including:
- Determining Even or Odd Values: The simplest and one of the most prevalent uses of `mod` is to check if a number is even or odd.
- Controlling Logic Flow: Modulus can enhance decision-making processes within scripts.
- Practical Calculations: For tasks that require specific calculations, such as pagination or interval checks.
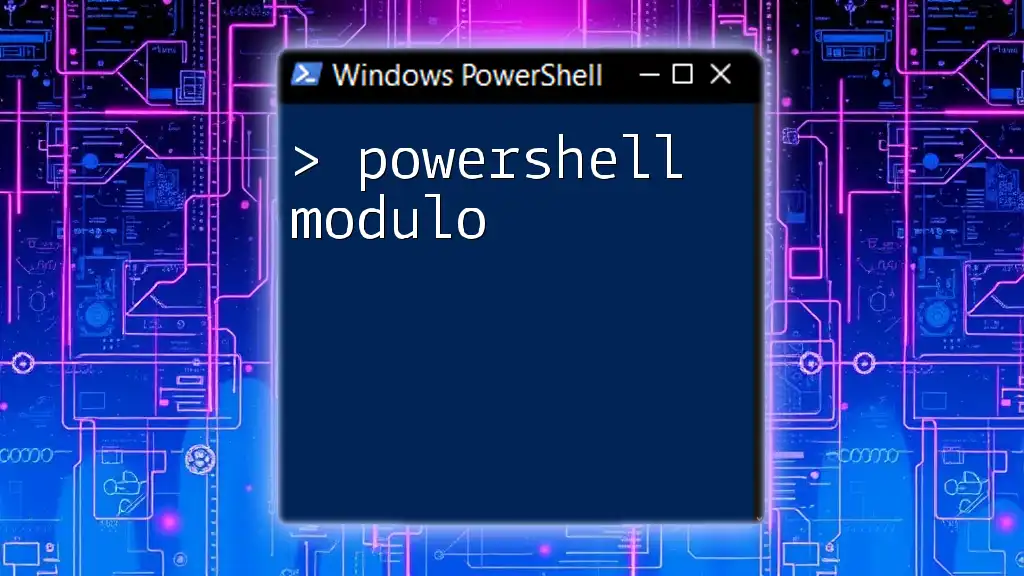
Basic Syntax of the Mod Command
Structure of the Mod Command
The basic syntax for using the modulus operator in PowerShell is straightforward. You define two integers and apply the modulus operator `%` to calculate the remainder of their division:
$result = $a % $b
In this command:
- $a represents the dividend (the number being divided).
- $b is the divisor (the number by which the dividend is divided).
- $result is the outcome, or the remainder of the division.
Examples of Using Modulus
Basic Example
To illustrate the basics, consider the following code snippet, which calculates the modulus of 10 divided by 3:
$result = 10 % 3
# Output: 1
In this case, 1 is the output because when 10 is divided by 3, the remainder is 1.
Real-World Example
A common application of the modulus operator is determining whether a given number is even or odd. Here’s an example:
$number = 7
if ($number % 2 -eq 0) {
"Even"
} else {
"Odd"
}
When executed, this script will output "Odd", indicating that 7 is not divisible by 2. This simple conditional check showcases how to leverage modulus in decision-making.
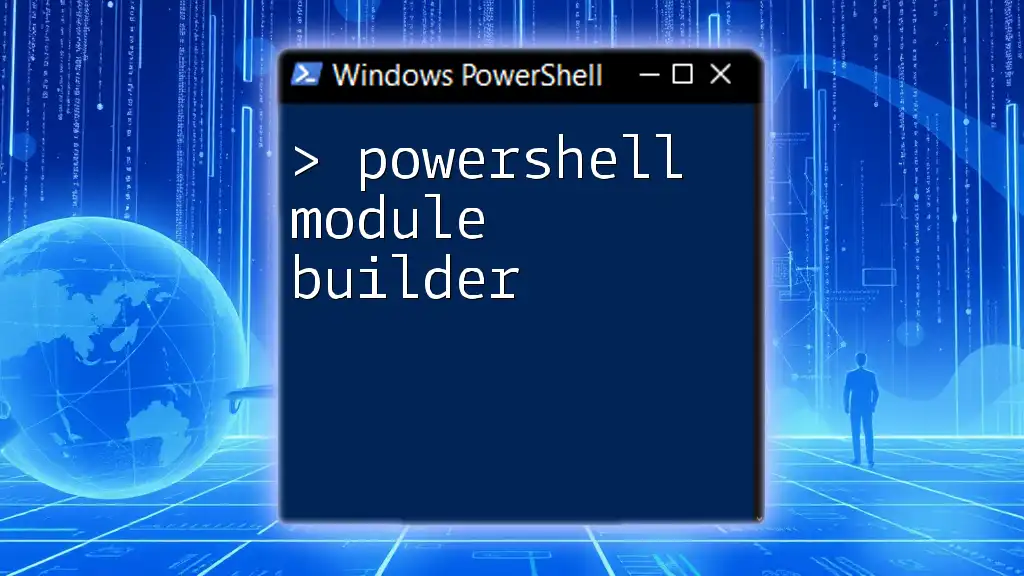
Advanced Application of Modulus in PowerShell
Looping through a Collection
You can take advantage of the modulus operator when iterating over a collection of items. The following example demonstrates this by checking each number in a range to determine if it is even or odd:
1..10 | ForEach-Object {
if ($_ % 2 -eq 0) {
"$_ is Even"
} else {
"$_ is Odd"
}
}
This code takes the range of numbers from 1 to 10, evaluates each number through the loop, and outputs whether each number is "Even" or "Odd." This method of utilizing modulus simplifies the code significantly while providing essential data efficiently.
Using Modulus for Pagination
Modulus can also prove invaluable in scenarios where you need to paginate results. For example, consider the following code that simulates pagination:
$data = 1..100
$pageSize = 10
$data | ForEach-Object {
if ($($_ % $pageSize) -eq 0) {
"Page $($_ / $pageSize) Complete"
}
}
In this example, the script processes a dataset of 100 items while checking if the current index is a multiple of 10, indicating the end of a page. This utility can help organize data when presenting information in manageable chunks.
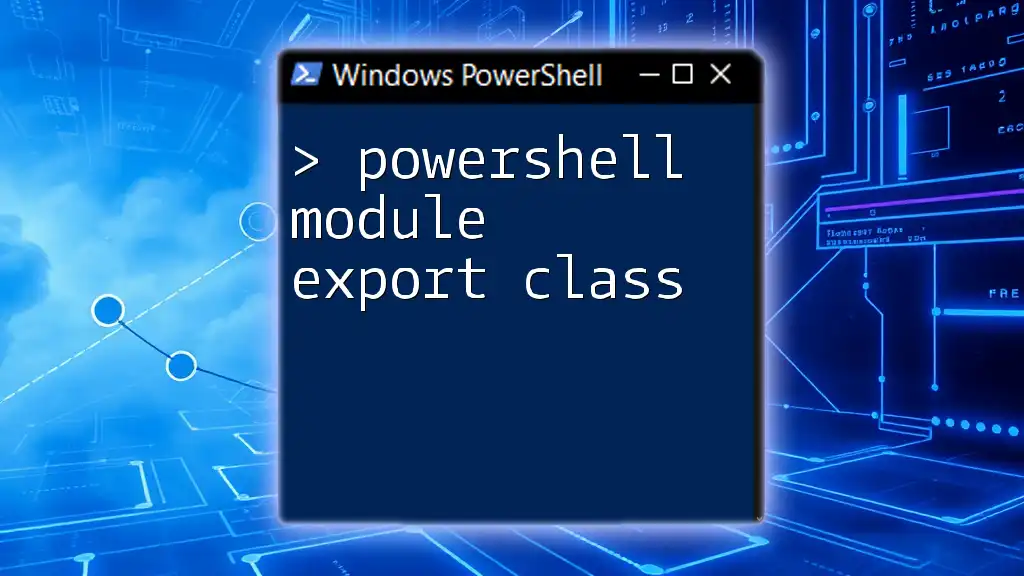
Common Mistakes and How to Avoid Them
Misunderstanding Integer vs. Floating-Point Division
A frequent misconception arises when dealing with integer and floating-point numbers in modulus operations. The two types can yield similar outputs but behave differently under certain conditions.
For example:
# Integer division
$number1 = 10
$number2 = 3
$modulus = $number1 % $number2 # Result is 1
# Floating point
$number3 = 10.0
$number4 = 3.0
$modulusFloat = $number3 % $number4 # Result is still 1
It's crucial to note that while the results may appear similar, the way PowerShell handles integers and floating points can lead to unexpected behavior, especially in larger computations.
Mistakes in Loop Logic
In loop constructs, overlooking logic can lead to inaccurate outcomes. Consider this code snippet:
For ($i = 1; $i -le 10; $i++) {
if ($i % 2 -eq 0) { Write-Output "Even" } # Potentially missing output for condition
}
In scenarios like this, ensuring comprehensive coverage of all conditions is vital. Always validate your conditions and output to guarantee accuracy within your loops.
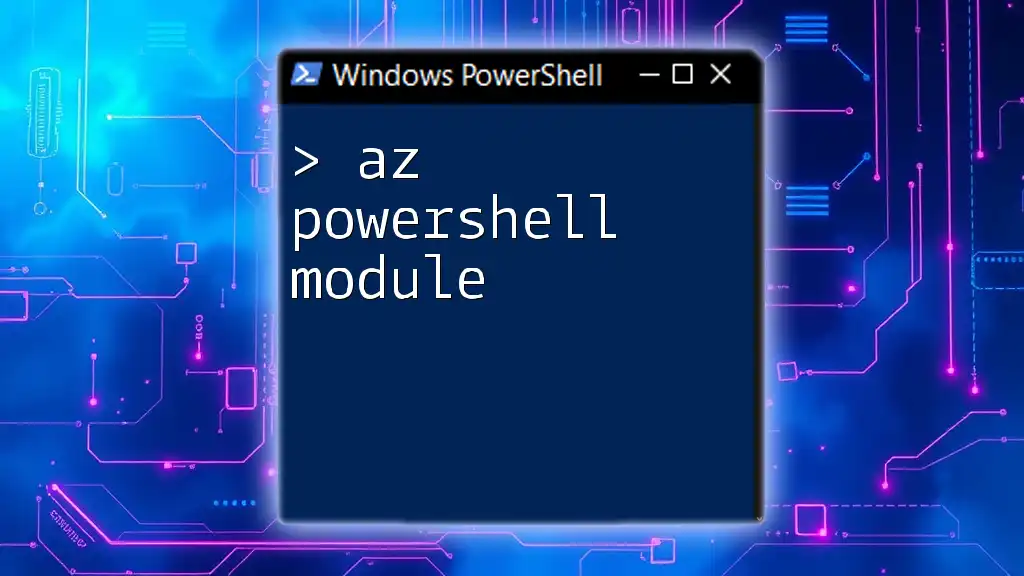
Best Practices for Using Modulus in PowerShell
Clear and Concise Code
When using modulus in PowerShell, prioritize code clarity. Use meaningful variable names and comment your logic effectively to aid understanding. This practice not only benefits you but also assists anyone else reading or maintaining your code.
Use Predefined PowerShell Functions
Leverage PowerShell’s built-in functions to complement your modulus logic. Functions like `Where-Object` can streamline workflows, especially in large datasets, ensuring concise and efficient coding.
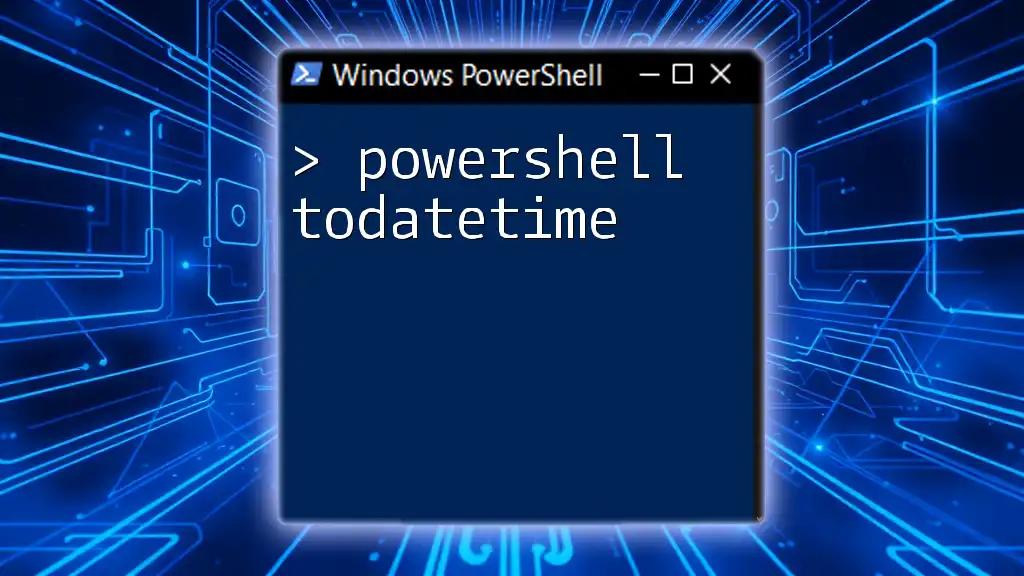
Real-life Use Cases for Modulus
Data Grouping
PowerShell’s modulus function can be instrumental in grouping data efficiently. For instance, you could segregate a list of items based on certain characteristics by utilizing modulus checks.
Event Scheduling
Another prominent case is in event scheduling. By employing modulus, you can set regular intervals for tasks or trigger repeating events smoothly within defined time frames.
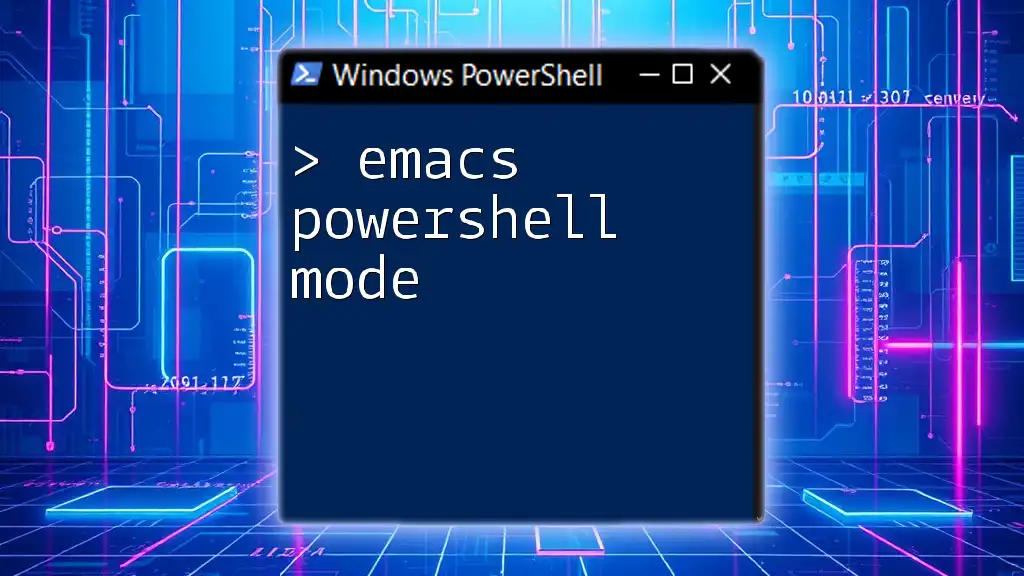
Conclusion
The mod operator in PowerShell is not just a simple mathematical tool; it’s an essential element that can enhance the functionality and efficiency of your scripts. By understanding its applications, syntax, and best practices, you’ll significantly elevate your PowerShell skills.
For those eager to delve deeper into this subject, I encourage you to explore official PowerShell documentation, participate in forums, and practice writing scripts. Your journey to mastering PowerShell will undoubtedly elevate your capability to automate, script, and solve complex problems effectively.