The `-notmatch` operator in PowerShell is used to determine if a string does not match a specified regular expression pattern, returning `$true` if it does not match, and `$false` if it does.
Here’s a code snippet demonstrating its usage:
$testString = "Hello, World!"
if ($testString -notmatch "PowerShell") {
Write-Host "The string does not contain 'PowerShell'."
}
Understanding the NotMatch Operator
PowerShell is a powerful scripting language and command-line shell designed for system administration. One of its critical features is the ability to perform complex data filtering and manipulation through various comparison operators, including the NotMatch operator. Understanding this operator is essential for those looking to streamline their scripting and automate their tasks.
What Does NotMatch Do?
The NotMatch operator, denoted as `-notmatch`, is used to evaluate a string against a regular expression and returns True if the string does not match the pattern defined by the regex. This operator plays a crucial role in data filtering and validation.
By contrast, when you see `-match`, it indicates a positive match—meaning the string fits the provided pattern. The NotMatch operator is particularly useful when you want to exclude data that fits certain criteria.
Syntax of NotMatch
To leverage the NotMatch operator, you need to familiarize yourself with its syntax. The general syntax is straightforward:
"String" -notmatch "Pattern"
Here's a simple example to illustrate its use:
"Sample Text" -notmatch "Text"
In this case, the expression evaluates to False because "Sample Text" contains "Text."
Using Regular Expressions
One of the most powerful aspects of the NotMatch operator is its integration with regular expressions (regex). Regular expressions allow for more complex pattern matching.
Consider this example where we wish to find strings that do not contain any numeric digits:
"Sample123" -notmatch "\d+" # This returns False because the string contains numbers.
In contrast, for a string without digits:
"Sample" -notmatch "\d+" # This returns True because the string contains no digits.
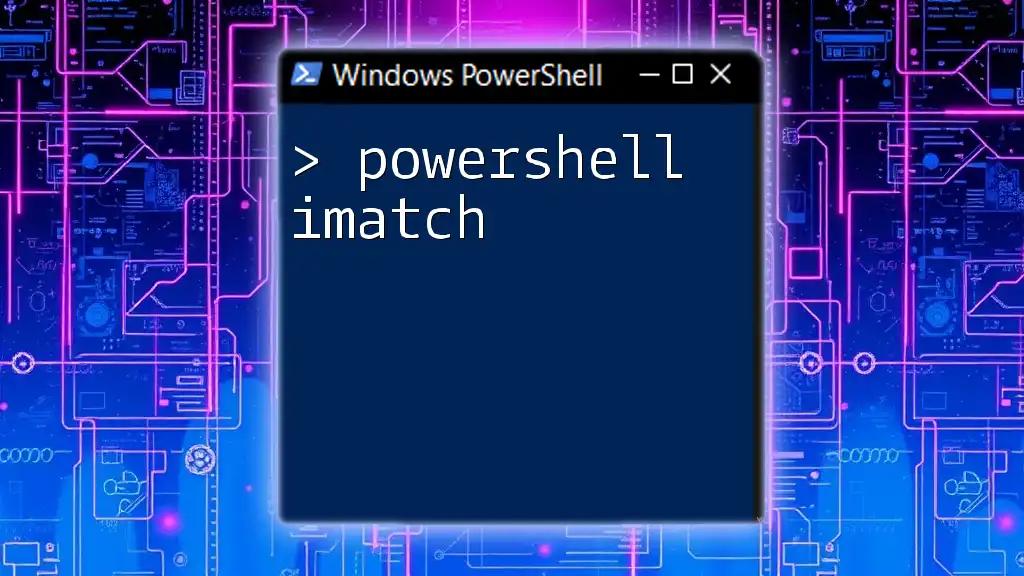
Practical Examples of Using NotMatch
Filtering Data with NotMatch
Example: Filtering Strings
You can utilize NotMatch to filter out unwanted strings from an array. For instance, if you want to exclude any fruits containing the substring "berry":
$array = "apple", "banana", "cherry", "date", "elderberry"
$filtered = $array | Where-Object { $_ -notmatch "berry" }
In this example, the resulting collection assigned to `$filtered` will include apple, banana, date—effectively excluding cherry and elderberry.
Example: Working with Files
Another practical application of the NotMatch operator is when you are working with file names. Imagine you want to list all files in a directory except those with a `.txt` extension:
Get-ChildItem | Where-Object { $_.Name -notmatch "\.txt$" }
This command retrieves all files and filters the results, omitting any files that end with `.txt`.
Advanced Scenarios Using NotMatch
Combining NotMatch with Other Operators
The NotMatch operator can be combined with other logical operators to create more complex queries. For example, suppose you want to list an array while excluding any elements that contain either "fruit" or "vegetable":
$array | Where-Object { $_ -notmatch "fruit" -and $_ -notmatch "vegetable" }
This incorporation of logical operators allows for fine-tuned control over the output of your command.
Using NotMatch with Custom Objects
When dealing with custom objects, NotMatch can still be effectively integrated. For example, consider you have an array of custom objects with user roles and you want to filter out admins:
$customObjects = @(
[PSCustomObject]@{ Name = "John Doe"; Role = "Admin" },
[PSCustomObject]@{ Name = "Jane Smith"; Role = "User" },
[PSCustomObject]@{ Name = "Alice Johnson"; Role = "User" }
)
$filteredUsers = $customObjects | Where-Object { $_.Role -notmatch "Admin" }
In this case, `$filteredUsers` will only contain the entries for Jane Smith and Alice Johnson.

Troubleshooting Common NotMatch Issues
Common Pitfalls
While using the NotMatch operator, it's easy to run into common pitfalls. A prominent issue relates to case sensitivity; `-notmatch` is case-sensitive by default. For example, the following will yield False:
"Sample" -notmatch "sample" # Returns False
Another common mistake occurs with regex syntax errors, which can cause unexpected results or errors.
Debugging Tips
To troubleshoot potential issues, it’s beneficial to break down your expression and test each component separately. If the command is not returning the expected results, simplify the regex pattern until you find where the error lies. Additionally, utilizing the `Get-Member` cmdlet can help you inspect the properties and methods of your objects while debugging.
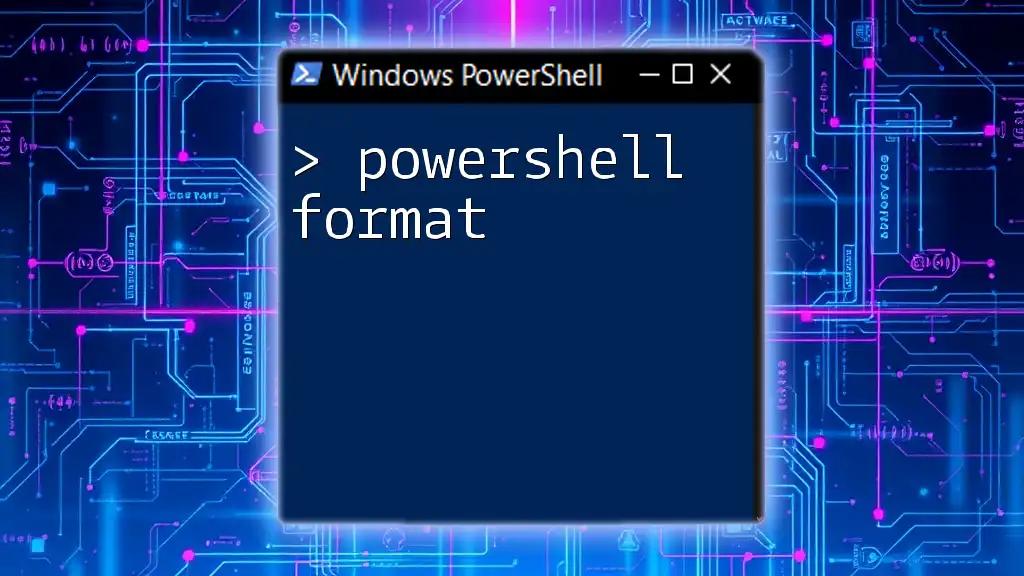
Conclusion
In summary, understanding and effectively using the NotMatch operator in PowerShell opens up a wealth of possibilities for data manipulation and filtering. By employing it with regular expressions, combining it with other logical operators, and applying it to arrays and custom objects, you can create powerful scripts that streamline your workflow.
To deepen your understanding, consider experimenting with various regex patterns and scenarios to become more proficient in using NotMatch. Engage with online resources, forums, and communities dedicated to PowerShell to further enhance your skills.