In PowerShell, you can use regular expressions (regex) to search and replace text in strings efficiently using the `-replace` operator.
Here's a code snippet demonstrating how to replace all occurrences of "cat" with "dog":
$string = "The cat sat on the mat."
$modifiedString = $string -replace 'cat', 'dog'
Write-Host $modifiedString
Understanding PowerShell and Regex
PowerShell is a powerful scripting language and command-line shell predominantly used for system administration and automation tasks. It provides a rich set of tools and functionalities to manage various system components effectively.
Regular Expressions (Regex) are sequences of characters that form a search pattern, primarily used for string searching and manipulation. Regex is beneficial in enhancing the capabilities of PowerShell when it comes to handling strings, especially for complex matching and replacements.
The Need for Regex Replacement in PowerShell
Regex is indispensable for string replacement due to its ability to match complex patterns easily. Unlike traditional string replacement methods, regex allows for nuanced control over what exactly is searched for and replaced.
Common scenarios where regex replacement shines include:
- Data cleansing: Removing unwanted characters or formatting from datasets.
- Configuration file modifications: Adjusting settings based on patterns.
- Log file parsing: Extracting meaningful data from unstructured logs.

Using the `-replace` Operator in PowerShell
The `-replace` operator in PowerShell is the most commonly utilized method for regex-based string replacement. Its basic syntax is:
$string -replace 'pattern', 'replacement'
Example: Basic String Replacement
Here’s a simple demonstration of using the `-replace` operator:
# Example: Simple string replacement
$originalString = "Hello World"
$modifiedString = $originalString -replace "World", "PowerShell"
Write-Output $modifiedString # Output: Hello PowerShell
In this example, the word "World" is replaced with "PowerShell," illustrating how straightforward the usage of `-replace` can be for simple string replacements.
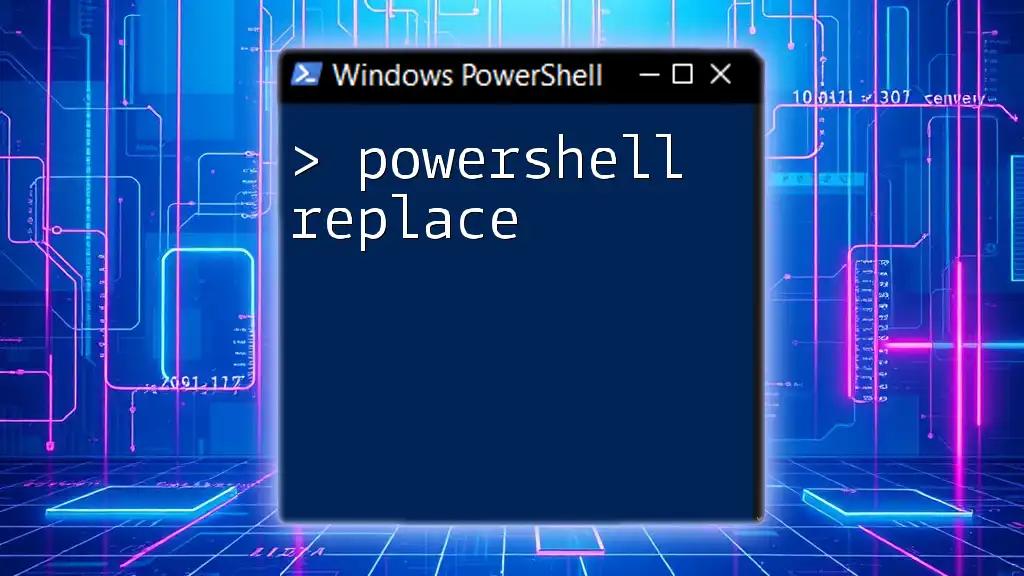
PowerShell Regular Expression Replace: Advanced Patterns
To leverage the full power of the `-replace` operator, understanding complex regex patterns becomes essential. Regex allows the formation of patterns that can match a variety of inputs, based on defined criteria, such as character classes, quantifiers, and anchors.
Example: Using Character Classes
Character classes allow matching any one of a set of characters. Here’s an example of removing all digits from a string:
# Example: Removing all digits from a string
$inputString = "PowerShell 2023"
$resultString = $inputString -replace "[0-9]", ""
Write-Output $resultString # Output: PowerShell
In this snippet, the regex pattern `[0-9]` matches any digit from 0 to 9, and replaces it with an empty string, effectively removing all numbers.

Using Capture Groups in Replacement
Capture groups are an advanced feature in regex that allows you to reference matched elements and rearrange them in the replacement text.
Example: Replacing with Capture Groups
Utilizing capture groups can be particularly effective when you need to manipulate text format. For instance, you might want to swap the first and last names:
# Example: Swapping first and last names
$nameString = "Doe, John"
$result = $nameString -replace "(\w+), (\w+)", '$2 $1'
Write-Output $result # Output: John Doe
In this example, `(\w+)` captures sequences of word characters—once for the last name and once for the first name—and `$2 $1` reorders them in the output.

Powershell String Replace Regex: Edge Cases and Limitations
Handling edge cases is important when working with regex. One crucial factor to consider is case sensitivity. By default, PowerShell's `-replace` operator is case-sensitive, which might yield unexpected results.
Example: Case Insensitive Replacement
To demonstrate a case-insensitive replacement, consider the following example:
# Example: Case insensitive replacement
$text = "PowerShell is awesome. powershell is powerful."
$result = $text -replace "powershell", "PowerShell", 'IgnoreCase'
Write-Output $result # Output: PowerShell is awesome. PowerShell is powerful.
In this case, both occurrences of "powershell" are replaced by "PowerShell," regardless of letter casing.
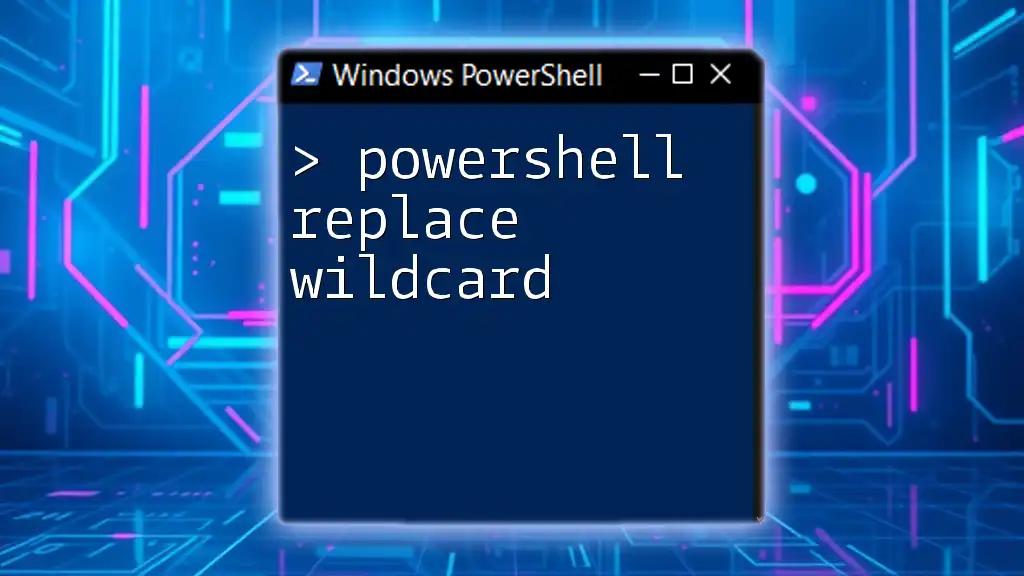
Best Practices for Using Regex Replace in PowerShell
When crafting your regex, it's essential to consider efficiency and readability. Keep these tips in mind for enhancing your regex usage:
-
Test and Debug Regex Patterns: Utilize tools like [regex101.com](https://regex101.com) to validate your expressions before applying them in scripts. This can help identify any potential pitfalls early.
-
Avoid Common Pitfalls: Some regex patterns can lead to performance issues, especially those that employ excessive backtracking. It's beneficial to profile your regex and optimize it for quicker execution.

Conclusion
In this guide, we explored the intricacies of using PowerShell replace regex for a variety of string manipulation tasks. From basic string replacements to complex patterns utilizing capture groups, regex in PowerShell opens up a world of possibilities for efficient string handling.
By understanding and implementing the strategies discussed, you're now well-equipped to handle any regex-based string replacement tasks in your PowerShell scripts. Start experimenting with these techniques to enhance your automation scripts and data manipulation capabilities.

Additional Resources
For further learning, consider exploring the PowerShell documentation for the `-replace` operator and additional regex resources such as cheat sheets or dedicated regex tutorials.