The PowerShell Mouse Jiggler is a simple script that automates mouse movements to prevent a computer from going to sleep or triggering a screensaver.
# Simple Mouse Jiggler Script
Add-Type -AssemblyName System.Windows.Forms
while ($true) {
[System.Windows.Forms.Cursor]::Position = New-Object System.Drawing.Point(0, 0)
Start-Sleep -Milliseconds 500
[System.Windows.Forms.Cursor]::Position = New-Object System.Drawing.Point(1, 1)
Start-Sleep -Milliseconds 500
}
Understanding Mouse Jigglers
What is a Mouse Jiggler?
A Mouse Jiggler is a tool designed to simulate mouse movement, effectively tricking your computer into thinking you are actively using it. This is especially useful in situations where an idle status can lead to screensavers, session timeouts, or other interruptions. Common use cases include keeping presentations active, avoiding session locks during important meetings, and ensuring that long-running scripts or processes remain active.
Types of Mouse Jigglers
There are two main categories of Mouse Jigglers:
-
Physical Mouse Jigglers: These are small devices that physically move your mouse by creating slight vibrations or movements. They are straightforward solutions but may not be practical for everyone.
-
Software Mouse Jigglers: These solutions utilize programming to simulate mouse movement. PowerShell offers a quick and effective way to implement this functionality directly through scripting.
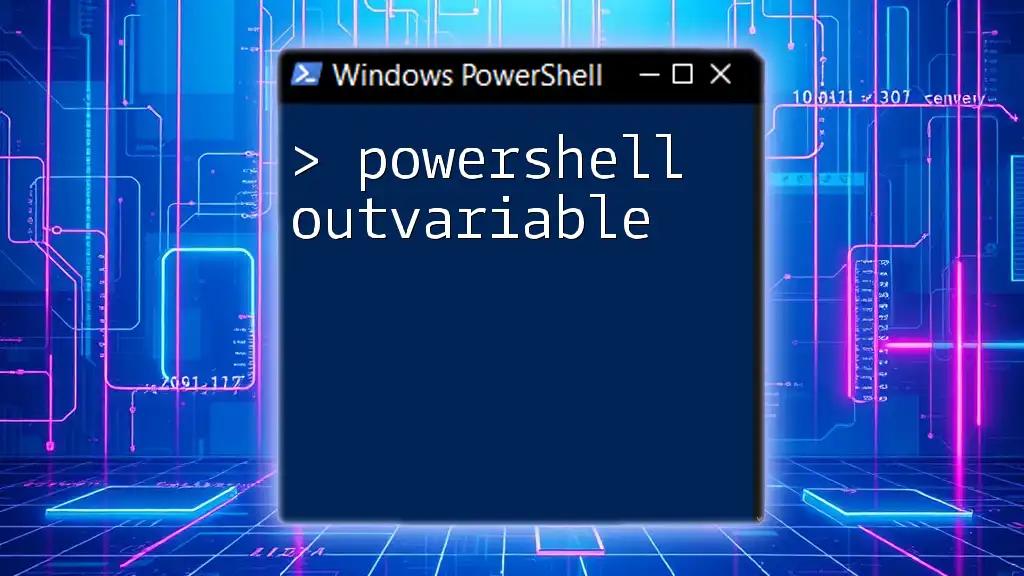
Setting Up Your Environment
Requirements for Running PowerShell Scripts
Before you begin, ensure that you are set up to run PowerShell scripts effectively. First, check your PowerShell version by running `$PSVersionTable.PSVersion` in the console. It's recommended to use PowerShell 5.1 or later for full compatibility with various commands.
Moreover, you may need to adjust your execution policy to allow scripts to run on your machine. You can set the policy with the following command:
Set-ExecutionPolicy RemoteSigned
Lastly, consider using a reliable Integrated Development Environment (IDE) like Visual Studio Code. This will facilitate writing and debugging your scripts.

Creating Your Own Mouse Jiggler in PowerShell
Basic Concept of Mouse Movement
Simulating mouse movement with PowerShell requires access to the Windows API. This is accomplished through the `Add-Type` cmdlet, allowing you to define new types and invoke methods from existing Windows libraries. Utilizing mouse events, you can create a script that generates minimal but effective movements to keep your session alive.
PowerShell Script Example
Here’s a simple example of a Mouse Jiggler script constructed using PowerShell:
Add-Type @"
using System;
using System.Runtime.InteropServices;
public class MouseJiggler {
[DllImport("user32.dll")]
public static extern void mouse_event(uint dwFlags, uint dx, uint dy, uint dwData, int dwExtraInfo);
public const int MOUSEEVENTF_MOVE = 0x0001;
public static void Jiggle() {
mouse_event(MOUSEEVENTF_MOVE, 1, 1, 0, 0);
mouse_event(MOUSEEVENTF_MOVE, -1, -1, 0, 0);
}
}
"@
while ($true) {
[MouseJiggler]::Jiggle()
Start-Sleep -Seconds 60
}
Explanation of the Script
This script defines a new class, `MouseJiggler`, which imports the `mouse_event` function from the `user32.dll` library. The `Jiggle` method uses this function to generate slight movements to the mouse cursor. Executing the script in an infinite loop (`while ($true)`) allows it to continue sending movement signals every 60 seconds, or however long you choose to set it.
Feel free to adjust the `Start-Sleep` duration to meet your needs, whether that’s longer intervals for more discreet activity or shorter intervals to maintain a more constant presence.
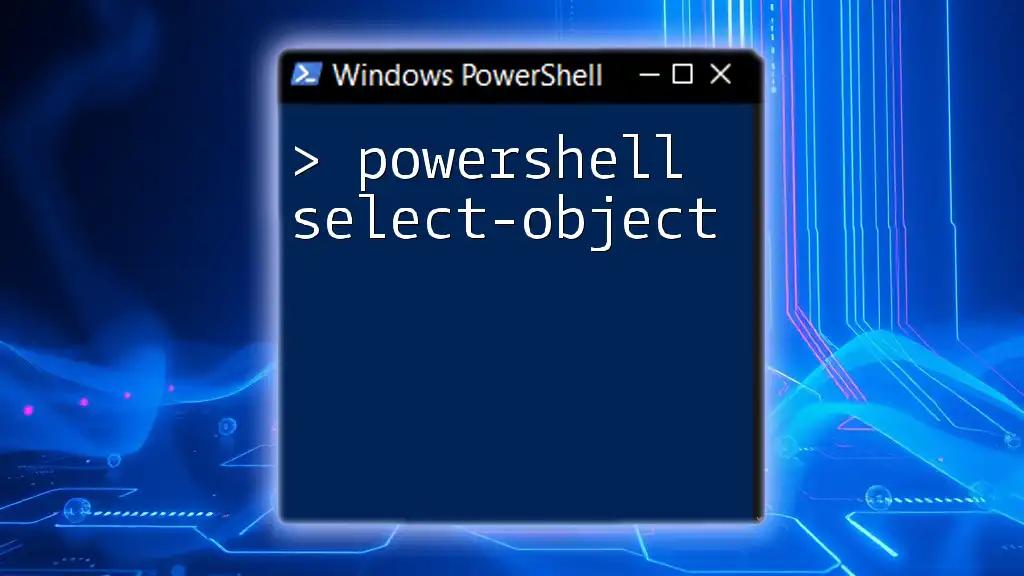
Running Your Script Safely
How to Execute the PowerShell Script
To run the above script, open PowerShell, copy the code into your preferred editor, and execute it. Be mindful of any errors that could arise, such as issues related to script execution policies. If you encounter errors, take the time to revise any permissions or consult documentation for troubleshooting.
Stopping the Script
Stopping the script can be as simple as closing the PowerShell window or terminating the process through Task Manager. However, be careful with abrupt termination, as it may leave system processes in an unpredictable state. Instead, using `CTRL + C` in the PowerShell window is a more graceful way to halt execution.
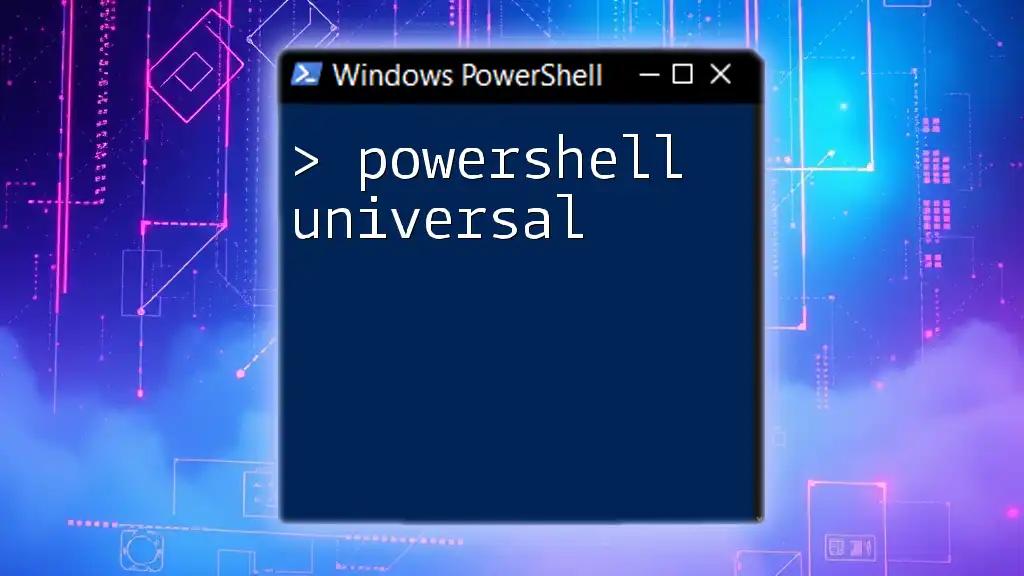
Use Cases for a PowerShell Mouse Jiggler
For Remote Workers
In today’s remote work environment, maintaining active sessions can be pivotal. With a PowerShell Mouse Jiggler, you can easily avoid interruptions that lead to rich collaboration tools becoming unresponsive. This means no more worrying about your connection being dropped during important online meetings.
In Educational Settings
Educators can also utilize this technology effectively. When giving presentations, a Mouse Jiggler can prevent screens from turning off, ensuring that visuals remain accessible. This prevents disruption during interactive plans that require student participation.
In Server Management
For IT professionals managing servers, avoiding unnecessary timeouts is critical. A Mouse Jiggler ensures your monitoring interfaces remain active, so you can maintain a vigilant watch over vital processes without fearing that your connection will time out.
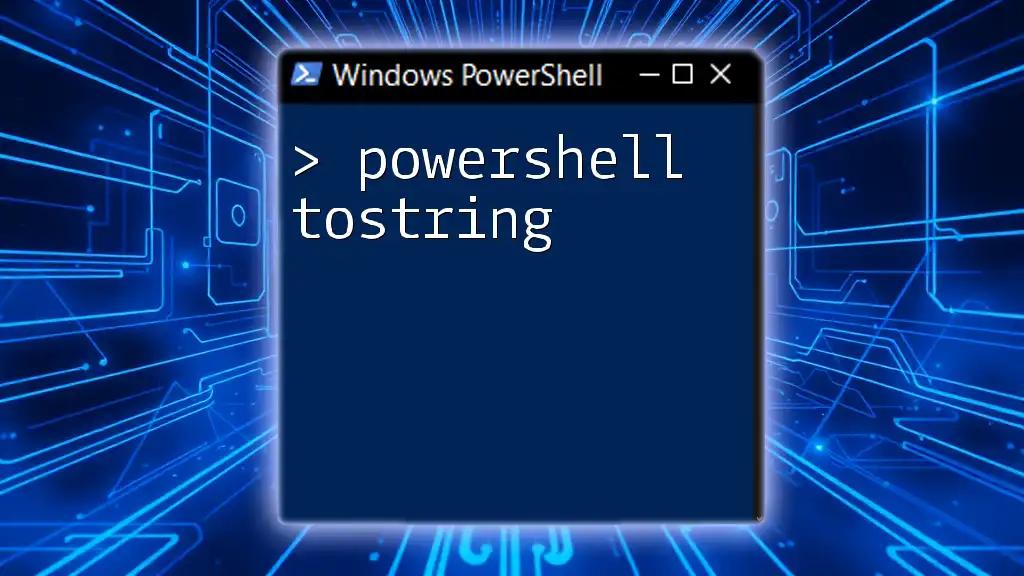
Alternatives to PowerShell Mouse Jiggler
Other Software Mouse Jiggler Options
While PowerShell is a robust solution, several software alternatives exist that can also serve as Mouse Jigglers. Applications like "Mouse Jiggler" or "Caffeine" can be downloaded for quick installation and easy usage without scripting. However, many of these may lack customization and could require additional system resources.
Using PowerShell with Other Technologies
For those interested in expanding functionality, consider integrating your Mouse Jiggler with task schedulers or creating a GUI using Windows Forms. This allows users to start, stop, or modify the settings from a user-friendly interface, enhancing usability for non-tech-savvy individuals.
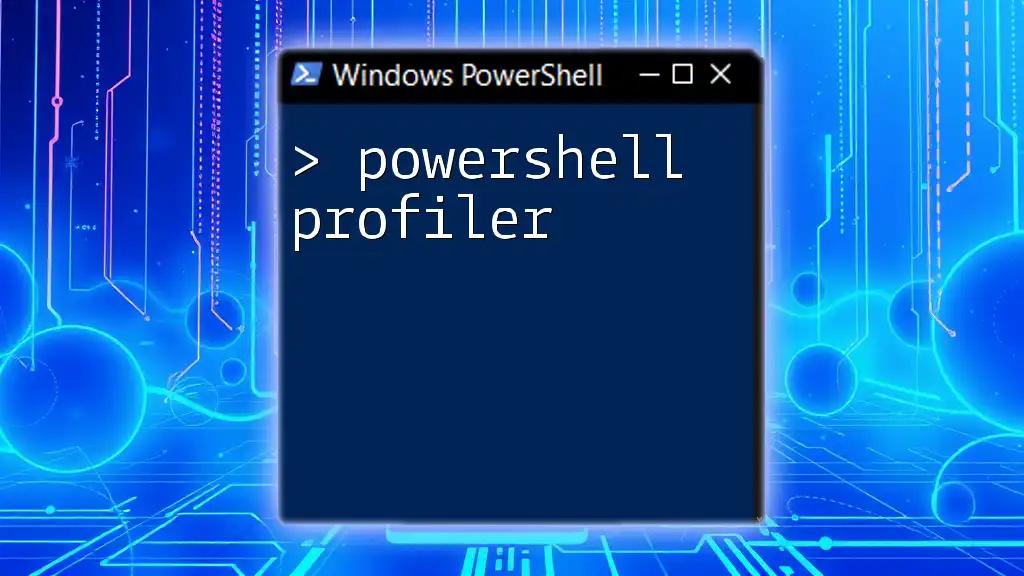
Best Practices and Considerations
Ethical Use of Mouse Jigglers
While a PowerShell Mouse Jiggler can significantly boost productivity, it’s essential to use it ethically and transparently. Always be aware of your workplace's policies regarding software and automation. Engaging in conversation with management or your IT department can help avoid potential misunderstandings.
Performance Considerations
Lastly, while implementing a mouse jiggler, it’s critical to monitor the impact on your system. In most cases, the performance hit will be negligible, but each environment is unique. Evaluating necessity before deployment will ensure that you do not overburden resources.
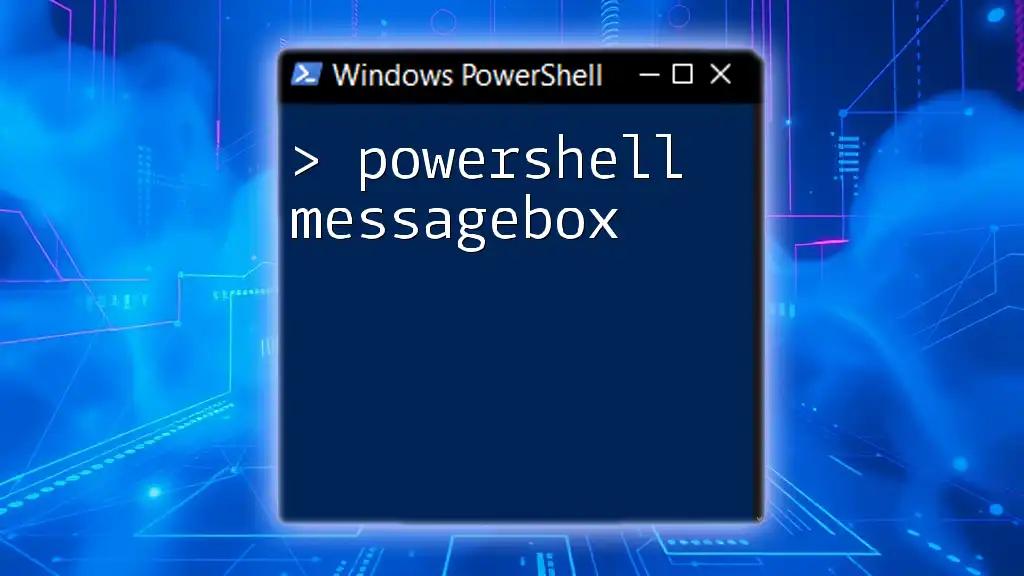
Conclusion
With a comprehensive understanding of the PowerShell Mouse Jiggler, you're now equipped to implement this tool effectively. Not only does this enhance your workflow by keeping sessions active, but it also empowers you to harness the capabilities of PowerShell for other automation tasks. Remember to experiment with the script and customize it for your specific needs.
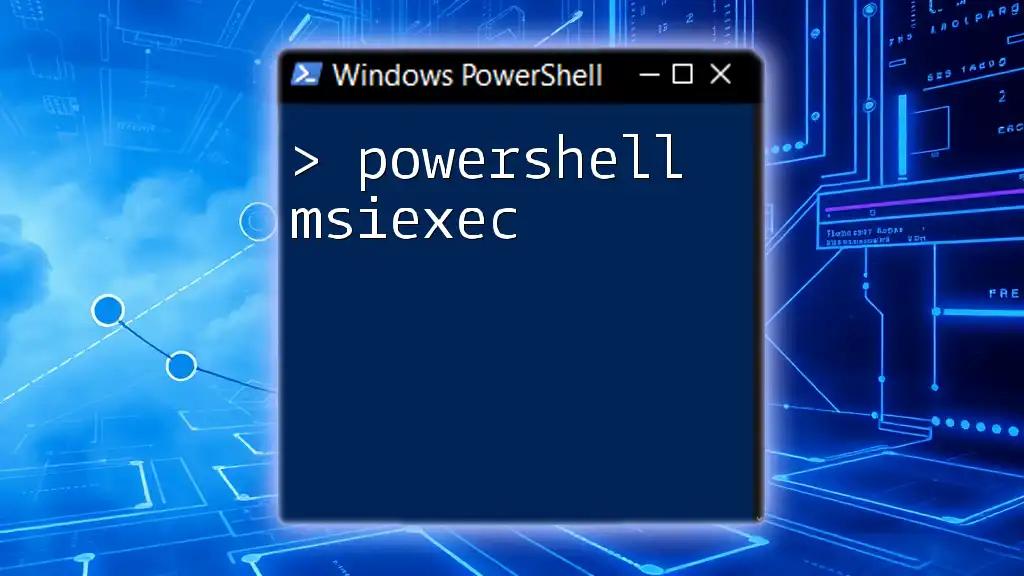
Additional Resources
Recommended Reading and Tools
Dive deeper into PowerShell scripting with official documentation available through Microsoft. Resources like online tutorials, forums, and PowerShell communities can provide invaluable support as you navigate this powerful tool’s offerings.
Community and Support
Joining PowerShell forums or participating in community discussions can help you stay updated with best practices and innovations in PowerShell scripting. Engaging with like-minded individuals can inspire new ideas and solutions to common challenges, enhancing your learning experience.