To exit a loop in PowerShell, you can use the `break` statement to terminate the loop immediately. Here's a code snippet demonstrating its usage:
for ($i = 0; $i -lt 10; $i++) {
if ($i -eq 5) { break }
Write-Host $i
}
In this example, the loop will output numbers 0 to 4 and then stop when `$i` equals 5.
Understanding PowerShell Loops
What are Loops?
In programming, a loop is a fundamental concept that allows you to execute a block of code multiple times. In PowerShell, loops are crucial for automating repetitive tasks and managing large datasets efficiently. There are several types of loops you can use in PowerShell, including:
- For loop: Executes a block of code a specified number of times.
- While loop: Continues to execute as long as a specified condition is true.
- Do...While loop: Ensures that code is executed at least once before the condition is tested.
The Need for Exiting Loops
While loops are powerful, there are times when you might need to exit them prematurely. This is especially true in situations such as:
- Infinite loops, where a loop runs indefinitely due to an unmet exit condition.
- Conditional exits when a specific condition requires the loop to stop, potentially improving performance or avoiding unnecessary processing.
- Dynamic scenarios where input data might change, leading to the necessity of breaking out of the loop based on varying conditions.
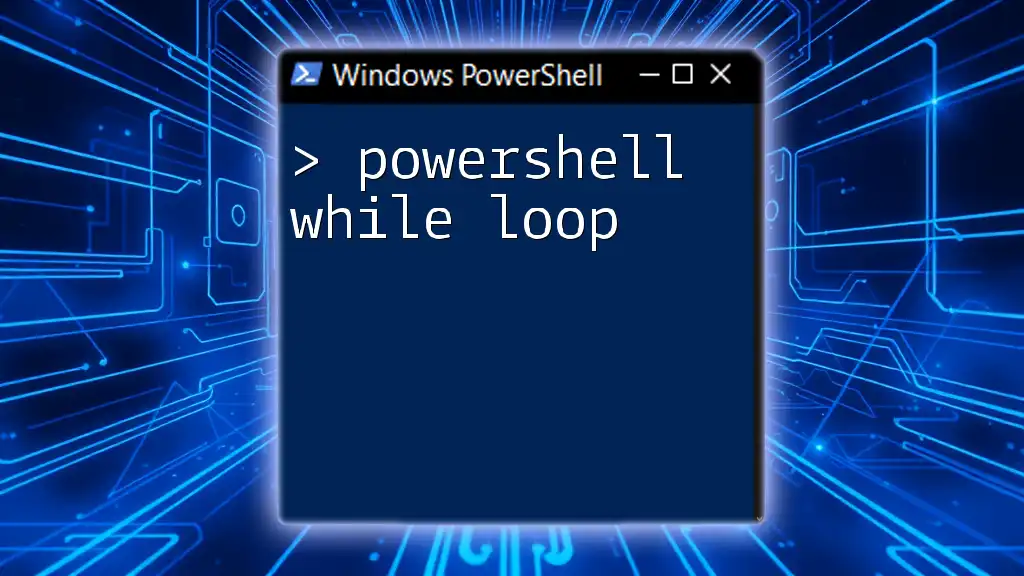
PowerShell Break Statement
What is the Break Statement?
The break statement is a control flow statement that allows you to exit from loops early. It is particularly valuable when you want to stop executing the loop based on a certain condition without waiting for the loop’s natural termination.
The syntax for the break statement is straightforward:
break
How to Use Break in PowerShell
To illustrate the basic use of the break statement, consider the following example, where a while loop continues until a particular count is reached:
$count = 0
while ($true) {
if ($count -eq 5) {
break
}
Write-Host "Count is: $count"
$count++
}
In this example, the loop will terminate when `$count` reaches 5. The break statement effectively halts the loop, avoiding unnecessary iterations and making the script more efficient.
Using Break to Exit Nested Loops
Nested loops can complicate matters when it comes to exiting. However, you can use the break statement to exit from an inner loop while keeping the outer loop intact. Here’s how:
for ($i = 0; $i -lt 3; $i++) {
for ($j = 0; $j -lt 5; $j++) {
if ($j -eq 2) {
break
}
Write-Host "i: $i, j: $j"
}
}
In this case, the inner loop will break when `$j` equals 2, but the outer loop will continue to execute. This selective control is beneficial in scenarios where you may want to process multiple sets of data without terminating the entire loop structure.
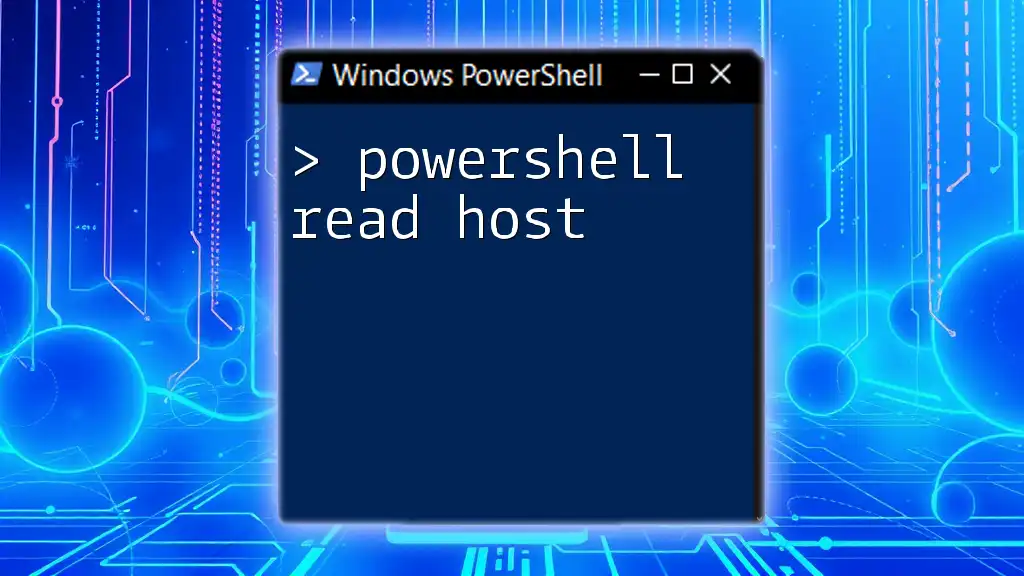
Advanced Techniques with Break
Combining Break with Conditions
Utilizing the break statement with conditions can enhance the flexibility of your loops. Here’s an example of how you might incorporate an if condition to break out of a loop:
$numbers = 1..10
foreach ($number in $numbers) {
if ($number -eq 7) {
break
}
Write-Host "Current number: $number"
}
In this scenario, the loop will exit as soon as the number 7 is encountered. This method is particularly useful when you're processing conditional data sets and need to terminate early based on specific requirements.
Error Handling using Break
Another interesting use case for the break statement involves error handling. The try-catch block can accommodate breaks to gracefully exit while handling exceptions. Here’s an example:
try {
# Simulating an error
throw "An error occurred"
} catch {
Write-Host "Error caught, exiting loop"
break
}
In this example, the break statement allows you to exit the loop when an error occurs, preventing further processing and providing a clear error handling mechanism. This approach can be crucial when dealing with data integrity issues or other exceptions during script execution.
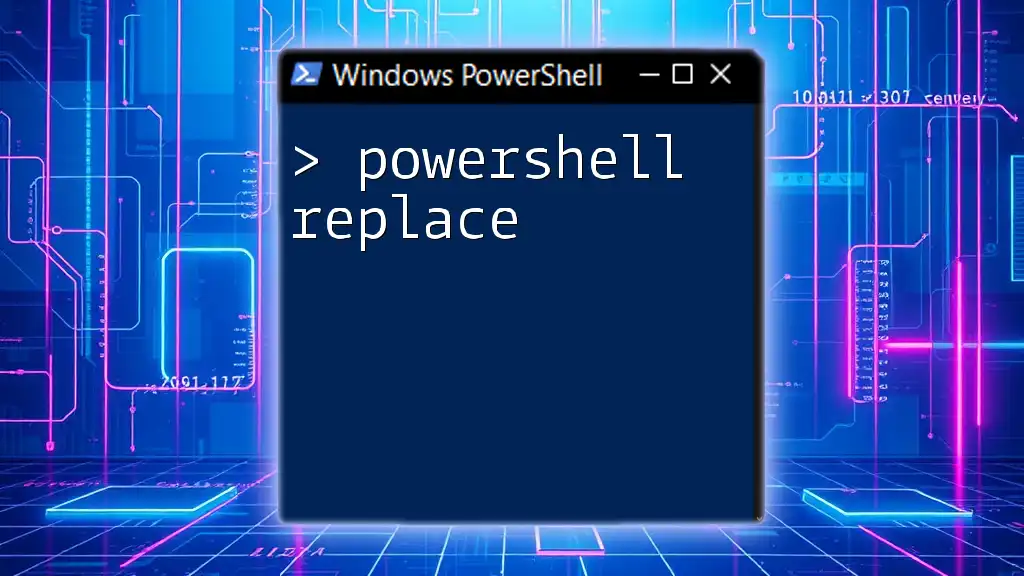
Best Practices
When to Use Break
While the break statement can be a powerful tool in controlling the flow of your scripts, it’s essential to use it judiciously. Guidelines for appropriate break usage include:
- Use it when you need to exit a loop under specific conditions.
- Avoid excessive use, which can lead to confusion in the code flow and degrade readability.
Alternatives to Break
In addition to the break statement, there are other control flow statements to consider:
- Continue: Skips the current iteration and proceeds with the next one.
- Return: Exits from a function and can also be used within loops when it's necessary to terminate processing.
Understanding when to use each of these alternatives can improve the clarity and intention of your scripts.
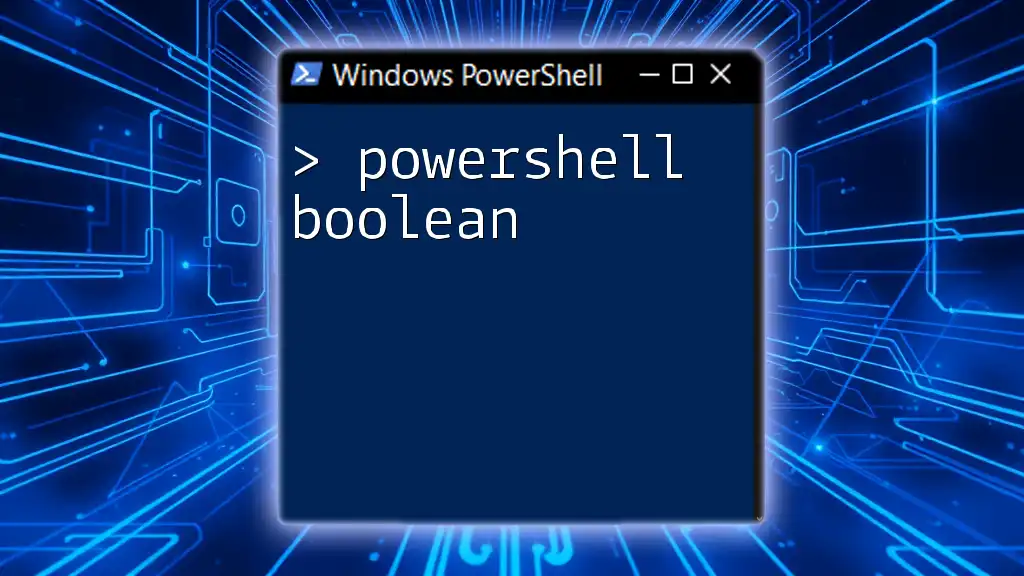
Conclusion
The power of the break statement in PowerShell cannot be overstated. It provides vital control over the execution of loops, making your scripts more efficient and responsive to conditions. By applying the techniques discussed, you can harness the capabilities of the break statement effectively. As you continue to explore PowerShell, remember to consider how the break statement can enhance your scripts and avoid unnecessary processing in your automation tasks.