In PowerShell, you can replace multiple strings in a single operation using the `-replace` operator along with a loop or a hashtable to map the strings you want to replace to their corresponding replacements. Here's a code snippet demonstrating this method:
$string = "Hello, World! Welcome to the World of PowerShell."
$replacements = @{ "World" = "Universe"; "Welcome" = "Greetings" }
foreach ($key in $replacements.Keys) {
$string = $string -replace $key, $replacements[$key]
}
Write-Host $string
This code replaces "World" with "Universe" and "Welcome" with "Greetings" in the input string.
Understanding String Replacement in PowerShell
What is String Replacement?
String replacement in PowerShell refers to the process of substituting parts of a string with new values. This functionality is crucial in automating tasks that require text formatting, data manipulation, or updating textual information in scripts.
Why Use PowerShell for String Manipulation?
PowerShell is a powerful scripting language that excels in handling various data types, including strings. One of the primary advantages of using PowerShell for string manipulation is its intuitive syntax and built-in operators that simplify complex tasks. Unlike many other scripting languages, PowerShell integrates seamlessly with .NET, allowing for advanced string handling capabilities without extensive code.
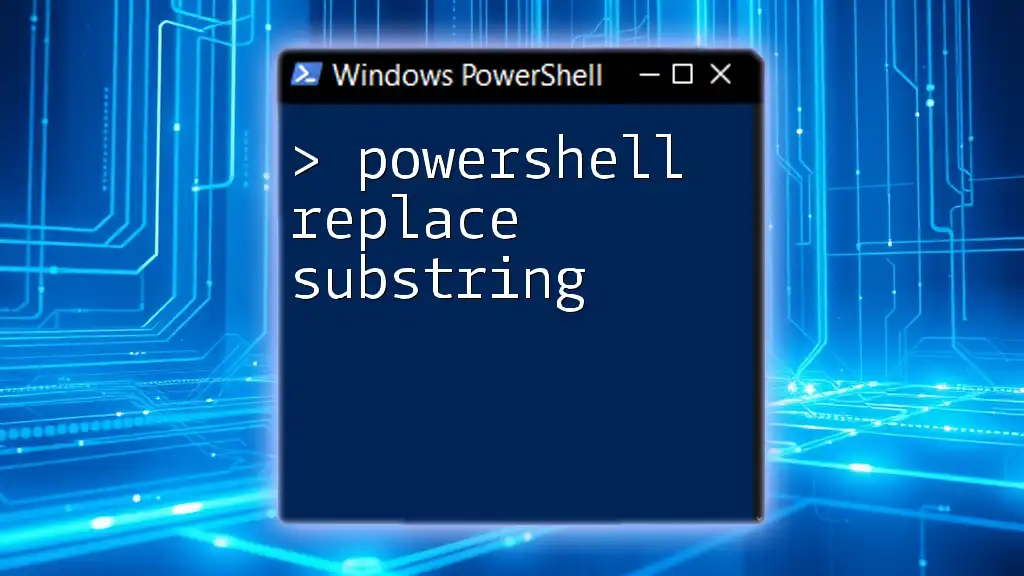
Basic String Replacement in PowerShell
The `-replace` Operator
At the core of string replacement in PowerShell is the `-replace` operator. This operator utilizes regular expressions, making it flexible and powerful for pattern matching.
Syntax:
String -replace 'Pattern', 'Replacement'
Example:
$string = "Hello World"
$result = $string -replace "World", "PowerShell"
Write-Output $result # Output: Hello PowerShell
In this example, we see how the `-replace` operator efficiently substitutes "World" with "PowerShell," demonstrating the operator's straightforward approach.
Replacing a Single String
You can easily replace a single substring using the `-replace` operator.
Example:
$text = "I love apples"
$updatedText = $text -replace "apples", "oranges"
Write-Output $updatedText # Output: I love oranges
In the above code, "apples" is replaced with "oranges" in the initial string. This method is both simple and effective for single-value substitution.
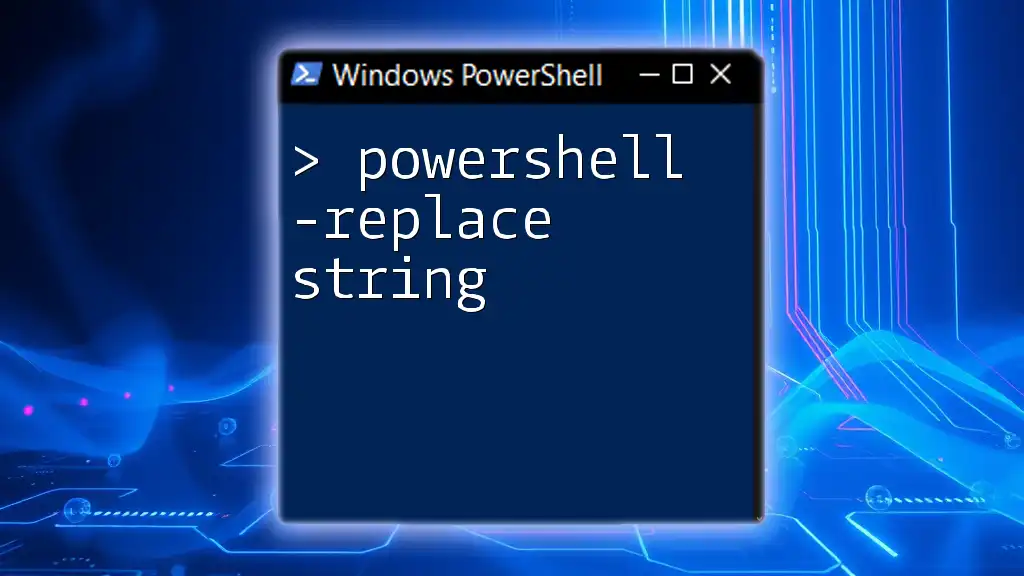
Advanced String Replacement Techniques
Replacing Multiple Strings with a Hash Table
When you need to perform multiple string replacements, you can use a hash table, significantly simplifying your code.
Using a Hash Table for Replacements: A hash table allows you to define pairs of patterns and their corresponding replacements.
Example:
$text = "I love apples and bananas"
$replacements = @{"apples"="oranges"; "bananas"="grapes"}
foreach ($key in $replacements.Keys) {
$text = $text -replace $key, $replacements[$key]
}
Write-Output $text # Output: I love oranges and grapes
In this example, we define a hash table with each fruit mapped to its replacement. The `foreach` loop iterates over the key-value pairs, replacing each occurrence in a single, organized operation.
Using `foreach` Loop for Multiple Replacements
An alternative approach is to leverage a `foreach` loop combined with arrays for flexibility.
Example:
$text = "I like Java and Python"
$replacements = @("Java", "Python")
$newValues = @("C#", "Ruby")
for ($i = 0; $i -lt $replacements.Length; $i++) {
$text = $text -replace $replacements[$i], $newValues[$i]
}
Write-Output $text # Output: I like C# and Ruby
Here, the `for` loop traverses the replacement arrays, enabling you to execute multiple replacements in a systematic manner. This technique affords greater control over the substitution process.
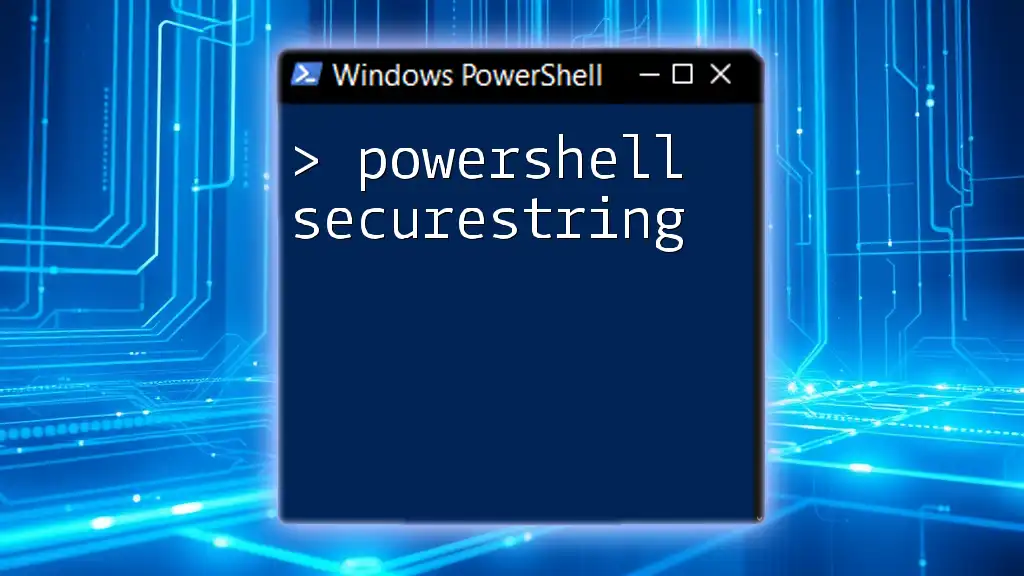
Using Regular Expressions for More Complex Cases
Introduction to Regular Expressions
Regular expressions (regex) are a powerful tool for pattern matching and string manipulation. They allow you to define complex patterns and perform advanced string replacements.
Replacing Patterns with Regular Expressions
The `-replace` operator also supports regex for pattern-based replacements.
Example: Replace all digits with a specific character:
$text = "My phone number is 123-456-7890"
$newText = $text -replace '\d', '#'
Write-Output $newText # Output: My phone number is ###-###-####
In this example, we replace all numeric digits (defined by `\d`) with the character `#`. This showcases how regex can be utilized for more efficient text processing.
Handling Special Characters with Regex
Using regular expressions involves escaping special characters that might otherwise be misinterpreted.
Example: Replacing special characters:
$text = "File *name*?.txt"
$escapedText = $text -replace '[\*\?]', '_'
Write-Output $escapedText # Output: File _name___.txt
In this instance, the special characters `*` and `?` are replaced with underscores. This demonstrates the necessity of proficient regex handling to manage diverse string formats effectively.
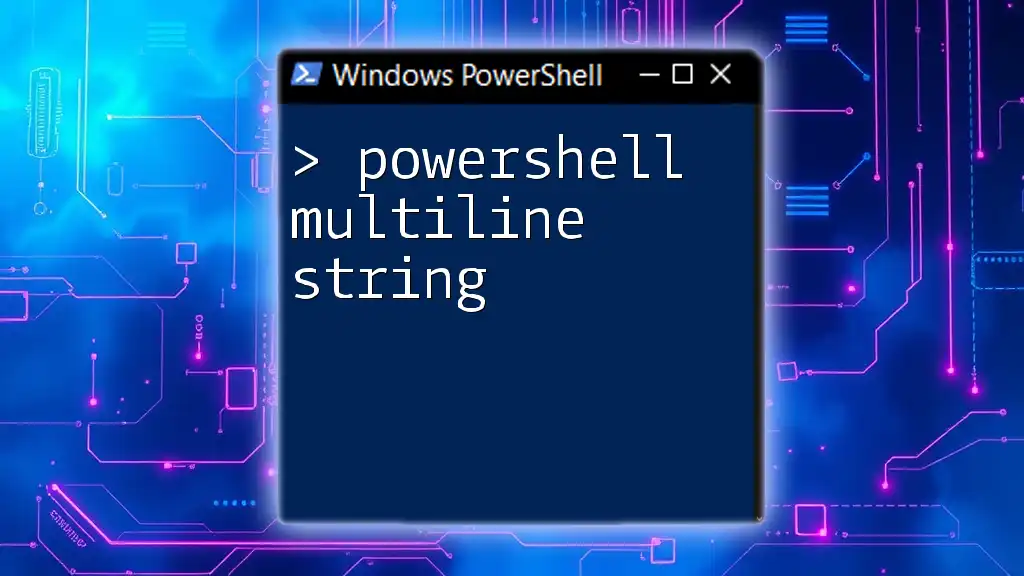
Performance Considerations
When to Use Bulk Replacements vs. Individual Replacements
When working with multiple string replacements, especially in larger strings or extensive datasets, consider the performance implications. Utilizing a hash table or a `foreach` loop can drastically enhance efficiency compared to multiple calls to `-replace`.
For scripts that require high performance, opt for bulk replacements to minimize the overhead. However, in simpler scenarios, individual replacements may be more straightforward and easier to read.
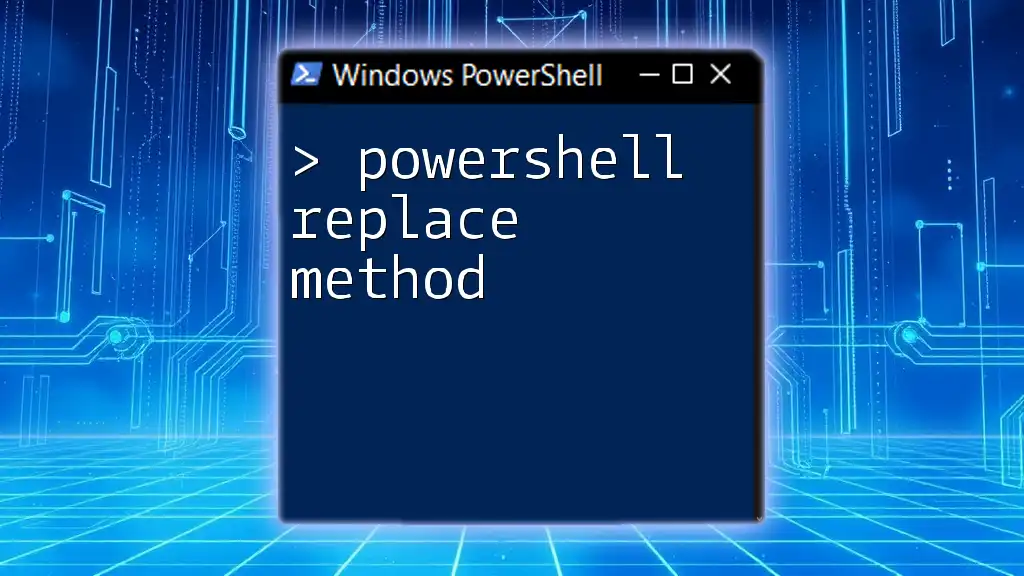
Conclusion
In summary, mastering how to PowerShell replace multiple strings is critical for efficient automation and text manipulation. By leveraging operators like `-replace`, along with hash tables and regular expressions, you can streamline your PowerShell scripts and improve your overall productivity. Practice using these techniques, experiment with examples, and you'll quickly become adept at handling strings in PowerShell.
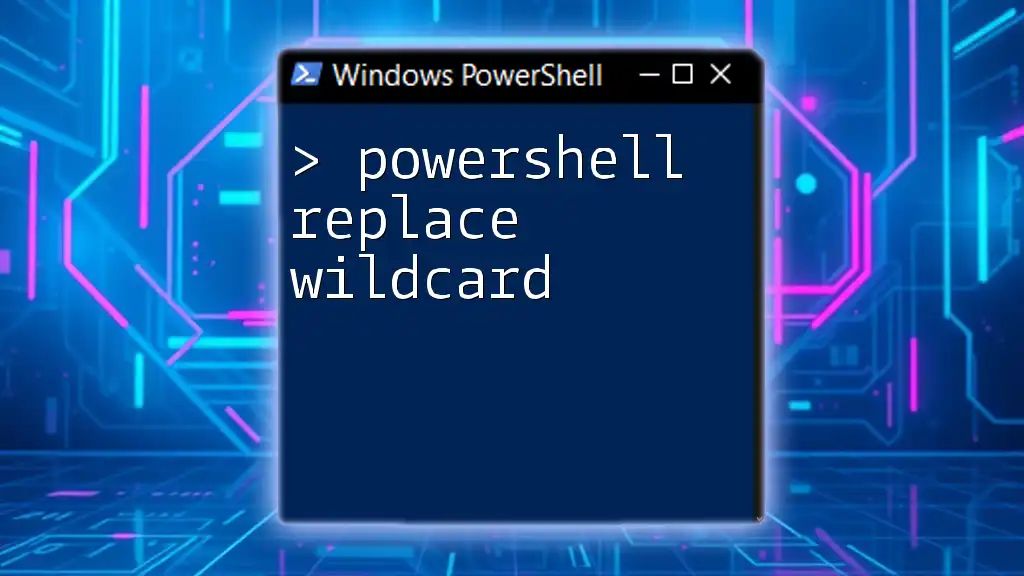
Additional Resources
Learn More About PowerShell
To further enhance your understanding of PowerShell string manipulation and replace techniques, consider exploring Microsoft's official documentation and other educational resources, including books, online courses, and video tutorials dedicated to PowerShell programming.