The PowerShell console log is a feature that allows users to capture and display output or error messages in the terminal, enhancing debugging and monitoring capabilities.
Here’s a simple code snippet to display a custom message in the console log:
Write-Host 'This is a PowerShell console log example!'
Understanding PowerShell Console
What is the PowerShell Console?
The PowerShell console is a command-line interface for executing PowerShell commands and scripts. Unlike PowerShell Integrated Scripting Environment (ISE), which provides a graphical interface, the console allows for direct interaction with scripts, making it an essential tool for system administrators and developers. It serves as the backbone for automating tasks and provides a platform for immediate feedback and testing.
Understanding the console’s functionality is crucial for effective script execution and debugging. Many advanced features of PowerShell can only be accessed through the console, making it a valuable resource for users looking to enhance their automation capabilities.
Benefits of Console Logging
Utilizing logging in the PowerShell console offers several advantages:
- Enhanced Tracking and Debugging: Console logs provide a traceable history of commands executed, allowing easier identification of issues when they arise.
- Monitoring Script Execution: Logging allows one to monitor the success or failure of tasks in real time, providing immediate feedback on performance.
- Historical Record: Keeping a record of commands and outputs can be invaluable for auditing and understanding past actions.

Getting Started with PowerShell Console Logs
Basic Commands for Console Logging
Understanding how to log information in the console is fundamental for any PowerShell user. The most straightforward commands for output include `Write-Host` and `Write-Error`.
How to Use `Write-Host` for Simple Output
`Write-Host` allows you to print messages directly to the console. This is useful for providing immediate feedback to the user. Here's a basic example:
Write-Host "This is an informational message."
In this example, the message "This is an informational message." will appear directly in the console, emphasizing the communication aspect of scripts.
Logging Errors with `Write-Error`
If something goes wrong, it’s essential to communicate that clearly. `Write-Error` serves that purpose by highlighting error messages. For instance:
Write-Error "This is an error message."
When executed, this command will display the error message prominently, alerting users to issues with greater visibility.
Redirecting Output to the Console
PowerShell recognizes various output streams, allowing you to handle standard outputs and errors effectively. You can use redirection operators to manage output efficiently.
Example: Capturing Output and Errors
Using the `Out-Host` cmdlet allows you to send output directly to the console while capturing errors might look like this:
Get-Process | Out-Host
Get-Process -Name NonExistentProcess 2>errorlog.txt
In this example, any error encountered while looking for a non-existent process will be redirected to `errorlog.txt`, while other outputs will be shown in the console.
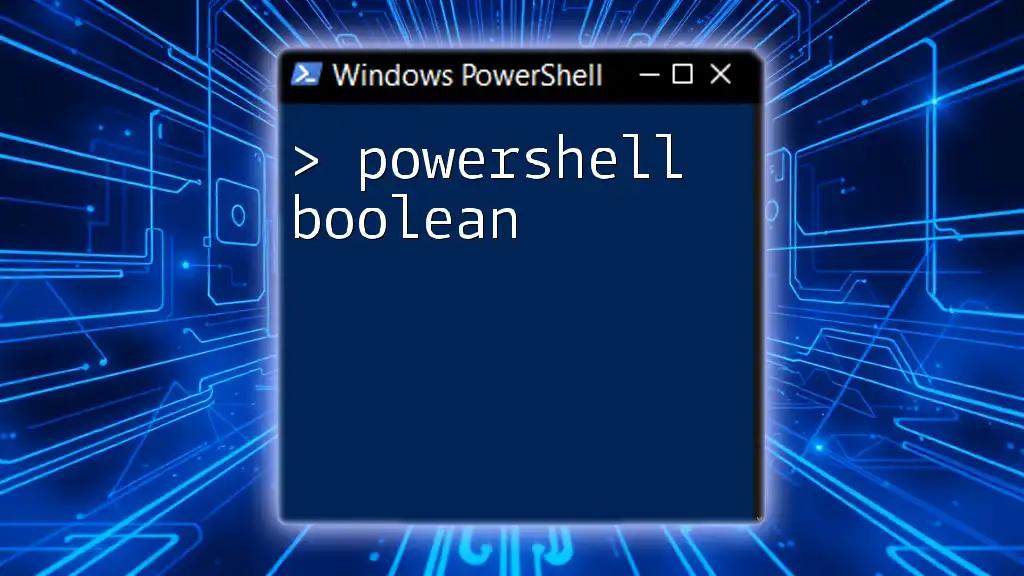
Advanced Console Logging Techniques
Using Transcript for Detailed Logging
Transcription is a powerful feature in PowerShell that records all output from the console session, providing a comprehensive view of activities.
What is a PowerShell Transcription?
A PowerShell transcript captures everything that's displayed on the console, including outputs and commands. This can be particularly useful for auditing and tracking actions.
How to Start and Stop a Transcript
Begin by using the `Start-Transcript` cmdlet and stop it using `Stop-Transcript`. Here’s how to implement it:
Start-Transcript -Path "C:\logs\sessionLog.txt"
# Your commands here
Stop-Transcript
When you run the above code, all subsequent commands and outputs are recorded in `sessionLog.txt` until you stop the transcription.
Custom Logging Functions
Creating Your Own Logging Function
Custom logging functions can enhance the flexibility and functionality of logging in PowerShell. By creating a dedicated function, you can tailor your logging needs to fit any scenario.
Step-by-Step Guide to Create a Logging Function
Here’s a simple example of how to create a logging function:
function Write-Log {
param (
[string]$Message,
[string]$LogFile = "C:\logs\customLog.txt"
)
$timestamp = Get-Date -Format "yyyy-MM-dd HH:mm:ss"
"$timestamp - $Message" | Out-File -FilePath $LogFile -Append
}
This function captures a log message along with a timestamp and appends it to the specified log file.
Usage Example:
Write-Log "This is a custom log entry."
When you execute this command, a new entry will appear in `customLog.txt`, allowing you to maintain a structured logging format.
Logging Severity Levels
Integrating severity levels into your logging can clarify the importance of each log entry.
Importance of Logging Levels
By categorizing logs into levels such as Info, Warning, and Error, you can prioritize your responses and streamline troubleshooting.
Modifying the Logging Function to Include Severity
Here’s how you could enhance the `Write-Log` function to incorporate severity levels:
function Write-Log {
param (
[string]$Message,
[ValidateSet("Info","Warning","Error")] [string]$Level = "Info",
[string]$LogFile = "C:\logs\customLog.txt"
)
$timestamp = Get-Date -Format "yyyy-MM-dd HH:mm:ss"
"$timestamp - [$Level] - $Message" | Out-File -FilePath $LogFile -Append
}
Practical Usage
You can call this enhanced logging function like this:
Write-Log "This is an informative message."
Write-Log "This is a warning message." -Level "Warning"
Write-Log "This is an error message." -Level "Error"
This method not only provides context for each log entry but also helps in sorting and filtering logs based on importance.
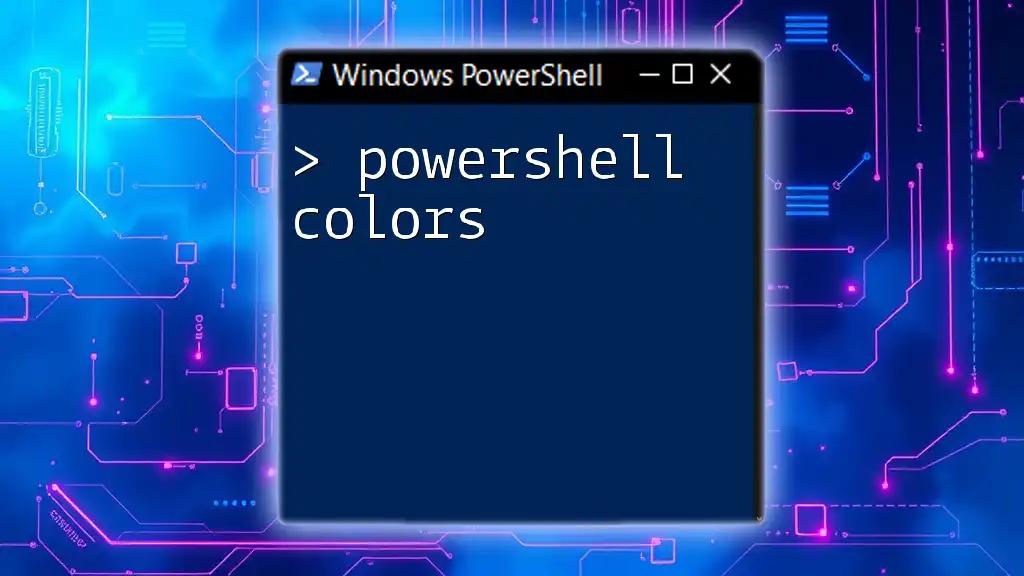
Best Practices for Console Logging
Structuring Log Entries
Consistency is key when creating log entries. Stick to a format that includes timestamps, message severity, and the message itself. This structure makes it easier for anyone to skim through logs and locate specific entries quickly.
Performance Considerations
Extensive logging can impact performance, especially during long script executions. To address this, consider:
- Reducing the frequency of log writing.
- Choosing to log only essential information.
- Using batch logging, where relevant messages are collected and written at intervals, rather than logging every single command.
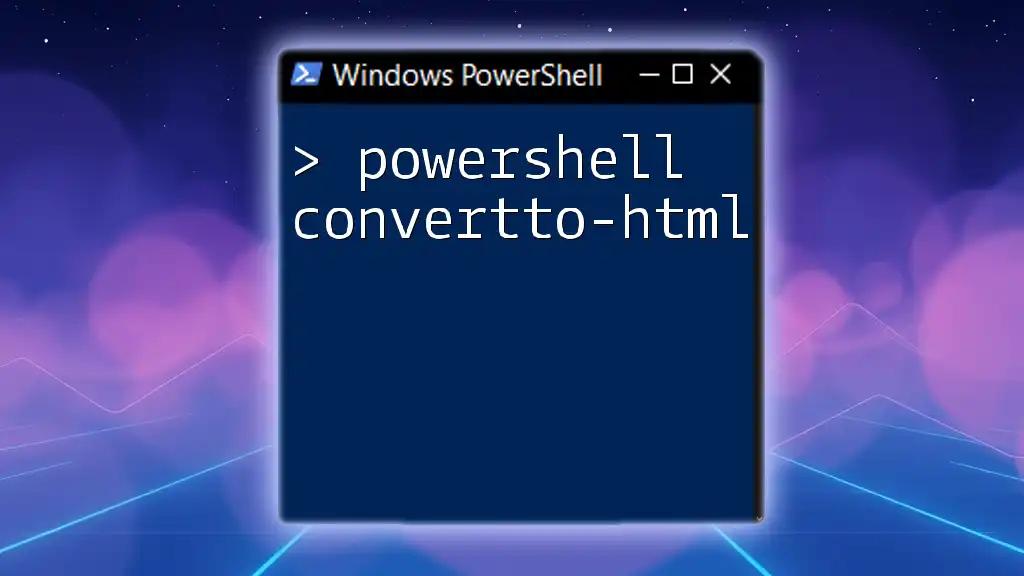
Practical Applications of PowerShell Console Logging
Debugging Scripts with Console Logs
Logs are invaluable for debugging, as they provide a real-time view of what is occurring within a script. For example, if a script fails, reviewing the log entries can allow you to identify the command responsible for the error.
By implementing a structured logging approach, you can isolate and troubleshoot issues efficiently, saving time during development.
Monitoring Long-Running Scripts
When monitoring longer running scripts, logs can serve as a progress tracking tool. For instance, you might log every significant step within a script, providing a thorough overview of its execution.
Incorporating log checks into scheduled jobs can also improve automation workflows. If a particular step fails, the logs can alert maintenance personnel to check specific job configurations or external dependencies.
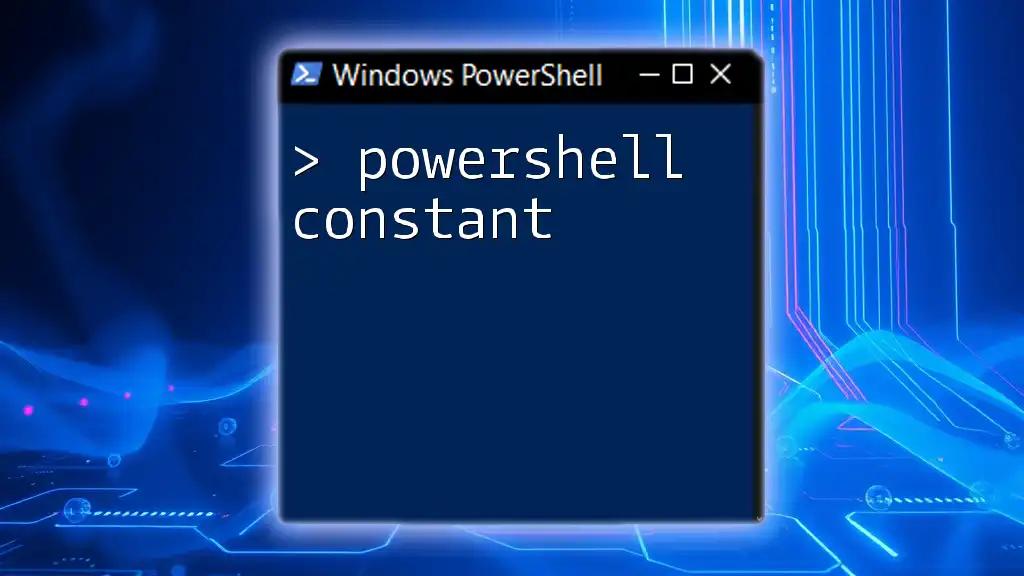
Conclusion
PowerShell console logging is an indispensable tool for anyone working with scripts, offering a way to enhance tracking, monitoring, and debugging effectively. By implementing the techniques and best practices outlined in this guide, you can improve the functionality and reliability of your PowerShell scripts. The incorporation of custom logging functions and severity levels can tailor logging to meet the needs of any project, ensuring a consistent and efficient workflow. Embrace these logging techniques to take full advantage of PowerShell's capabilities.
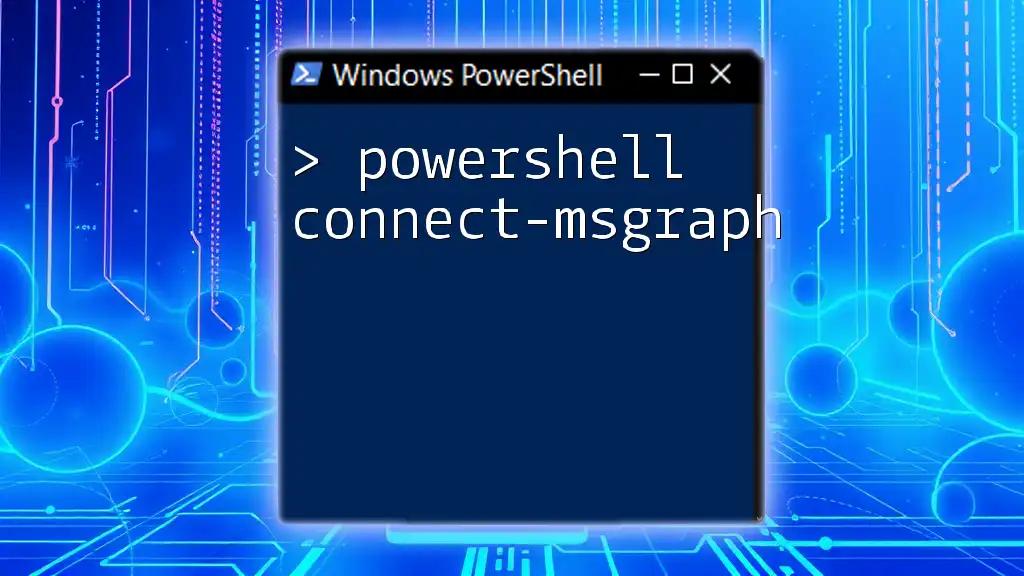
Additional Resources
For further learning on PowerShell console logs and advanced scripting techniques, consult PowerShell documentation, community forums, and user groups to deepen your understanding and enhance your skills.