A PowerShell multidimensional array is an array that allows you to store data in a grid-like format, enabling you to access elements using multiple indices.
Here's a code snippet to define and access a multidimensional array in PowerShell:
# Create a 2x2 multidimensional array
$array = @(
@(1, 2),
@(3, 4)
)
# Access an element (row 1, column 0)
Write-Host $array[1, 0] # Output: 3
Introduction to Multidimensional Arrays in PowerShell
In PowerShell, an array is a data structure that holds multiple values in a single variable, allowing for efficient organization and manipulation of data. When dealing with complex datasets, you might find yourself needing a multidimensional array. This type of array enables you to store and access data in a grid-like format, which is particularly useful when working with tabular data or mathematical matrices.
What is an array?
Arrays are fundamental in programming and are used to group related data. In PowerShell, arrays can be one-dimensional, where you have a list of values, or multidimensional, where you can access data using multiple indexes.
Understanding multidimensional arrays
A multidimensional array is an array that has more than one dimension, typically referred to as rows and columns. This structure allows you to create data representations that resemble tables, which can be easily navigated and manipulated.
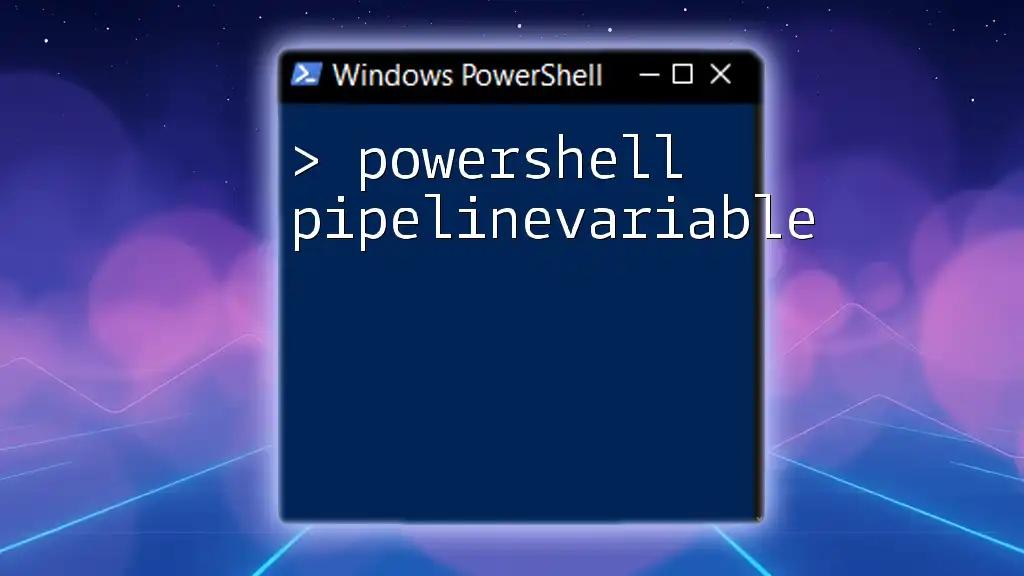
Creating Multidimensional Arrays in PowerShell
Declaring a Multidimensional Array
To declare a multidimensional array in PowerShell, you utilize a specific syntax that involves the `@` symbol. Here's how you can create an empty multidimensional array:
$myArray = @(@(), @())
In this example, the outer `@()` indicates that you are creating an array, and the inner `@()` signifies that it will hold additional arrays. This structure sets up the foundation for a two-dimensional array.
Populating a Multidimensional Array
Populating your multidimensional array is straightforward. By using two index numbers, you can specify which element you want to set a value for. Here’s how to do that:
$myArray[0, 0] = "First"
$myArray[0, 1] = "Second"
In this case, `0, 0` refers to the first row and first column, while `0, 1` refers to the first row and second column. This indexing system allows for flexible data management.
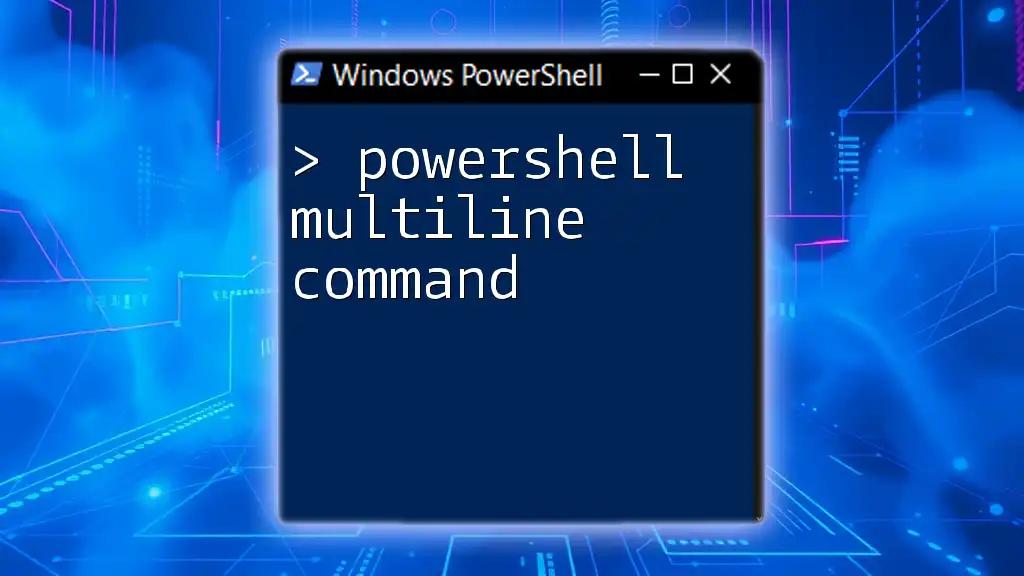
Accessing Elements of a Multidimensional Array
Using Indexes to Retrieve Data
Retrieving data from a multidimensional array is done similarly to how you populated it. By using the same index structure, you can access the information stored in those positions:
$firstElement = $myArray[0, 0]
Here, `$firstElement` will hold the value `"First"`.
Looping Through a Multidimensional Array
To effectively access and manipulate all elements in a multidimensional array, you may need to loop through its contents. Here's a nested loop that displays all the elements:
for ($i = 0; $i -lt 2; $i++) {
for ($j = 0; $j -lt 2; $j++) {
Write-Output $myArray[$i, $j]
}
}
This code iterates over each row and then each column, printing out all the elements in the multidimensional array. Understanding nested loops is critical for efficiently managing more complex datasets.
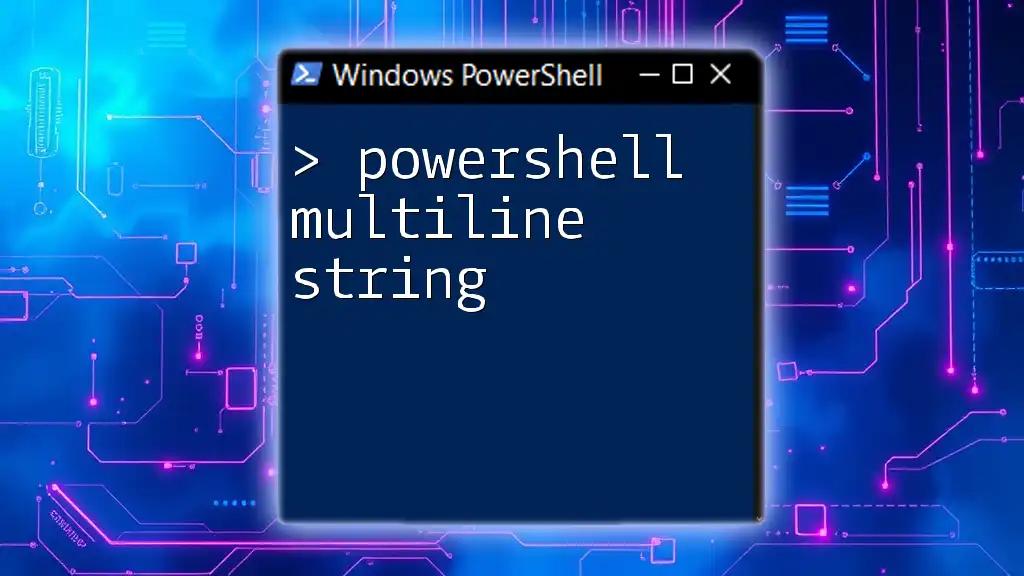
Common Use Cases for Multidimensional Arrays
Storing Tabular Data
Multidimensional arrays excel at representing data in tabular format. For instance, you can set up a simple data structure to hold headings and datasets like this:
$tableData = @(@("Name", "Age"), @("Alice", 30), @("Bob", 25))
In this array, the first row contains the headers, while subsequent rows contain the data entries. This approach makes it easy to manage datasets that resemble tables.
Matrix Operations
Mathematical matrices can also be represented using multidimensional arrays in PowerShell. Such structures enable operations like matrix multiplication or addition.
For example, consider an addition operation on two 2x2 matrices represented as multidimensional arrays. In a complete example, you would first populate two arrays and then write logic to perform the addition for corresponding elements.
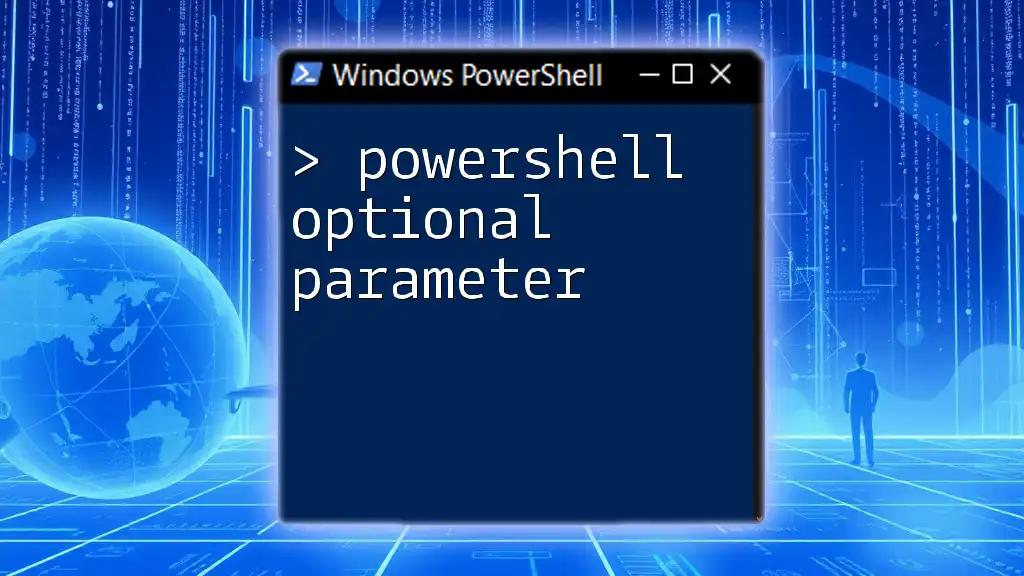
Modifying Elements in a Multidimensional Array
Updating Values
The process of updating values within a multidimensional array is as simple as assigning new data to a specific index. For instance:
$myArray[1, 0] = "UpdatedValue"
This command changes the second row and first column's value to `"UpdatedValue"`. Always ensure that the indices specified are within the array's bounds to avoid errors.
Adding New Dimensions
You may need to expand your multidimensional array by adding new rows or columns as your dataset grows. This can be accomplished dynamically, but it’s essential to remember that PowerShell arrays have a fixed size once declared. Hence, if you're expanding your array, you may want to create a new multidimensional array and copy existing data.

Practical Examples and Scenarios
Building a Simple Contact List
Creating a contact list is a practical way to utilize multidimensional arrays. Below is an example of a simple representation of this use case:
$contacts = @(@("Name", "Phone"), @("John", "1234"), @("Jane", "5678"))
The first row represents the column headings, while subsequent rows hold the data for each contact. You can also loop through this array to display or modify contact information easily.
Creating a Simple Game Scoreboard
Another illustrative use case involves keeping track of game scores. Here’s how you could structure a basic scoreboard:
$scores = @(@("Player", "Score"), @("Alice", 50), @("Bob", 75))
You can then access each player’s score via their respective indexes and easily update scores as the game progresses.

Troubleshooting and Best Practices
Common Errors and Solutions
While working with multidimensional arrays, you may encounter common pitfalls such as index out of range errors, which occur when accessing an index that does not exist. Always validate the dimensions of your array before referring to specific indices.
Best Practices for Management
When working with large datasets, ensure you manage memory effectively by evaluating when to use multidimensional arrays versus other data structures. Additionally, always comment your code for clear navigation and understanding, which is paramount for future modifications or debugging.
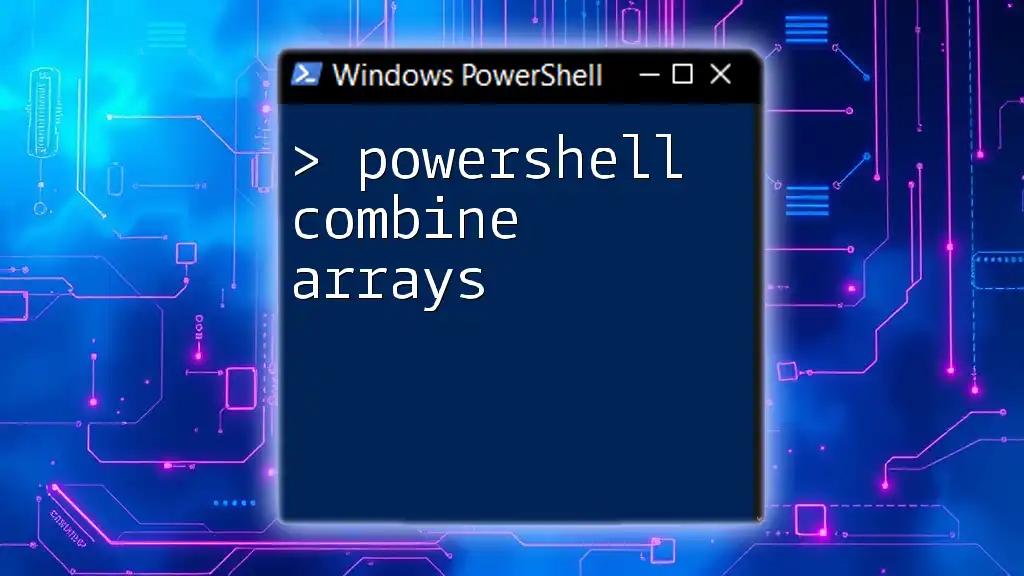
Conclusion
In summary, the `powershell multidimensional array` is a versatile tool for structuring complex datasets adeptly. Mastering the creation, manipulation, and retrieval of data from multidimensional arrays will enhance your PowerShell scripting capabilities significantly. Practice regularly to solidify these concepts, ensuring you become proficient in handling multidimensional arrays with ease.