The `Read-Host` cmdlet in PowerShell allows you to prompt the user for input, enabling interactive script behavior by capturing input from the command line.
Here’s a code snippet to illustrate its usage:
$name = Read-Host 'Please enter your name'
Write-Host "Hello, $name!"
Understanding Read-Host PowerShell Command
What is Read-Host?
The `Read-Host` cmdlet in PowerShell is designed to prompt users for input during the execution of a script. This feature opens up a wider range of possibilities for interactive scripts, allowing scripts to be dynamic and responsive to user needs. Whether you need to gather simple data or sensitive information, `Read-Host` is an invaluable tool.
Key Parameters of Read-Host
One of the main features of the `Read-Host` cmdlet is its `-Prompt` parameter, which allows you to provide a custom message or question to the user. This enhances the user experience by making it clear what type of input is being requested.
For example, a script that asks for a user's name could utilize the following code:
$userInput = Read-Host -Prompt "Please enter your name"
Write-Output "Hello, $userInput!"
In this snippet, the prompt clearly indicates what information is needed, making it straightforward for users to provide the correct input.
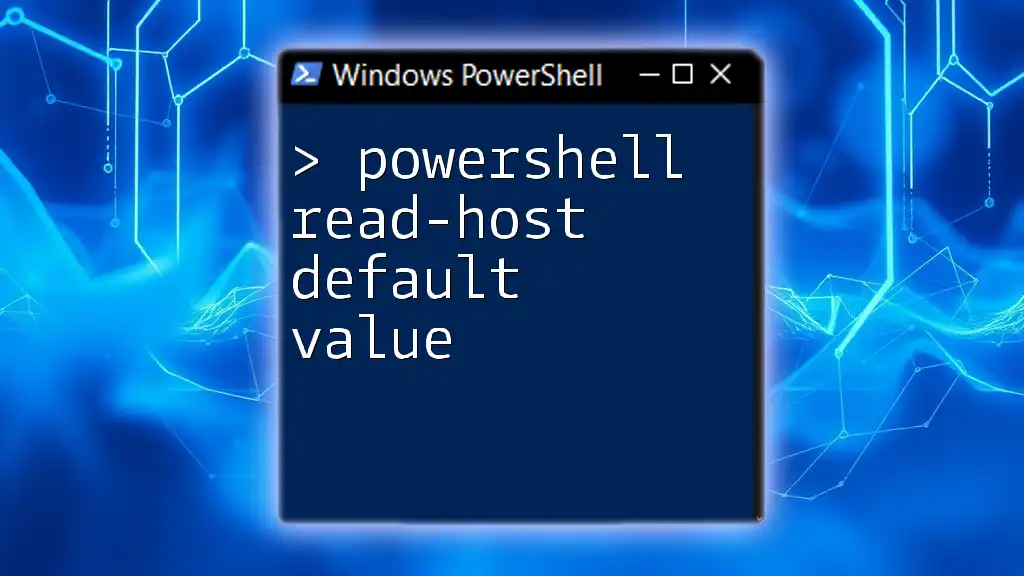
Basic Usage of Read-Host in PowerShell
Simple Input Example
One of the simplest applications of `Read-Host` is to collect information from a user. The example given above serves as a clear illustration, where the script requests the user's name and then greets them accordingly. This simple interaction can be the foundation of more complex scripts.
Collecting Sensitive Input
When dealing with sensitive information, such as passwords, `Read-Host` has an option to mask the input, ensuring that it remains confidential. This is achieved using the `-AsSecureString` parameter, which transforms the input into a secure string.
For instance:
$password = Read-Host -Prompt "Enter your password" -AsSecureString
Using the `-AsSecureString` parameter prevents the password from being displayed on the screen, enhancing security in scripts where sensitive data is handled.
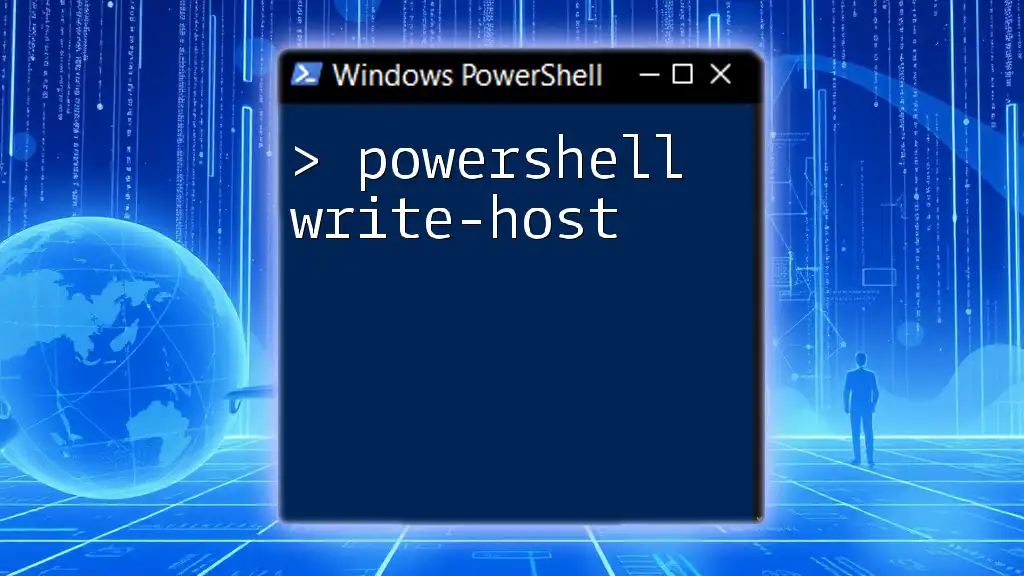
Advanced Features of Read-Host
Validating User Input
It’s crucial to validate user input to avoid errors or malicious entries. After collecting input, you can use conditional statements to enforce rules or guidelines. For example, you could check that a user’s age isn’t negative:
$age = Read-Host -Prompt "Please enter your age"
if ($age -lt 0) {
Write-Output "Age cannot be negative."
} else {
Write-Output "You are $age years old."
}
This snippet demonstrates how basic validation can help maintain data integrity within your scripts.
Using Read-Host in Loops
For scripts where repeated input may be necessary, `Read-Host` can be used within loops. This approach allows users to provide input multiple times until a specific condition is met. A simple example is a script that continues to ask if the user wishes to proceed:
do {
$response = Read-Host -Prompt "Do you want to continue? (yes/no)"
} while ($response -ne "no")
This example will keep the script active and engaged with the user until they choose to stop.
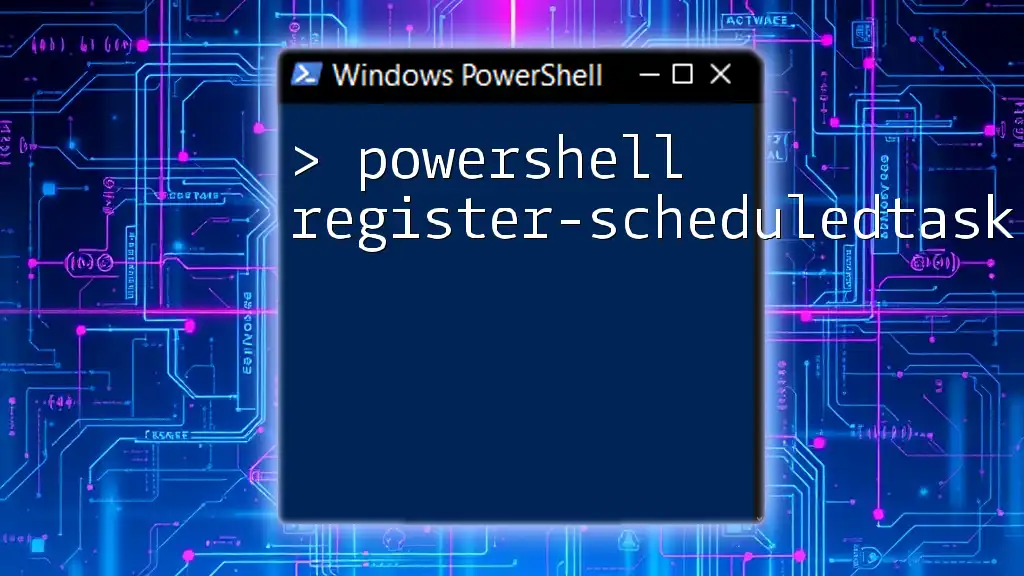
Practical Applications of Read-Host in Scripts
Interactive Scripts
`Read-Host` significantly enhances the interactivity of your scripts. For instance, you can create a menu-driven user interface where users can select options. Using prompts effectively, you can guide users through a series of choices, keeping engagement high.
Incorporating Read-Host for Configurations
Another critical application of `Read-Host` is in configuring systems or applications. For example, you might ask users for configuration details before executing a setup process:
$serverName = Read-Host -Prompt "Please enter the server name"
$port = Read-Host -Prompt "Enter the port number"
Write-Output "Configuring connection to $serverName on port $port."
By capturing these details interactively, your scripts can adapt to various environments without hard-coded values.
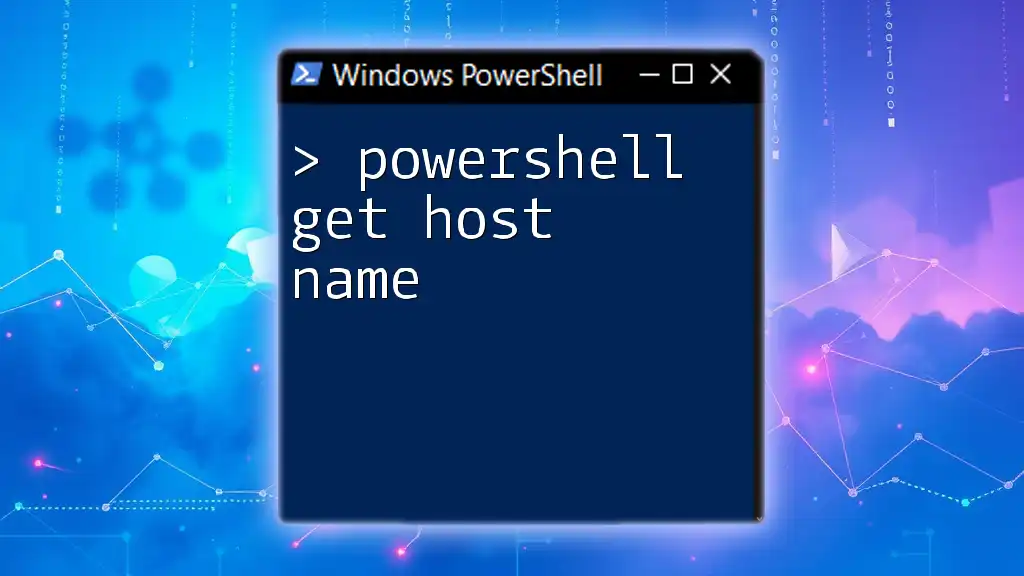
Best Practices When Using Read-Host
Keeping Code Clean and Readable
When using `Read-Host`, clarity is essential. Ensure that your prompts are meaningful and that your code remains legible. Use descriptive variable names and consistent formatting for an easy-to-follow script. This not only aids in future modifications but also enhances collaboration with other developers.
Security Considerations
Always keep security in mind when collecting user input. Be cautious about how you handle and store sensitive data. Using `-AsSecureString` is one way to mitigate risks, but it’s also essential to enforce secure practices throughout your entire script, including data encryption when necessary.
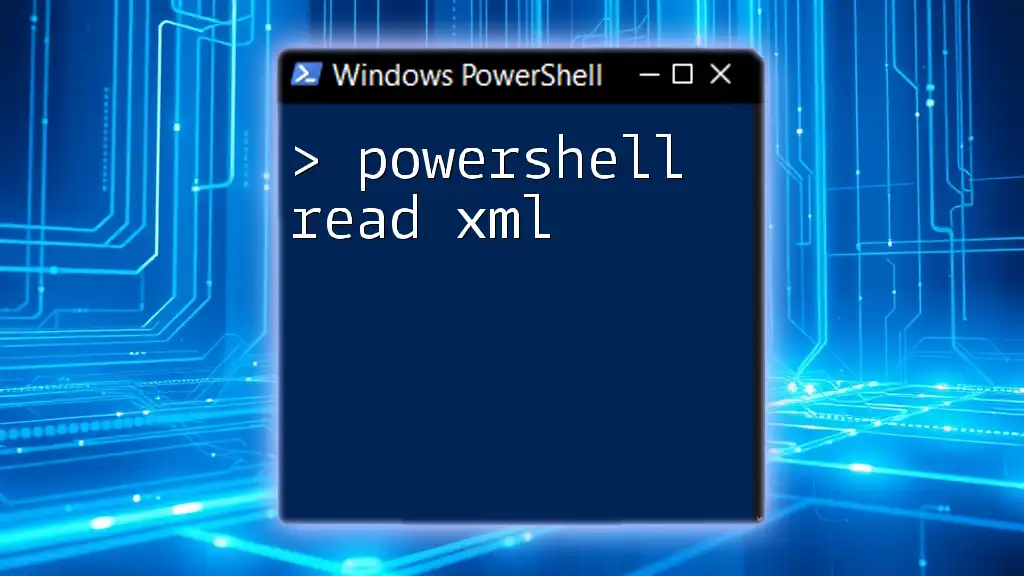
Conclusion
The `PowerShell Read-Host` cmdlet is a versatile and powerful tool that enhances interactivity in your scripts. Whether you're capturing user input for a configuration setting or needing to validate entries to ensure data integrity, `Read-Host` opens the door for creating dynamic scripts.
By experimenting with the examples provided and considering best practices, you can effectively harness the power of `Read-Host` in your PowerShell projects. Happy scripting!
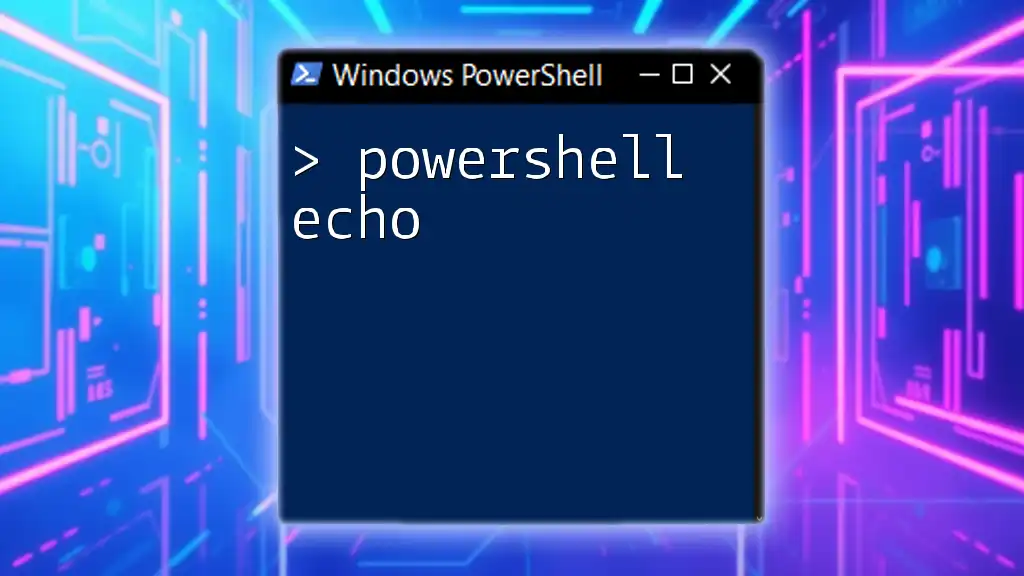
Additional Resources
For further understanding and exploration of `Read-Host`, consider consulting the official PowerShell documentation for a deep dive into its capabilities. Engaging with community forums can also provide valuable insights and shared experiences that can enhance your learning journey in PowerShell scripting.