The `Read-Host` command in PowerShell allows you to prompt users for input while also providing a default value that is used if they simply press Enter without typing anything.
Here’s an example code snippet demonstrating how to use `Read-Host` with a default value:
$input = Read-Host -Prompt 'Enter your name' -Default 'Guest'
Write-Host "Hello, $input!"
Understanding Read-Host
What is Read-Host?
`Read-Host` is a cmdlet in PowerShell that allows you to prompt users for input during script execution. This cmdlet pauses script execution until the user provides some input. It is particularly useful when you want to make a script interactive or require input parameters that can’t be predefined.
The basic syntax of the command is as follows:
Read-Host "Prompt message"
This cmdlet is not just limited to accepting plain text. It can also be used to gather sensitive information securely, such as passwords, by using the `-AsSecureString` parameter.
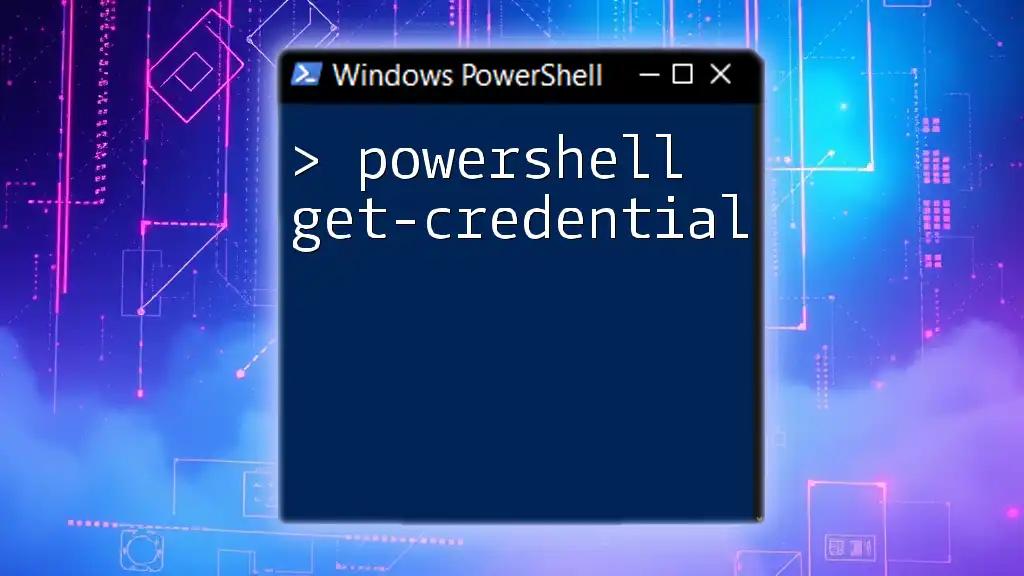
Default Value in Read-Host
What is a Default Value?
A default value is a pre-set value provided in a prompt that enables users to either accept it by pressing Enter or overwrite it with their desired input. Default values can help speed up user interactions and reduce errors by guiding users on what is expected, providing a reference that is usually relevant or sensible.
How to Implement Default Values in Read-Host
To implement default values in the `Read-Host` cmdlet, you can use a conditional statement to check if the user has provided any input. If no input is given, the script can set a predetermined default value. The pattern typically looks like this:
- Prompt for user input.
- Check if the received input is empty.
- If the input is empty, assign the default value.
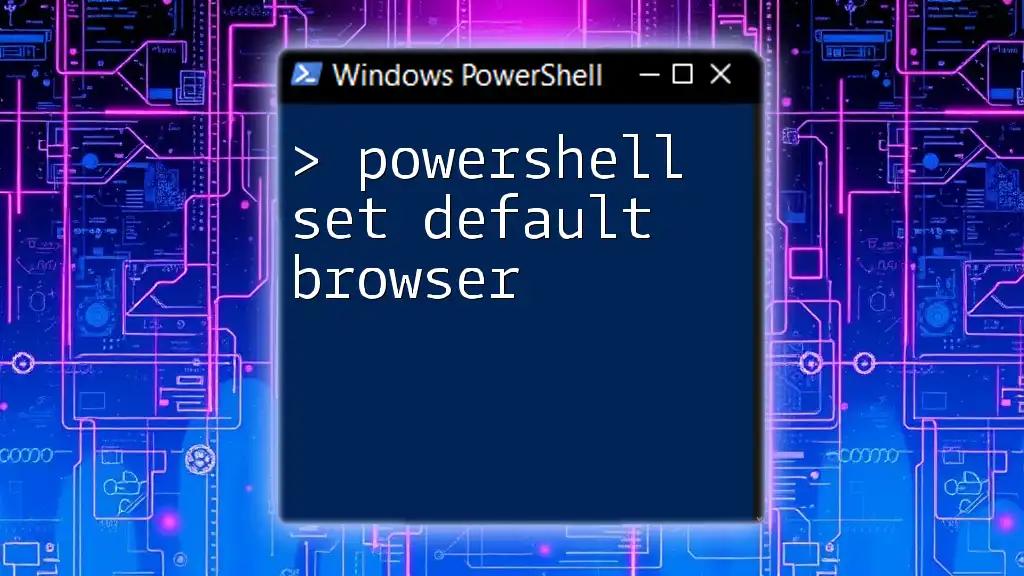
Code Examples
Example 1: Basic Usage of Read-Host with Default Value
In this basic example, we prompt the user for their name and provide a default value of "John Doe":
$userName = Read-Host "Enter your name (default: John Doe)"
if (-not $userName) {
$userName = "John Doe"
}
Write-Host "Hello, $userName!"
Explanation:
- The command `Read-Host` prompts the user to enter their name.
- If the user simply presses Enter without typing anything, the condition `-not $userName` will evaluate to true, and `$userName` will be set to "John Doe".
- Finally, we greet the user with the name they entered or the default value if they didn’t provide any.
Example 2: Using Default Values in Complex Scenarios
Here is another example that prompts for a favorite color, again with a default value of "Blue":
$favoriteColor = Read-Host "Enter your favorite color (default: Blue)"
if (-not $favoriteColor) {
$favoriteColor = "Blue"
}
Write-Host "Your favorite color is $favoriteColor!"
You can see how this could be adapted for more complex input processes by using additional conditional checks or user interactions. Such practices make your script more robust and flexible.
Example 3: Validating User Input when Using Default Values
When working with user input, it’s essential to ensure that the input is valid. Here’s how you can implement simple input validation:
$ageInput = Read-Host "Enter your age (default: 30)"
if (-not $ageInput) {
$ageInput = 30
}
if ($ageInput -lt 0) {
Write-Host "Please enter a valid age."
} else {
Write-Host "You are $ageInput years old."
}
Explanation:
- After capturing the input, the script checks if the user input is missing. If it is, the variable `$ageInput` is set to 30.
- Next, it verifies if the entered age is valid (greater than or equal to zero). If the input is invalid, it prompts the user to enter a valid age.
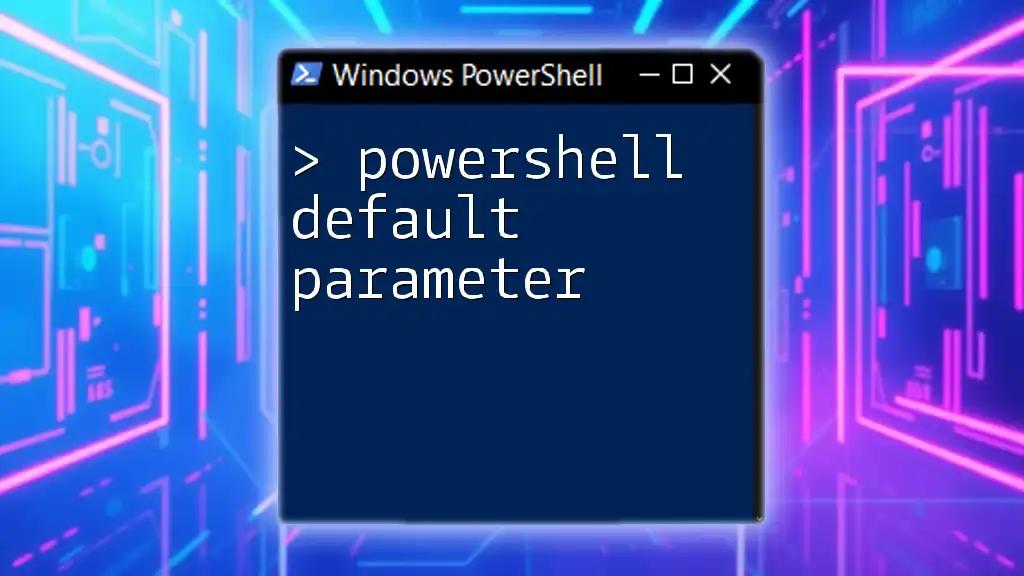
Best Practices
Effective Prompts
When creating prompts using `Read-Host`, clarity is crucial. Well-crafted messages help users understand what type of input is expected. Ensure you communicate effectively by providing clear instructions and hinting at default values.
Ensuring Robustness in Scripts
Always anticipate and handle potential issues with user input. Implement robust validation checks to ensure the accuracy and validity of the input, especially for critical variables.
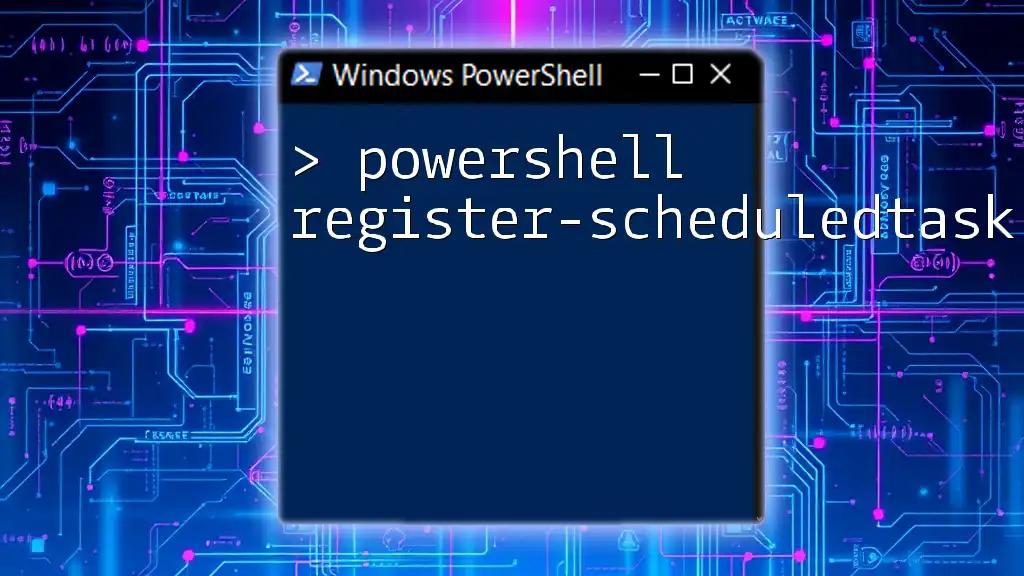
Troubleshooting Common Issues
Common Pitfalls with Default Values
One of the most common mistakes made when using default values is forgetting to check for empty input. If you neglect the conditional statement to catch empty inputs, your script may behave unexpectedly. Always ensure that your script can handle edge cases and invalid inputs gracefully.
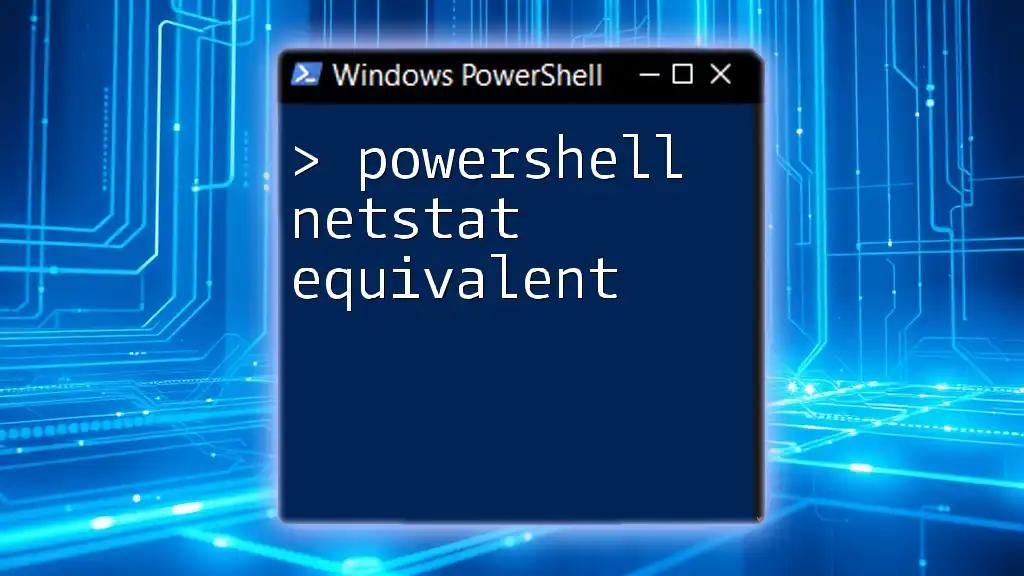
Conclusion
Incorporating `Read-Host` with default values in PowerShell scripts can greatly enhance user experience and streamline data collection during script execution. By following effective practices in prompt crafting and input validation, you can improve the robustness and reliability of your scripts. Experimenting with the examples provided will give you hands-on experience and a deeper understanding of how to leverage `Read-Host`.
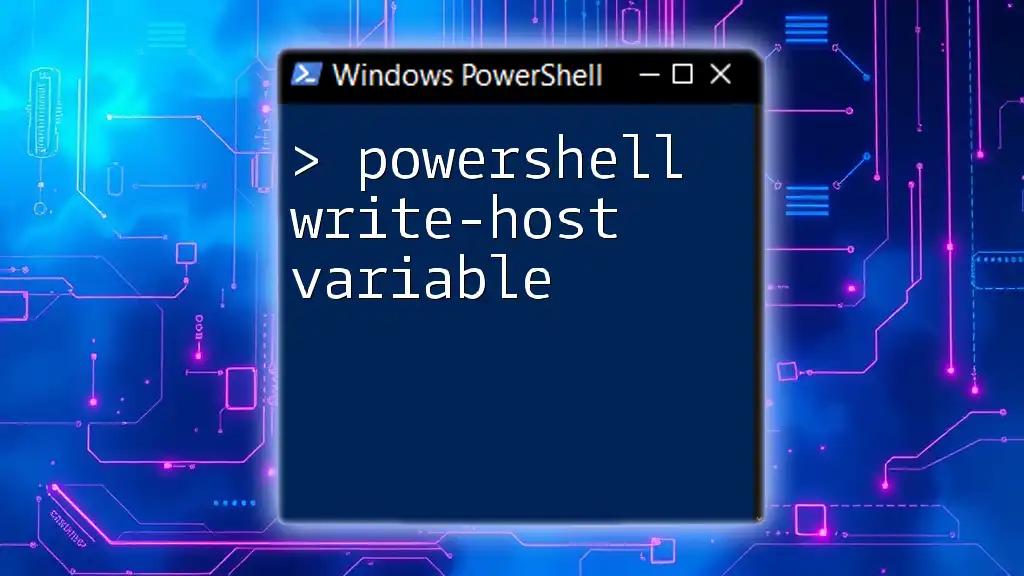
Further Resources
For more information on PowerShell scripting techniques, consider exploring additional readings, tutorials, and documentation detailing advanced features of PowerShell. Participating in online PowerShell communities and forums can also provide valuable insights and support from fellow learners and experts.
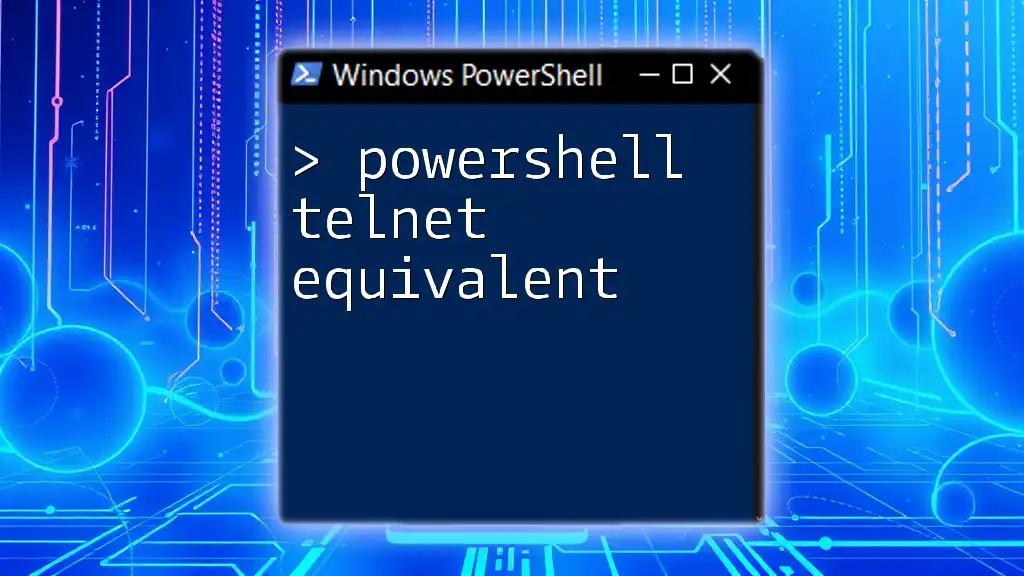
Call to Action
If you found this guide helpful, subscribe for more insights on mastering PowerShell. We welcome feedback and topic suggestions for future articles to enhance your PowerShell learning journey!