Dot sourcing in PowerShell allows you to run scripts within the current session, enabling access to functions and variables defined in that script without creating a new scope.
Here’s how you can use dot sourcing in PowerShell:
. C:\Path\To\YourScript.ps1
Introduction to PowerShell Dot Sourcing
What is Dot Sourcing?
Dot sourcing is a powerful feature in PowerShell that allows you to run a script within the current scope of your session. By using dot sourcing, any functions, variables, or aliases defined in the script remain accessible after the script completes, unlike when you execute a script normally.
Benefits of Using Dot Sourcing
Dot sourcing provides several advantages, making it a vital tool for PowerShell users:
- Reusability of Scripts: Once a script is dot-sourced, you can use its functions or variables throughout your session without needing to redefine them.
- Simplifying Complex Scripts: You can break your scripts into smaller modules, improving organization and readability.
- Scope Management: Dot sourcing allows better control over variable scope, helping avoid conflicts and unintended overwrites.
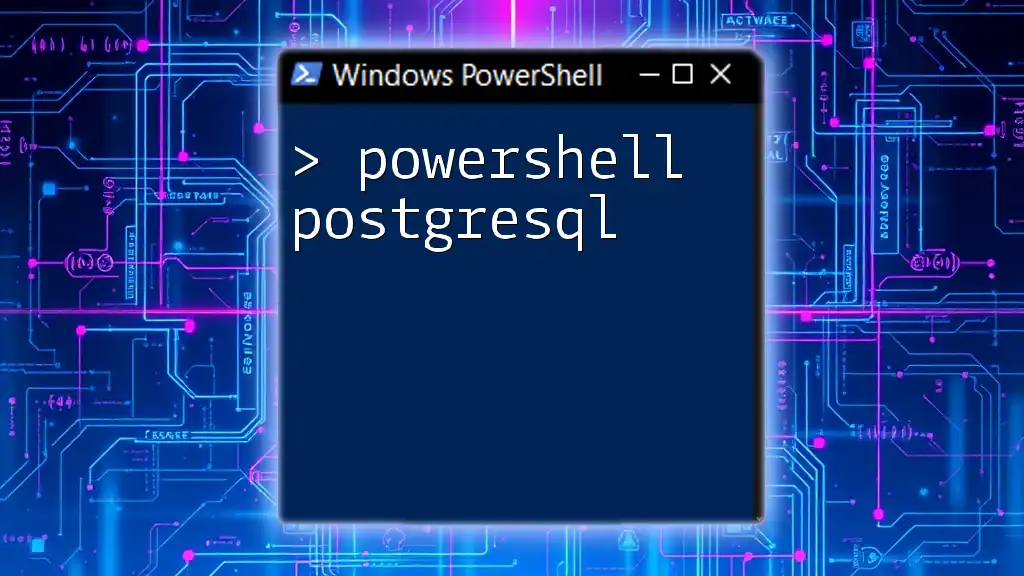
Understanding the Basics of PowerShell Dot Sourcing
Definition of Dot Sourcing
To dot source a script, you follow this syntax: `. <scriptname>`, where the dot (.) represents the dot sourcing operation. When you run this command, PowerShell executes the script in the context of the current scope, keeping its functions and variables available.
Key Concepts Related to Dot Sourcing
-
Execution Context: Dot sourcing executes the script in the current scope, affecting both local and global variables. If you simply run a script, its elements are confined to their internal scope unless specifically designed otherwise.
-
Script vs. Command: Use dot sourcing when you want to retain the script’s defined elements in your current session. If you simply execute a script file, the variables and functions declared within that script won't be accessible afterward.
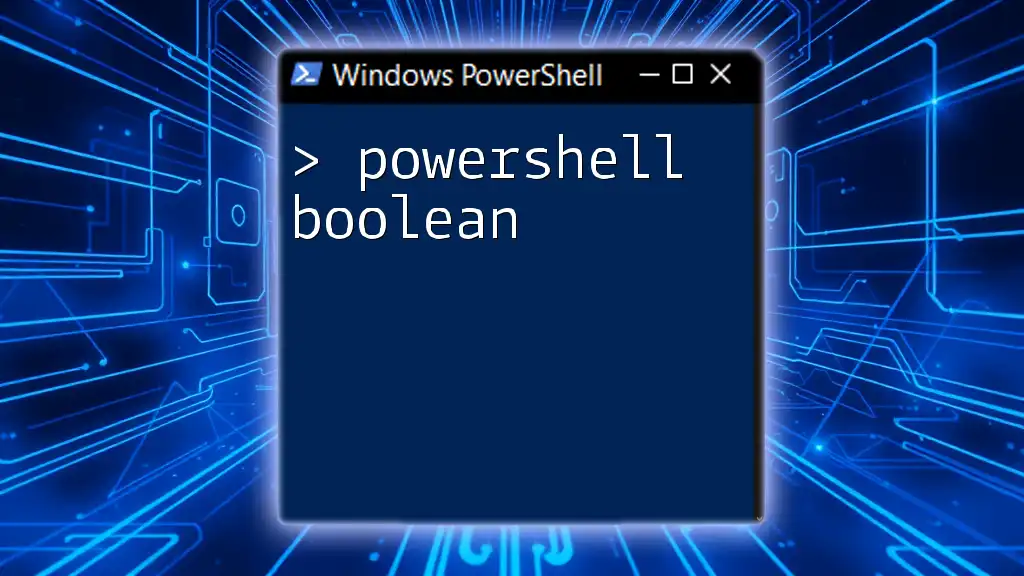
How to Use Dot Sourcing in PowerShell
Basic Syntax and Structure
The basic syntax for dot sourcing is straightforward. Suppose you have a script named `MyScript.ps1` located in the current directory. To dot source it, you would run:
. .\MyScript.ps1
This command loads all of the content in `MyScript.ps1` into your current session.
Invoking Functions and Variables
You can easily define functions within a script and call them after dot sourcing. For example, consider the following code:
function SayHello {
"Hello, world!"
}
If this function is defined in `MyFunctions.ps1`, you can dot source the script and call the function:
. .\MyFunctions.ps1
SayHello # This will output: Hello, world!
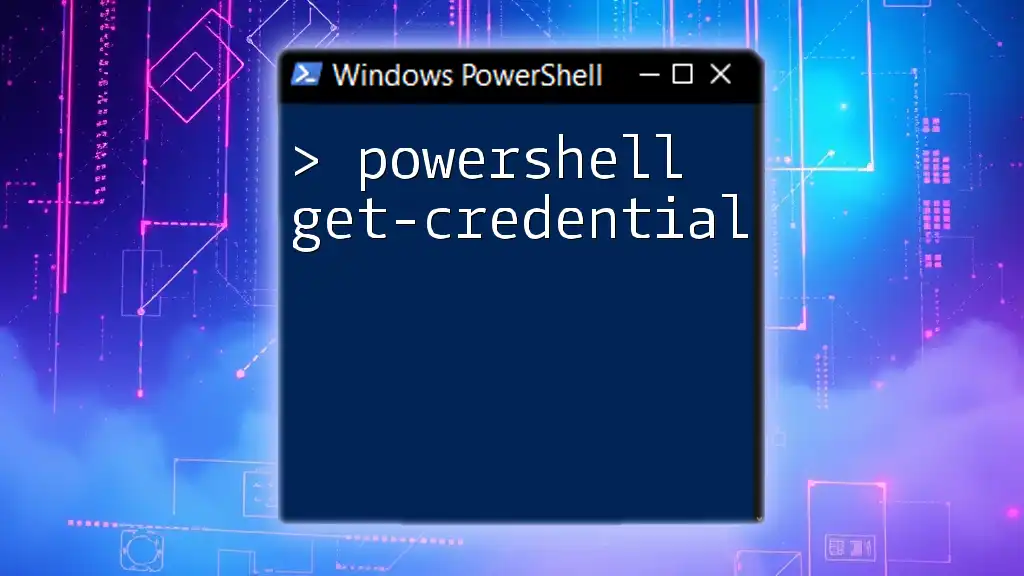
Common Use Cases for Dot Sourcing
Shared Scripts Across Different Sessions
When you have a configuration script that sets common variables for multiple scripts or sessions, using dot sourcing is ideal. For example, if `MyConfig.ps1` contains variable definitions:
$global:ConfigPath = "C:\MyConfig"
You can load this configuration once and easily access `ConfigPath` across different scripts.
Using Libraries of Functions
Dot sourcing is also useful for organizing reusable functions in a library script. Define multiple functions in a single script and load them all at once:
# MyLibrary.ps1
function Get-DateString {
return (Get-Date).ToString("yyyy-MM-dd HH:mm:ss")
}
function Log-Message {
param([string]$Message)
"$($dateString) - $Message"
}
After dot sourcing `MyLibrary.ps1`, you can use both functions seamlessly.
Managing Scope in Large Scripts
When writing large scripts, scope management becomes crucial. Dot sourcing allows you to control variable visibility and prevent errors from conflicting names. By keeping shared variables in a dedicated script and dot sourcing them, you can minimize scope collisions.
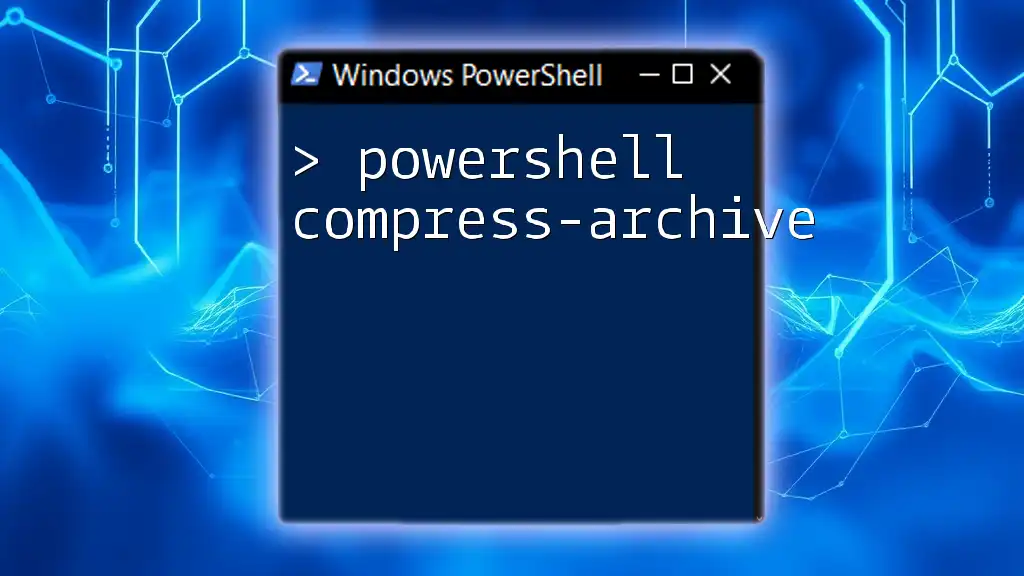
Advanced Dot Sourcing Techniques
Dynamic Script Loading
You can enhance flexibility by dynamically loading scripts using variables. Here’s an example:
$scriptPath = ".\MyDynamicScript.ps1"
. $scriptPath
This technique is particularly beneficial when constructing scripts that have varying behaviors based on input or conditions.
Using Dot Sourcing in Modules
In PowerShell modules, dot sourcing plays a vital role in structuring code. By organizing functions and variables within a module and dot sourcing them, you ensure efficient code reusability. Modules leverage dot sourcing behind the scenes to make functions available to users once imported.
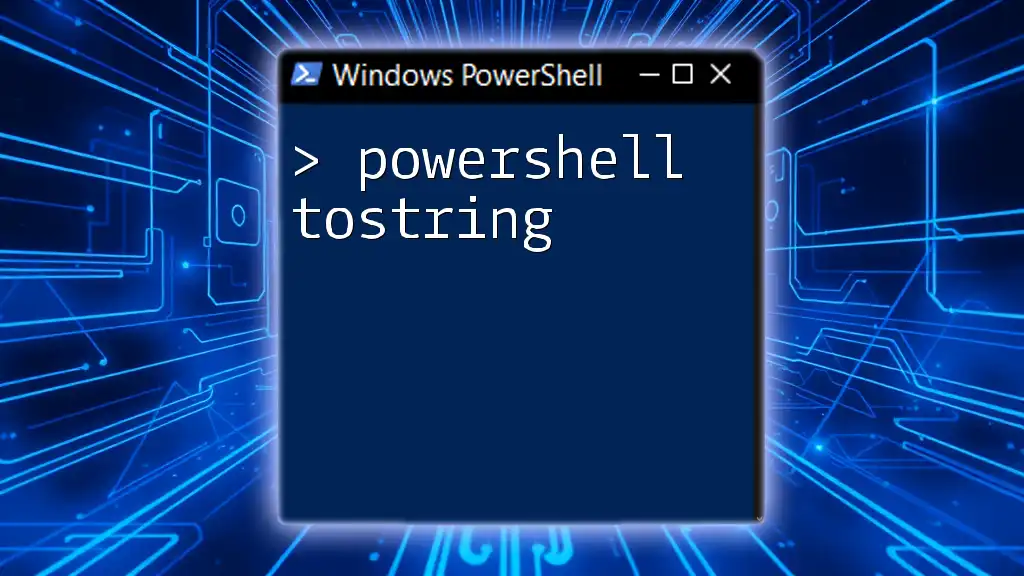
Troubleshooting Common Issues with Dot Sourcing
Common Errors
One of the most frequent errors encountered in dot sourcing is the file not found error. This occurs if the script's path is incorrect or if the script isn't located in the directory specified. Always double-check your paths when facing this issue.
Variable Scope Conflicts
When multiple scripts contain variables with the same name, dot sourcing can lead to unexpected results. To avoid this, adopt consistent naming conventions and scope your variables appropriately.
Debugging Dot Sourced Scripts
To debug dot-sourced scripts effectively, you can use the `-Verbose` or `-Debug` flags while running your scripts, which provide additional output helpful for understanding script behavior.
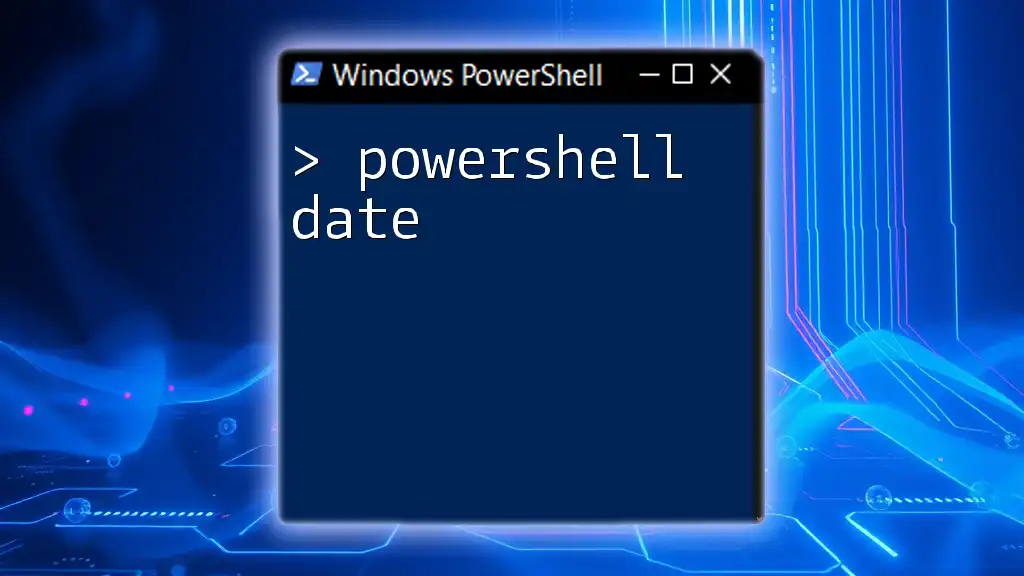
Best Practices for Dot Sourcing in PowerShell
Organizing Your Scripts
Keep your scripts consistently organized. Place related functions and variables together in specific script files, aiding maintainability and readability.
Documenting Your Functions and Scripts
Incorporate comments and documentation within your scripts. This not only helps you but also assists others who may work with your code in the future.
Limiting the Use of Global Variables
Global variables can lead to conflicts and unpredictable behavior. Use them sparingly and prefer local variables when possible to maintain clean and manageable code.
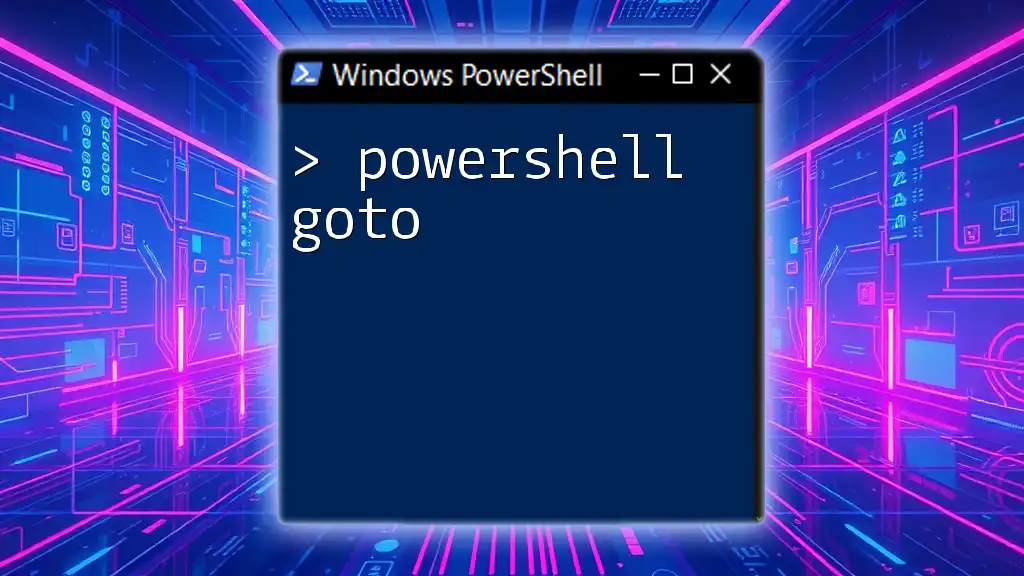
Conclusion
Incorporating dot sourcing into your PowerShell practices is essential for enhancing script efficiency and clarity. With its ability to promote reusability and scope management, dot sourcing empowers users to write cleaner, more organized scripts. Don’t hesitate to experiment with dot sourcing, as it's a valuable addition to your PowerShell toolkit.
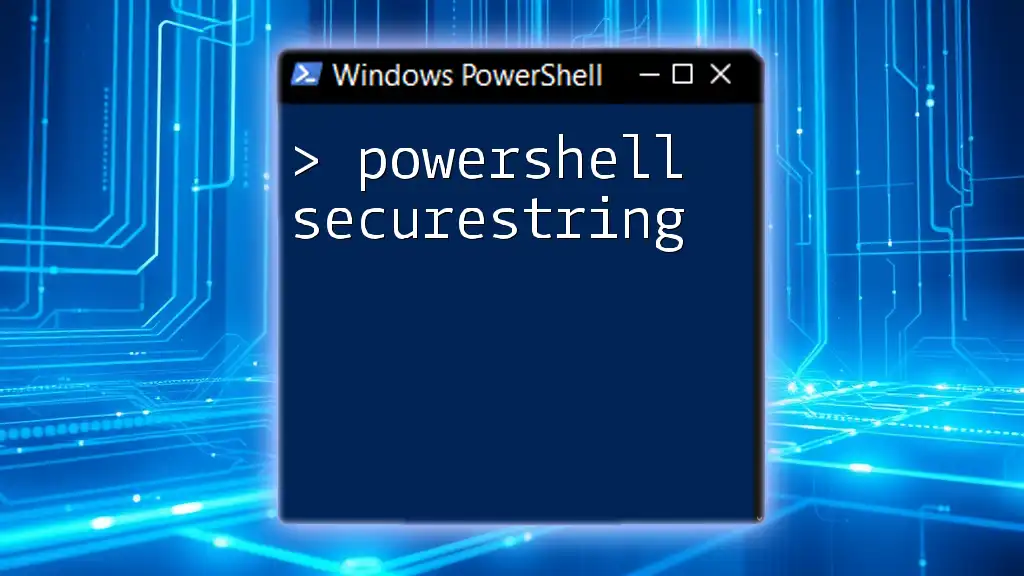
Additional Resources
As you continue your journey with PowerShell, consider exploring additional resources such as online documentation, tutorials, and PowerShell community forums. Engaging with others can enrich your knowledge and provide insights into best practices.