In PowerShell, you can execute two commands on one line by separating them with a semicolon, allowing for efficient scripting and command execution.
Write-Host 'Hello, World!'; Get-Date
Understanding PowerShell Command Structure
What is a PowerShell Command?
A PowerShell command is a structured instruction that directs the PowerShell engine to perform a specific task. It follows a verb-noun syntax, where the verb signifies the action to be performed and the noun indicates the target object of that action. For instance, the command `Get-Process` retrieves a list of currently running processes.
The Need for Multiple Commands
Combining multiple commands serves to enhance efficiency and streamline workflows in PowerShell. In many scenarios, executing a series of tasks in one line can significantly reduce the time and effort spent on repetitive tasks. For instance, rather than running separate commands to gather information, users can obtain the desired data in a single line, which saves time and simplifies the scripting process.
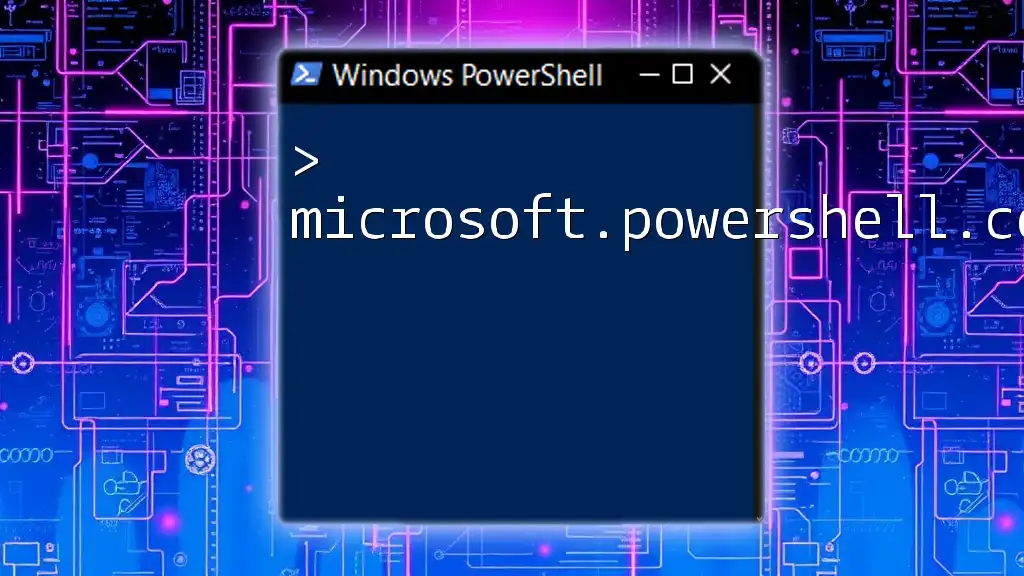
Methods to Run Multiple Commands in One Line
Using Semicolons
One of the simplest methods to execute PowerShell two commands one line is by utilizing semicolons (`;`) to separate commands. This allows the PowerShell engine to run each command sequentially, moving to the next command regardless of the outcome of the previous one.
Example Code Snippet:
Get-Process; Get-Service
In the snippet above, the `Get-Process` command retrieves a list of active processes, while `Get-Service` lists the services running on the system. The commands execute one after the other, making it a straightforward approach to gather information.
Utilizing Pipe (`|`) Operator
The pipe operator (`|`) is a powerful feature in PowerShell that allows you to send the output of one command directly as an input to another. This is particularly beneficial when you want to filter, manipulate, or format data from the preceding command.
Example Code Snippet:
Get-Process | Where-Object { $_.CPU -gt 100 }
In this example, `Get-Process` fetches all running processes, and the pipe operator forwards this list to `Where-Object`, which filters the processes to only those consuming more than 100 CPU units. This method effectively demonstrates how to achieve more complex operations in a single line.
Using the ForEach Command
The `ForEach` command can be employed to iterate over a collection of items and execute a command for each item. This is particularly useful when the output from one command involves multiple objects.
Example Code Snippet:
Get-Service | ForEach-Object { $_.Name }
Here, `Get-Service` retrieves all services, and `ForEach-Object` processes each object, outputting just the service names. This format not only condenses the operation into a single line but also provides a clear view of the specific information needed.
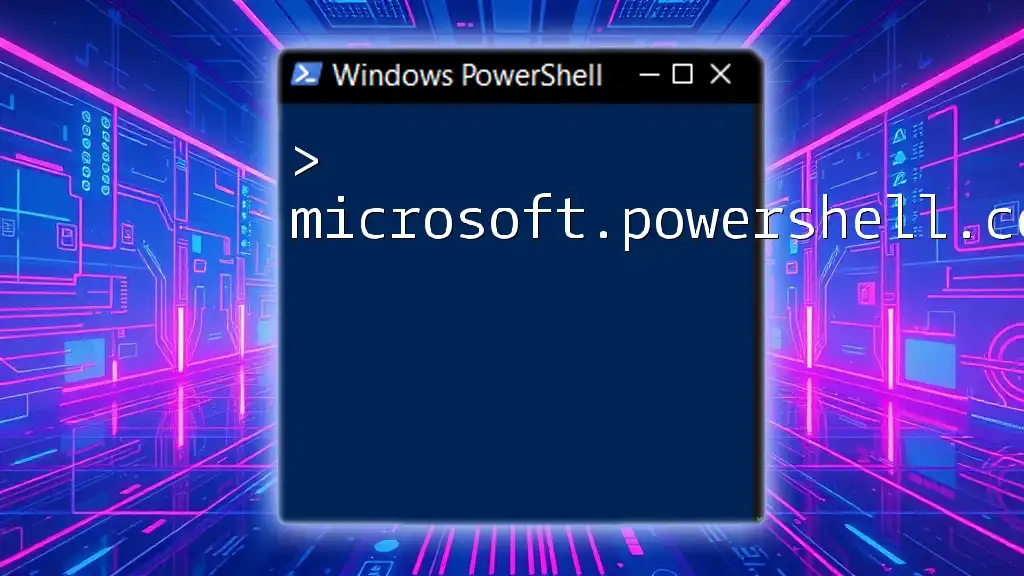
Combining Multiple Commands for Efficient Scripting
Chaining Commands
Chaining commands allows you to run multiple actions in sequence, creating a powerful workflow within a single line. Each command can pass its output directly to the next command, producing well-structured results.
Example Code Snippet:
Get-Process | Sort-Object CPU -Descending | Select-Object -First 5
In this line, `Get-Process` fetches the list of processes, `Sort-Object` arranges them by CPU usage in descending order, and `Select-Object` picks the top five processes. By chaining commands in this way, you can quickly derive insightful data, all within one concise expression.
Conditional Command Execution
Conditional commands allow for dynamic scripting where subsequent commands can depend on the success or failure of prior commands. It enhances script adaptability and reliability.
Example Code Snippet:
Get-Command -Name Get-Process; if ($?) { Write-Host "Command found!" }
In this line, we first check for the existence of the `Get-Process` command. If the command exists—indicated by `$?` being true—the script outputs "Command found!" This control structure is invaluable for ensuring commands only execute under appropriate conditions.
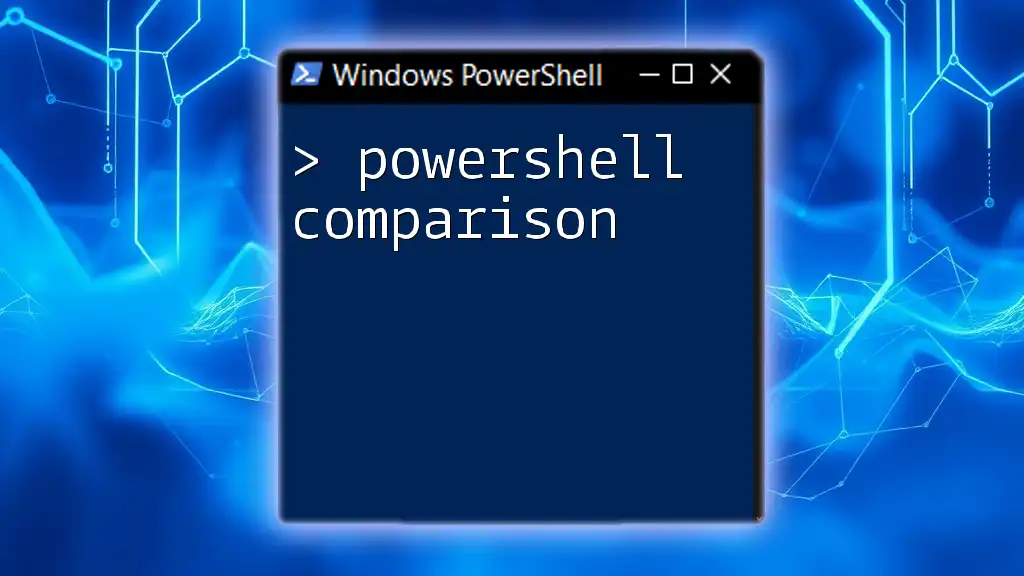
Best Practices for Using Multiple Commands on a Single Line
Readability vs. Conciseness
While it’s tempting to condense scripts into short lines, striking a balance between conciseness and readability is crucial. Excessive one-liners can become challenging to understand and maintain, especially for other users or yourself in the future. Always aim to write code that is clear and self-explanatory, allowing for easy modifications without extensive deciphering.
Error Handling in One-Liners
Implementing robust error handling in your one-liners is essential for creating reliable scripts. You need to anticipate potential errors and handle them gracefully, ensuring the script can continue operation or provide informative feedback.
Example Code Snippet:
Get-Process -Name NonExistentProcess -ErrorAction SilentlyContinue; if ($?) { "Process is running" } else { "Process not found" }
This line attempts to get a process that doesn’t exist. By using `-ErrorAction SilentlyContinue`, the command suppresses typically verbose error messages, allowing for a smoother user experience. The following conditional check provides feedback, confirming whether the process is found or not, allowing efficient error management within a single line.
Use Cases
Real-world applications of combining commands in PowerShell extend across various administrative and scripting tasks. For instance, system administrators often use PowerShell two commands one line to quickly check system health, such as fetching both running services and their statuses without needing multiple separate commands.
For another example, in data analysis, a series of commands can filter log files to extract only relevant entries while displaying counts or summaries instantly. The versatility of executing multiple commands in a single line greatly enhances productivity and effectiveness in performing system administrations.
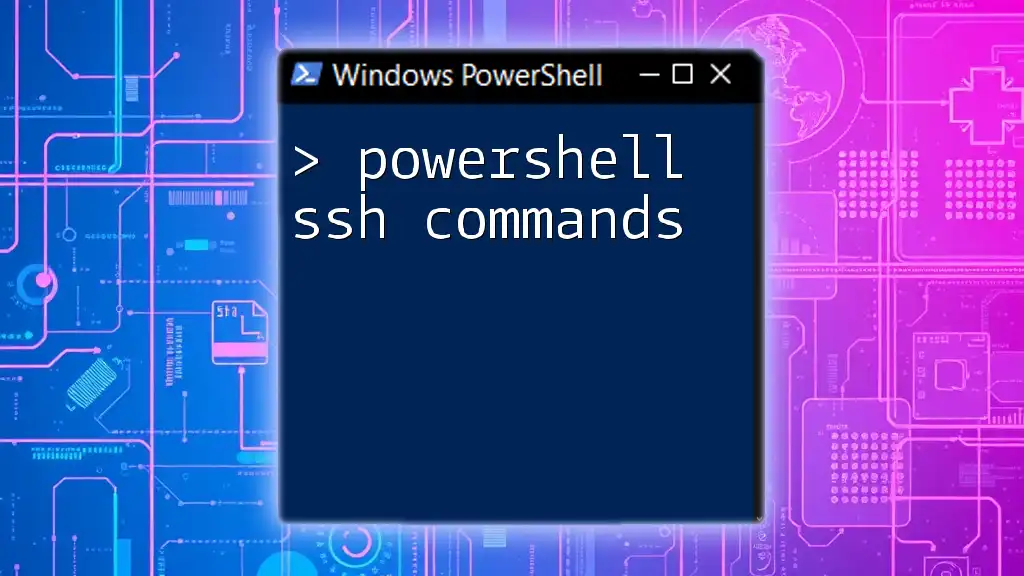
Conclusion
By mastering the techniques for executing PowerShell two commands one line, users can significantly improve efficiency and streamline their workflows. Practicing these methods allows you to explore the full power of PowerShell scripting, enabling complex tasks to be handled in concise and effective manners. Remember to prioritize readability and robustness in your scripts, which ultimately leads to better maintenance and adaptability for future tasks. Happy scripting!
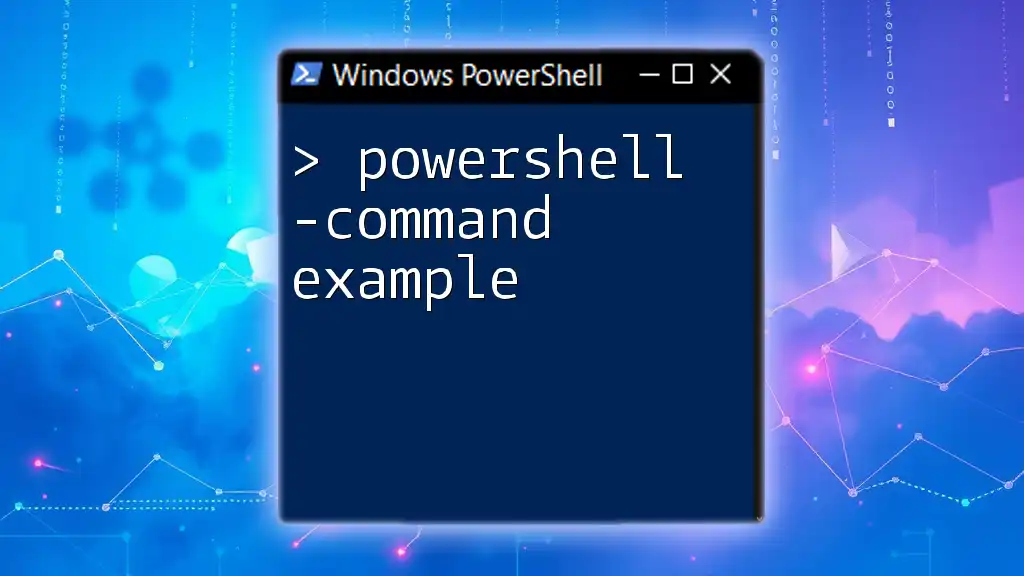
Additional Resources
To further enhance your PowerShell skills, consider exploring the official PowerShell documentation, taking structured online courses, or diving into recommended readings that provide more advanced insights and practical exercises. Engaging with the PowerShell community can also provide valuable tips and shared experiences to inform your scripting practices.