The PowerShell `Replace` method allows you to substitute specified substrings within a string with new values, enhancing data manipulation and text processing capabilities.
$string = "Hello, World!"
$modifiedString = $string.Replace("World", "PowerShell")
Write-Host $modifiedString # Outputs: Hello, PowerShell!
Understanding the Basics of String Manipulation
The PowerShell Replace Method operates on strings, which are sequences of characters. In PowerShell, a string is a basic data type, and mastering its manipulation is crucial for scripting and automation. String manipulation allows you to modify text dynamically, making it an invaluable tool in your PowerShell toolkit.
The Replace Method specifically enables you to substitute specific parts of a string with another value, giving you control over how strings are processed and displayed.
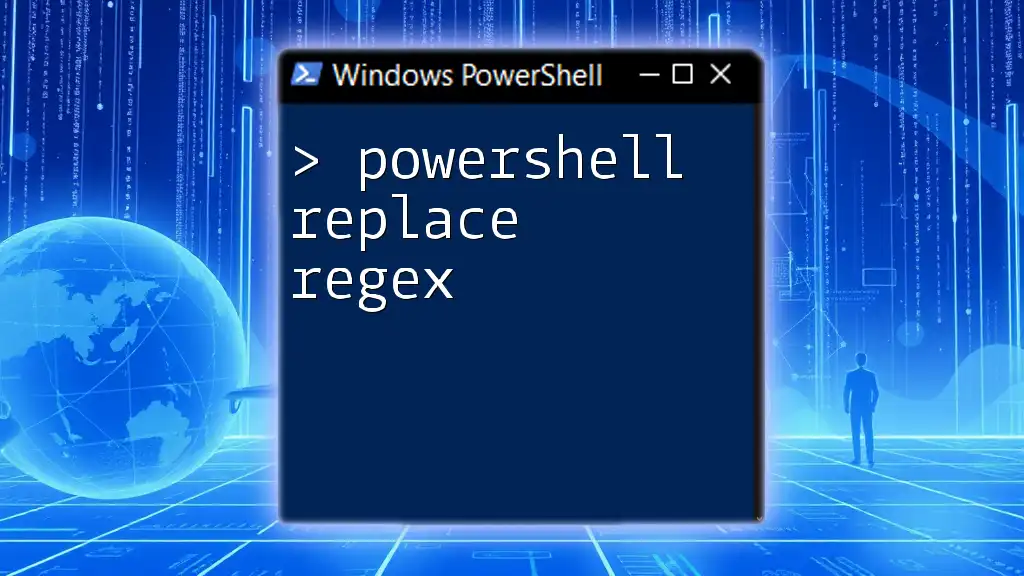
The Replace Method Explained
The syntax for using the Replace Method in PowerShell is straightforward:
string.Replace(oldValue, newValue)
Here’s a breakdown of the components:
- string: The original string you want to manipulate.
- oldValue: The substring that you wish to replace.
- newValue: The substring that will replace the `oldValue`.
When you use the Replace method, it returns a new string with the specified replacements made; it does not change the original string. This is a critical characteristic of string manipulation in PowerShell, as it maintains data integrity while providing the flexibility to work with variations of the original string.
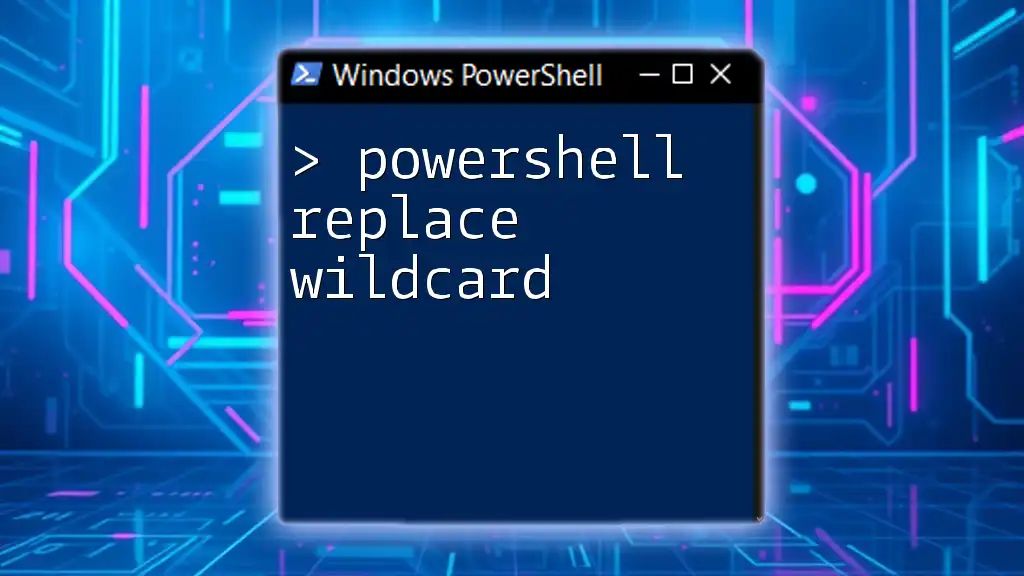
Practical Examples of Using the Replace Method
Basic Usage Examples
A simple and effective illustration of the PowerShell Replace Method is shown here:
$originalString = "Hello, World!"
$newString = $originalString.Replace("World", "PowerShell")
In this code snippet, `"World"` is replaced with `"PowerShell"`. As a result, the `newString` variable now contains `"Hello, PowerShell!"`.
This behavior exemplifies how the Replace method provides a quick and efficient means to modify string content.
Replacing Multiple Instances
If you need to replace multiple occurrences of a substring, the Replace method can handle this seamlessly. Consider the following example:
$text = "The rain in Spain stays mainly in the plain."
$updatedText = $text.Replace("in", "on")
In this scenario, the method will replace every instance of `"in"` with `"on"`. After execution, `updatedText` will read, "The rain on Spain stays mainly on the plain." This can be useful in various applications, such as formatting text or correcting typos across a document.
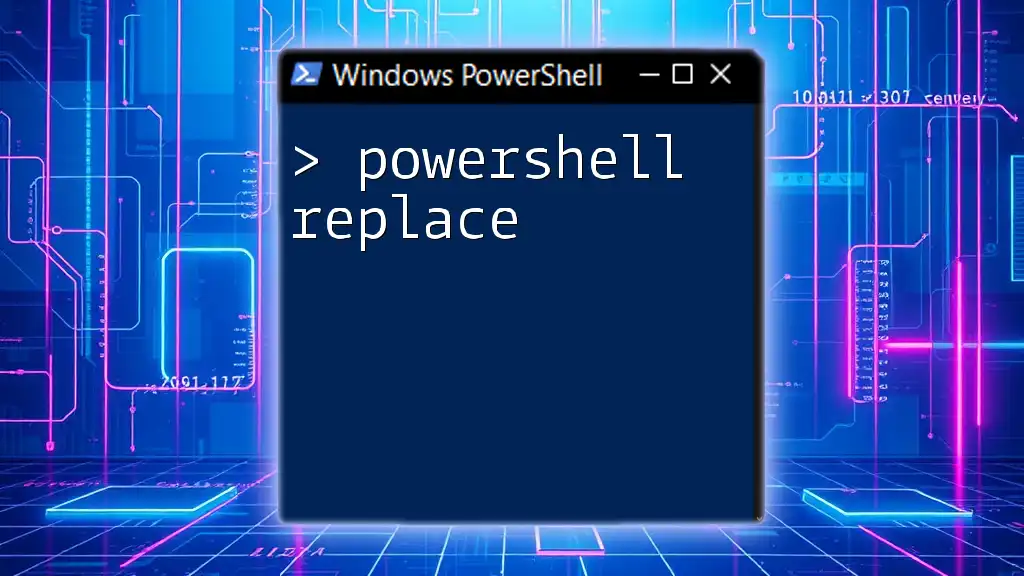
Advanced Scenarios with the Replace Method
Case Sensitivity
It is essential to understand that the Replace method is case-sensitive. This means that using different cases for `oldValue` and the string being searched will not produce a replacement. For instance:
$stringCase = "PowerShell is powerful"
$replacedCase = $stringCase.Replace("powerful", "awesome")
In this example, since "powerful" does not match "PowerShell" (due to case differences), no replacement occurs, and `replacedCase` remains `"PowerShell is powerful"`.
Using Regular Expressions for Complex Replacements
For more complex string manipulation, you can utilize regular expressions alongside the Replace method. This approach allows for more nuanced replacements based on patterns rather than fixed strings.
For example:
$input = "123-456-7890"
$result = [regex]::Replace($input, '\d{3}', 'XXX')
In this case, the regular expression `'\d{3}'` identifies every group of three digits. The outcome is that all three-digit sets in the string will be replaced with "XXX", resulting in `result` equal to `"XXX-XXX-XXX0"`. This is particularly powerful for operations such as data masking or transformation.
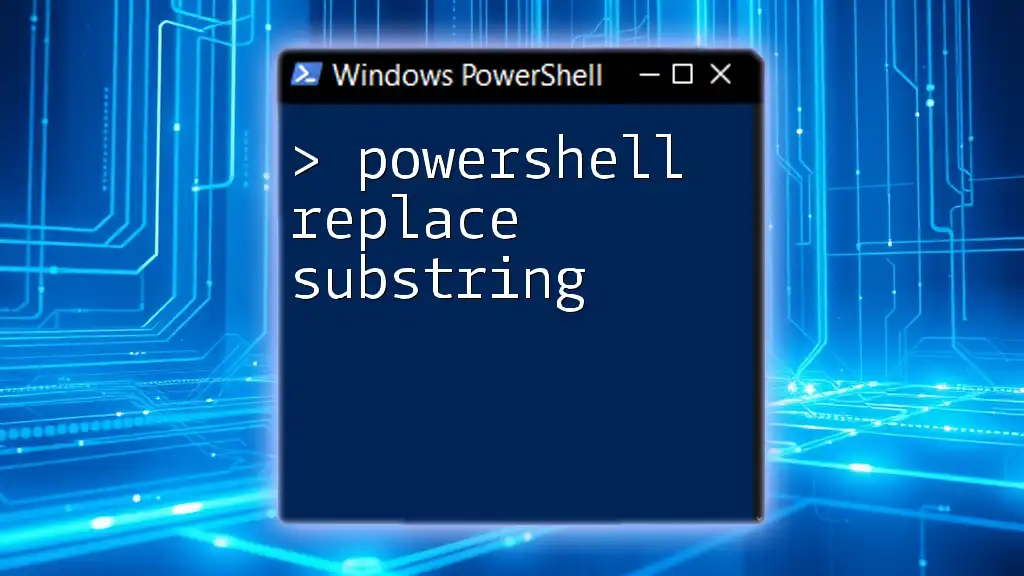
Common Issues and Troubleshooting
Common Errors When Using Replace
One frequent mistake when utilizing the PowerShell Replace Method is failing to account for the case sensitivity. If the substring you are searching for does not match exactly, no changes will be made.
Another common issue arises when the `oldValue` you are trying to replace doesn't exist in the string. If that’s the case, the original string remains unchanged.
Troubleshooting Tips
When issues arise, the best approach is to just print the original and resultant strings to understand whether any changes have taken place. For instance:
Write-Host "Original: $originalString"
Write-Host "Updated: $newString"
This can shed light on whether the Replace method worked as expected.
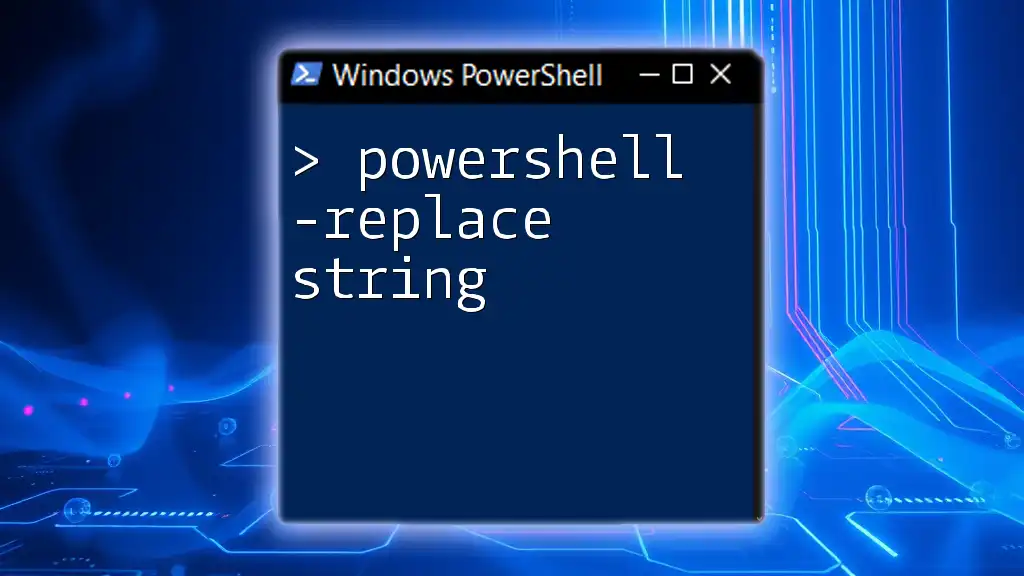
Best Practices for Using the Replace Method
When to Use the Replace Method
The Replace Method should be your go-to for straightforward string substitutions. It is efficient for quick fixes or for real-time data transformation within scripts.
Performance Considerations
While the Replace method is performant for most tasks, avoid using it in tight loops or handling very large strings where frequent replacements are necessary. In such cases, you may want to explore other methods or string-building techniques to enhance efficiency.
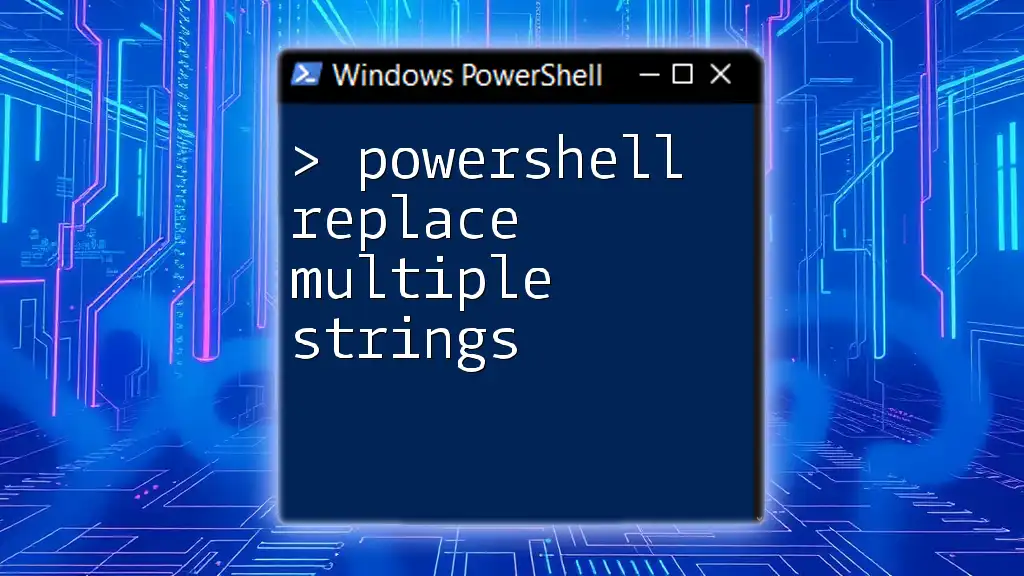
Conclusion
In summary, the PowerShell Replace Method proves to be a versatile tool for any PowerShell user. It allows for easy string manipulation, whether you're executing basic substitutions or undertaking complex regex-based replacements. Gaining proficiency with the Replace method will undoubtedly enhance your scripting capabilities, allowing for dynamic and efficient automation solutions.
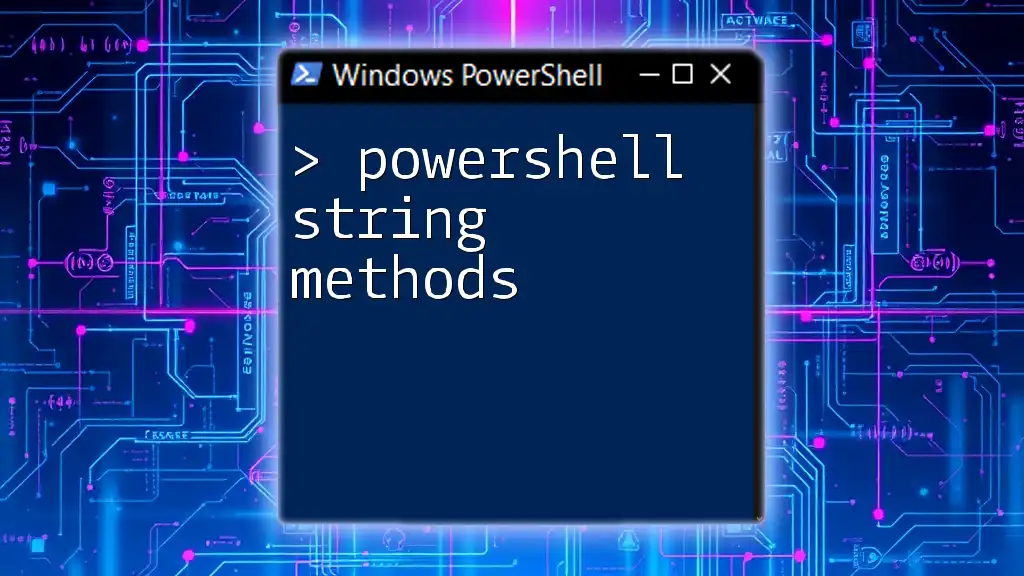
Additional Resources
For those interested in furthering their PowerShell knowledge and skills, numerous resources exist, including official PowerShell documentation and vibrant community forums. Engaging with these materials can provide deeper insights and foster a more robust understanding of PowerShell and its capabilities.