Sure! Here's a concise explanation for your post along with a PowerShell code snippet:
In this PowerShell post, you'll learn how to display a simple message using the `Write-Host` cmdlet.
Write-Host 'Hello, World!'
Introduction to PowerShell
What is PowerShell?
PowerShell is a powerful scripting language and command-line shell designed primarily for system administrators. It provides a versatile environment for automating tasks, managing system configurations, and handling complex workflows. With its rich feature set, including support for cmdlets, object-oriented programming, and pipelines, PowerShell simplifies the management of Windows environments.
Why Use PowerShell?
Using PowerShell offers numerous advantages:
- Automation: Automate repetitive tasks, reducing time and potential errors.
- Integration: Seamlessly integrates with other Microsoft products, cloud services, and third-party applications.
- Flexibility: Supports both interactive use and scripting, allowing for quick command execution as well as more complex script development.
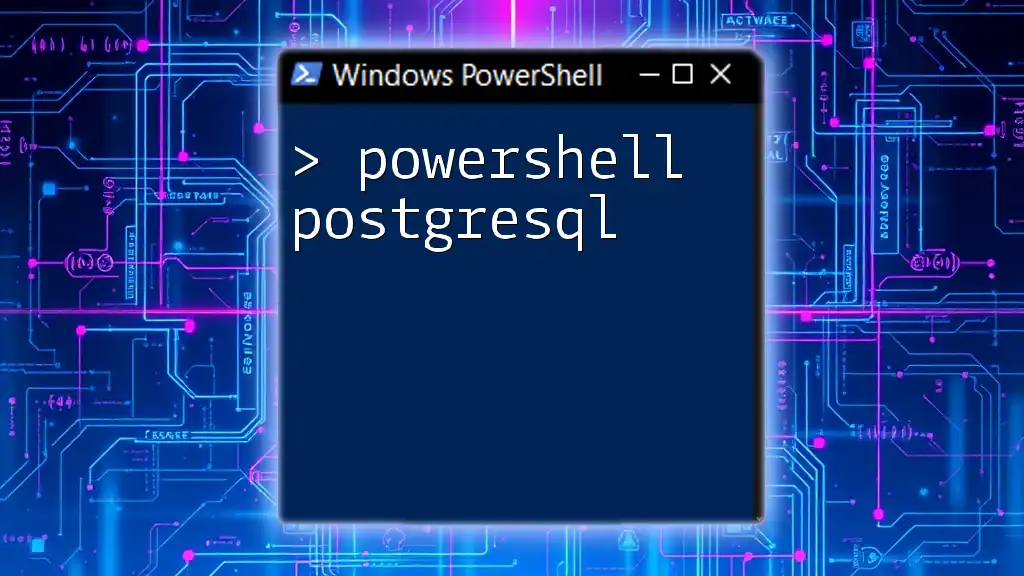
Setting Up PowerShell
Installing PowerShell
Before diving into using PowerShell, you need to ensure it's installed on your machine. Most Windows versions come with PowerShell pre-installed. For those working beyond Windows (e.g., macOS or Linux), you can download PowerShell from the official [GitHub repository](https://github.com/PowerShell/PowerShell). Follow the installation instructions specific to your operating system for a smooth setup process.
Launching PowerShell
Once installed, you can launch PowerShell in several ways:
- Search in the Start Menu: Type "PowerShell" and select either "Windows PowerShell" or "Windows PowerShell ISE".
- Run Dialog: Press `Win + R`, type `powershell`, and hit enter.
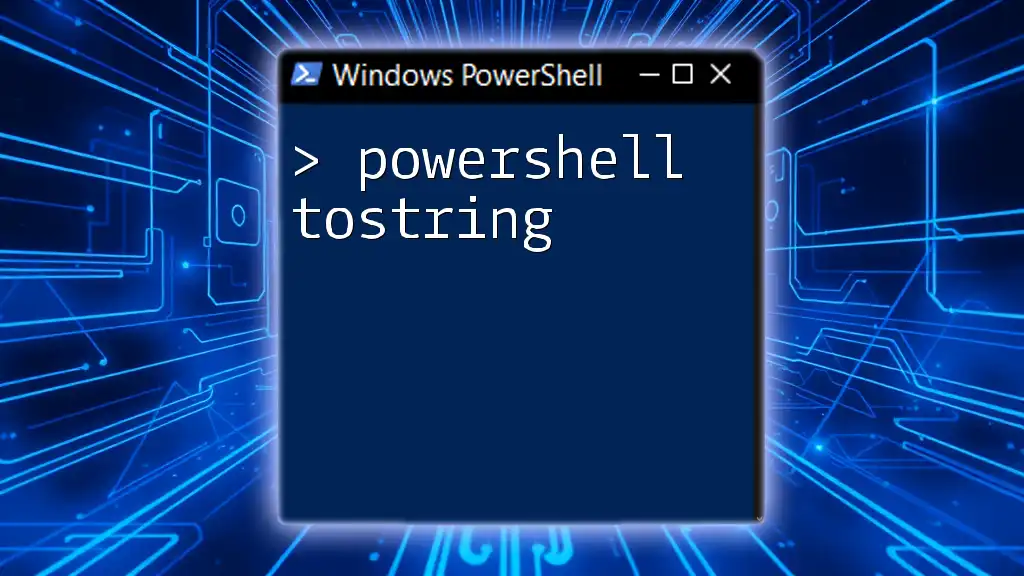
Understanding PowerShell Syntax
Basic Command Structure
Understanding the command structure is crucial for effective use of PowerShell. Each command, called a cmdlet, has a specific function and often consists of a verb-noun pair, followed by parameters. For example:
Get-Process | Where-Object { $_.CPU -gt 100 }
In this command:
- Get-Process retrieves a list of currently running processes.
- Where-Object filters the results based on specified criteria.
- `$_` represents the current object in the pipeline, in this case, each process.
Variables and Data Types
PowerShell allows you to store data in variables using the `$` symbol. Understanding the different data types is essential for effective scripting. For instance, you can define a string variable as follows:
$greeting = "Hello, World!"
This string can now be manipulated or displayed throughout your script. PowerShell supports various data types like integers, arrays, and hashes, each serving specific purposes in your automation tasks.
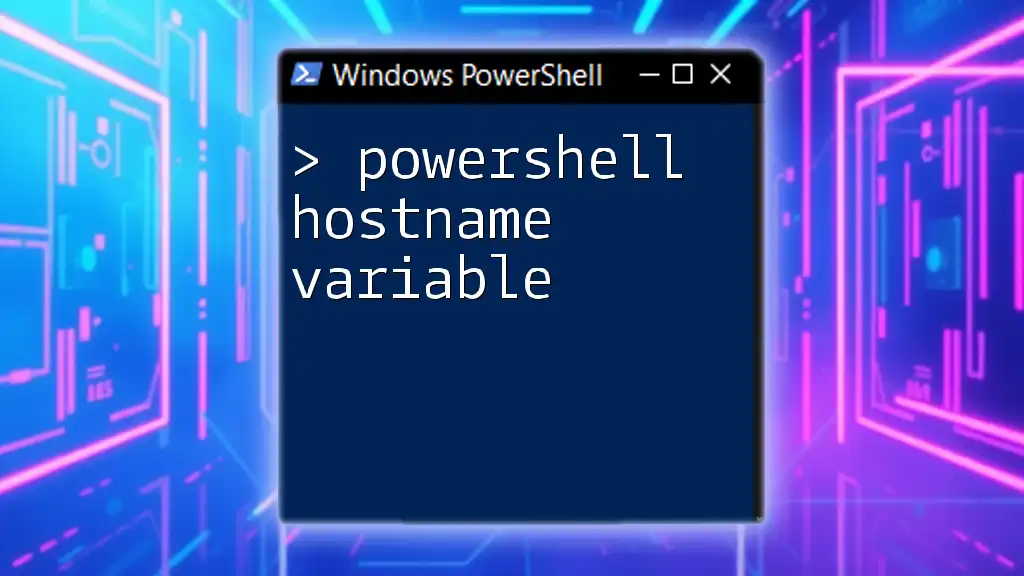
Common PowerShell Commands
Exploring Cmdlets
Cmdlets are the building blocks of PowerShell. Here are a few commonly used ones:
- Get-Help: This cmdlet provides detailed information about other cmdlets.
Get-Help Get-Service
- Get-Service: Lists the services running on your system.
These commands facilitate easy exploration and learning, enabling users to familiarize themselves with PowerShell’s capabilities.
Working with Files and Directories
PowerShell excels in file management. You can navigate and manipulate file systems effortlessly. For example, to display all items in a specified directory:
Get-ChildItem C:\Path\To\Directory
To remove a specific file, use the `Remove-Item` cmdlet:
Remove-Item C:\Path\To\File.txt
These commands streamline file management tasks, enhancing productivity.
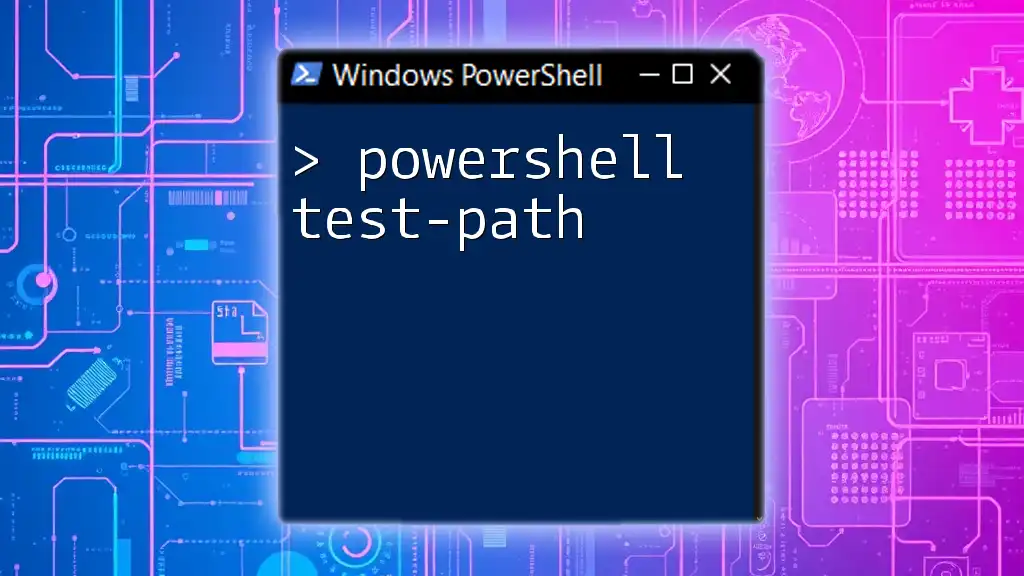
Advanced PowerShell Features
Working with Objects
One of PowerShell’s strengths is its ability to handle objects instead of plain text. This allows for more complex operations. For example, you can retrieve process details and select specific properties:
$processes = Get-Process
$processes | Select-Object Name, CPU
This returns a list of process names along with their CPU usage, making it easier to analyze resource consumption.
Creating Simple Functions
Functions allow for code reuse and better organization in your scripts. Defining a function in PowerShell is straightforward. Here’s an example that calculates the square of a number:
function Get-Square {
param ($number)
return $number * $number
}
You can call this function and pass any number to receive its square, simplifying tasks that require repeated calculations.
Error Handling and Debugging
Handling errors effectively is crucial when scripting. PowerShell provides robust error handling features. Using `Try`, `Catch`, and `Finally`, you can manage exceptions gracefully:
Try {
Get-Item "C:\NonExistentFile.txt"
} Catch {
Write-Host "File not found!"
}
This structure enhances the stability of your scripts by allowing you to respond to unexpected situations.
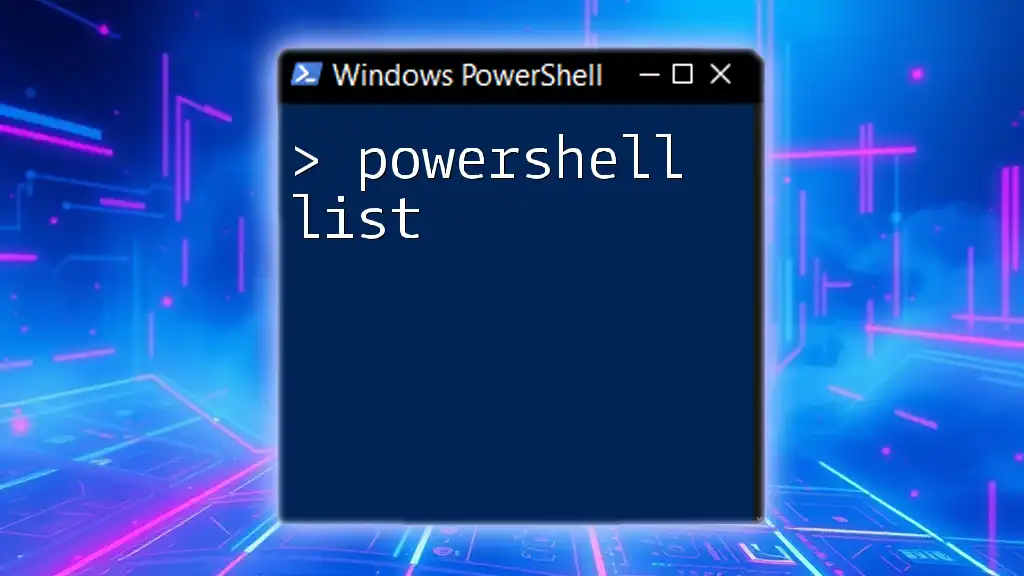
Best Practices for PowerShell Scripting
Writing Readable Scripts
Clarity is key in scripting. Use comments to explain complex logic and maintain consistent formatting. This makes it easier for both you and others to understand your scripts in the future.
Using Modules
PowerShell modules expand its functionality. You can find existing modules or create your own to encapsulate useful functions. To import a module:
Import-Module ModuleName
Exploring the PowerShell Gallery will provide you with a wealth of pre-built modules that can save you considerable time.
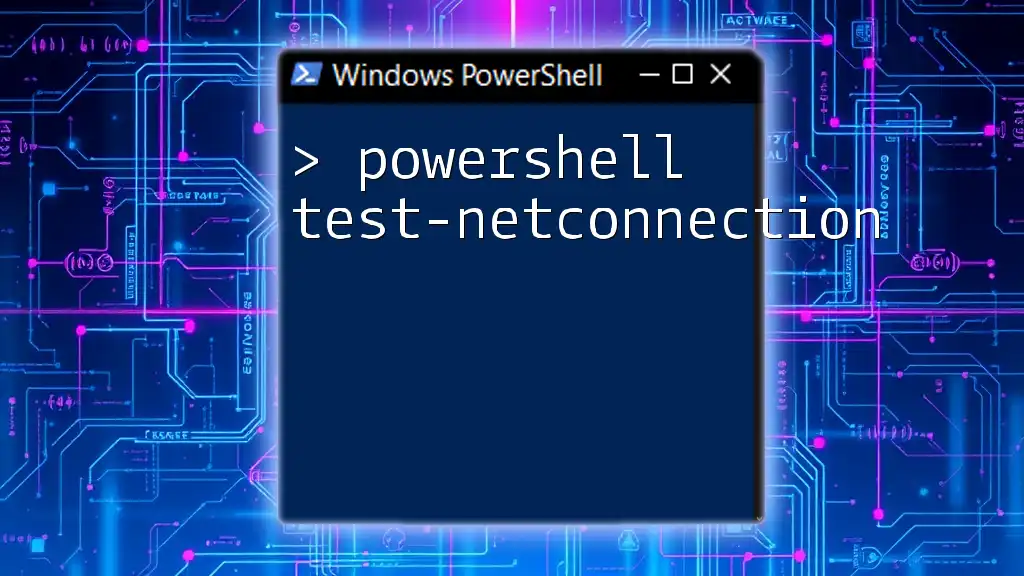
Conclusion
Recap of Key Concepts
In summary, mastering PowerShell enhances your ability to manage systems efficiently and automate complex tasks. This guide offers foundational knowledge, from understanding cmdlets to implementing error handling techniques.
Encouragement to Explore More
Embrace the vast capabilities of PowerShell. Explore additional resources—such as documentation, online communities, and tutorials—to expand your skills and become proficient in leveraging PowerShell’s potential.