The `-match` operator in PowerShell is used to determine if a string matches a specified regular expression pattern, returning $true or $false based on the match result.
$inputString = "Hello, World!"
$pattern = "World"
$result = $inputString -match $pattern
Write-Host $result # This will output: True
What is Regex?
Regular Expressions (regex) are a powerful tool for string searching and manipulation. They are essentially patterns that can be used to match character combinations in strings. In scripting and automation, regex is invaluable for tasks such as searching for specific text, validating user input, or even extracting data from larger strings.
Basic Components of Regex
Literal Characters
Literal characters are the most straightforward regex elements. They represent the exact characters you want to match. For example, if you want to check if a string contains the term "PowerShell", you can do so using:
"PowerShell is great" -match "PowerShell" # Returns True
Special Characters and Metacharacters
Regex also includes special characters that modify how matches are interpreted. Here are a few common ones:
- Dot (`.`): Matches any single character except a newline.
- Asterisk (`*`): Matches zero or more occurrences of the preceding element.
- Plus Sign (`+`): Matches one or more occurrences of the preceding element.
- Caret (`^`): Indicates the start of a string.
- Dollar Sign (`$`): Indicates the end of a string.
For example, using the dot to match any character would look like this:
$string = "cater"
if ($string -match "c.t.e.r") {
"Matched!"
}

PowerShell's -match Operator
The `-match` operator in PowerShell is specifically designed to allow you to perform regex-based pattern matching against strings. It returns `True` if the pattern matches and `False` otherwise.
Syntax and Basic Usage
The syntax of `-match` looks like this:
<expression> -match <regex_pattern>
Here’s a basic example:
$string = "PowerShell is awesome"
$string -match "awesome" # Returns True
In this instance, the string "PowerShell is awesome" passes the `-match` test with the regex pattern "awesome".

Working with Regular Expressions in PowerShell
Capturing Groups
Capturing groups allow you to retrieve specific parts of the string that you’re matching. Groups are defined in regex using parentheses `()`.
How to Use Capturing Groups
For instance, if you want to extract the year, month, and day from a date string formatted as "YYYY-MM-DD", you can use capturing groups:
$string = "Today is 2023-10-19"
if ($string -match '(\d{4})-(\d{2})-(\d{2})') {
$year = $matches[1]
$month = $matches[2]
$day = $matches[3]
}
In this code, `$matches[1]` will store "2023", `$matches[2]` will store "10", and `$matches[3]` will store "19".
Using the $matches Automatic Variable
The `$matches` automatic variable gets auto-populated with the results of the last successful `-match`.
Explanation of $matches
When you perform a match, PowerShell uses the `$matches` array to hold the matches. The whole match is in `$matches[0]`, and any capturing groups from your regex pattern are stored from `$matches[1]` onward.
Example:
$string = "Today is 2023-10-19"
if ($string -match '(\d{4})-(\d{2})-(\d{2})') {
$year = $matches[1] # Outputs 2023
$month = $matches[2] # Outputs 10
$day = $matches[3] # Outputs 19
}
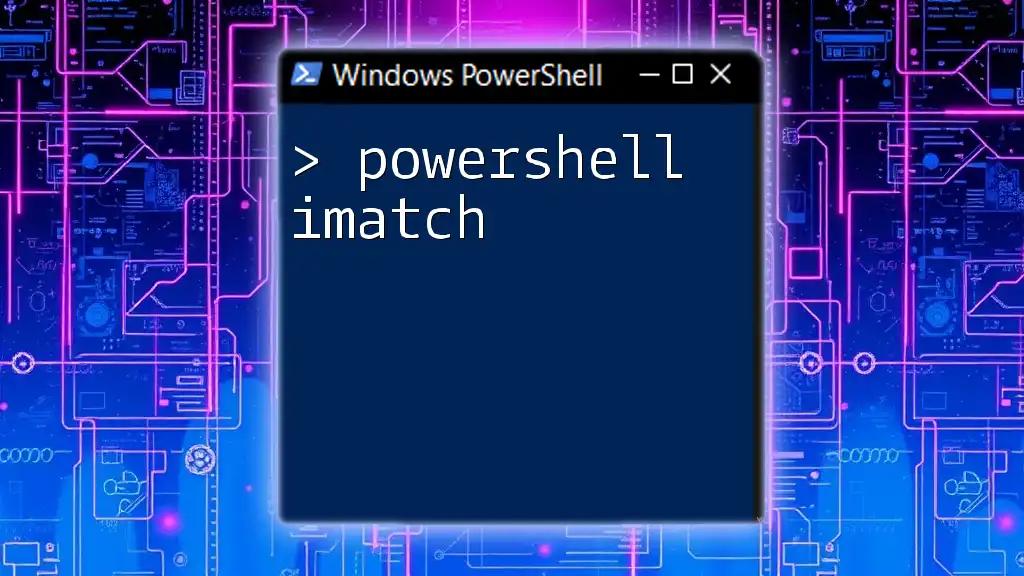
Common Regex Patterns for PowerShell
Email Validation
Validating email addresses is a common use case for regex in PowerShell. A basic regex pattern for matching valid email addresses looks like this:
$email = "test@example.com"
if ($email -match '^[\w-\.]+@([\w-]+\.)+[\w-]{2,4}$') {
"Valid Email"
}
This pattern checks for the structure of emails, ensuring they follow a standard format.
Phone Number Validation
Similarly, you can create a regex pattern that validates U.S. phone numbers:
$phone = "(555) 123-4567"
if ($phone -match '^\(\d{3}\) \d{3}-\d{4}$') {
"Valid Phone Number"
}
This regex pattern ensures that the phone number matches the expected format of `(XXX) XXX-XXXX`.
URL Matching
Regex can also be effective for validating URLs. Here’s an example:
$url = "https://www.example.com"
if ($url -match '^(https?://)?(www\.)?([-a-zA-Z0-9@:%_+.~#?&//=]*)$') {
"Valid URL"
}
This pattern checks for an optional "http://" or "https://" prefix and a valid domain structure.

Best Practices for Using Regex in PowerShell
Performance Considerations
When using regex, remember that complex patterns can lead to performance issues. It's usually best to keep regex as simple and readable as possible. Break down complex patterns into smaller ones whenever feasible to enhance both clarity and execution time.
Error Handling
Not all matches will succeed, and it's essential to have error handling in place. You can use `try-catch` structures when working with regex to manage potential failures gracefully.
try {
$result = "example" -match '[A-Za-z]'
} catch {
Write-Host "An error occurred: $_"
}
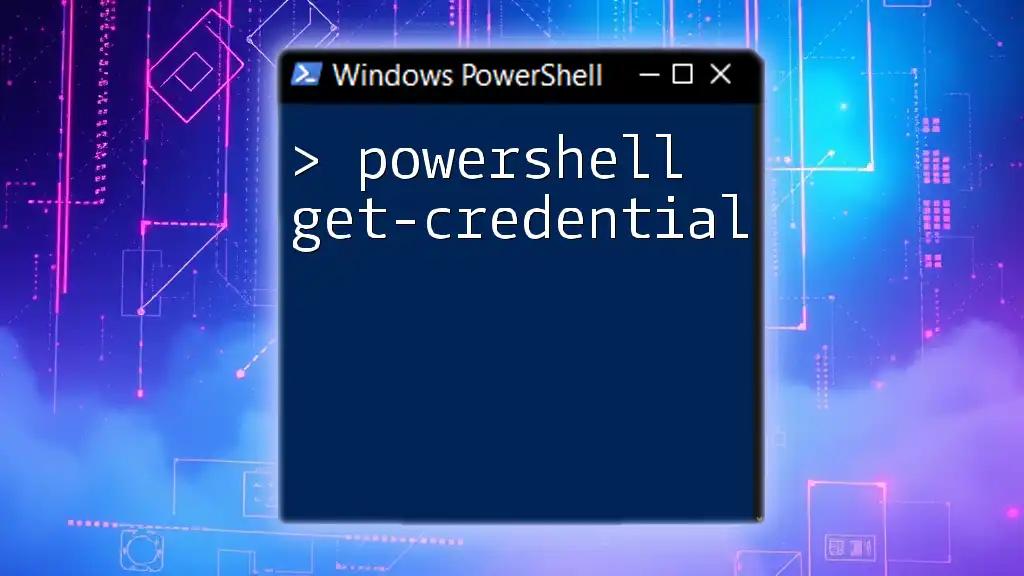
Conclusion
Through this guide, we have explored how to effectively use the `-match` operator with regex in PowerShell. Understanding patterns, capturing groups, and best practices ensures that you can harness the full power of regex in your scripting endeavors. Practice with the provided examples to deepen your comfort and expertise with PowerShell regex.

Additional Resources
For further exploration, consider looking into the official PowerShell documentation related to regex and PowerShell's string manipulation capabilities. Online regex testers can also be invaluable when crafting more complex patterns. Deepening your knowledge in both regex and PowerShell will significantly boost your scripting effectiveness.