PowerShell runspaces enable you to run commands concurrently, allowing for efficient use of resources and improved performance in script execution.
$runspace = [runspacefactory]::CreateRunspace()
$runspace.Open()
$script = { Write-Host 'Hello from a runspace!' }
$pipeline = $runspace.CreatePipeline()
$pipeline.Commands.AddScript($script)
$pipeline.Invoke()
$runspace.Close()
Introduction to PowerShell Runspaces
What are Runspaces?
Runspaces in PowerShell are lightweight, isolated environments where PowerShell code can be executed. They enable the execution of commands and scripts in parallel, leading to improved performance, especially in scenarios where multiple tasks can run concurrently.
Why Use Runspaces?
PowerShell runspaces are excellent for efficiency. They facilitate the simultaneous execution of multiple commands without creating an entirely separate PowerShell process for each one, as is the case with background jobs. This can significantly reduce overhead and improve the overall speed of script execution.
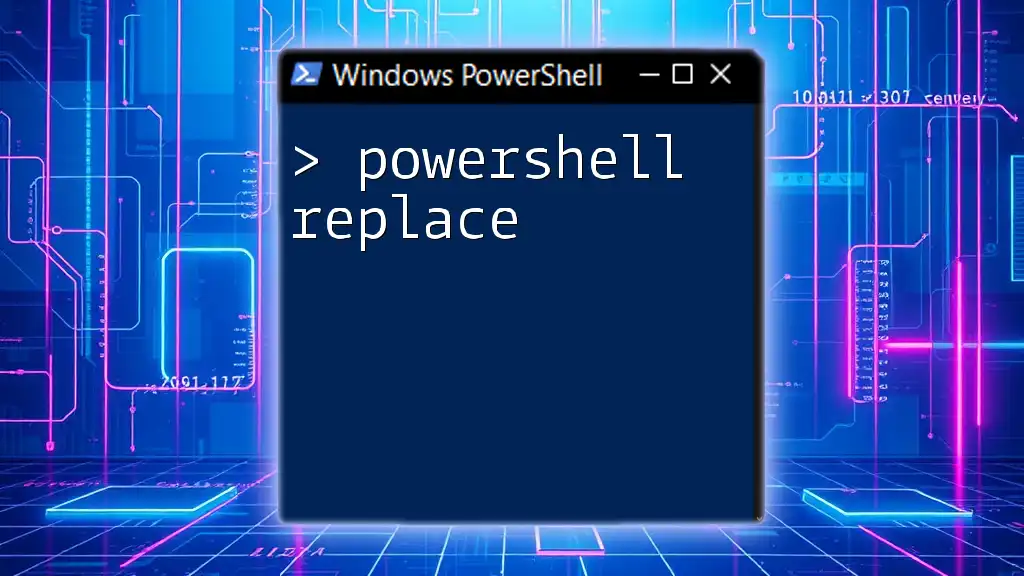
Understanding the Structure of Runspaces
Core Components of Runspaces
To get the most out of PowerShell runspaces, it’s essential to understand the primary components involved:
- Runspace: The core environment for executing PowerShell commands.
- Pipeline: A sequence of commands that are executed in a specific order.
- RunspacePool: A collection of runspaces that can be reused, enhancing performance and resource utilization.
Runspace Lifecycle
Runspaces have a distinct lifecycle that includes the following stages: creation, opening, execution, and closing. This structured lifecycle ensures that resources are managed efficiently.
Here’s a simple example of creating and opening a runspace:
$runspace = [powershell_runspace]::Create()
$runspace.Open()
# Use runspace ...
$runspace.Close()
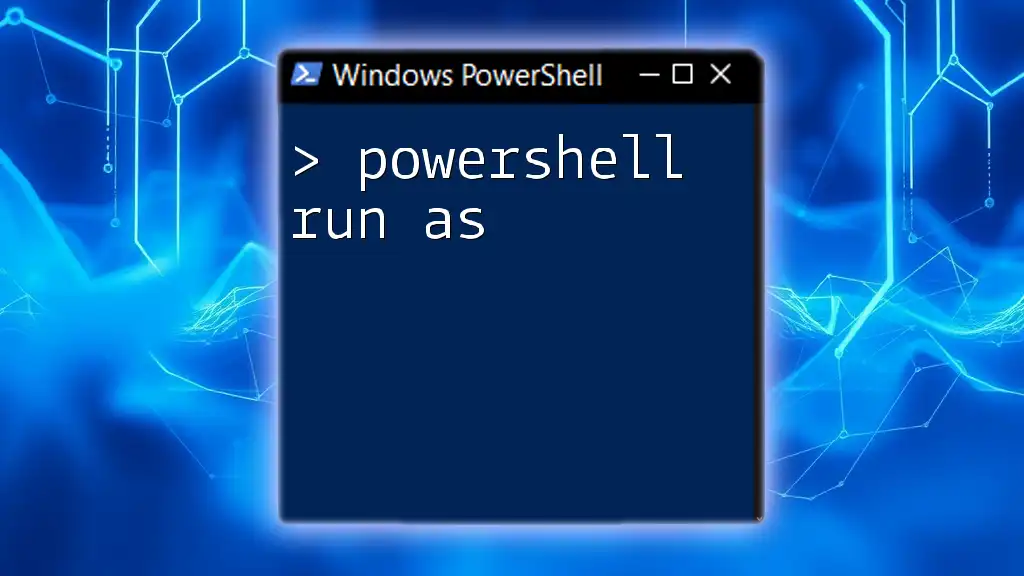
Setting Up Your First Runspace
Creating a Simple Runspace Example
To get started with runspaces, let’s create a simple example where we retrieve system information using the `Get-Process` cmdlet. This will demonstrate the essential setup.
$runspace = [powershell_runspace]::Create()
$powerShell = [powershell]::Create()
$powerShell.AddScript("Get-Process")
$runspace.Open()
$results = $powerShell.Invoke()
$runspace.Close()
In this example, we create a new runspace instance, along with a PowerShell instance to hold our script. We then open the runspace, execute the script using `Invoke()`, and close the runspace afterward.
Handling Output from Runspaces
Retrieving and managing output from runspaces is crucial. After executing a command in a runspace, you can capture the results as follows:
$results = $powerShell.Invoke()
foreach ($result in $results) {
Write-Output $result
}
This code iterates through the results and outputs each one, demonstrating how easy it is to work with the output data generated by runspaces.
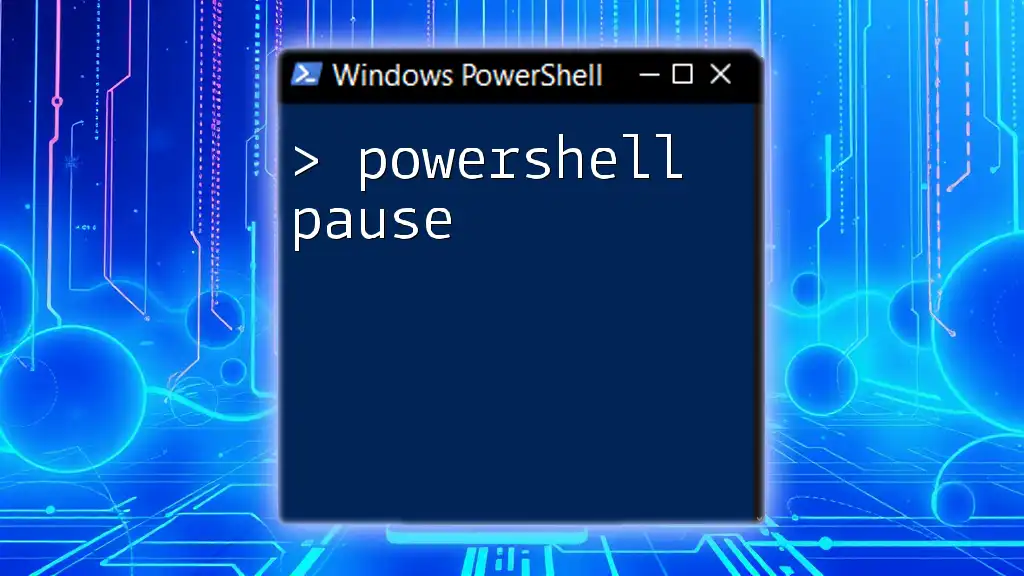
Advanced Runspace Concepts
Runspace Pools
Runspace pools allow you to manage multiple runspaces efficiently. By creating a pool, you can allocate a manageable number of runspaces that can be reused, promoting better resource management and reducing the overhead of frequently creating and disposing of runspaces.
Here’s an example of creating and using a runspace pool:
$runspacePool = [runspacefactory]::CreateRunspacePool(1, 5)
$runspacePool.Open()
# Execute multiple jobs within the pool...
$runspacePool.Close()
In this scenario, we create a runspace pool with a minimum of one and a maximum of five runspaces. This allows you to execute multiple tasks simultaneously while keeping resource consumption in check.
Working with Multiple Runspaces
Creating multiple runspaces for concurrent execution can enhance performance significantly. You can easily manage multiple scripts running in parallel.
Here’s an example illustrating how to run multiple scripts simultaneously using multiple runspaces:
$runspaces = @()
1..10 | ForEach-Object {
$runspace = [powershell_runspace]::Create()
# Prepare each runspace with a job/script...
}
In this loop, you can dynamically create a runspace for each task and prepare them for execution. This technique is beneficial in batch processing scenarios or tasks that can be easily parallelized.
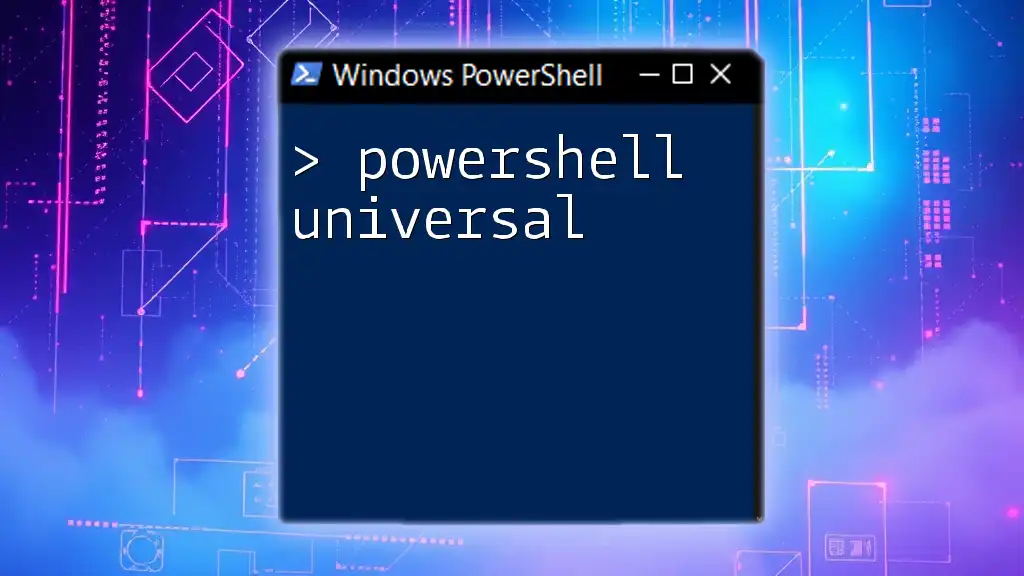
Error Handling in Runspaces
Common Errors and Exceptions
While working with runspaces, you may encounter various errors, such as failures in command execution or pipeline issues. Understanding how to handle these exceptions is key to building robust scripts.
Try/Catch Blocks for Error Management
Implementing error handling in runspaces is straightforward. Using try/catch blocks allows you to gracefully manage errors while running commands.
Here’s how you can use a try/catch structure within a runspace:
try {
# Runspace code...
} catch {
Write-Error "An error occurred: $_"
}
In this setup, if the code within the try block fails, the catch block will execute, providing an error message without crashing the entire script.
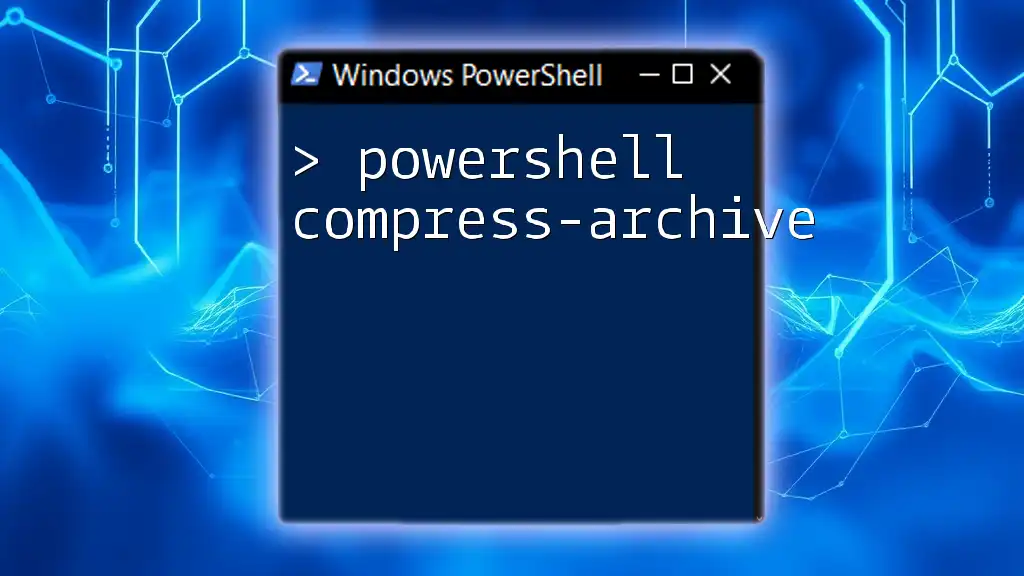
Optimizing Performance with Runspaces
Performance Tips for Runspaces
To maximize the efficiency of your runspaces, you should keep the following tips in mind:
- Batching commands: Executing commands in groups can lead to performance improvements.
- Avoid overloading: Respect the capabilities of your hardware to prevent performance bottlenecks.
Measuring Performance
You can compare the performance of runspaces against traditional sequential processing methods. Here’s a snippet to measure execution time:
$watch = [System.Diagnostics.Stopwatch]::StartNew()
# Runspace Execution
$watch.Stop()
Write-Output "Execution Time: $($watch.ElapsedMilliseconds) ms"
This sample code uses a stopwatch to measure how long it takes to run the commands, helping you evaluate the efficiency of your runspace implementation.
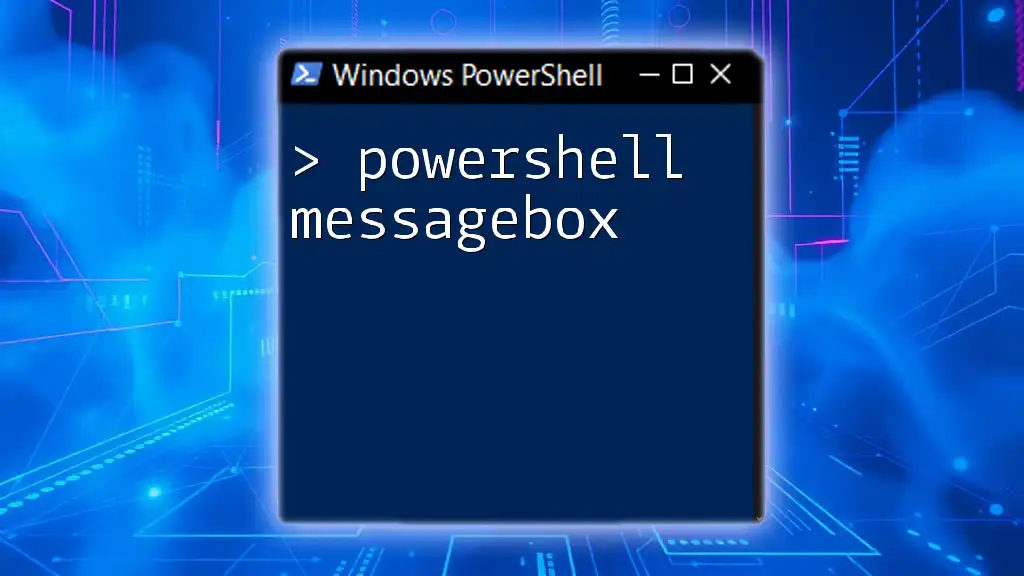
Use Cases for PowerShell Runspaces
System Administration Tasks
Runspaces are especially valuable for system administrators who regularly perform repetitive tasks. For example, gathering system metrics from multiple servers can be executed in parallel.
Data Processing and Automation
In data processing tasks, such as running reports or integrating various data sources, runspaces allow scripts to execute concurrently, ensuring minimal wait times and efficient resource use.
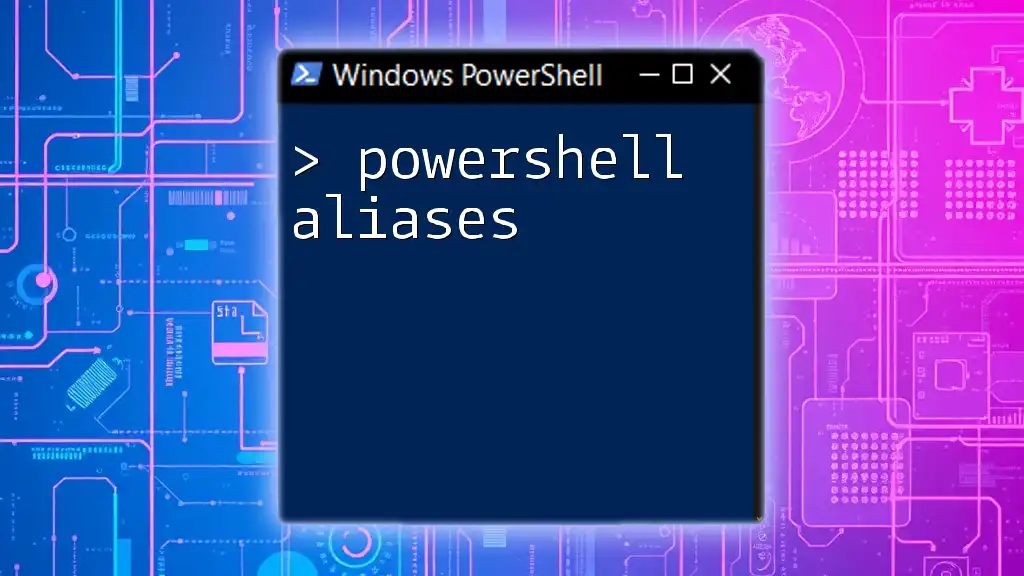
Conclusion
In recap, PowerShell runspaces are a powerful feature that enhances the execution of PowerShell scripts by allowing concurrent execution and efficient resource management. By understanding how to create, manage, and optimize runspaces, you can significantly boost your scripting capabilities.
I encourage you to experiment with runspaces in your PowerShell scripts. Explore the different functionalities and discover how runspaces can optimize your workflow and improve performance.
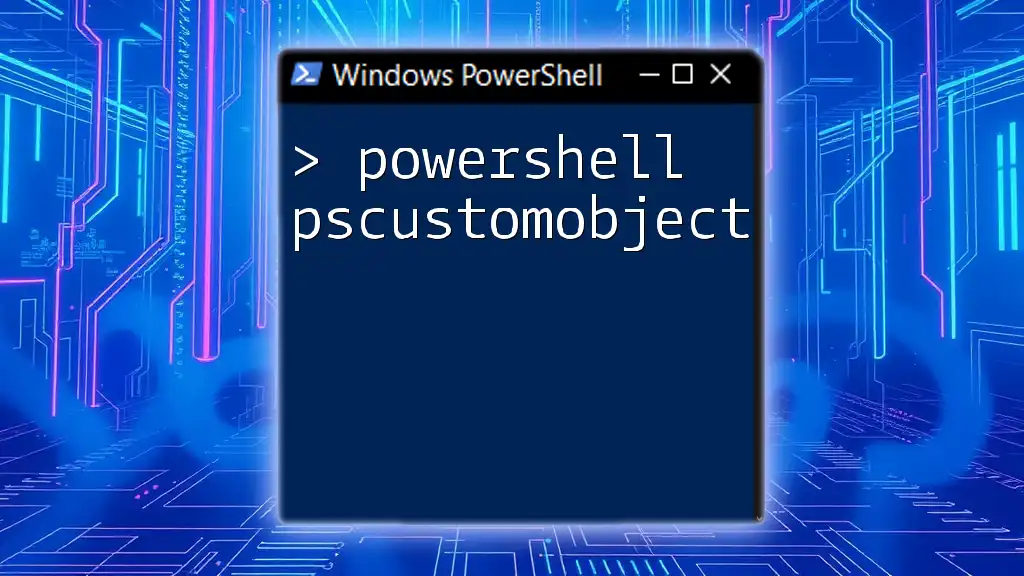
Additional Resources
Be sure to check out various online resources, blogs, and official documentation for further reading and advanced techniques regarding PowerShell runspaces. Feel free to share your experiences and follow our company for more insights and tutorials in the world of PowerShell!