The `Out-Variable` parameter in PowerShell allows you to store the output of a command into a specified variable for later use, enhancing your script's flexibility and efficiency.
Here's an example code snippet demonstrating this:
Get-Process | Out-Variable myProcesses
In this example, the output of the `Get-Process` command is stored in the variable `$myProcesses`.
Understanding PowerShell Outputs
PowerShell is fundamentally designed to facilitate command-line scripting. One of the core concepts that PowerShell utilizes is the pipeline, where the output of one command can easily flow into another. By default, PowerShell handles outputs in a straightforward manner, primarily displaying them in the console. However, this can sometimes lead to limitations when you want to reuse or manipulate those outputs later on. This is where the `OutVariable` parameter becomes transformative.
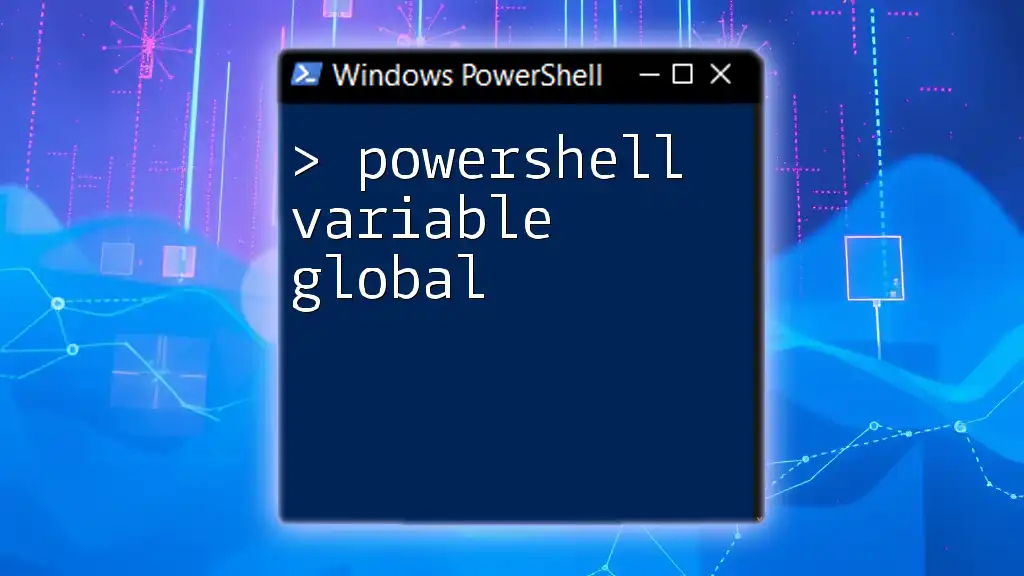
What is OutVariable?
The `OutVariable` parameter in PowerShell allows users to store command outputs into a variable without the need for traditional assignment. This is particularly beneficial when you want to reference the output later in your script or when you want to chain multiple commands without losing track of previous outputs.
In essence, OutVariable captures the output of a command executed in a pipeline and allows you to continue processing that output later without cluttering the console or using intermediate variables.
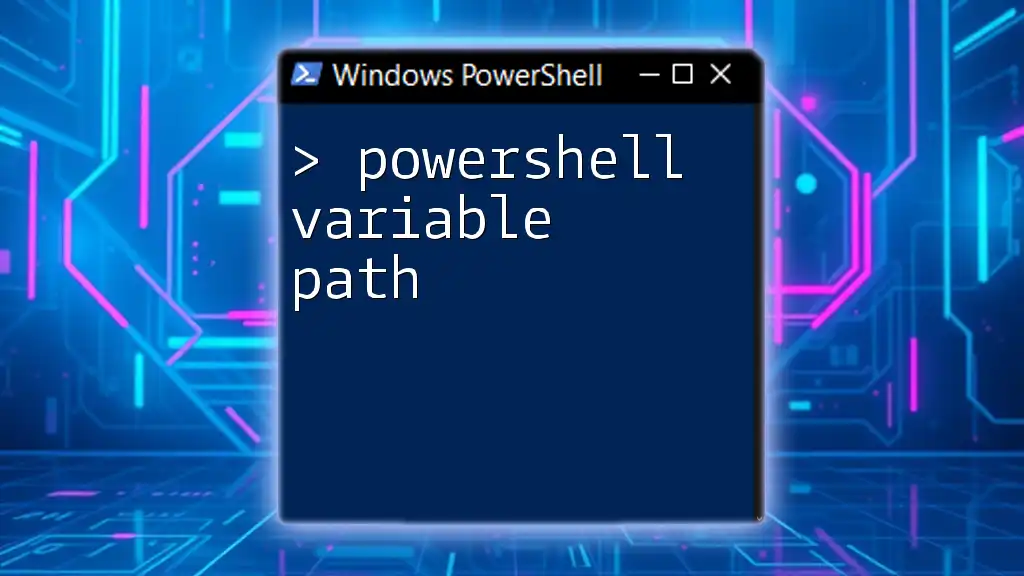
Benefits of Using OutVariable
Enhanced Scripting Efficiency
Utilizing the OutVariable parameter can vastly increase the efficiency of your scripts. When you're collecting and analyzing data from commands, overwriting variables every time can lead to code that is not only less efficient but also more challenging to read.
For example, instead of running a command, getting its output, and then manually assigning it to a variable, you can succinctly use:
Get-Process -OutVariable processList
This enables you to both view the process list and use it for additional commands later on.
Error Handling and Debugging
Another key benefit of using OutVariable is its impact on error handling and debugging. If a command generates an error, but you have an OutVariable capturing the intended output, debugging becomes significantly easier. You can check the contents of the OutVariable even if the command fails:
Get-Service -Name 'NonExistentService' -OutVariable serviceInfo
Write-Host "Service Info: $serviceInfo"
In cases where you are uncertain about command safety or outcomes, having those outputs stored can save time and headaches.
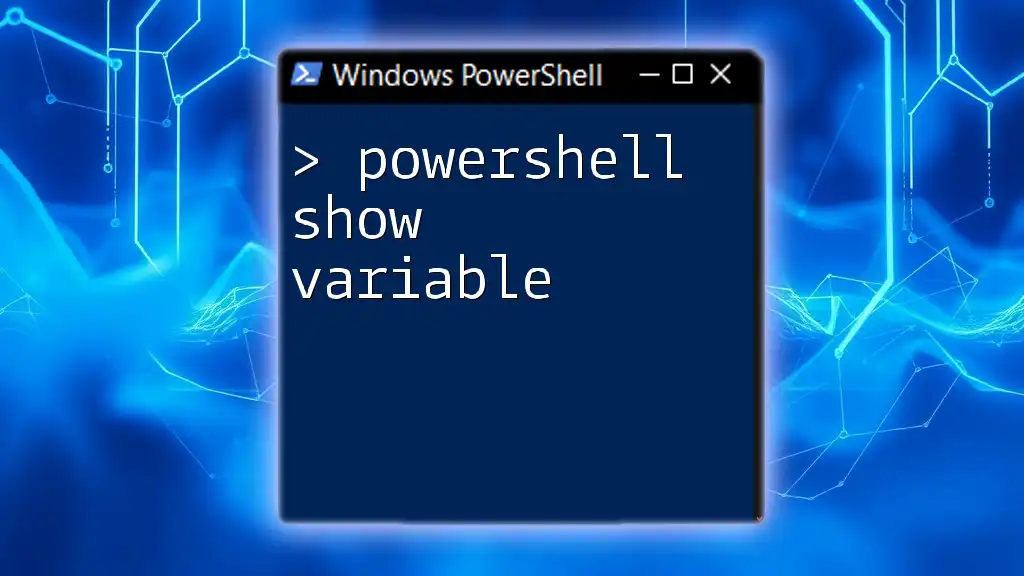
Basic Syntax of OutVariable
The syntax of the OutVariable command is indeed straightforward. The general structure follows that of any PowerShell cmdlet but includes the `-OutVariable` parameter followed by the variable name you want to store the output into.
For example:
Get-Command -OutVariable cmdList
This command fetches all available commands in PowerShell and stores them in the variable `cmdList`.
Where to Place the -OutVariable Parameter
It’s essential to know where to position the `-OutVariable` parameter. Unlike some parameters that can be placed after the cmdlet or at the end of the command chain, OutVariable must be included in the command that generates the output.
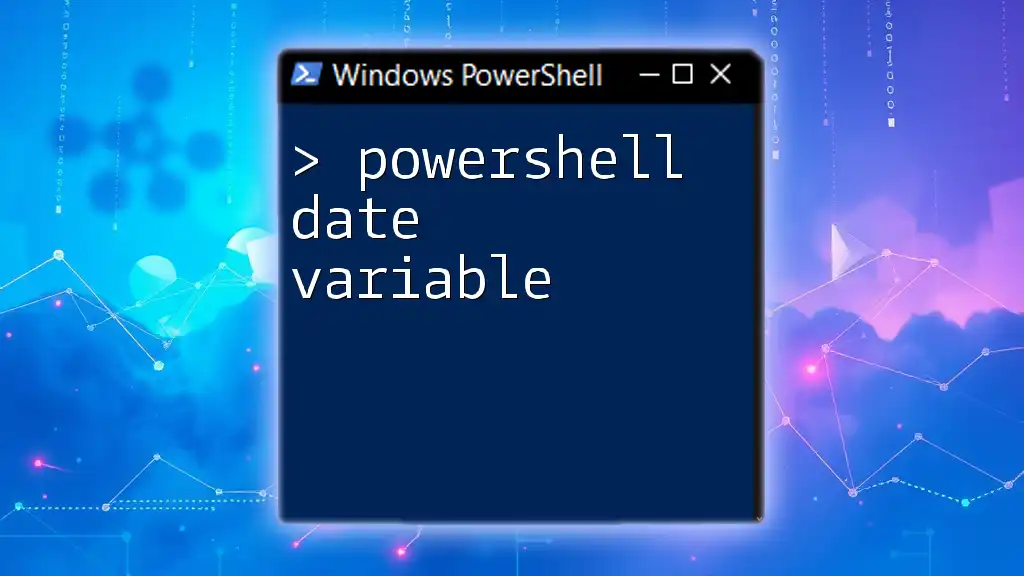
Practical Examples of OutVariable
Storing Command Output in Variables
One of the simplest yet powerful usages of OutVariable is during command execution where you want to work with the output later. For instance:
Get-Command -OutVariable cmdList
Write-Host "Stored commands: $cmdList"
This not only allows you to see the available commands on the screen but also keeps them stored for any further operations.
Using OutVariable with Multiple Cmdlets
Chaining commands is a common practice in PowerShell scripting. Here’s how you can efficiently use OutVariable:
Get-Service | Where-Object { $_.Status -eq 'Running' } -OutVariable runningServices
Write-Host "Running services stored: $runningServices"
This retrieves all running services and stores them in `runningServices`, enabling you to process the variable later without losing the initial output data.
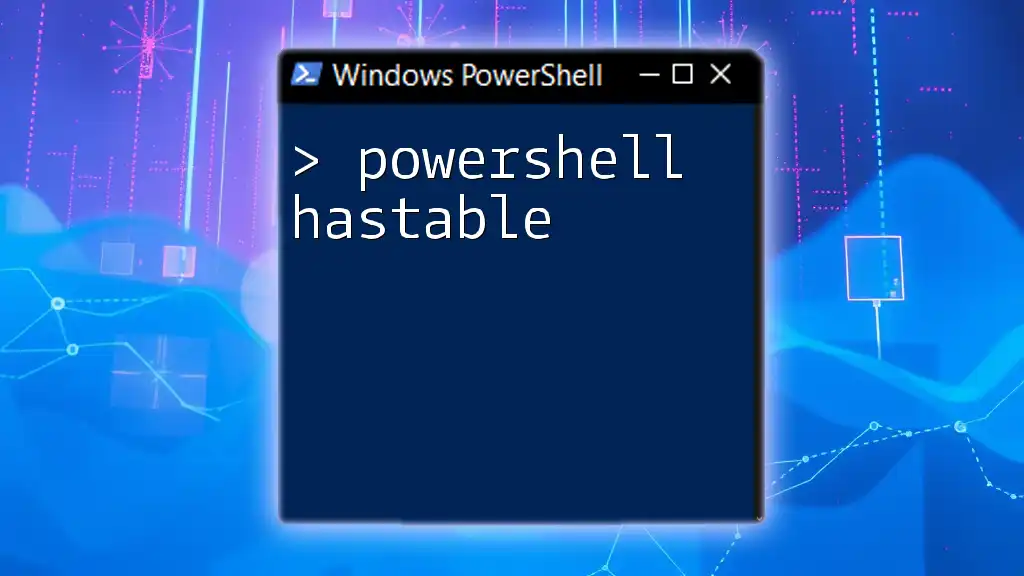
Best Practices for Using OutVariable
Naming Conventions
Using clear and descriptive variable names is crucial for maintainability. By using names that reflect the data contained, you ensure that your scripts remain understandable even after a period of time.
Limiting Scope of Variables
PowerShell can create numerous variables during script execution. To avoid clutter and potential conflicts, limit the number of OutVariables and consider cleaning them up after their use, especially in larger scripts.
Commenting Your Code
Appropriate documentation through comments is essential. Each time you use an OutVariable, include comments that clarify what data is being captured and how it will be utilized later. This practice will make your code accessible to others and your future self.
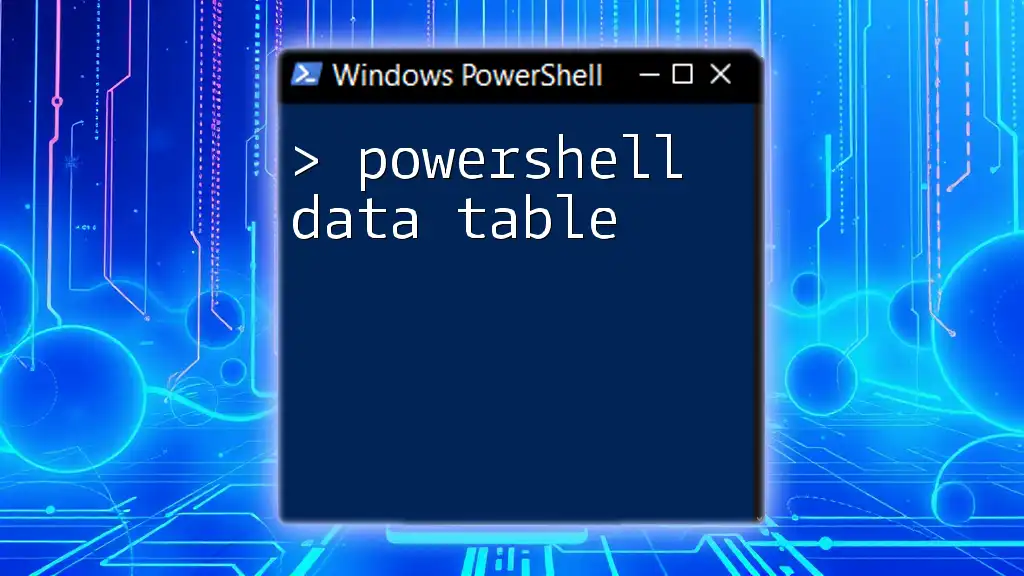
Common Mistakes to Avoid
Overusing OutVariable
While OutVariable is a powerful tool, it’s important to recognize when its use is unnecessary. If the output of a command is not needed later, there’s little point in capturing it. Avoid excessive variable creation as it can lead to confusion and poor performance.
Not Managing Variable Output
Failing to manage the size and contents of variables can eventually degrade the performance of your scripts. Remember to clear or manage large OutVariables responsibly to prevent memory bloat.
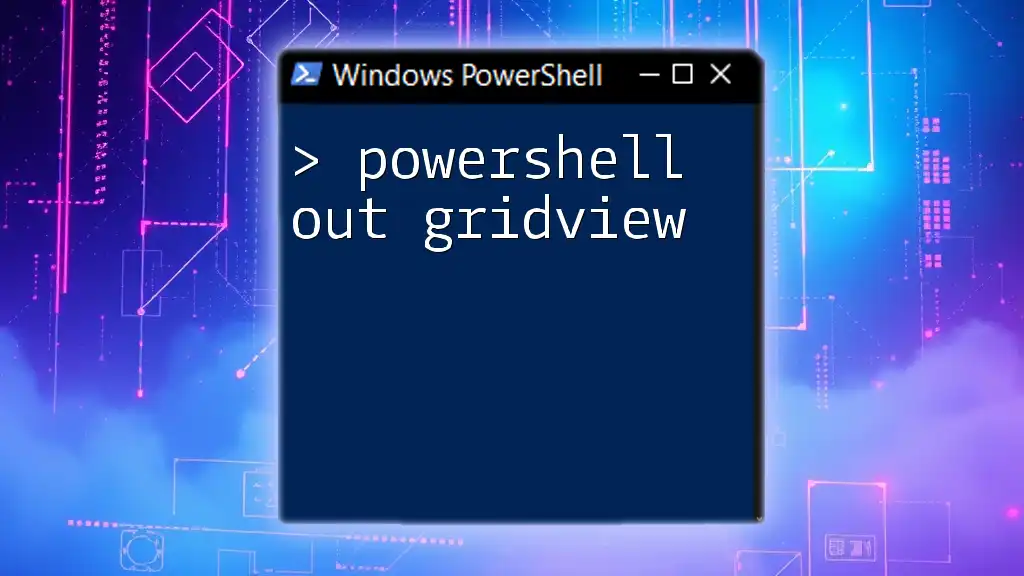
Conclusion
The OutVariable parameter is an indispensable feature of PowerShell that elevates your scripting capabilities. By improving efficiency, handling errors effectively, and simplifying outputs management, OutVariable proves invaluable for both novice users and seasoned scripters alike.
Consider integrating OutVariable into your scripting practices to enhance performance and readability. As you continue your PowerShell journey, you'll discover even more ways this feature can streamline your workflow.
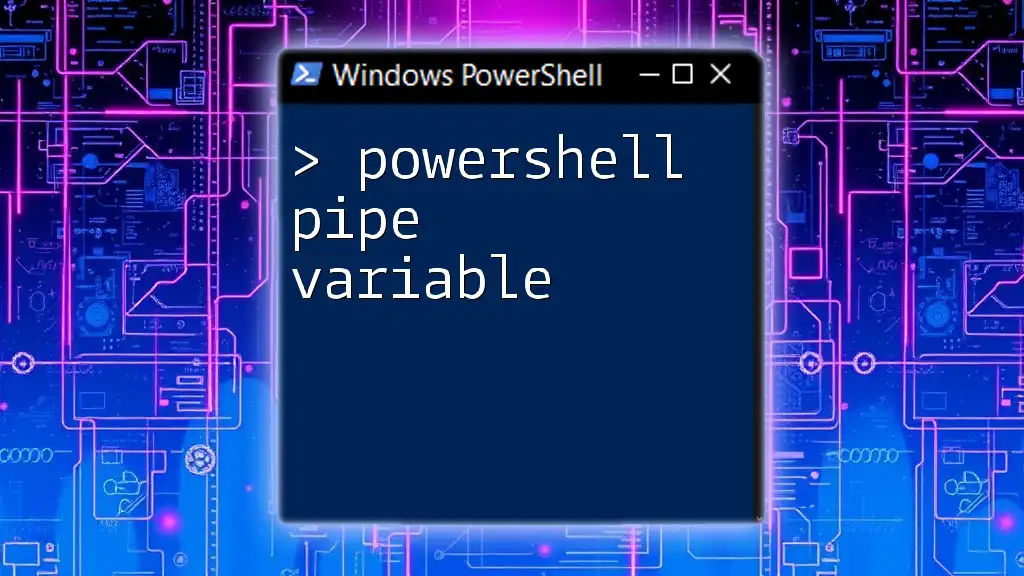
Additional Resources
For further insight, explore related commands like `Select-Object` and `Export-Csv` for advanced output manipulation techniques. Engaging with the PowerShell community on forums and social networks can also provide ongoing support and knowledge as you enhance your scripting skills.