To remove multiple files in PowerShell, you can use the `Remove-Item` cmdlet with a wildcard or a list of file names.
Here's a code snippet to illustrate:
Remove-Item 'C:\Path\To\Your\Files\*.txt', 'C:\Path\To\Your\Files\file1.txt', 'C:\Path\To\Your\Files\file2.txt'
Explanation of PowerShell's Capabilities
PowerShell is a powerful command-line tool that enables you to perform a multitude of tasks in Windows environments. It allows users to automate processes, manage system configurations, and perform advanced file management tasks. For anyone serious about their productivity or IT operations, learning to use PowerShell effectively is a significant asset.

What is the `Remove-Item` Cmdlet?
The `Remove-Item` cmdlet in PowerShell plays a crucial role in file management. This command is primarily used to delete files or directories, allowing users to clear out unnecessary data quickly. While `Remove-Item` is excellent for file deletions, it's important to know that it is not the only command available. Understanding when to use `Remove-Item` versus other commands, such as `Clear-Content` or `Stop-Process`, is essential for effective system management.
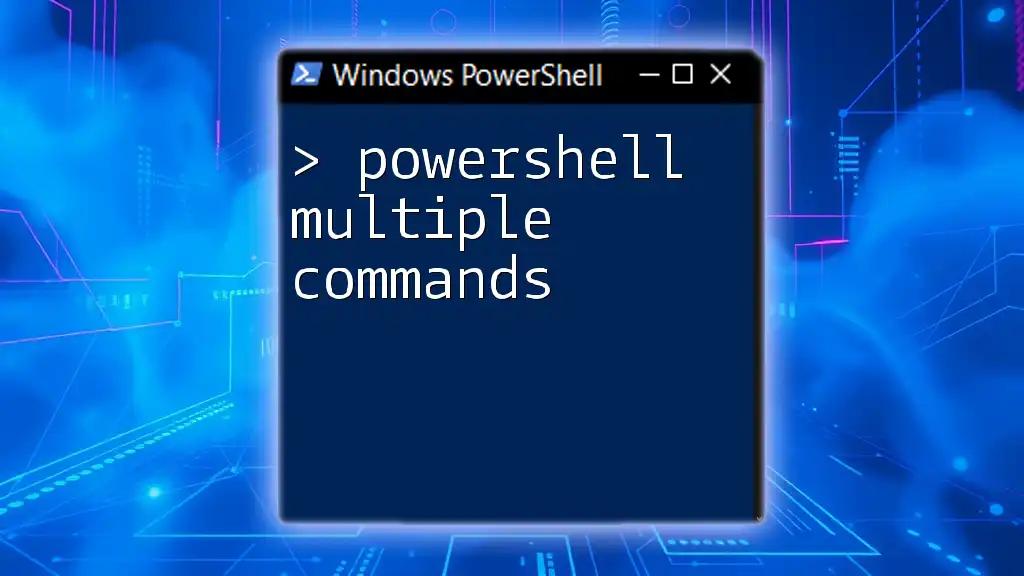
The Basics of File Removal in PowerShell
Understanding File Paths
When working with PowerShell, comprehending the difference between absolute and relative paths is fundamental. An absolute path specifies the location of a file or directory within the entire file system, starting from the root directory (e.g., `C:\Users\Username\Documents\file.txt`). In contrast, a relative path begins from the directory you are currently in. For instance, if you are already in the `Documents` directory, you can simply reference the file as `file.txt`.
Syntax of the `Remove-Item` Cmdlet
Learning the syntax for `Remove-Item` is the first step toward effective file deletion. The general structure of the command is as follows:
Remove-Item -Path 'C:\path\to\your\file.txt'
This command effectively tells PowerShell to delete the specified file.

Removing Multiple Files
Using Wildcards
Wildcards offer a powerful way to manage multiple files simultaneously. The two main wildcards used in PowerShell are `*` (which represents zero or more characters) and `?` (which represents a single character).
For example, if you want to remove all `.txt` files within a specific directory, your command would look like this:
Remove-Item -Path 'C:\path\to\your\*.txt'
This command will delete every file ending with `.txt` in the target directory. It’s a quick way to declutter without needing to specify each file individually.
Specifying Multiple Files with Commas
Another straightforward approach to removing multiple files involves direct specification. You can list the files you want to delete within the same command, separated by commas:
Remove-Item -Path 'C:\path\to\your\file1.txt', 'C:\path\to\your\file2.txt'
This method is clear and allows you to target specific files directly, which is particularly useful when their names do not share a common pattern.
Using Arrays to Remove Files
For more complex scenarios, creating an array of files provides a structured method of targeting multiple items. Arrays can be especially handy when needing to remove a larger number of files. Here’s an example of how to create an array and use it with `Remove-Item`:
$files = @('C:\path\to\your\file1.txt', 'C:\path\to\your\file2.txt')
Remove-Item -Path $files
This code snippet allows for easier management and organization of your file deletions.
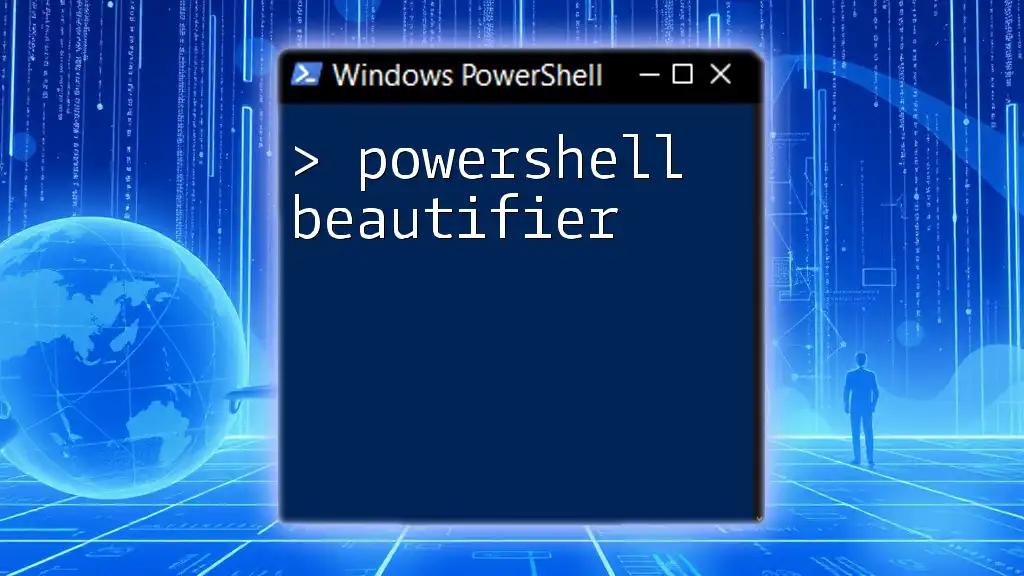
Best Practices for Removing Files
Testing Commands with `-WhatIf`
Before executing potentially destructive commands, using the `-WhatIf` parameter is a best practice. This acts as a safeguard, showing you what would happen if the command were actually run, without making any changes. Here's an example:
Remove-Item -Path 'C:\path\to\your\*.txt' -WhatIf
When you run this command, PowerShell returns a message detailing which files would be deleted, allowing you to confirm that the action is correct before proceeding.
Confirming Deletions with `-Confirm`
The `-Confirm` switch adds an extra layer of protection by prompting for confirmation before executing the deletion. This feature is particularly useful in production environments where mistakes can be costly. For example:
Remove-Item -Path 'C:\path\to\your\file.txt' -Confirm
By using this command, PowerShell will ask you to verify your intention to delete the specified file.
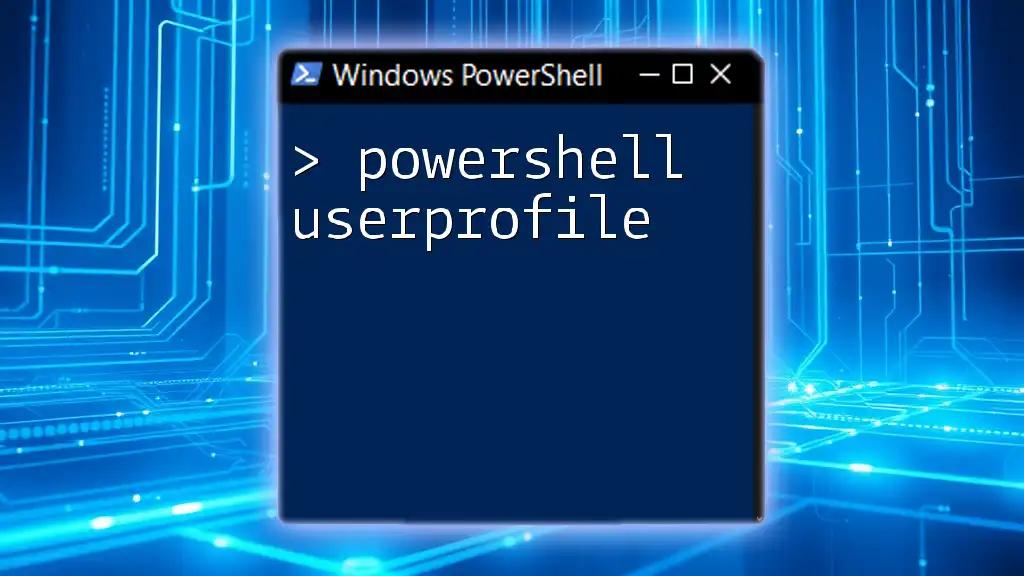
Handling Errors and Unexpected Outcomes
Common Errors with `Remove-Item`
When using `Remove-Item`, users may encounter common errors. One of the most frequent is a permission issue, where PowerShell does not have the necessary rights to delete the file. In such cases, running PowerShell as an administrator may resolve the problem.
Another common error is the "file path not found" message. This generally occurs if the specified file does not exist. Double-checking the file’s existence and the accuracy of the path can help mitigate this issue.
Logging Deletions
For accountability and tracking, keeping a log of file deletions can be beneficial. This is especially important in environments where file deletions must be audited. You can log deleted files as follows:
$deletedFiles = @()
$filesToRemove = @('C:\path\to\your\file1.txt', 'C:\path\to\your\file2.txt')
foreach ($file in $filesToRemove) {
Remove-Item -Path $file -ErrorAction SilentlyContinue
$deletedFiles += $file
}
$deletedFiles | Out-File -FilePath 'C:\path\to\your\deletion-log.txt'
This script removes the specified files while keeping a record of what was deleted, which can be output to a log file for future reference.
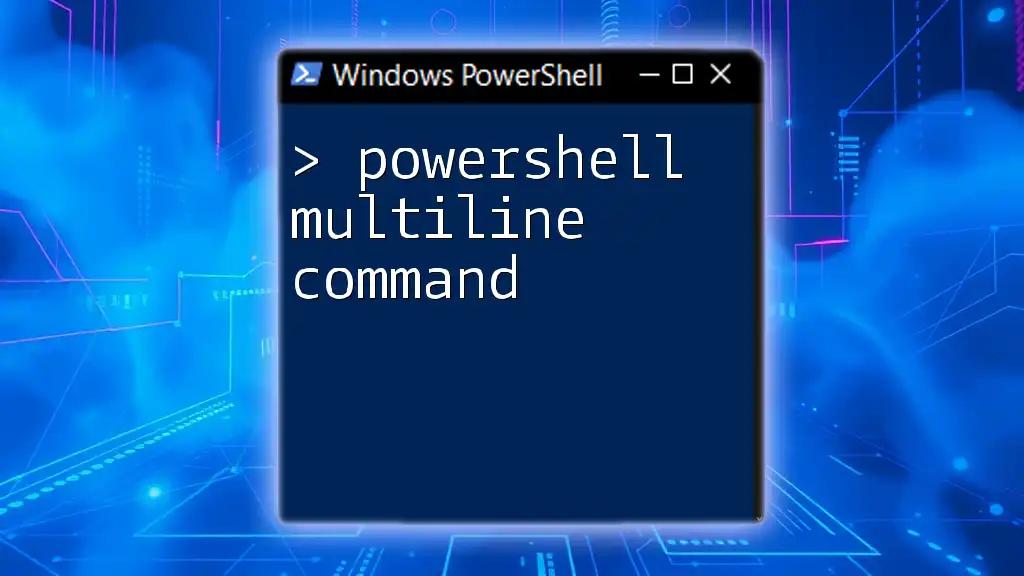
Conclusion
Using PowerShell to rm multiple files offers tremendous flexibility and efficiency in file management. By mastering the `Remove-Item` cmdlet and its various options—like wildcards, direct listing, and arrays—users can perform file deletions quickly without compromising safety.
Encouragingly, practicing these commands in a controlled setting allows you to become more comfortable with PowerShell and enhances your productivity. As you continue your journey, explore additional PowerShell resources to deepen your understanding of this powerful tool.