In PowerShell, a variable can store a path to a file or directory, making it easier to reference and manipulate in your scripts. Here's a simple example:
$path = "C:\Users\YourUsername\Documents"
Write-Host "The variable path is: $path"
What is a PowerShell Variable?
Definition of PowerShell Variables
In PowerShell, a variable is a named storage location where you can hold data. Variables allow you to store information temporarily and use it throughout your script or session, making them essential for automating tasks and managing data efficiently.
Types of PowerShell Variables
PowerShell supports various types of variables, each tailored for specific scenarios:
-
Scalar Variables: These variables store a single value. Their simplicity makes them highly useful for basic tasks. For example:
$name = "John Doe"
Here, `$name` becomes a container holding the string "John Doe".
-
Array Variables: These allow you to store multiple values in a single variable. Arrays are excellent when you need to manage collections of items. For instance:
$fruits = @("Apple", "Banana", "Cherry")
This assigns an array of strings to the `$fruits` variable. You can access elements in the array using an index.
-
Hash Table Variables: Hash tables are key-value pair collections that provide a robust way to associate related data. For example:
$person = @{"Name"="John"; "Age"=30}
In this case, `$person` holds information about an individual, linked by meaningful keys.
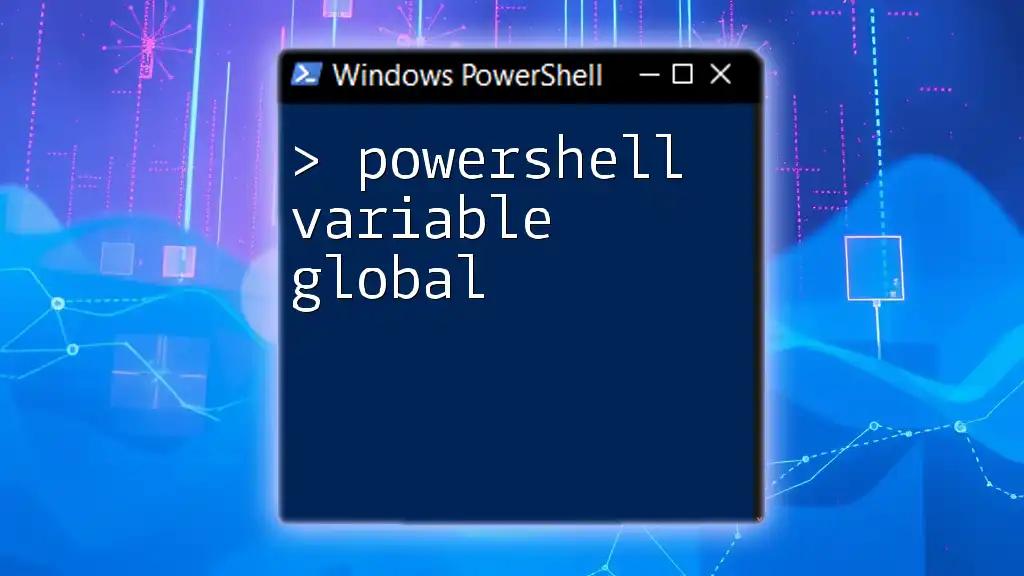
Understanding Variable Paths in PowerShell
What is a Variable Path?
A PowerShell variable path is a way to reference and access the data contained in variables. Variable paths are particularly important when working with complex data structures, as they let you navigate through layers of variables to retrieve or manipulate data.
Default Variable Paths
PowerShell provides default variables that can be used at any time. They hold critical system information and settings that facilitate user operations. Some common default variables include:
-
$PSHOME: This variable points to the installation directory of PowerShell. It can be accessed with:
$PSHOME
-
$Profile: This variable is related to user profiles. It contains the path to your PowerShell profile file, which runs every time PowerShell starts. You can display its path using:
$Profile
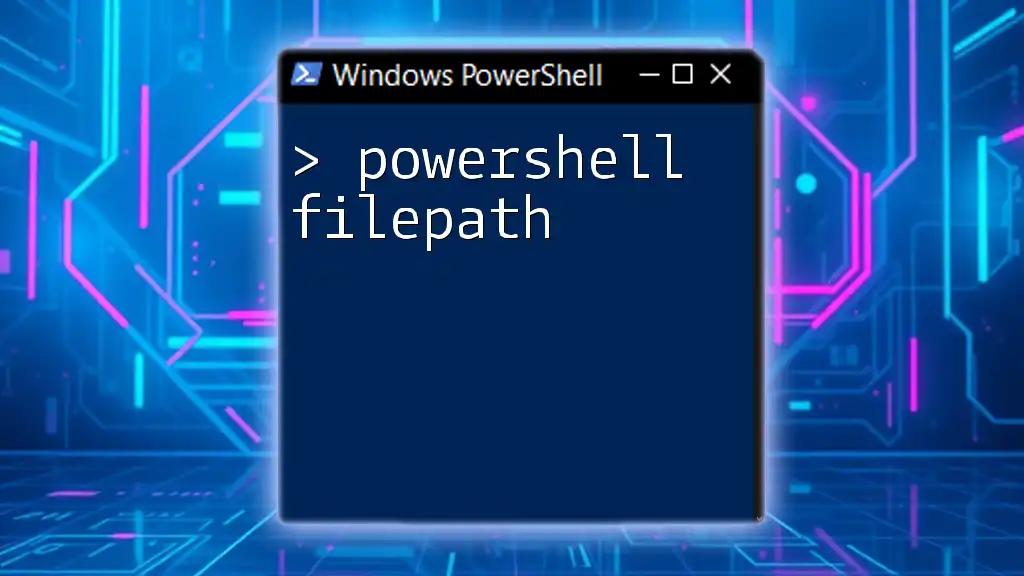
Working with Variable Paths
Accessing Variable Paths
To utilize variable paths effectively, it's crucial to understand their scope. Variables in PowerShell have different scopes, which dictate how they can be accessed:
-
Local Variables: These are accessible only within the function or script where they were defined.
-
Global Variables: These can be accessed from anywhere in your PowerShell session. You can define a global variable like this:
$Global:myGlobalVar = "Hello"
Modifying Variable Paths
After defining your variables, you might need to modify their values. For example, if you had an array of numbers and wanted to update the first element, you could do it as follows:
$myArray = @(1, 2, 3)
$myArray[0] = 10
This sets the first element of `$myArray` to 10.
Exploring Nested Variable Paths
In PowerShell, it's possible to create complex data structures, such as nested variables. For example, you can define a hash table containing another hash table to represent a person's details and their address:
$person = @{
Name = "John"
Address = @{
City = "New York"
State = "NY"
}
}
$city = $person.Address.City
Here, `$city` will yield "New York" as it drills down into the nested hash table associated with `$person`.
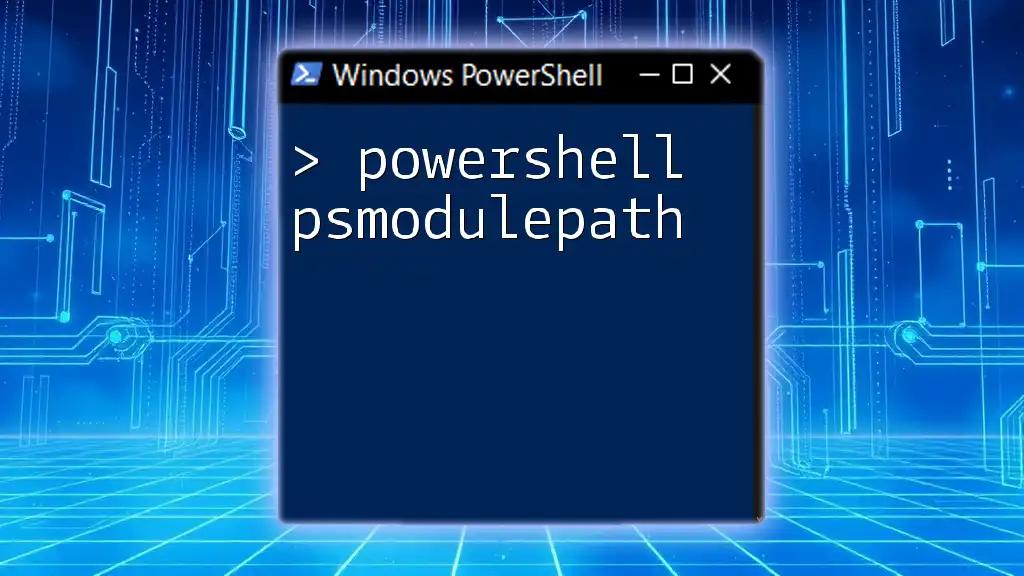
Best Practices for Using PowerShell Variable Paths
Naming Conventions
It's essential to give your variables meaningful names. Descriptive names not only improve the readability of your code but also make it easier for you and others to understand the purpose of each variable. Common conventions include camelCase and PascalCase.
Scope Management
Understanding the scope of variables is crucial to avoid conflicts. By controlling the scope, you can ensure that functions or scripts do not unintentionally overwrite each other’s data. Always consider the scope when defining variables, especially in larger scripts.
Cleaning Up Variables
It's a good practice to remove unused variables after their purpose has been served. This helps in managing memory and keeping the environment clean. You can remove a variable using:
Remove-Variable -Name myVariable -Scope Global
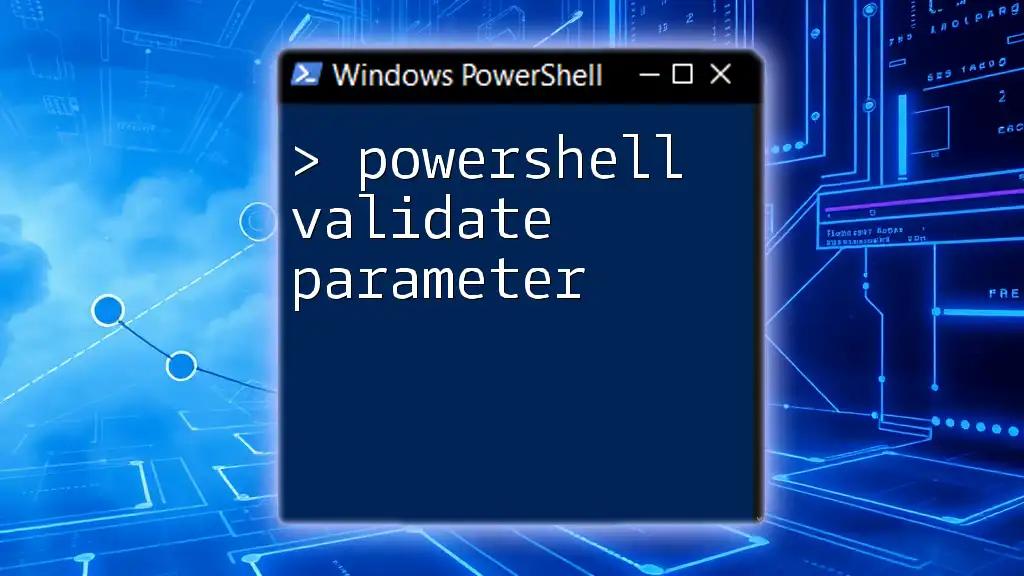
Troubleshooting Common Issues
Variable Not Found Errors
One of the most common issues when dealing with variables is receiving a "variable not found" error. This usually happens due to misspellings, incorrect paths, or scope. Always check for typos and confirm that the variable is defined in the correct scope.
Scope Conflicts
If you have variables with the same name in different scopes, you may encounter conflicts. Be aware of which variable you are accessing by using the correct scope modifier (`$Global:`, `$Local:`, etc.). This helps prevent unexpected results.
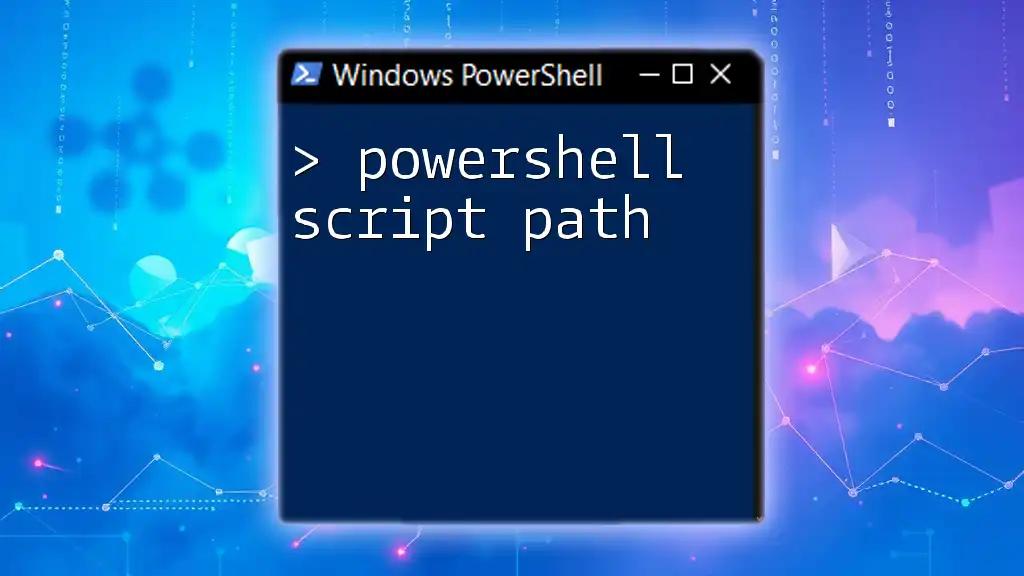
Conclusion
Understanding PowerShell variable paths is critical for effective scripting and automation. By mastering how to define, access, and manage variables and their paths, you can write more efficient and error-free PowerShell scripts. Practicing these concepts will enhance your PowerShell skills and confidence, enabling you to automate tasks with finesse.
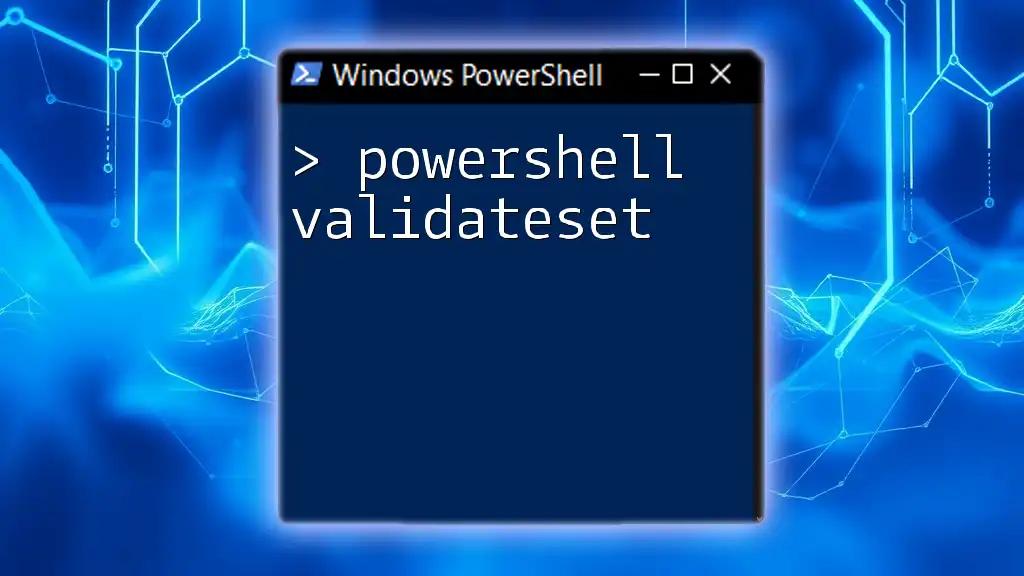
Additional Resources
For further learning, consider exploring recommended books, online courses, and the official PowerShell documentation. These resources can provide deeper insights and advanced techniques to enhance your PowerShell capabilities.
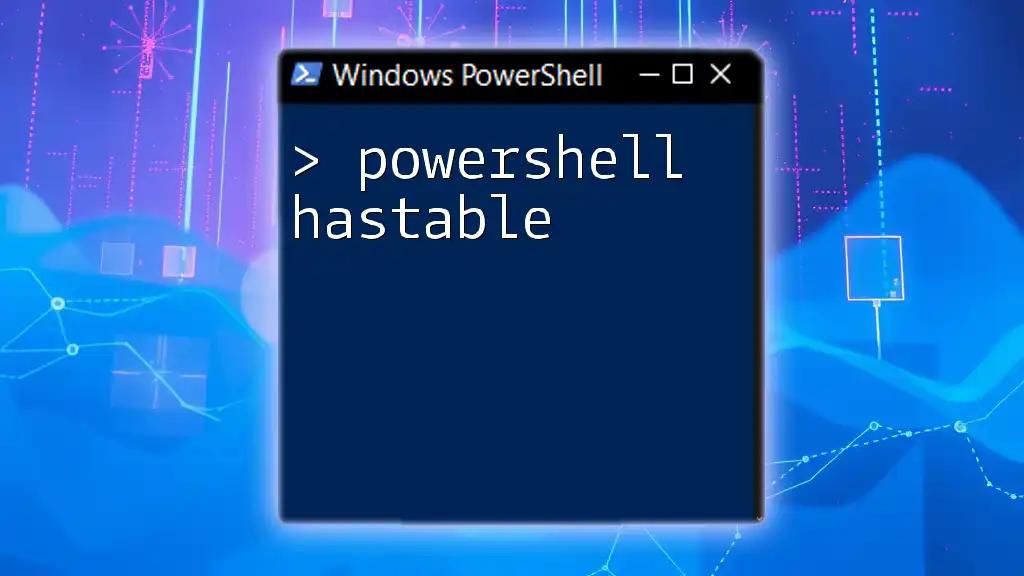
FAQs About PowerShell Variable Paths
What is the difference between local and global variables?
Local variables are accessible only within the context they are defined, while global variables can be accessed from anywhere in your PowerShell session.
How can I list all defined variables?
You can easily list all defined variables using the command:
Get-Variable
Can I use special characters in variable names?
While you can include certain special characters in variable names, it's prudent to stick to alphanumeric characters and underscores for readability and to avoid potential issues.