The `Pause` command in PowerShell is used to halt the execution of a script, allowing the user to read information or take necessary actions before proceeding, and can be implemented with the following code snippet:
Write-Host "Press any key to continue..."
$null = $host.UI.RawUI.ReadKey("NoEcho,IncludeKeyDown")
Understanding the Need for Pausing in PowerShell Scripts
Why Pause in PowerShell?
In scripting, pauses serve a crucial role. They allow users to control the flow of execution, ensuring that the script behaves as expected in various scenarios. Imagine running a script that performs a critical task; a pause gives you a moment to double-check inputs or outputs before proceeding. This can be invaluable in environments where the slightest mistake can lead to undesirable outcomes.
Common Scenarios for Using PowerShell Pause
Pausing is particularly beneficial in numerous situations:
- User Prompts: When scripts require user interaction before carrying out potentially impactful actions, a pause can prompt the user to confirm their choice.
- Readability: If your script generates extensive output data, adding a pause can allow users to read and digest this information before continuing.
- Resource Management: Complex processes often consume significant resources. By pausing, you can ensure that a task completes before proceeding to a subsequent step, thereby preventing overwhelm.

How to Implement a Pause in PowerShell
Using the `Start-Sleep` Cmdlet
The most straightforward way to introduce a pause in your script is via the `Start-Sleep` cmdlet. This cmdlet halts the script's execution for a specified number of seconds.
Syntax:
Start-Sleep -Seconds <int>
For example, consider you want to pause your script for 10 seconds. You can implement it like this:
Write-Host "The script will now pause for 10 seconds."
Start-Sleep -Seconds 10
Write-Host "Continuing the script..."
This method is ideal for cases where you want the script to simply wait for a predefined period.
Using `Read-Host` for User Input
Another effective method for pausing a PowerShell script is using the `Read-Host` cmdlet. This cmdlet prompts the user for input, and the script will not continue until the user has provided input, effectively creating a pause that invites interaction.
Syntax:
$input = Read-Host "Press Enter to continue"
Here's how you might use it in a script:
Write-Host "Script execution paused. Press Enter to continue..."
Read-Host
Write-Host "Resuming script execution..."
This is particularly useful in interactive scripts where user confirmation is vital before proceeding.
Combining Commands for Efficient Pausing
Creating a seamless user experience often involves combining different methods to manage pauses effectively. This allows you to build a comprehensive flow in your script.
Example:
Write-Host "Preparing to execute a task in 5 seconds..."
Start-Sleep -Seconds 5
Read-Host "Press Enter to start the task"
In this instance, the script pauses for a brief period, then prompts the user to press Enter, allowing them to prepare mentally for the next action.

Best Practices for Pausing PowerShell Scripts
When to Use `Start-Sleep` vs. `Read-Host`
Choosing between `Start-Sleep` and `Read-Host` depends heavily on the desired interaction level. If your goal is purely to create a delay, `Start-Sleep` is your go-to. However, if you need a user prompt that requires a conscious action to continue, then `Read-Host` is preferable.
Pros and Cons:
- Start-Sleep: Quick and effective for timed delays, but lacks interaction.
- Read-Host: Encourages user engagement but requires user action to proceed.
Ensuring User-Friendly Pausing
Effective pauses should come with clear messaging. Communicate the reason behind each pause, helping users understand what is happening in the script and why waiting is crucial. For example, instead of just a pause, tell users what action is pending or what background process is occurring.
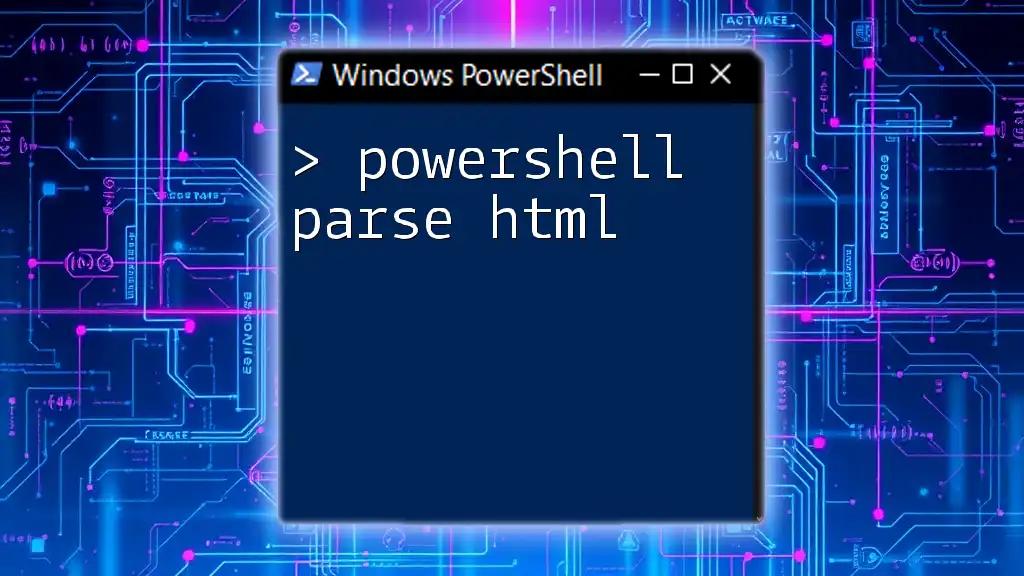
Advanced Pausing Techniques
Using Timers and Conditional Pauses
Sometimes, you might want to pause only when particular conditions are met. This allows for more intelligent script behavior.
Example:
$condition = $true
if ($condition) {
Write-Host "Condition met, pausing for 3 seconds..."
Start-Sleep -Seconds 3
}
In this situation, the pause only occurs if the condition evaluates to true, preventing unnecessary delays when they are not warranted.
Pausing in Loops
When working with loops, implementing pauses becomes particularly valuable. It allows for controlled execution within iterative processes, making them more manageable.
Example:
foreach ($item in $items) {
Write-Host "Processing $item..."
Start-Sleep -Seconds 2
}
In this loop, after each item is processed, the script pauses for 2 seconds, allowing time for observation or further action if needed.
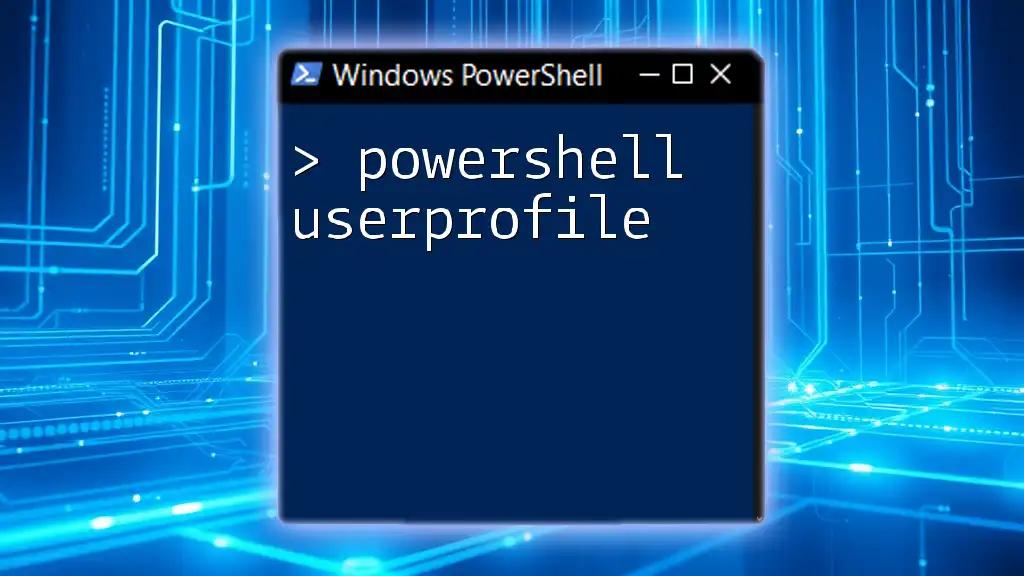
Troubleshooting Common Issues with PowerShell Pausing
Identifying Common Errors
When implementing pauses, scriptwriters often encounter issues such as excessive delays, unintentional infinite pauses, or user confusion due to poorly phrased prompts. It’s crucial to test scripts with various scenarios to ensure that pauses do not disrupt the intended flow.
Real-world Scenarios of Misuse
Consider a scenario where a script depends on user input but neglects to provide a clear instruction. If users aren’t informed that they need to act to continue, they may become confused or frustrated, halting productivity. Learning from such mistakes ensures better implementation of pauses in future scripts.
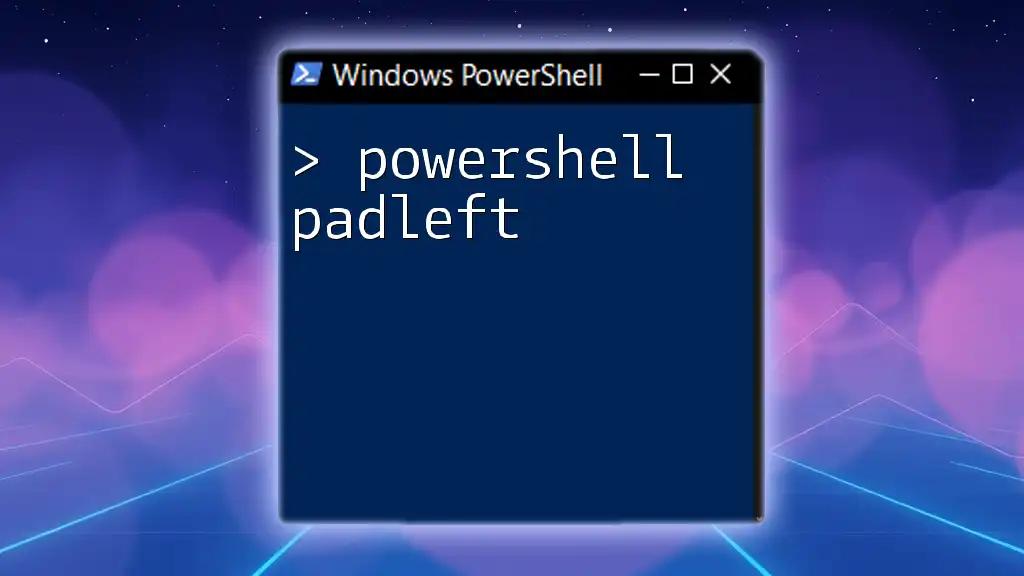
Conclusion
The ability to pause a PowerShell script is a powerful tool in a developer’s toolkit. By strategically using `Start-Sleep` and `Read-Host`, you can enhance user interaction, maintain control over script execution, and improve the overall functionality of your PowerShell scripts.
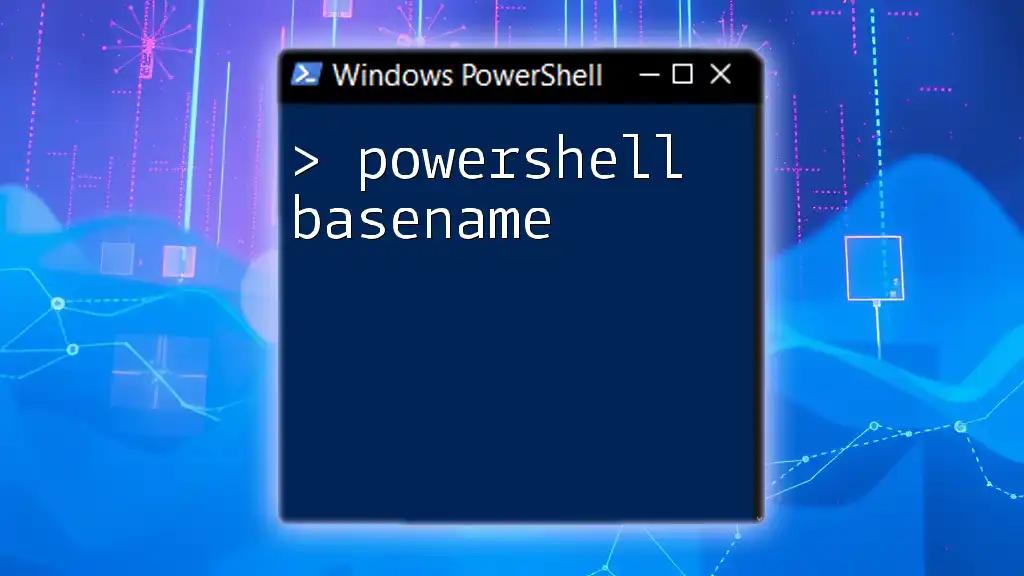
Additional Resources
For those eager to further their knowledge, official PowerShell documentation is a fantastic resource. Additionally, exploring blogs and tutorials may also provide valuable insights and advanced techniques.

Call to Action
I encourage you to start implementing pauses in your PowerShell scripts today! Take a moment to experiment with different approaches and discover how they can streamline your scripting process. Don't forget to subscribe or follow for more tips and resources on mastering PowerShell.