PowerShell chain commands allow you to execute multiple commands in a single line by using semicolons to separate them, enabling more efficient scripting and automation.
Write-Host 'Hello, World!'; Get-Date; Get-Process
Understanding PowerShell Chaining Commands
What is Chaining in PowerShell?
Chaining commands in PowerShell allows you to string together multiple commands into a single line of code. This practice not only streamlines your workflow but also enhances your scripting capabilities. When commands are chained, the outcome of one command can influence the execution of subsequent commands, providing an intuitive approach to manage complex tasks.
Why Chain PowerShell Commands?
Chaining commands can drastically improve efficiency by reducing the time it takes to execute a series of tasks. Instead of running each command individually and waiting for each to complete, chaining enables you to execute multiple commands in a single go. Moreover, this practice leads to greater readability of scripts. A well-structured chain reflects the logical flow of tasks being executed, making scripts easier to understand for yourself and others.
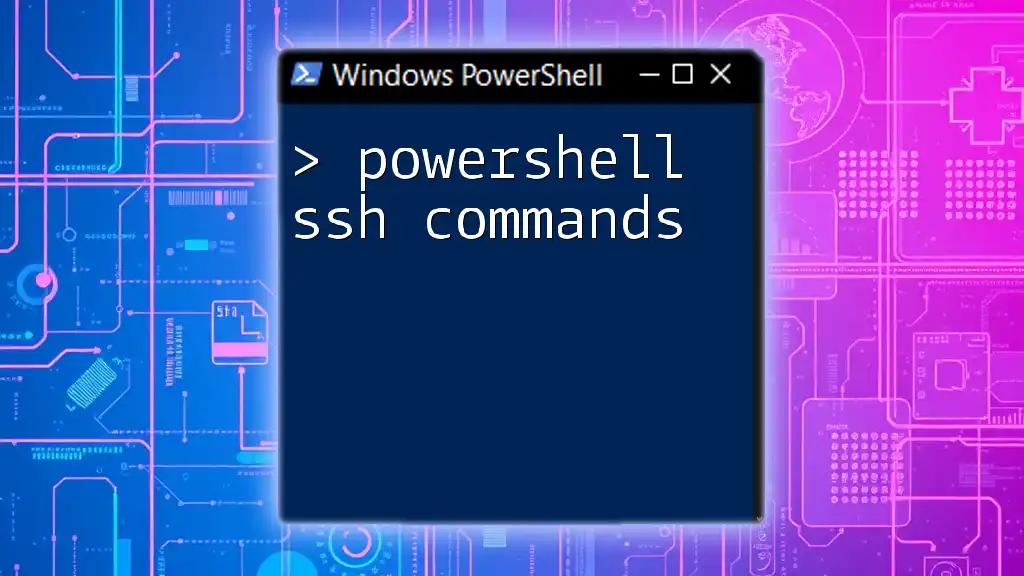
Basic Techniques for Chaining Commands
Using the Semicolon (;)
The semicolon (`;`) is the most basic form of command separation in PowerShell. By placing a semicolon between commands, you can execute them sequentially.
Example:
Get-Process; Get-Service
In this example, `Get-Process` retrieves all active processes, and `Get-Service` fetches the status of services. Both commands execute one after the other, but they do not depend on the success of each other.
Discussion: Similar to using a period in a sentence, semicolons should be used to link related thoughts (commands in this case). Using them judiciously can lead to smoother, more structured scripts.
The Use of `&&` Operator
The `&&` operator chains commands such that the next command is executed only if the previous command succeeds. This is particularly useful for conditional workflows.
Example:
Test-Path "C:\Test" && Write-Host "Path exists"
In this case, `Write-Host` will only run if `Test-Path` returns true (i.e., the specified path exists).
Discussion: The `&&` operator is excellent for scenarios where subsequent actions depend on the successful execution of the previous command, thereby creating a flow that mimics logical reasoning in day-to-day tasks.
The Use of `||` Operator
Conversely, the `||` operator executes the next command only if the previous one fails. This allows for robust error handling in scripts.
Example:
Test-Path "C:\NonExistent" || Write-Host "Path not found"
Here, the message is printed only if `Test-Path` fails, which is helpful for notifying users about issues.
Discussion: Using `||` can enhance the resilience of scripts, allowing you to provide feedback on failures without halting the execution of other independent commands.
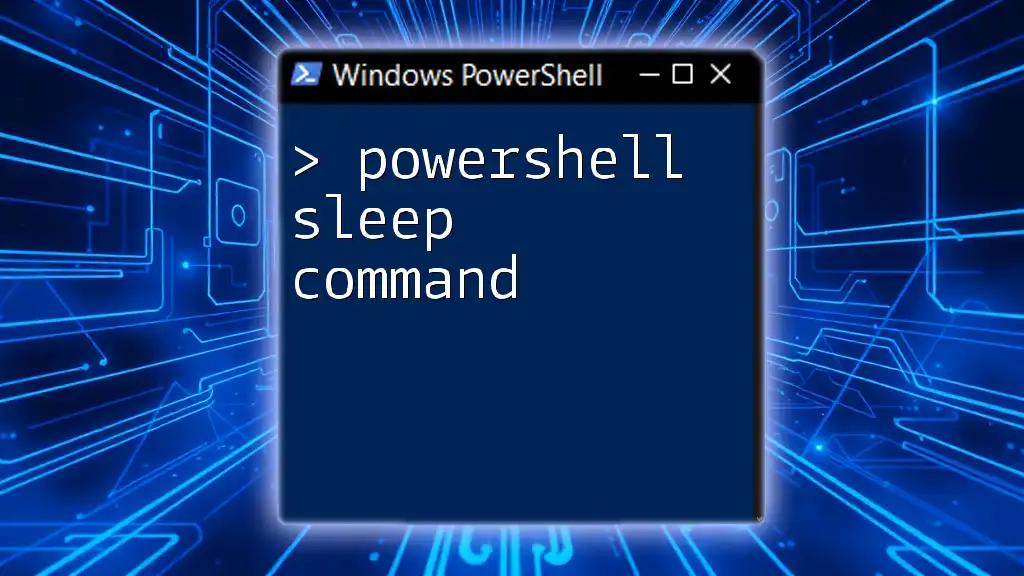
Advanced Techniques for Chaining Commands
Combining Pipelines with Chained Commands
One of the most powerful features of PowerShell is the ability to combine pipelines with chained commands. The pipeline operator (`|`) allows you to send the output from one command directly into another command as input.
Example:
Get-Process | Where-Object { $_.CPU -gt 100 }
In this case, you’re fetching processes and filtering those that utilize more than 100 CPU cycles. The use of `Where-Object` helps narrow down results efficiently.
Discussion: This chaining mechanism is especially crucial for transforming data on-the-fly, allowing you to conduct complex queries with minimal effort.
Chaining with Conditional Statements
You can also integrate conditional logic directly within your chains using if-statements, enhancing your scripts' control flow.
Example:
if (Test-Path "C:\Test") { Remove-Item "C:\Test" } else { New-Item "C:\Test" }
In this code, if the path exists, it gets removed; otherwise, a new item is created. This demonstrates how chaining can lead to concise yet powerful scripts.
Discussion: Using conditional statements effectively allows for dynamic scripting that adjusts to different scenarios based on user interaction or system state.
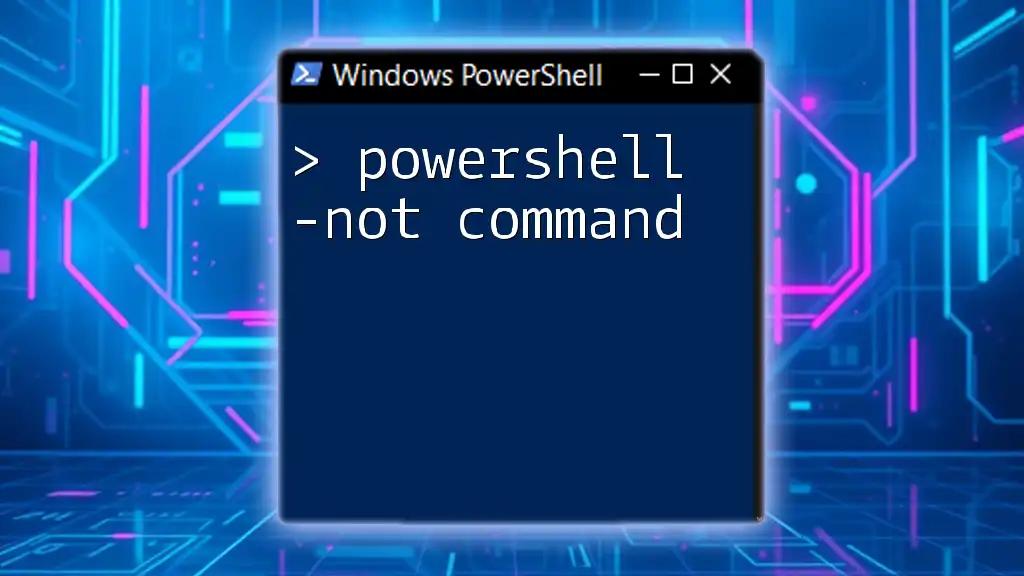
Real-World Applications of PowerShell Command Chaining
Automating Daily Tasks
PowerShell chaining can significantly automate repetitive daily tasks. For instance, you could create a script that performs backups, cleans temporary files, and logs operations—all chained into one cohesive action.
Example:
Copy-Item "C:\Source\*" -Destination "D:\Backup\"; Remove-Item "C:\Source\*" -Recurse; Write-Host "Backup completed and source cleared."
This chain first copies all files, removes the originals, and then logs a message, achieving multiple objectives seamlessly.
Discussion: Automation not only saves time but also reduces human error, paving the way for efficient system management.
System Monitoring Scripts
Chaining commands can create effective monitoring scripts that provide real-time insights into system performance.
Example:
Get-Service | Where-Object { $_.Status -ne 'Running' } | ForEach-Object { Write-Host "Service down: $($_.Name)" }
This command checks all services and outputs the ones that aren't running, providing a quick health check for the system.
Discussion: Monitoring scripts can be scheduled to run periodically, contributing to ongoing system health checks and proactive problem resolution.
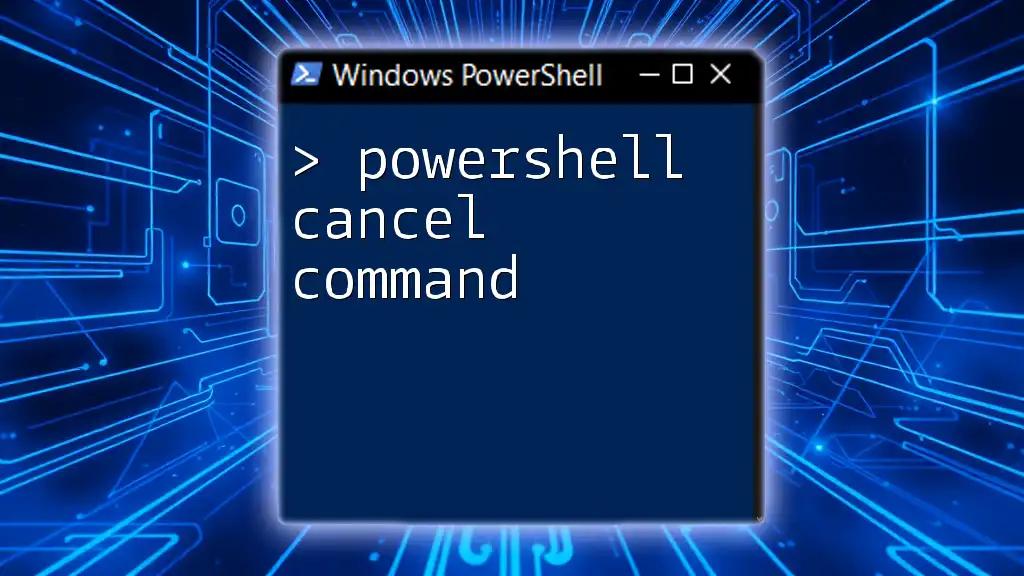
Best Practices for Chaining PowerShell Commands
Prioritizing Readability
When chaining commands, it’s important to maintain readability. Long chains can quickly become unwieldy and confusing.
Example:
Get-Service | Where-Object { $_.Status -eq 'Running' } | ForEach-Object { $_.Name }
This chain remains clear and concise while still performing multiple tasks.
Tip: Keep chains short and simple. Break complex logic into functions or separate scripts if necessary to enhance maintainability.
Error Handling Strategies
Error handling is critical in scripting. Always anticipate potential failures and implement error handling.
Example:
try {
Get-Content "C:\file.txt" || throw "File not found"
Write-Host "File read successfully"
} catch {
Write-Host $_.Exception.Message
}
Here, if the file fails to read, an error is caught and a message is displayed, preventing the script from crashing unexpectedly.
Discussion: Building robust scripts requires anticipating failure points. Proper error handling can provide valuable feedback and allow your scripts to recover gracefully from unexpected situations.
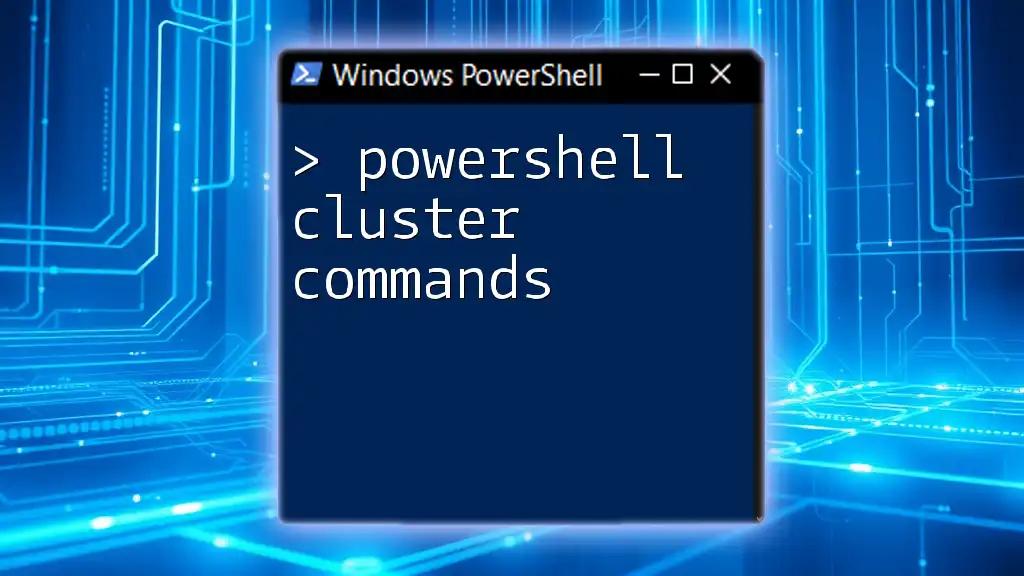
Troubleshooting Common Issues with Chained Commands
Common Errors and Fixes
Errors in chained commands often stem from syntax issues, incorrect assumptions about command availability, or misunderstanding command structure.
Ensure that each command is valid and the expected data types are passed appropriately. Using `Get-Help` with each command can clarify available options and expected outcomes.
Using Verbose and Debug Parameters
To further enhance your troubleshooting, you can use PowerShell’s built-in parameters like `-Verbose` and `-Debug` to gain insights into command execution.
Example:
Get-Service -Name 'wuauserv' -Verbose
With `-Verbose`, PowerShell provides additional information on what the command is doing behind the scenes, which can help diagnose issues more effectively.
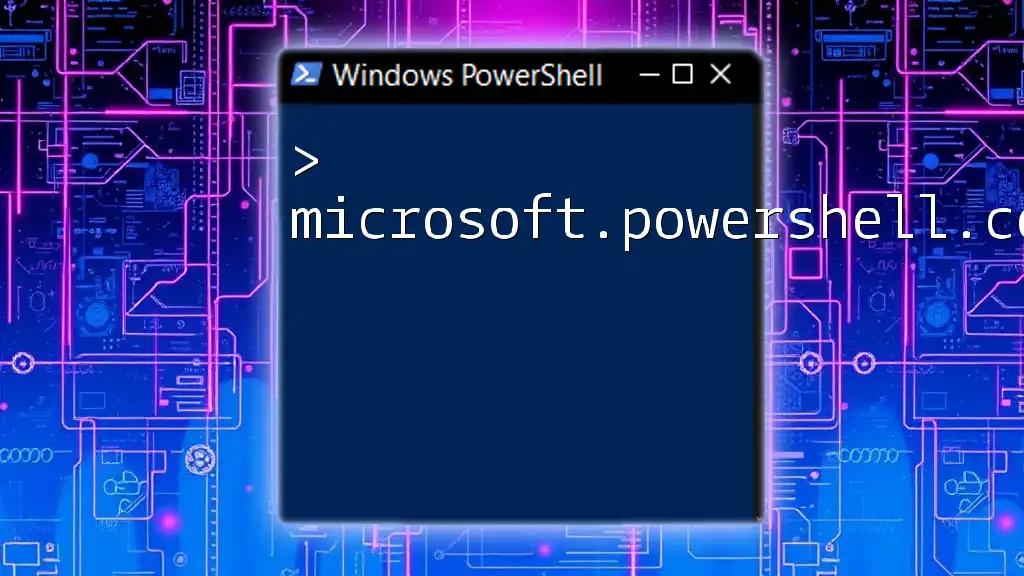
Conclusion
Mastering PowerShell chain commands is essential for any serious PowerShell user. Chaining allows for increased productivity and efficiency as you streamline workflows, automate tasks, and carry out complex functions with elegance and clarity. By embracing chaining and mastering proper techniques, you can unleash the full potential of PowerShell in your daily tasks and scripts. Remember, practice makes perfect—keep exploring and experimenting with different chaining techniques to unlock new capabilities in your automation toolkit.
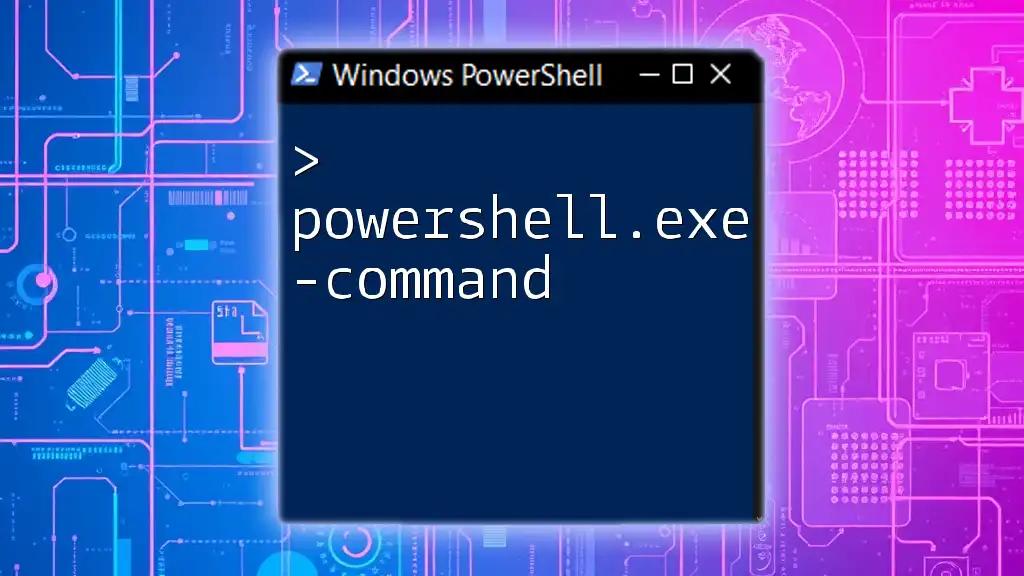
Additional Resources
For further learning, consider exploring the official Microsoft PowerShell documentation and various online tutorials that delve deeper into advanced features and best practices in PowerShell scripting. Engaging with the community through forums and social platforms can also accelerate your learning journey.