The `Join-Path` cmdlet in PowerShell is used to combine a path and a child path into a single path string, ensuring that the correct directory separator is used.
$fullPath = Join-Path -Path 'C:\Users' -ChildPath 'Documents\Report.txt'
Write-Host $fullPath
Understanding Paths in PowerShell
What is a Path?
In computing, a path refers to a string that specifies the unique location of a file or directory within a file system. In PowerShell, understanding how to work with paths is essential for effective scripting and automation. Paths can be categorized into absolute paths, which provide the full location starting from the root of the file system, and relative paths, which are defined in relation to the current working directory.
Why Use Join-Path?
The cmdlet Join-Path plays a critical role in managing paths by allowing you to combine multiple path segments seamlessly. Using `Join-Path` is preferable over manual string concatenation for several reasons:
- Error Prevention: When constructing paths manually, it's easy to overlook slashes or accidentally create incorrect paths. `Join-Path` automatically manages these aspects for you.
- Code Readability: Scripting in a clear and concise manner increases code maintainability. With `Join-Path`, your intent is immediately clear to anyone reviewing your code.
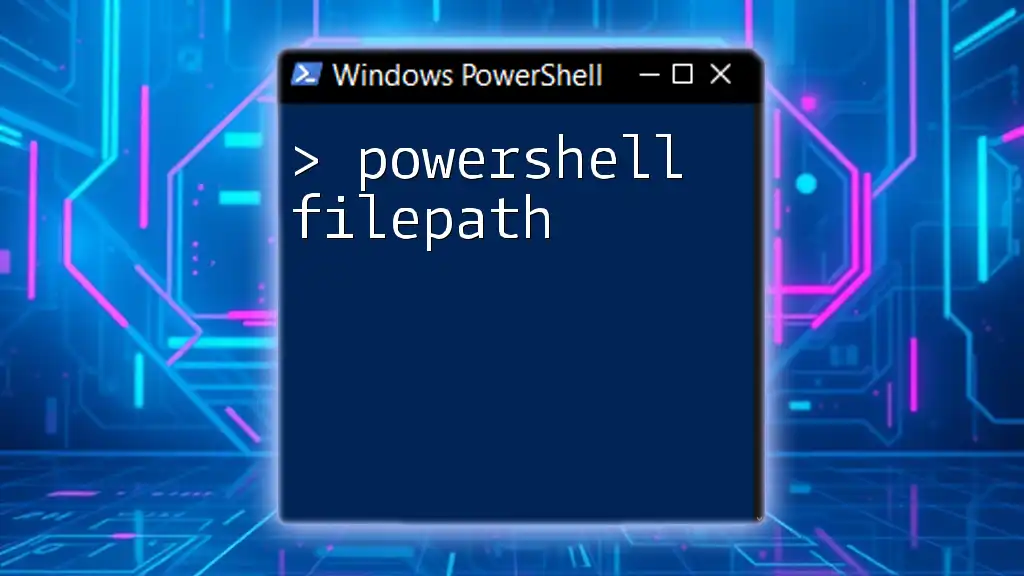
Getting Started with Join-Path PowerShell
Basic Syntax of Join-Path
The basic structure of the `Join-Path` cmdlet is straightforward:
Join-Path -Path 'C:\Users' -ChildPath 'Documents'
In the example above, the cmdlet takes two parameters: `-Path` and `-ChildPath`. The result will be a combined path of `C:\Users\Documents`, showcasing how nicely `Join-Path` simplifies path construction.
Key Parameters
Path Parameter
The `-Path` parameter is the primary input for your base directory. It represents the main location from which you want to build your path. It accepts both absolute and relative paths.
ChildPath Parameter
The `-ChildPath` parameter is where you specify the additional directory or file you want to append to the `Path`. If `ChildPath` is specified without a leading backslash, it will be appended to `Path` correctly.
Default Behavior
`Join-Path` has a set of default behaviors which help streamline your scripting process. For instance, when using paths with trailing separators:
Join-Path -Path 'C:\Users\' -ChildPath 'Public'
In this case, `Join-Path` effectively produces `C:\Users\Public`, demonstrating its ability to handle trailing slashes without creating unnecessary duplicates.
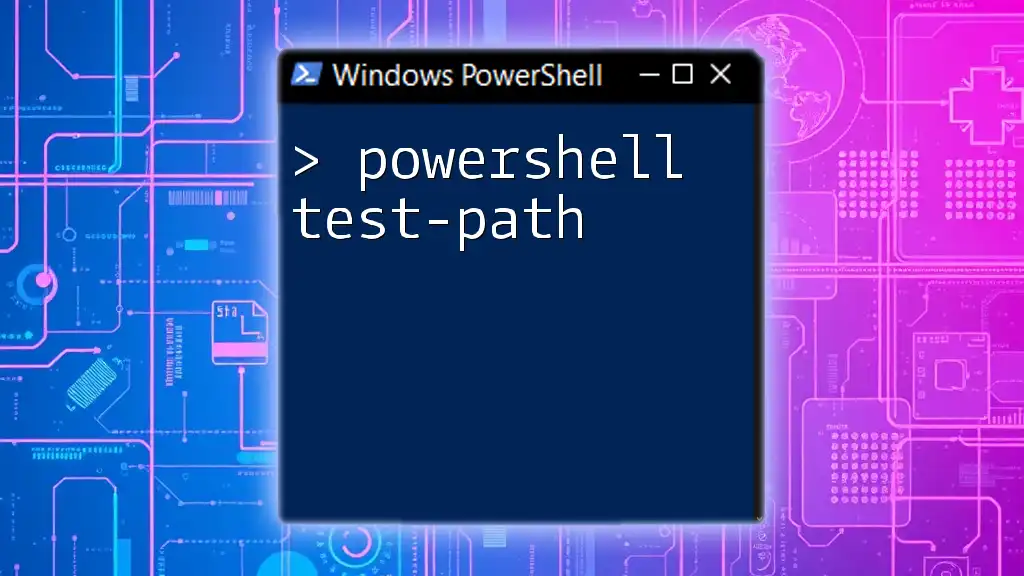
Practical Use Cases of Join-Path PowerShell
Combining Directories
Real-world scenarios often require you to merge directory paths. For instance, when you want to navigate to a script folder within your projects, you might write:
$path = Join-Path -Path 'C:\Projects' -ChildPath 'Scripts'
This command keeps your code clean and minimizes the chance for mistakes.
Constructing File Paths
When dealing with specific files, constructing paths becomes crucial. Let's say you need to access a presentation file within a user’s Documents. You could do so with:
$filePath = Join-Path -Path 'C:\Users\John' -ChildPath 'Documents\presentation.pptx'
This approach allows PowerShell to handle path formatting while you just specify the locations.
Dynamic Path Building
For more complex scripts, dynamic path building using variables can greatly enhance your efficiency. Consider a scenario where you are manipulating date-specific reports:
$baseDir = 'C:\Data'
$subFolder = '2023\Reports'
$fullPath = Join-Path -Path $baseDir -ChildPath $subFolder
Here, `$fullPath` results in `C:\Data\2023\Reports`, allowing for easier adjustments and readability in your scripts.
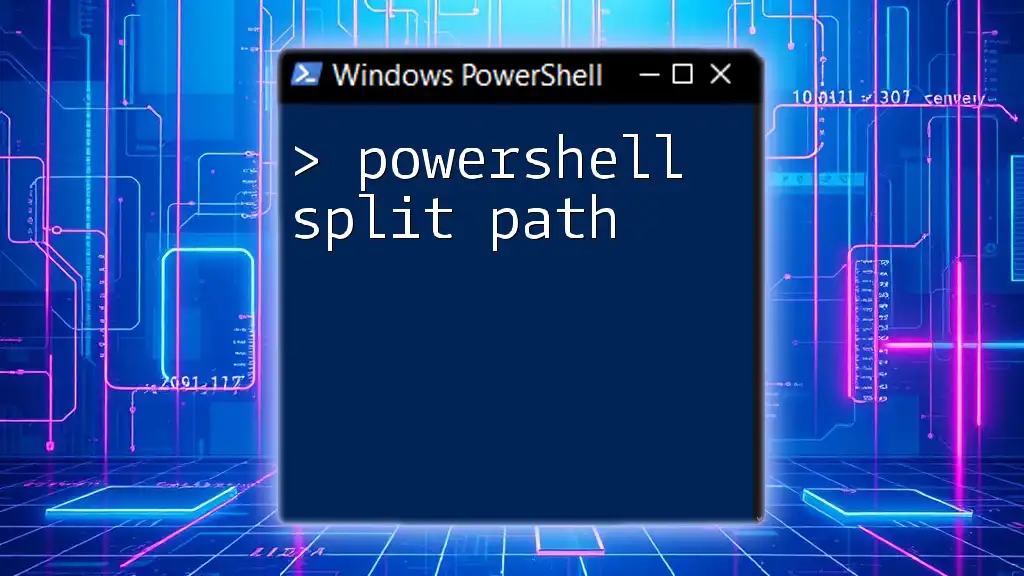
Additional Tips for Using Join-Path
Handling Multiple Child Paths
If your project requires handling multiple child paths, you can nest `Join-Path` calls like this:
$nestedPath = Join-Path -Path (Join-Path -Path 'C:\Data' -ChildPath '2023') -ChildPath 'Reports'
This technique ensures that you maintain clarity and organization within your scripts.
Using Join-Path in Scripts
Incorporating `Join-Path` within automated scripts is best practice. Not only does it bolster your code's reliability, but it also simplifies changes. Instead of revisiting concatenation methods, you can focus on adjusting path parameters.
Common Pitfalls
When using file paths, mistakes are common. The wrong path can cause scripts to fail or lead to poor performance. Being mindful of path conventions helps avoid errors. For instance, accidental trailing slashes can alter results, so ensure your paths are formatted correctly.
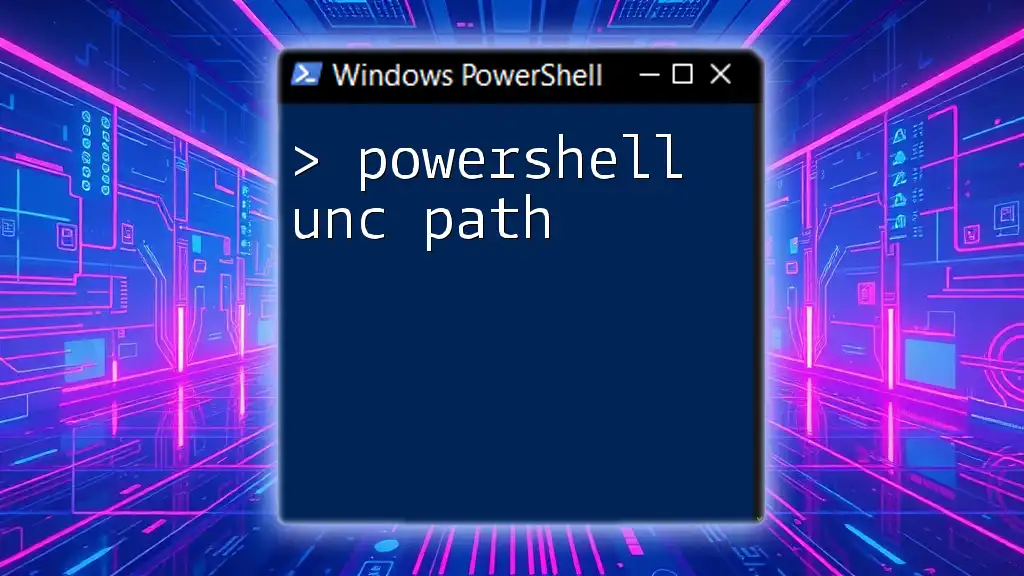
Alternatives to Join-Path
Manual Path Manipulation
While you can certainly combine paths using manual string concatenation, this method is usually more error-prone. Strongly consider using `Join-Path` to make your scripts cleaner and easier to read.
Other Path-Related Cmdlets
PowerShell also includes other cmdlets that handle paths, such as `Resolve-Path` and `Split-Path`. These can be incredibly useful in different contexts, particularly when you need to resolve or dissect existing paths.
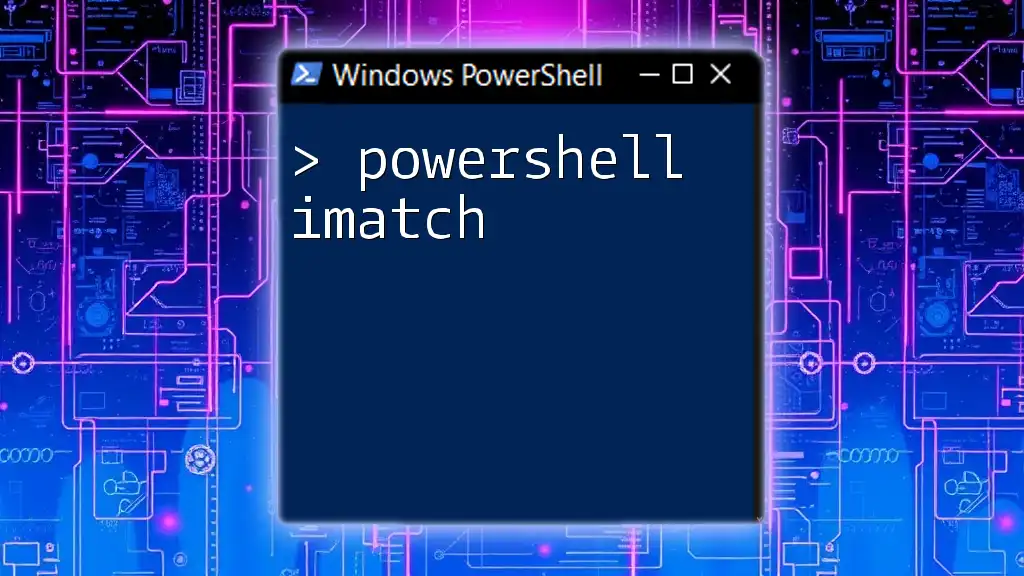
Conclusion
In summary, Join-Path is an invaluable cmdlet for anyone working in PowerShell. It not only simplifies the process of path manipulation but also enhances script reliability and readability. By practicing and applying the concepts outlined here, you'll elevate your PowerShell scripting skills and optimize your workflows.
For further learning, consider exploring official documentation or engaging with the PowerShell community for best practices and new insights.