In PowerShell, you can execute multiple commands in a single line by separating them with a semicolon, allowing for efficient scripting and automation.
Write-Host 'Hello, World!'; Get-Process; Get-Service
Understanding PowerShell Commands
PowerShell commands are the building blocks of the PowerShell environment. At their core, they are known as cmdlets, which follow a specific syntax and are designed to perform specific tasks. Each cmdlet consists of a verb and a noun that describes what action it performs and on what object, respectively.
For example, `Get-Process` retrieves a list of currently running processes, illustrating the straightforward naming convention in PowerShell. This clear structure makes it easy to understand and utilize commands effectively, especially when combining them.
When executing multiple commands, understanding how they interact is essential. By learning to run commands sequentially or in combination, you can automate tasks and streamline administrative processes.
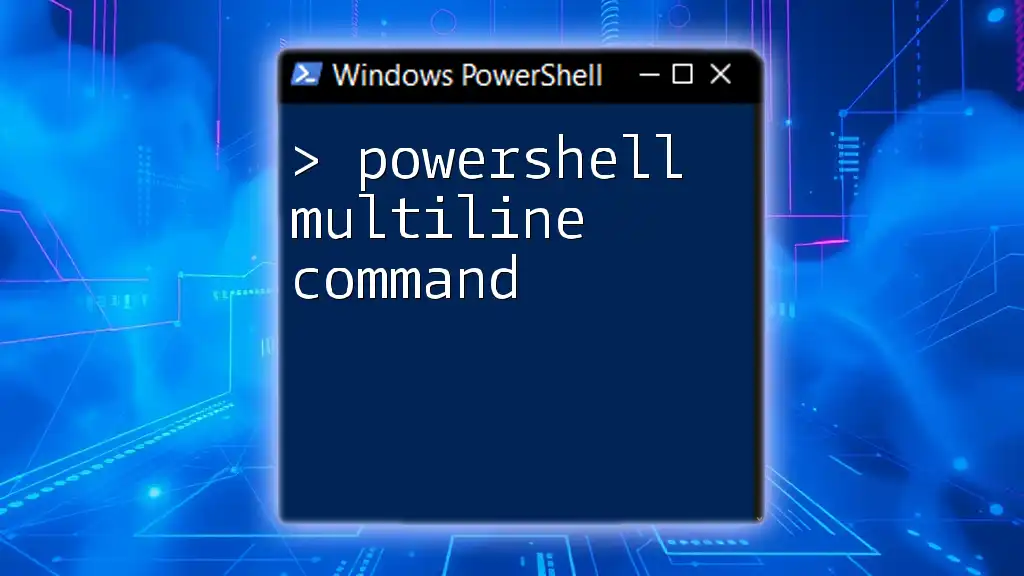
Running Multiple Commands in PowerShell
Running multiple commands in PowerShell is crucial for improving efficiency and automating workflows. Executing commands collectively can save significant time, especially for repetitive tasks that may involve multiple steps.
Chain PowerShell Commands
Chaining commands allows you to execute several instructions in one line instead of running them separately. There are specific operators commonly used for this purpose:
- Semicolon (`;`): This operator separates commands and allows them to be executed in sequence. Each command will run independently of the others.
- Pipeline (`|`): This operator allows you to pass the output of one command directly into the next, creating a seamless flow of information.
Example: Here’s how to use the semicolon to chain two simple commands:
Get-Process; Get-Service
In this case, `Get-Process` retrieves running processes while `Get-Service` lists available services, each executed in succession.
PowerShell Multiple Commands on One Line
Executing multiple commands in a single line can greatly enhance productivity. It enables you to run concise commands quickly.
To execute commands on one line, you can separate them using a semicolon. This method allows for rapid execution without needing to run each command individually.
Example:
Get-Process; Stop-Process -Name "notepad"
In this example, `Get-Process` runs first to list running processes, followed by `Stop-Process` to terminate the Notepad application if it's running.
Run Multiple PowerShell Commands Sequentially
Sequential execution is a powerful feature in PowerShell, allowing you to run commands in specific order, particularly when the output of one command influences the next.
You can also use parentheses to group commands when desired. This adds an extra layer of control over the execution sequence.
Example:
(Get-EventLog -LogName Application); (Get-EventLog -LogName System)
Here, the commands are executed one after the other, with the results of the Application log appearing before those of the System log.
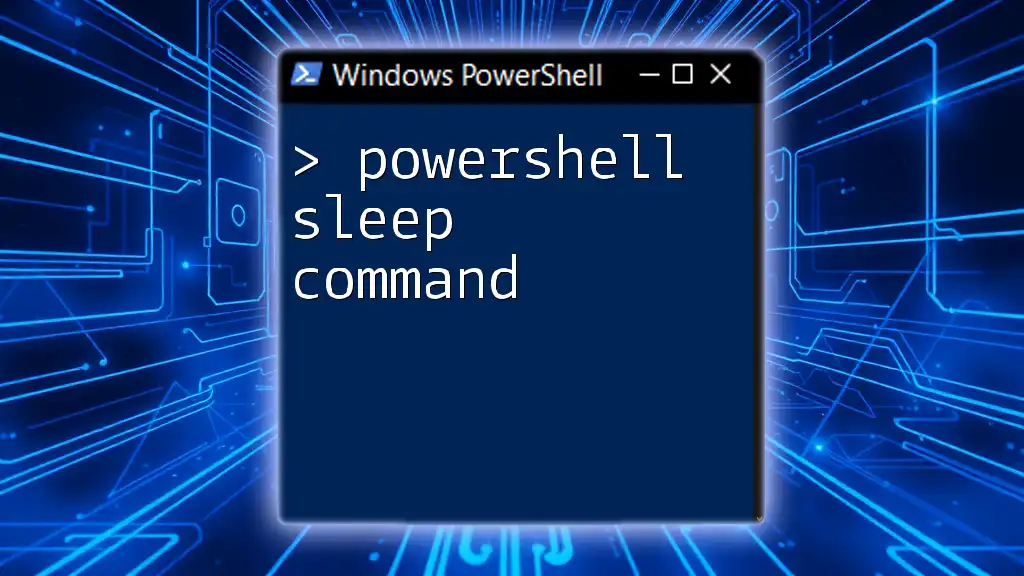
Running Two Commands Together
There are circumstances where you may want to run two commands simultaneously to enhance performance. In these cases, the `Start-Job` cmdlet is particularly effective.
This cmdlet allows you to execute commands as background jobs, meaning they won’t block the terminal while they run.
Example:
Start-Job -ScriptBlock { Get-Process }; Start-Job -ScriptBlock { Get-Service }
In this scenario, both `Get-Process` and `Get-Service` are executed in parallel, allowing you to retrieve data from both commands simultaneously.
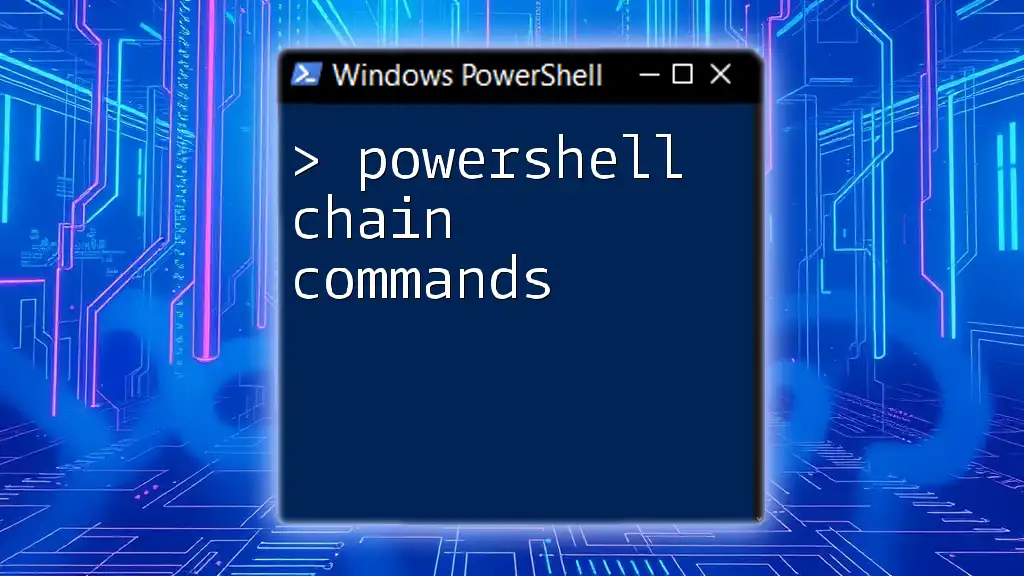
Advanced Techniques
For more complex requirements, PowerShell provides advanced features like `Invoke-Command`, which allows for remote command execution. This is especially useful when managing multiple systems.
Example: Here’s how to run multiple commands on a remote machine:
Invoke-Command -ComputerName Server01 -ScriptBlock { Get-Process; Get-Service }
This command retrieves the list of processes and services running on `Server01`. It’s an effective way to manage several machines without needing to log into them individually.
Error handling is another essential aspect when executing multiple commands. Using `try-catch` blocks can help you manage errors gracefully.
Example:
try {
Get-Process; Stop-Process -Name "nonexistentProcess"
} catch {
Write-Host "An error occurred: $_"
}
In this example, if the command to stop a nonexistent process fails, the error will be caught, allowing you to display a meaningful message without terminating the script.
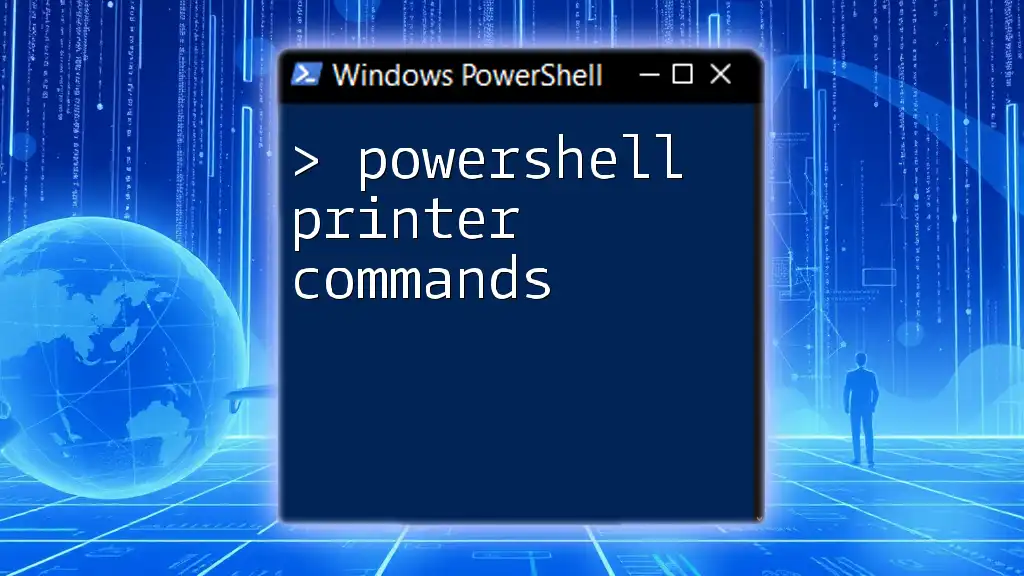
Best Practices for Running Multiple Commands
To maximize your efficiency with multiple commands, consider the following best practices:
-
Readability vs. Compactness: While it’s tempting to use one-liners, maintaining readability is crucial, especially for complex scripts. Well-structured commands are easier to understand and maintain.
-
Testing Individually: It's often wise to test commands independently before chaining them to ensure each one performs as expected.
-
Documentation: Whenever you create complex scripts with multiple commands, document what each section of the script does. This will be invaluable for future reference and for others who may review your work.
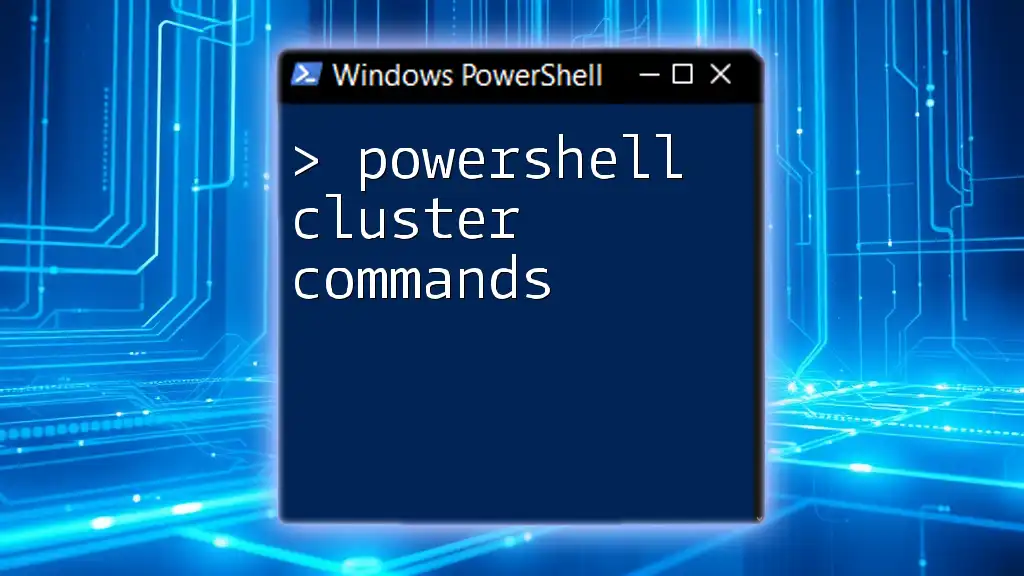
Conclusion
Mastering PowerShell multiple commands capabilities significantly impacts your productivity and automation potential. Whether you’re chaining commands, running them in parallel, or employing advanced techniques like remote execution, the ability to execute multiple commands effectively transforms how you approach PowerShell scripting and system management.
By practicing the techniques discussed in this guide, you can harness the full power of PowerShell to streamline operations and enhance efficiency in your daily tasks or within your organization.