PowerShell can effectively parse HTML content using the `Invoke-WebRequest` cmdlet, allowing you to extract specific elements from web pages with ease.
Here's a simple code snippet demonstrating how to parse HTML:
$response = Invoke-WebRequest -Uri 'https://example.com'
$html = $response.Content
$parsedHtml = $html | Select-Xml -XPath '//h1' | ForEach-Object { $_.Node.InnerText }
$parsedHtml
This command fetches the HTML from the specified URL and extracts text from all `<h1>` elements.
Understanding HTML Structure
HTML, or HyperText Markup Language, is the standard markup language used to create web pages. It consists of various elements, tags, and attributes that define how content appears on the web. Understanding these components is crucial for effective HTML parsing.
Basic components of HTML
- Elements: The building blocks of HTML, which can be tags, text, or other elements.
- Tags: These define elements, such as `<div>`, `<p>`, or `<h2>`.
- Attributes: Modifiers that specify additional information about an element, for instance, `<a href="http://example.com">Link</a>`.
What is a DOM (Document Object Model)?
The DOM is a representation of the document structure, allowing programming languages, like PowerShell, to interact with and manipulate the HTML as an object. By understanding the DOM, you can effectively navigate through the elements of an HTML document.
Why parsing HTML is beneficial in various applications
Parsing HTML enables automation, data extraction, and even web scraping. Whether collecting data for reporting, conducting market research, or automating repetitive tasks, PowerShell's ability to parse HTML is an invaluable skill.

Getting Started with PowerShell HTML Parsing
To effectively parse HTML, you need to leverage specific PowerShell modules that help in retrieving and manipulating web content. The most common ones are:
- `Invoke-WebRequest`: Used to fetch the HTML content from web pages.
- `Select-Xml`: Allows you to utilize XPath to navigate through XML-like structures within the HTML.
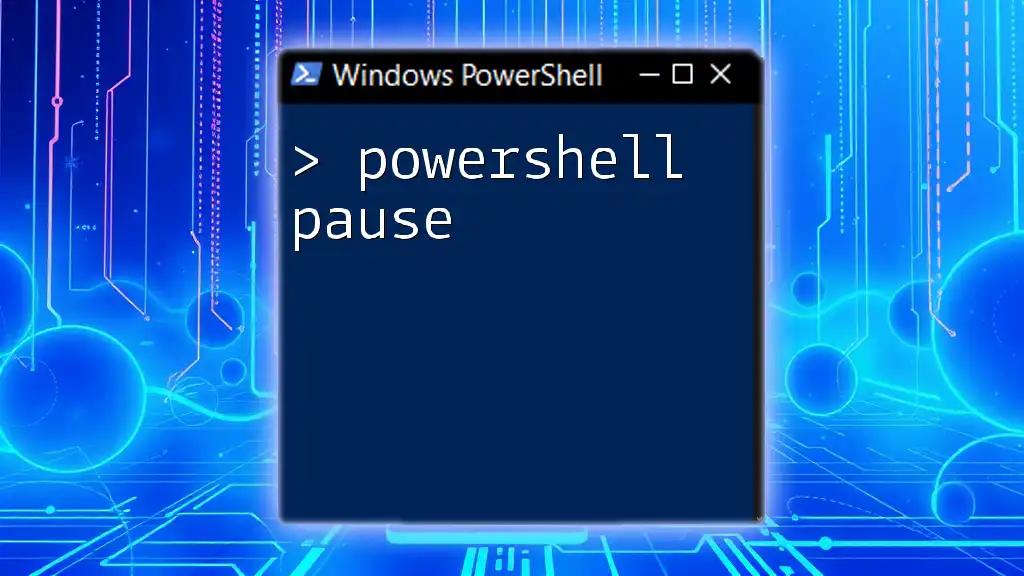
PowerShell Parsing HTML: The Basics
Fetching HTML content using PowerShell
To begin, you'll first need to retrieve the HTML content of a web page. The following example demonstrates how to use `Invoke-WebRequest` to achieve this:
$response = Invoke-WebRequest -Uri "http://example.com"
$htmlContent = $response.Content
In this snippet, we are requesting HTML content from "http://example.com" and storing it in the `$htmlContent` variable.
Converting HTML to an object model for easier parsing
Once you have the HTML content, you should convert it to an object model that makes it easier to manipulate. This can typically be done with the `.ParsedHtml` property:
$html = $response.ParsedHtml
This line casts the raw HTML into a parsed format allowing you to interact with it more intuitively.
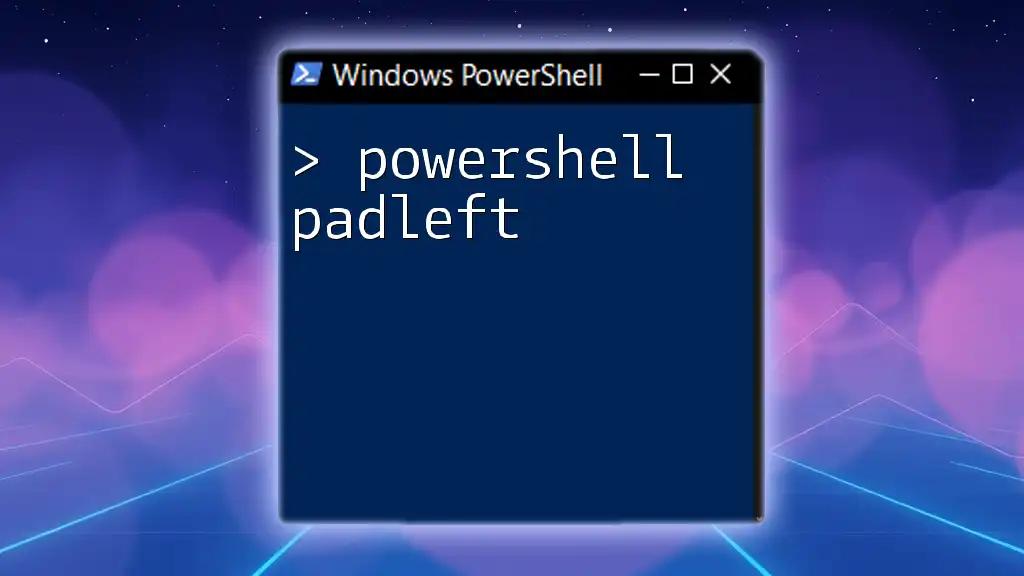
Working with PowerShell HTML Parser
Using the HTML parser to extract information
After parsing, you can navigate through the DOM to extract information. Here’s an example of how to retrieve all `<h2>` elements from the HTML:
$elements = $html.getElementsByTagName("h2")
foreach ($element in $elements) {
$element.innerText
}
In this code, we are selecting all `<h2>` tags and iterating through them to extract their inner text content.
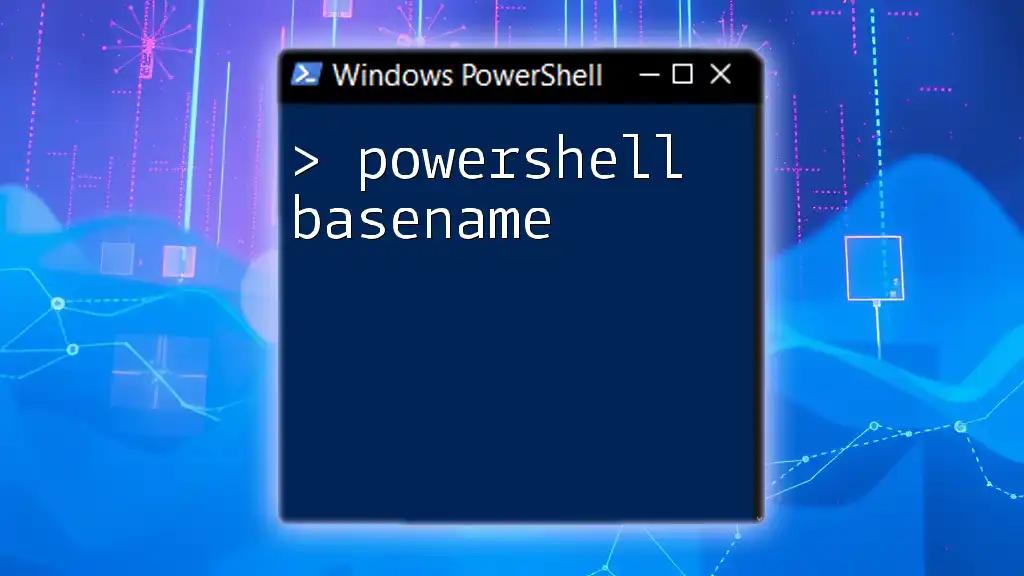
Advanced Techniques for PowerShell Parsing HTML
Filtering and selecting specific data
When parsing HTML, it's often essential to filter elements based on class names or IDs. Here’s how you can access elements by class name:
$elements = $html.getElementsByClassName("class-name")
This line retrieves all elements with the specified class, providing a way to focus your results on only the relevant portions of the HTML.
Using `Select-Xml` for XPath queries
For more complex queries, PowerShell allows you to use XPath with the `Select-Xml` cmdlet. XPath provides a syntax to navigate through elements in the XML-like structure of parsed HTML. Here’s an example:
$xmlDoc = [xml]$html
$result = $xmlDoc.SelectNodes("//div[@class='target-class']")
In the code above, we convert the parsed HTML into an XML format and then use XPath to select all `<div>` elements with a specific class. This method greatly enhances your ability to target distinct pieces of data.
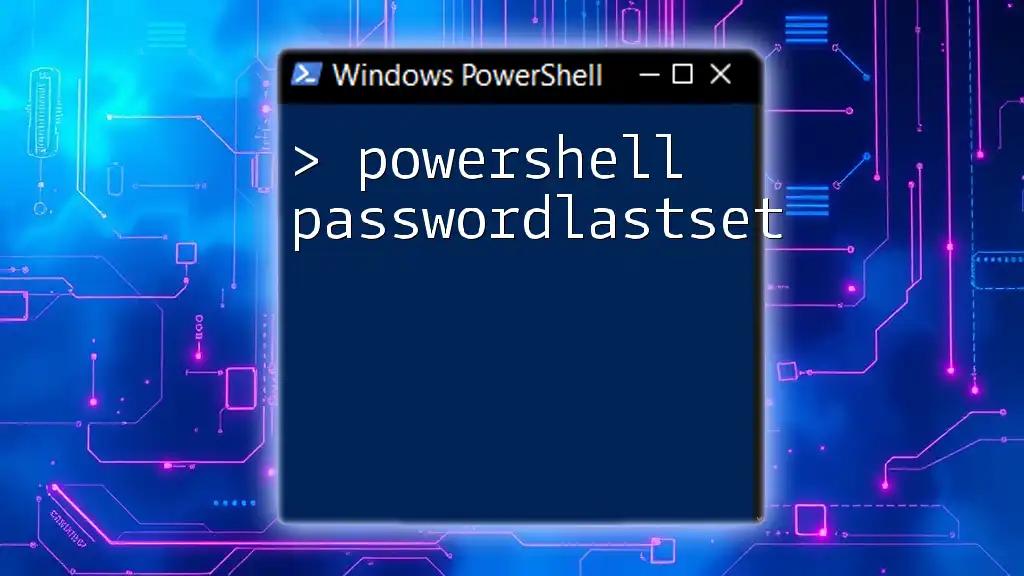
Common Use Cases for PowerShell HTML Parsing
PowerShell HTML parsing can be applied in various contexts:
- Web scraping for data analysis: Extracting data from websites for further analysis.
- Collecting and aggregating content from multiple sources: Gathering information from several pages into a single report.
- Automated report generation: Creating regular reports from online data without manual inputs.
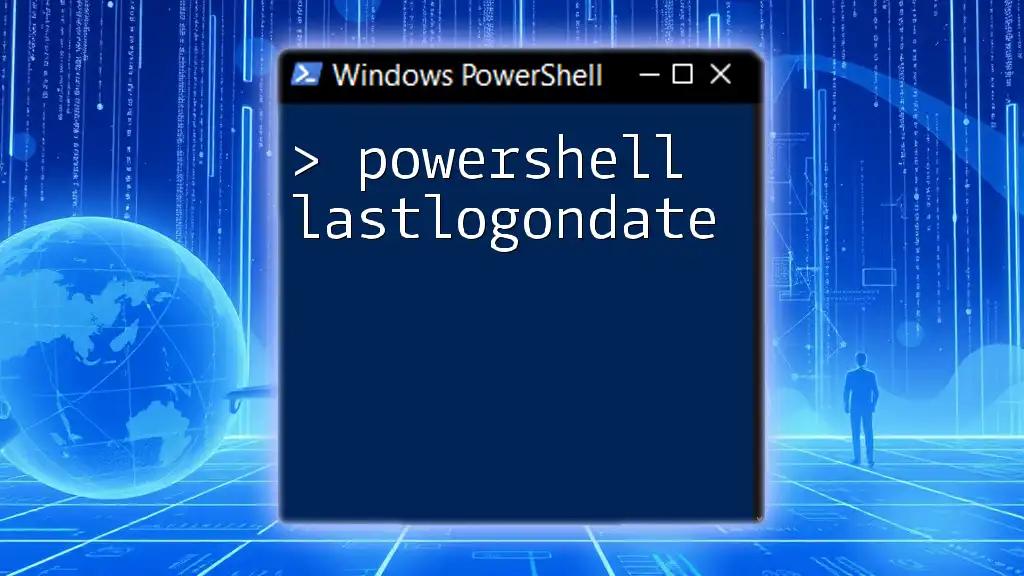
Handling Dynamic Content
One limitation of static HTML parsing is that it may not work well with dynamically generated content, often created using JavaScript. In such cases, consider using APIs if they are available. For scenarios requiring JavaScript execution, PowerShell can leverage Selenium WebDriver to obtain dynamic content.
Example of using Selenium WebDriver
While it goes beyond simple HTML parsing, employing Selenium allows PowerShell to automate browser interactions:
# Example setup for Selenium in PowerShell (ensure you have the appropriate drivers)
Add-Type -Path "C:\path\to\Selenium.WebDriver.dll"
$driver = New-Object OpenQA.Selenium.Chrome.ChromeDriver
$driver.Navigate().GoToUrl("http://example.com")
$html = $driver.PageSource
$driver.Quit()
This snippet launches a browser, navigates to the URL, collects the page source, and then terminates the browser session.
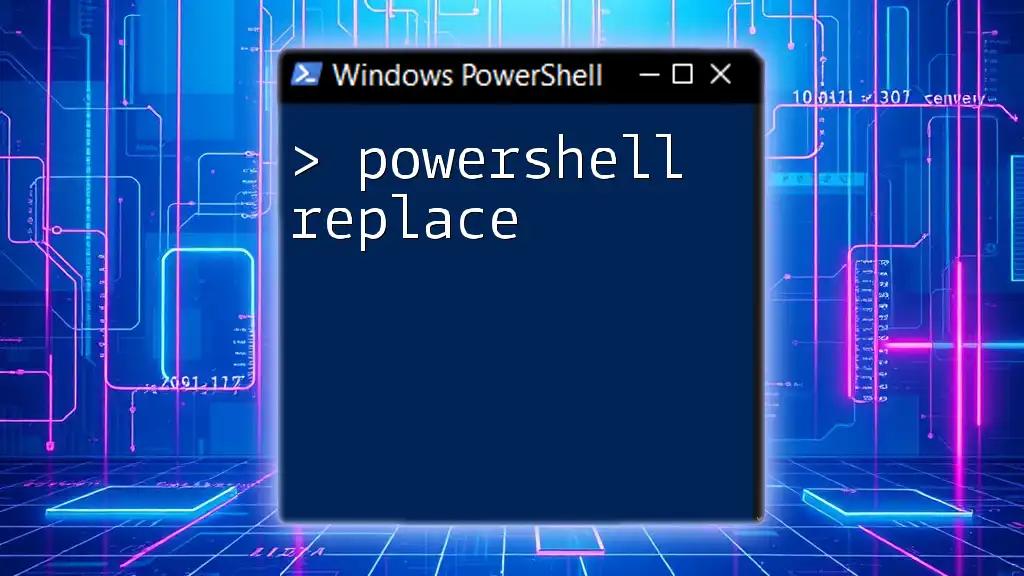
Best Practices for PowerShell HTML Parsing
When engaging in HTML parsing with PowerShell, keep in mind the following best practices:
- Handle exceptions and errors: Always account for potential errors, such as connection issues or missing elements.
- Consider performance with large HTML files: For extensive documents, optimize your code to avoid slowdowns.
- Ensure compliance with web scraping laws: Always check a website's `robots.txt` file and abide by legal and ethical guidelines.
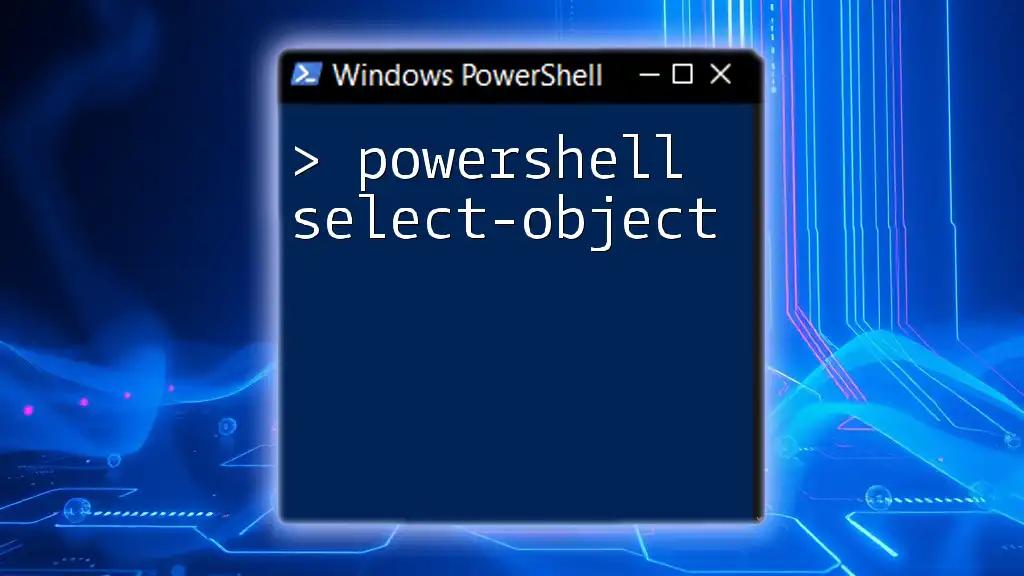
Conclusion
Parsing HTML with PowerShell opens a world of automation and data extraction opportunities. By mastering the techniques outlined in this guide, you can significantly enhance your ability to manipulate web content effectively. Whether for personal use or professional applications, the practice of PowerShell parsing can ultimately save you time and resources.
Explore and experiment with these techniques to become proficient in utilizing PowerShell's capabilities for HTML parsing! For further learning, consider diving into additional resources and documentation that focus on PowerShell and web automation.