A PowerShell while loop repeatedly executes a block of commands as long as a specified condition remains true.
Here’s a simple example:
$count = 1
while ($count -le 5) {
Write-Host "Count is $count"
$count++
}
Understanding PowerShell While Loop
How While Loops Work in PowerShell
A PowerShell while loop is a control flow statement that allows code to be executed repeatedly based on a specified condition. When the condition evaluates to `true`, the code within the loop is executed. This process continues until the condition becomes `false`.
Basic Syntax
The basic syntax of a while loop in PowerShell is as follows:
while (<condition>) {
# Code to execute
}
Here, `<condition>` is a logical expression that determines whether the loop will continue executing. If the condition is `true`, the code inside the braces is run. Understanding this structure is crucial for effectively implementing while loops in your scripts.

Executing a While Loop in PowerShell
Basic Example of a While Loop
To illustrate how a PowerShell while loop functions, consider the following simple example:
$counter = 0
while ($counter -lt 5) {
Write-Host "Counter is: $counter"
$counter++
}
In this example, we initialize a variable `$counter` to `0`. The loop checks if `$counter` is less than `5`. If it is, it executes the code inside the loop, printing the current value of `$counter` and incrementing it by `1`. This process repeats until `$counter` reaches `5`, at which point the condition evaluates to `false`, and the loop terminates.
The output will be:
Counter is: 0
Counter is: 1
Counter is: 2
Counter is: 3
Counter is: 4
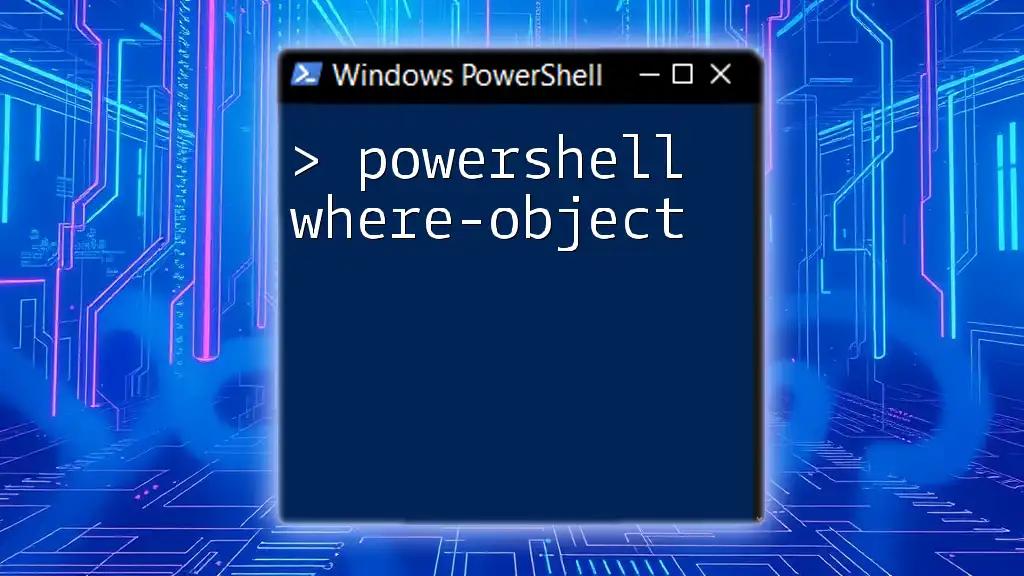
Practical Applications of PowerShell While Loop
Use Cases for While Loops
While loops are particularly useful for scenarios where the number of iterations is not predetermined. For example, they can be employed in:
- Monitoring tasks where the loop continually checks for a condition until it changes.
- Input validation, where the loop continues to prompt the user until valid input is received.
Example: Monitoring System Resources
Consider a situation where you want to monitor system processes actively. This can be accomplished using the following while loop:
while (Get-Process | Measure-Object).Count -gt 0 {
Get-Process | Sort-Object CPU -Descending | Select-Object -First 5
Start-Sleep -Seconds 5
}
Here, the loop continuously checks for running processes. As long as there are processes running (`Count -gt 0`), it retrieves the top five processes using the most CPU resources and then pauses for five seconds before the next iteration. This kind of loop is invaluable for system administrators needing to keep track of resource utilization in real time.
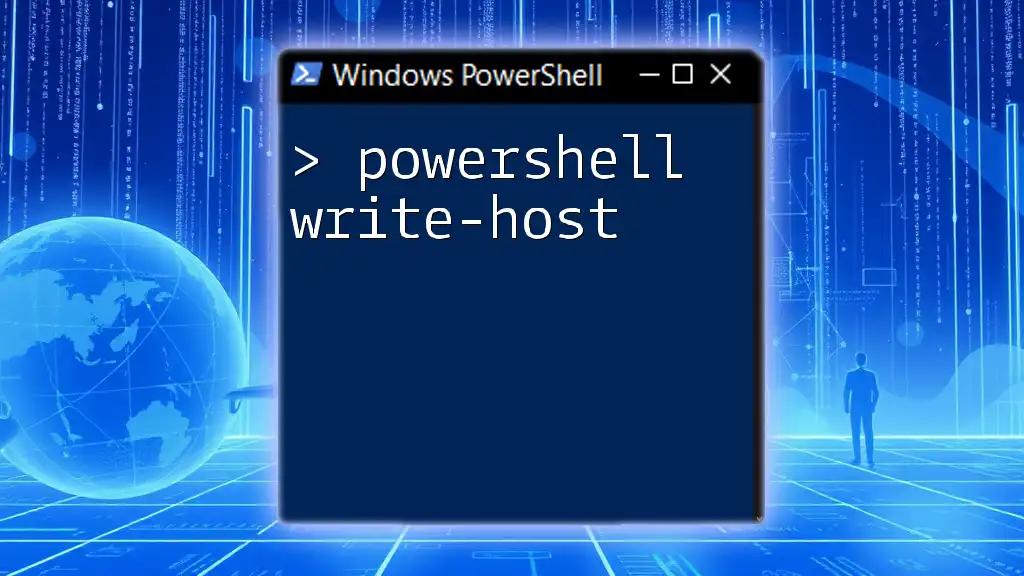
Variations of the While Loop
Using PowerShell While True
A while true loop is a specialized form that runs indefinitely until explicitly broken out of. It often looks like this:
while ($true) {
# Code to run indefinitely
}
An infinite loop can be useful in scenarios such as server scripts or applications that need to keep running until stopped manually. However, caution is required, as careless use can lead to unresponsive scripts. Always ensure you have a clear exit strategy, such as a conditional statement or user interrupt.

Tips for Using PowerShell While Loops Effectively
Avoiding Infinite Loops
One of the most common pitfalls when working with PowerShell while loops is inadvertently creating an infinite loop. To avoid this, keep the following strategies in mind:
- Ensure the loop has a clearly defined exit condition.
- Test loop conditions using debugging tools to validate the logic before execution.
Combining While Loops with Other Statements
Integrating if statements within a while loop can add extensive functionality. For example:
$value = 0
while ($value -lt 10) {
if ($value % 2 -eq 0) {
Write-Host "$value is even"
}
$value++
}
In this script, the loop continues until `$value` reaches `10`. Inside the loop, it checks if the value is even using the modulo operator. If the condition is true, it prints that the value is even. This showcases how while loops can be combined with conditional logic to achieve complex behaviors in your scripts.
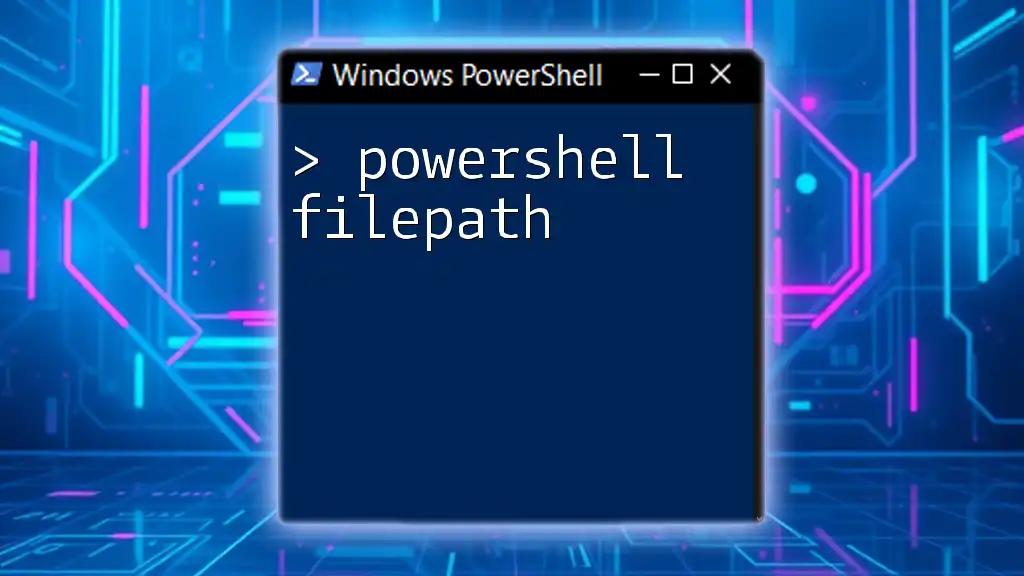
Conclusion
In summary, mastering the PowerShell while loop is a foundational skill for anyone looking to automate tasks or enhance their scripting capabilities. Whether you're checking system processes, validating inputs, or creating infinite loops, understanding how to leverage while loops will significantly boost your efficiency and effectiveness in scripting.
Remember, experimentation is key; create your own scripts using while loops to reinforce your understanding. The more you practice, the more comfortable you will become with this powerful tool in PowerShell.
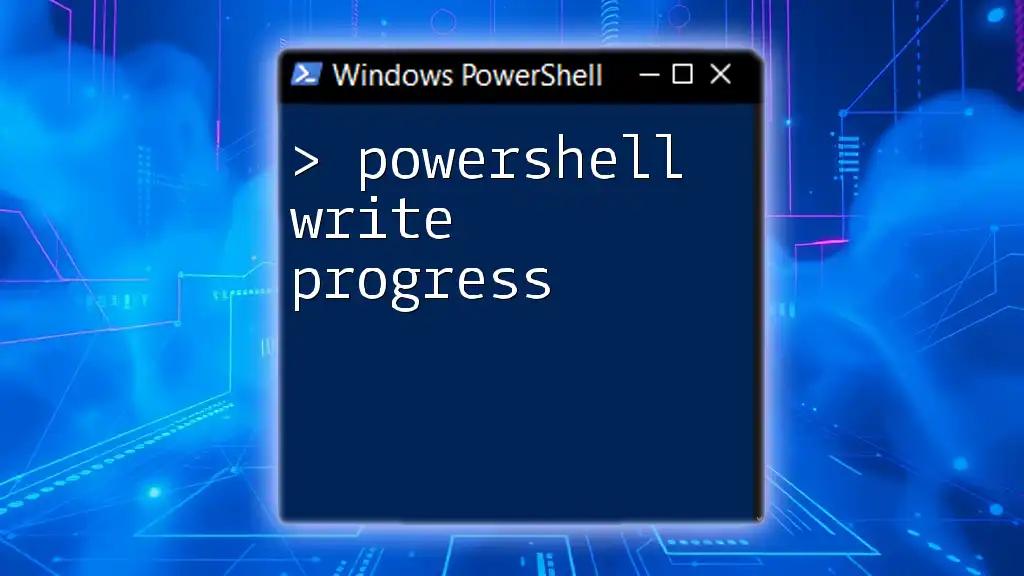
Additional Resources
To further enrich your learning experience, consider exploring the official [Microsoft PowerShell Documentation](https://docs.microsoft.com/en-us/powershell/) for detailed insights on loops and control flow statements. Joining online forums and communities dedicated to PowerShell can also provide valuable support and knowledge-sharing opportunities. Happy scripting!