PowerShell UNC path allows you to access shared folders or files on a network using their Universal Naming Convention by specifying the path in the format `\\ServerName\ShareName`.
Here's a code snippet demonstrating how to list the contents of a shared folder using a UNC path:
Get-ChildItem '\\ServerName\ShareName'
Understanding UNC Path Syntax
A Universal Naming Convention (UNC) Path serves as a standard way to access shared resources on a network, eliminating the need for mapped drives. The syntax follows a straightforward structure:
\\ServerName\ShareName\Path\To\File
Each component plays a vital role:
- Server Name: This is the name of the server hosting the shared resource. It can be an IP address or a hostname.
- Share Name: This is the name of the shared resource. It denotes the directory on the server that users can access.
- Subdirectories and Files: These specify the hierarchy within the shared resource and point to specific files or folders.
For example, `\\MyServer\Documents\Report.pdf` directs you to a file named `Report.pdf` located in the `Documents` folder on `MyServer`.
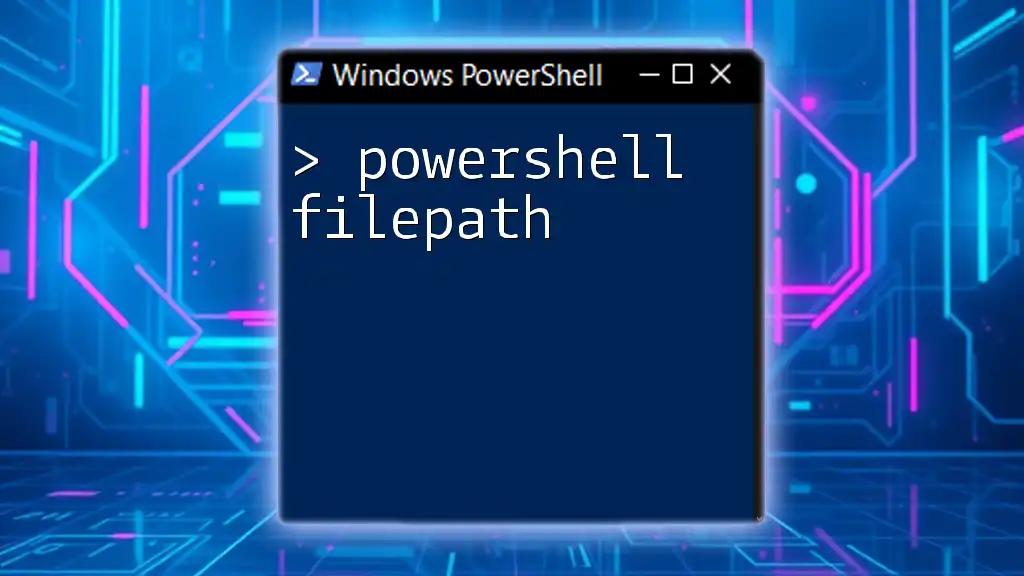
Accessing UNC Paths in PowerShell
Using PowerShell to interact with UNC paths enhances automation and streamlines administrative tasks. One of the most common ways to access these paths is by using `Get-ChildItem`, which lists the contents of a specified directory.
Basic Command Example:
Get-ChildItem \\ServerName\ShareName\
Upon executing this command, PowerShell will return a list of files and folders located in the shared directory. If accessing large datasets or directories, consider incorporating filters to refine your results or specifying the file type.
Another useful cmdlet is `Set-Location`, which allows users to change the current working directory to the specified UNC path.
Changing Current Directory:
Set-Location \\ServerName\ShareName\
By navigating directly to the UNC path, you can simplify subsequent commands without needing to repeatedly specify the full path.
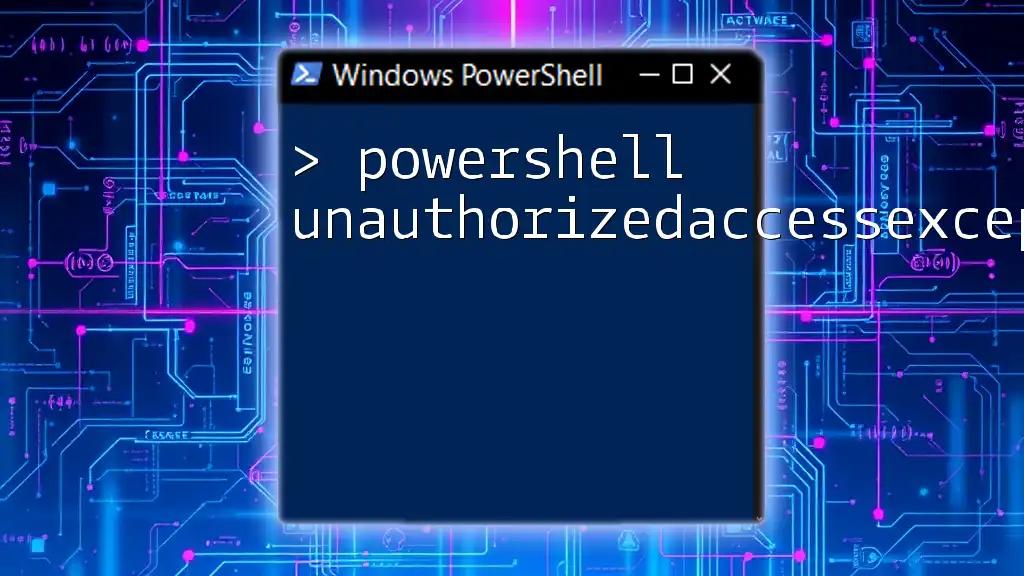
Working with UNC Paths: Common Commands
Copying Files to a UNC Path
PowerShell provides efficient methods for managing file transfers to UNC paths. The `Copy-Item` cmdlet is commonly used for this purpose.
Using Copy-Item:
Copy-Item -Path "C:\LocalFile.txt" -Destination "\\ServerName\ShareName\"
This command copies a file from the local machine to the designated network shared directory. This technique is beneficial for backing up local files or distributing files across the network.
Moving Files to a UNC Path
Similarly, `Move-Item` allows you to transfer files to a UNC path while removing them from their original location.
Using Move-Item:
Move-Item -Path "C:\LocalFile.txt" -Destination "\\ServerName\ShareName\"
This is especially useful for organizing files within network locations or clearing out local storage after ensuring files have been successfully transferred.
Deleting Files from a UNC Path
For file management, deleting unnecessary files can free up space and maintain organization. You can use the `Remove-Item` cmdlet to delete files from a UNC path.
Using Remove-Item:
Remove-Item "\\ServerName\ShareName\FileToDelete.txt"
Be cautious with this command; deleting files in a shared directory can impact multiple users. It’s advisable to double-check the path and file name before executing such operations.
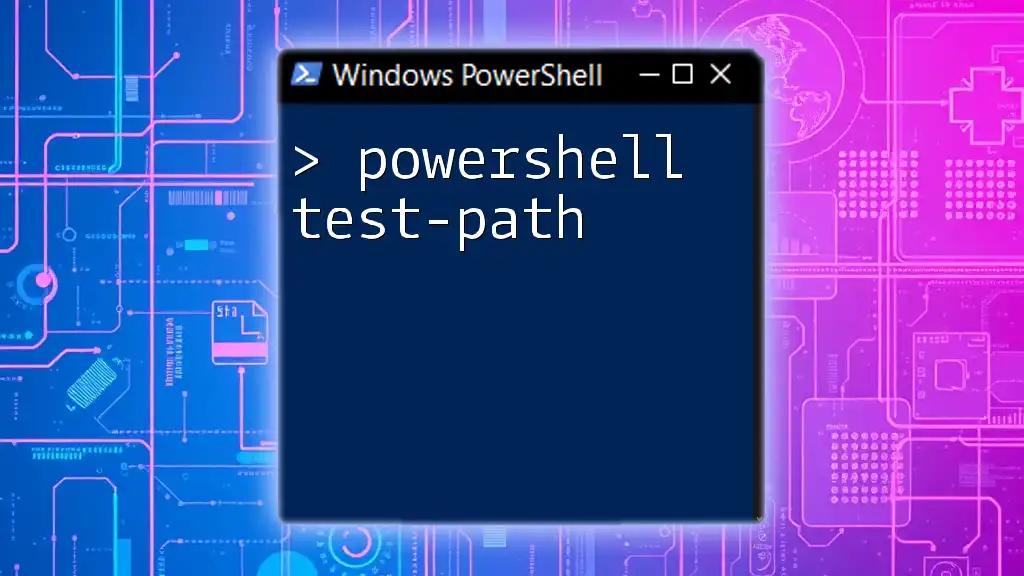
Best Practices for Using UNC Paths
Ensuring Network Availability
Before executing scripts or commands that interact with UNC paths, it’s crucial to verify the network connection. Utilizing `Test-Connection` can preemptively check if the server is accessible:
Test-Connection -ComputerName "ServerName" -Count 2
Using Credentials for Accessing UNC Paths
In many environments, accessing UNC paths may require proper credentials. PowerShell enables the management of credentials through the `New-PSDrive` cmdlet.
Example of Credential Management:
$credential = Get-Credential
New-PSDrive -Name "X" -PSProvider FileSystem -Root "\\ServerName\ShareName" -Credential $credential
This snippet prompts for a username and password before mapping the UNC path, ensuring secure access.
Error Handling in Scripts
To enhance the reliability of your scripts, consider implementing error handling using `try-catch` blocks. This allows you to gracefully handle unexpected issues during execution.
Implementing Error Handling:
try {
Get-ChildItem \\ServerName\ShareName\
} catch {
Write-Host "Error accessing UNC path: $_"
}
This technique captures and displays error messages, helping you diagnose potential issues more effectively.

Troubleshooting Common UNC Path Issues
While using UNC paths can be straightforward, several common issues may arise:
Permissions Problems
Access denied errors are frequent when users lack the necessary permissions to a shared resource. Ensure that the user account you’re using has adequate rights configured within the shared folder settings.
Network Issues
Inconsistent network connectivity can lead to timeouts or inaccessible shares. Use tools like `ping` or `Test-Connection` to check the server's availability.
Incorrect Path Syntax
Always review your path syntax when encountering problems. Occasionally, simple typographical errors can lead to frustration. Ensure that names are correctly spelled and that you include all necessary components, such as escape characters when required.
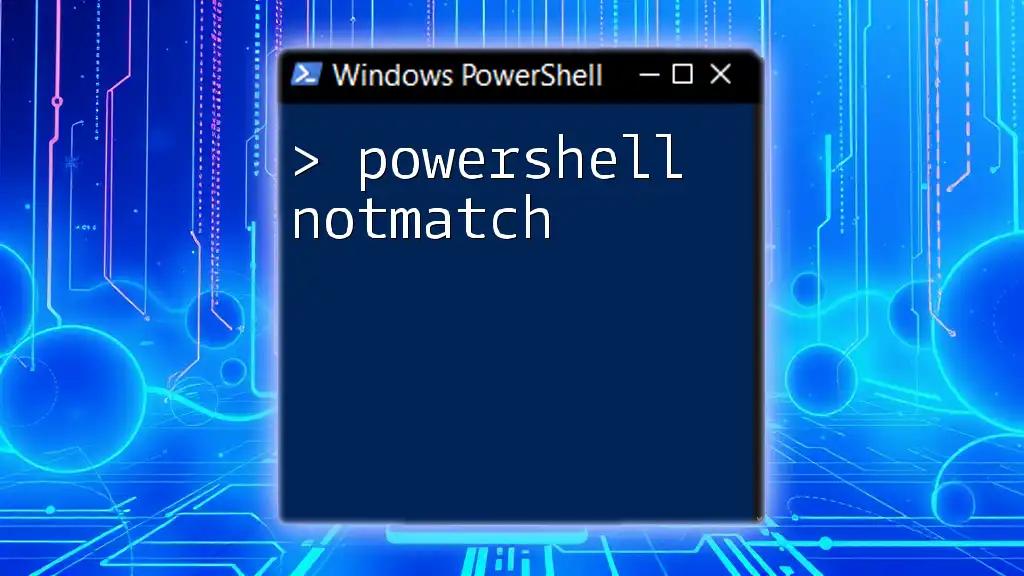
Real-World Applications of UNC Paths in PowerShell
Automating Backup Processes
PowerShell scripts can automate backup operations, streamlining data management in a networked environment. For example, creating a scheduled task that copies files from local systems to a UNC path can ensure regular backups without manual intervention.
Batch File Operations
The ability to perform batch operations is a powerful feature of PowerShell. By scripting multiple file operations, such as copying, moving, or even renaming, you can efficiently manage large volumes of data stored on network shares.
Monitoring File Changes on a UNC Path
Using the `FileSystemWatcher` class gives you real-time monitoring capabilities for directory changes. This can be crucial for audit logs or trigger-based operations.
Example Setup:
$watcher = New-Object System.IO.FileSystemWatcher
$watcher.Path = "\\ServerName\ShareName\"
By setting up an event handler, you can respond automatically to changes such as file additions or deletions.

Conclusion
Utilizing PowerShell with UNC paths provides excellent flexibility and power for file and directory management in network environments. The ability to automate, securely access, and manipulate files on shared resources streamlines workflows and enhances productivity. By following best practices and employing appropriate error handling, you can effectively manage network resources and optimize your PowerShell scripts.