In PowerShell, default parameters allow you to specify a default value for a parameter in a function, making it optional for the user to provide an input if the default is acceptable.
Here's a code snippet demonstrating the usage of a default parameter:
function Greet {
param (
[string]$Name = "World" # Default parameter
)
Write-Host "Hello, $Name!"
}
Greet # Outputs: Hello, World!
Greet -Name "Alice" # Outputs: Hello, Alice!
Understanding Parameters in PowerShell
What are Parameters?
In PowerShell, parameters are fundamental components of functions that allow users to pass information into those functions. This enhances usability and flexibility, enabling you to customize the function's behavior based on the provided input.
Types of Parameters
Positional Parameters are the simplest form, where the order in which you pass the arguments matters. For example, in a function defined as `Function Foo ($a, $b)`, `$a` is the first parameter, and `$b` is the second.
Named Parameters allow you to specify the name of the parameter when calling a function, providing greater clarity and flexibility. For instance, you can call `Foo -b 2 -a 1`, rearranging the order without any issues.
Switch Parameters function as Boolean flags. They don't require a value; instead, their presence indicates "true." For example, a function using a switch parameter could enable verbose output when the flag is set.
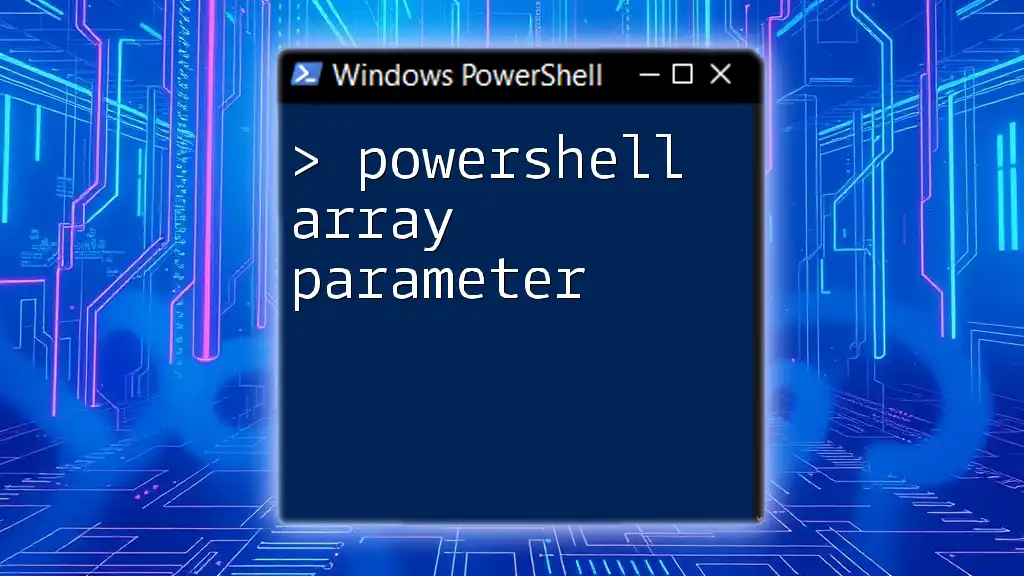
Default Parameters in PowerShell Functions
What is a Default Parameter?
A default parameter is a parameter that has a pre-set value defined within a function. It allows the function to operate smoothly even when no arguments are passed by the user. This can significantly streamline processes, especially for users who may not provide input frequently.
How to Set Default Parameters
You can define default parameters by assigning a value in the function's `param` block. Here’s an example:
function Greet {
param(
[string]$Name = "User"
)
"Hello, $Name!"
}
In this example, if a user calls `Greet` without any input, they will receive the output "Hello, User!" This feature is particularly valuable as it allows for the graceful handling of unexpected input scenarios.
Calling a Function with Default Parameters
By setting default parameters, users can call the function without needing to specify every argument.
For instance:
Greet # Output: Hello, User!
Greet -Name "Alice" # Output: Hello, Alice!
In the first call, the default value is used, while in the second call, the user specifies "Alice," overriding the default behavior. This illustrates the flexibility that default parameters offer in function calls.
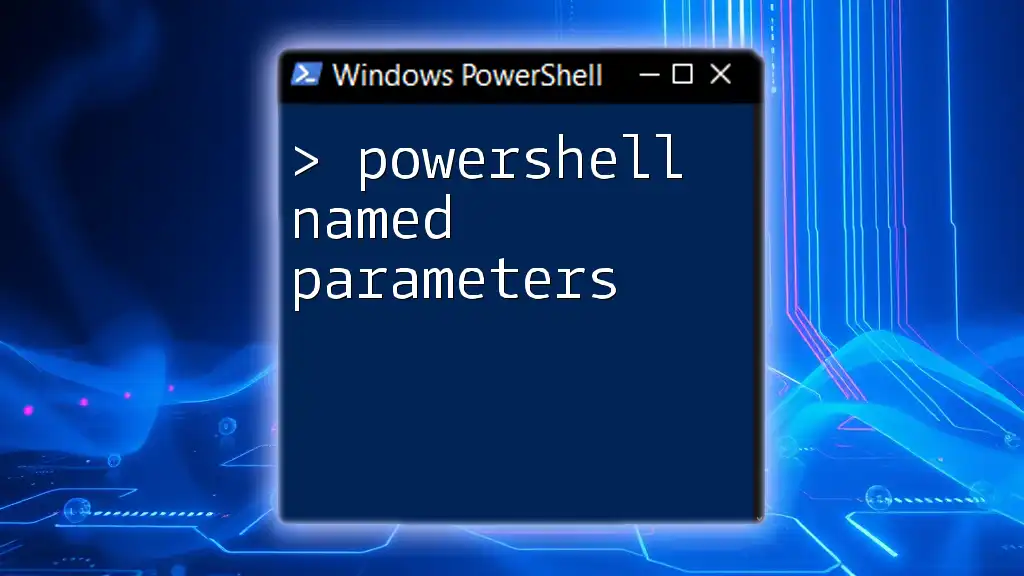
Defining Multiple Default Parameters
Setting Up Functions with Multiple Default Parameters
You can also define multiple default parameters in a single function to provide even more versatility. Here’s a practical example:
function Introduce {
param(
[string]$FirstName = "John",
[string]$LastName = "Doe"
)
"My name is $FirstName $LastName."
}
Example of Calling with Different Parameter Combinations
Using the `Introduce` function, you can make calls in various ways:
Introduce # Output: My name is John Doe.
Introduce -FirstName "Jane" # Output: My name is Jane Doe.
Introduce -LastName "Smith" # Output: My name is John Smith.
This flexibility allows the function to cater to different contexts without requiring users to provide all arguments every time.
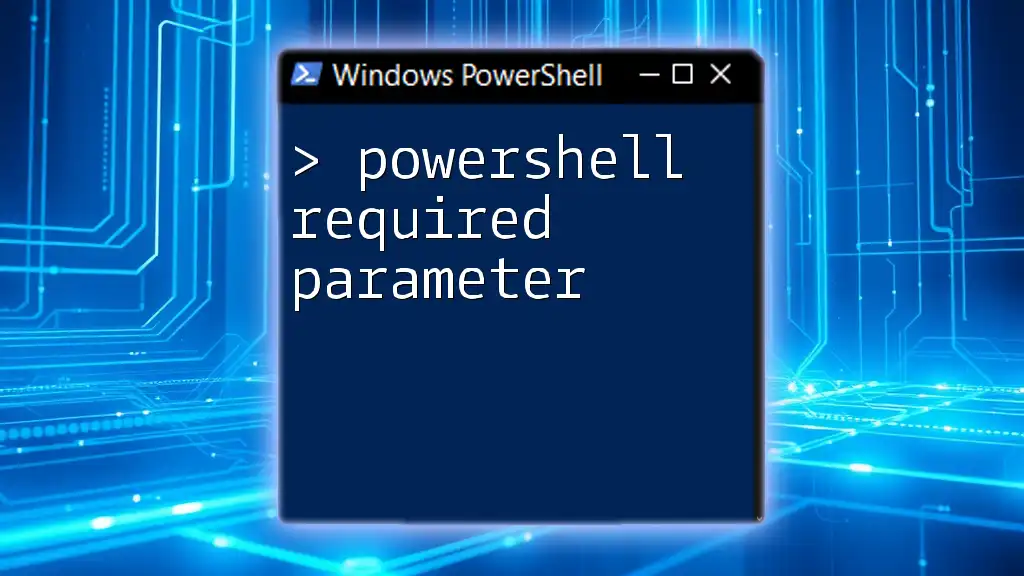
Best Practices for Default Parameters
When to Use Default Parameters
Consider using default parameters when you want to provide a common baseline or "fallback" behavior for your functions. They can make functions more user-friendly and reduce the potential for errors if the user forgets to provide a parameter.
Keeping Functions Readable
Always strive to make your functions easy to read and understand. When using default parameters, ensure that the defaults themselves are meaningful and relevant to the function's purpose. Clear documentation is key—make sure to explain what default values will lead to, so users aren't confused when they receive unexpected output.
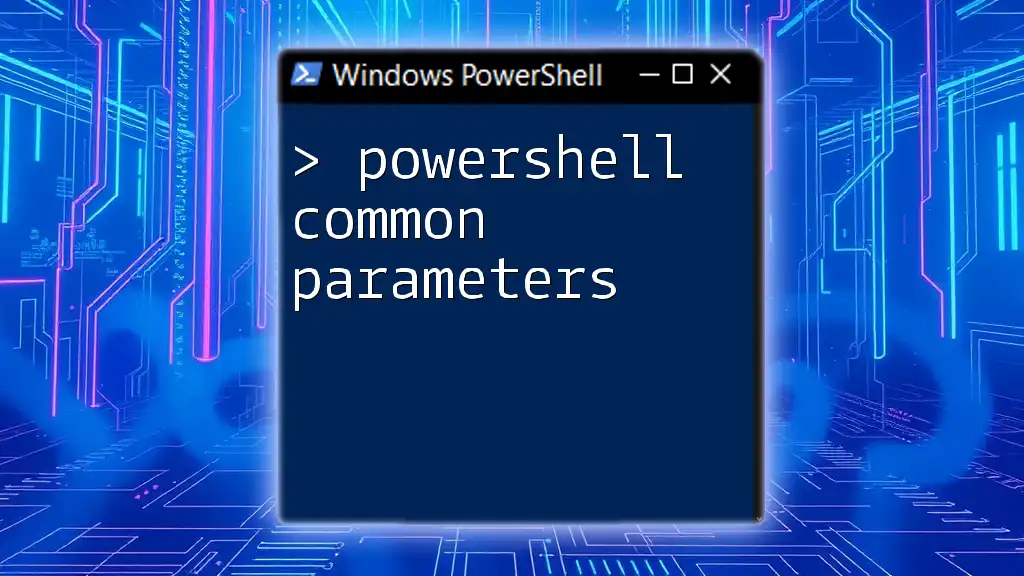
Advanced Usage of Default Parameters
Working with Optional Parameters
Default parameters can be combined with optional parameters to create versatile functions. Here’s an example:
function ShowMessage {
param(
[string]$Message = "This is a default message.",
[bool]$IsImportant = $false
)
if ($IsImportant) {
"IMPORTANT: $Message"
} else {
$Message
}
}
Example of Calling the Function with Optional Parameters
When you call this function, various outputs can occur:
ShowMessage # Output: This is a default message.
ShowMessage -IsImportant $true # Output: IMPORTANT: This is a default message.
ShowMessage -Message "Hello" # Output: Hello.
This demonstrates how combining both optional and default parameters allows for a rich user experience and flexible function behavior.
Combining Default Parameters with Validation
You can utilize validation attributes alongside default parameters, enhancing the robustness of your functions. For instance:
function ValidateInput {
param(
[ValidateSet("Small", "Medium", "Large")]
[string]$Size = "Medium"
)
"You selected the size: $Size."
}
With the `ValidateSet` attribute, if a user tries to pass an invalid size (e.g., “ExtraLarge”), PowerShell will throw an error, preventing unintended behavior.
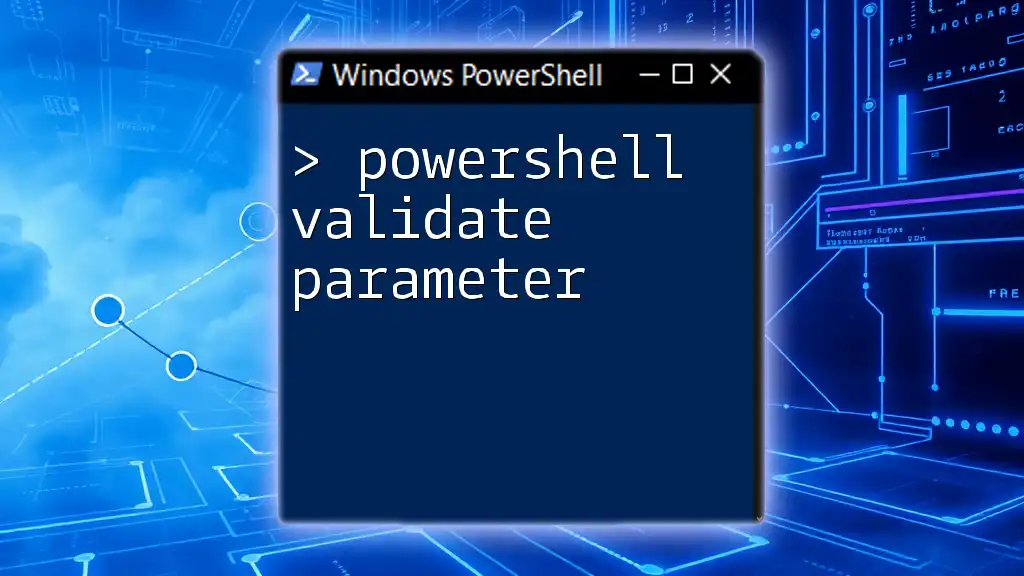
Common Mistakes to Avoid
Overriding Default Parameters Incorrectly
One common mistake when working with default parameters is misunderstanding how to override them. Users sometimes call a function without realizing that the defaults will apply unless explicitly overridden with correctly named parameters. Always clarify the parameters in your documentation.
Failure to Provide Context
Using default parameters meaningfully is crucial. Developers should avoid using default values that do not provide clarity or context; otherwise, users may be left confused about the function's purpose. For instance, if the default value does not relate to the function's theme, it can lead to misunderstandings.
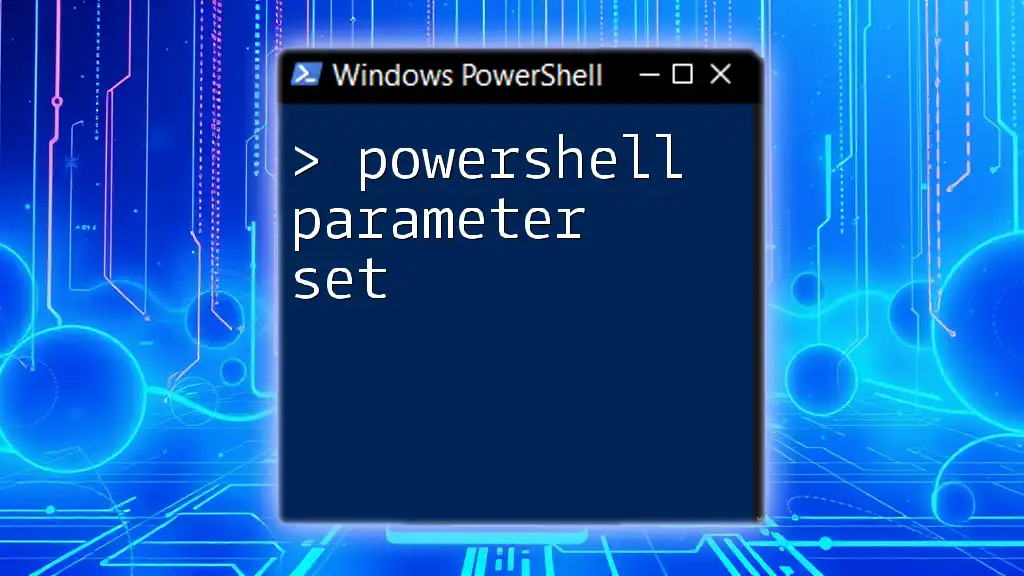
Conclusion
Mastering PowerShell default parameters significantly enhances the usability and elegance of your scripts. They offer an excellent balance between flexibility and control, simplifying the user's experience in passing function arguments. Commit to practicing with default parameters in your functions to deepen your understanding and improve your PowerShell scripting skills.
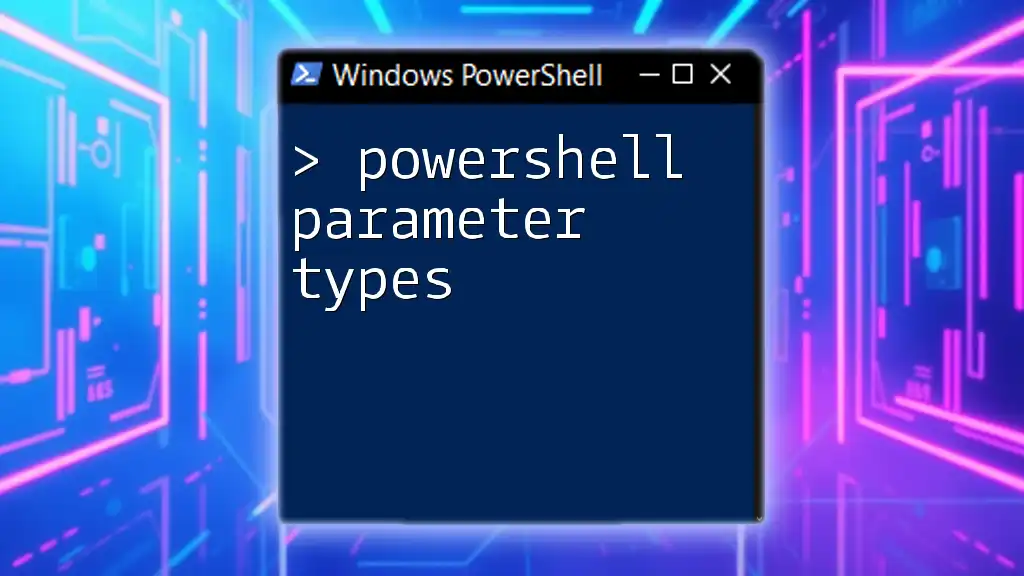
Additional Resources
For deeper learning and further exploration of default parameters and PowerShell functions, refer to the official PowerShell documentation and consider enrolling in dedicated PowerShell courses to hone your skills.