The `FullName` property in PowerShell is used to retrieve the full path of a file or directory object, providing a clear way to reference its exact location in the file system. Here's a simple code snippet to demonstrate this:
# Get the full name of a specified file
$file = Get-Item 'C:\example\myfile.txt'
Write-Host $file.FullName
Understanding the FullName Property
What is the FullName Property?
The `FullName` property in PowerShell represents the complete path of a file or directory. This path includes the drive letter, all folder names, and the filename itself. It differs from other path properties such as `Name`, which provides only the filename, and `Path`, which represents the parent directory path without the complete context.
For instance, if you have a file located at `C:\Users\Username\Documents\File.txt`, the value of its `FullName` would be:
C:\Users\Username\Documents\File.txt
The clarity provided by `FullName` is essential for scripting, as it eliminates ambiguity about which file or folder is being referenced.
Importance of FullName in File Management
In system administration and automation tasks, knowing the `FullName` of files and directories allows for precise operations. It prevents errors that can occur when using relative paths or when dealing with multiple files with the same name in different directories.
Real-world scenarios where `FullName` plays a crucial role include:
- File backups: When writing scripts to back up files, referencing the complete path ensures that the correct files are selected and copied.
- Automated reports: Generating reports that include file information often requires exact paths to avoid confusion when files are processed.
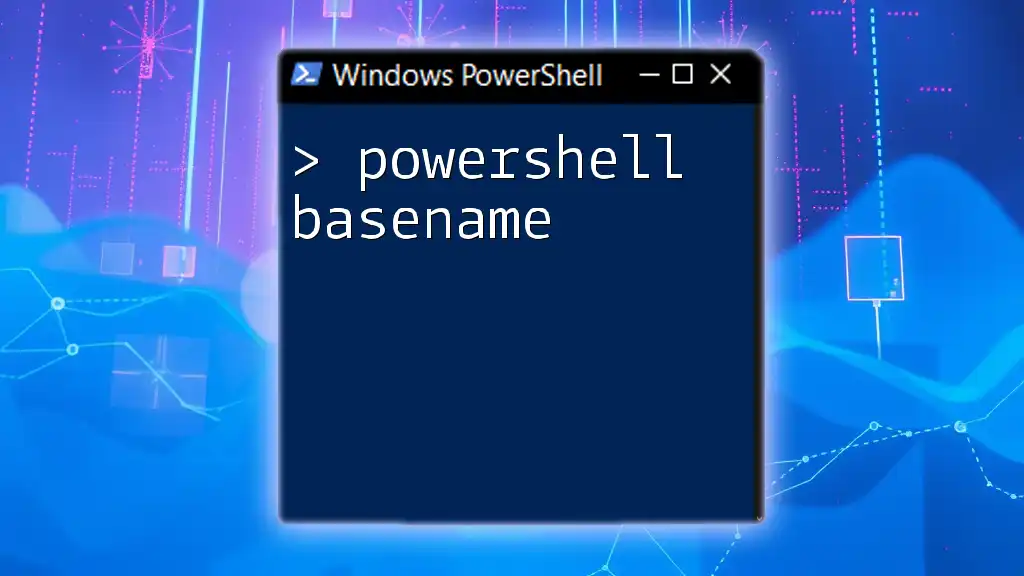
Working with FullName in PowerShell
Getting the FullName of Files
To retrieve the `FullName` of a specific file, you can use the `Get-Item` cmdlet combined with `Select-Object`:
Get-Item "C:\path\to\your\file.txt" | Select-Object FullName
This command fetches the item specified, and the output will be a neatly formatted line showing the `FullName` of the file. Understanding the output is crucial for confirming the file path before proceeding with further operations.
Getting the FullName of Directories
Similarly, you can fetch the `FullName` for directories using the same approach:
Get-Item "C:\path\to\your\directory" | Select-Object FullName
This will display the complete path of the specified directory, ensuring you know exactly where you're working within the file system.
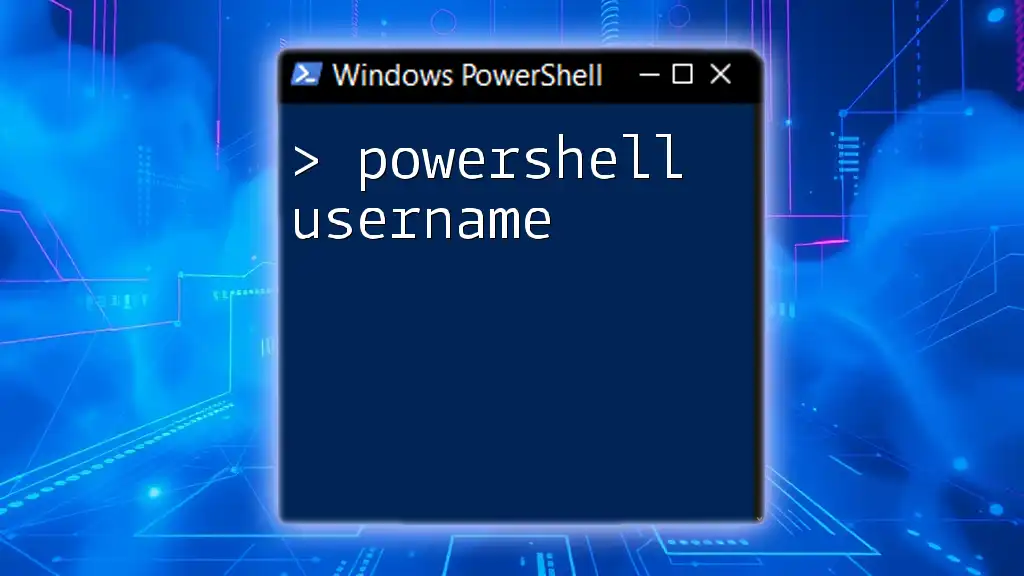
Practical Examples
Example 1: Retrieving FullName for Multiple Files
When you want to list the `FullName` of all `.txt` files in a directory, you can utilize the `Get-ChildItem` cmdlet:
Get-ChildItem "C:\path\to\your\directory\*.txt" | Select-Object FullName
This command retrieves all text files in the given directory, providing their complete paths in a straightforward list. This capability is particularly useful for monitoring or processing a batch of files in scenarios where you expect to handle multiple items.
Example 2: Using FullName in a Script
A practical application of the `FullName` property is in creating a backup script. Here’s a simple example:
$files = Get-ChildItem "C:\path\to\your\directory\*.txt"
foreach ($file in $files) {
Copy-Item -Path $file.FullName -Destination "C:\backup\directory\$($file.Name)"
}
In this script, `Get-ChildItem` retrieves all `.txt` files, and the `foreach` loop uses their `FullName` to copy them to a backup directory. This demonstrates how `FullName` facilitates operational accuracy and eases the coding process.
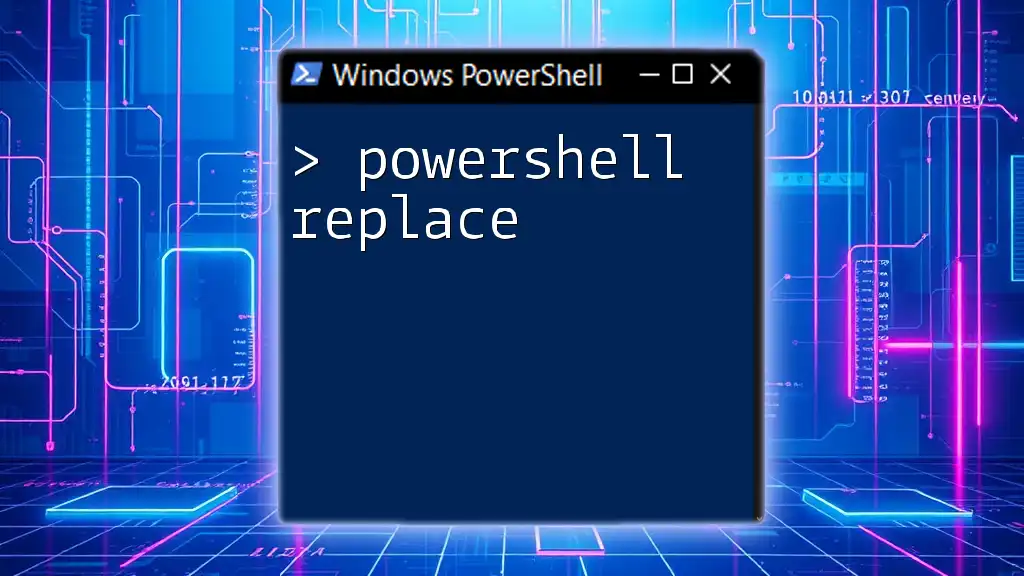
Customizing FullName Output
Formatting the FullName
In some instances, you might want to format or manipulate the `FullName` string. For example, if you want to replace part of the string to make it more user-friendly, you can do the following:
$fullName = (Get-Item "C:\path\to\your\file.txt").FullName
$formattedName = $fullName -replace 'C:\\', 'Drive:\'
This uses a regular expression to substitute "C:\" with "Drive:". Formatting output is beneficial in enhancing readability for users who may not be as familiar with standard file system nomenclature.
Combining FullName with Other Properties
You can also enhance the information presented alongside `FullName`. For instance, retrieving several properties simultaneously can deliver contextual details about the files:
Get-ChildItem | Select-Object FullName, Length, LastWriteTime
This command shows not just the `FullName` but also the file size (`Length`) and the last modified timestamp (`LastWriteTime`). Such comprehensive output can aid in monitoring and auditing tasks by providing essential details at a glance.
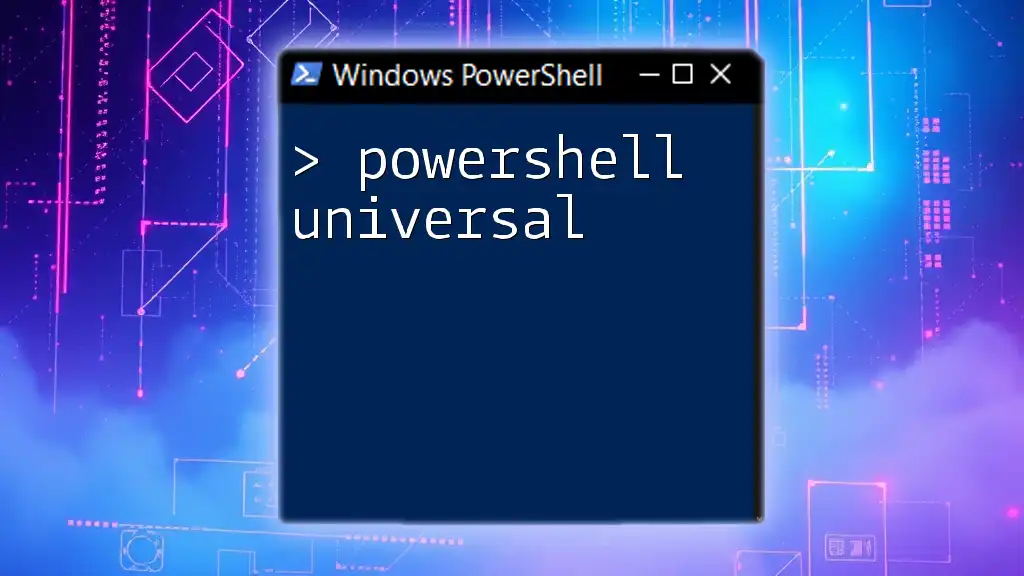
Troubleshooting Common Issues
Errors Related to File Paths
When working with file paths, various common errors can crop up, particularly with incorrect path references leading to failure messages. A typical example is the “File Not Found” error when referencing a non-existent file. Ensure that the path syntax is correct and that the file exists at the specified location.
To handle these errors proactively, you might implement conditional checks in your scripts:
$file = "C:\path\to\your\file.txt"
if (Test-Path $file) {
Write-Host "File exists. FullName: $(Get-Item $file).FullName"
} else {
Write-Host "File does not exist."
}
Cmdlet Limitations
While `FullName` is incredibly useful, it is important to be aware of its limitations in certain cmdlets, or when dealing with network paths, symbolic links, or when the underlying file system does not conform to expected standards. Always verify how your functions behave in various environments to avoid unexpected results.
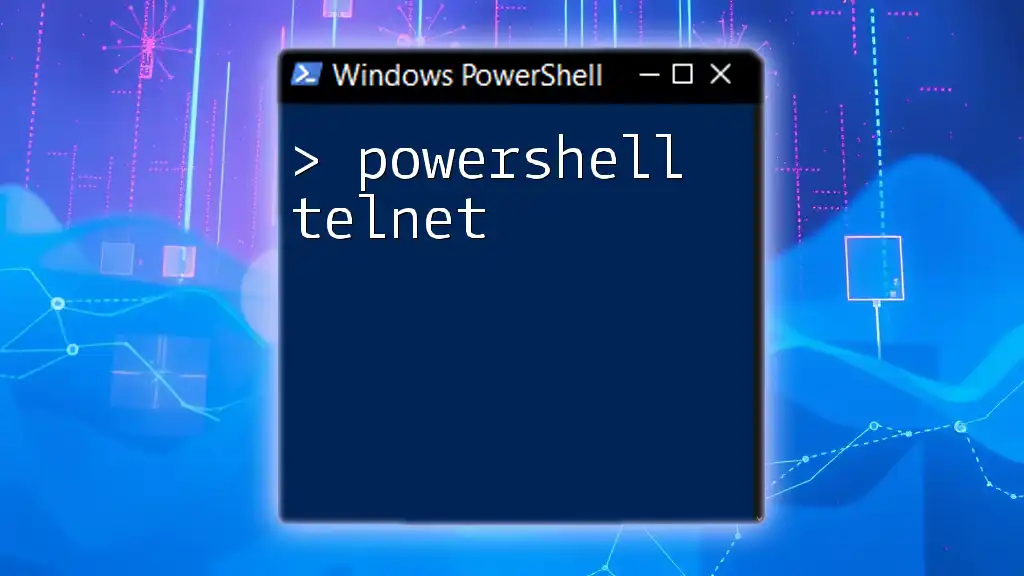
Conclusion
The `FullName` property in PowerShell is a powerful tool that enhances file management and automation capabilities. By understanding and leveraging `FullName`, you can create more efficient and error-free scripts, promoting better management practices in your PowerShell endeavors. Practicing the use of `FullName` in different contexts will not only improve your scripting skills but will also help streamline your workflow in various system administration tasks.
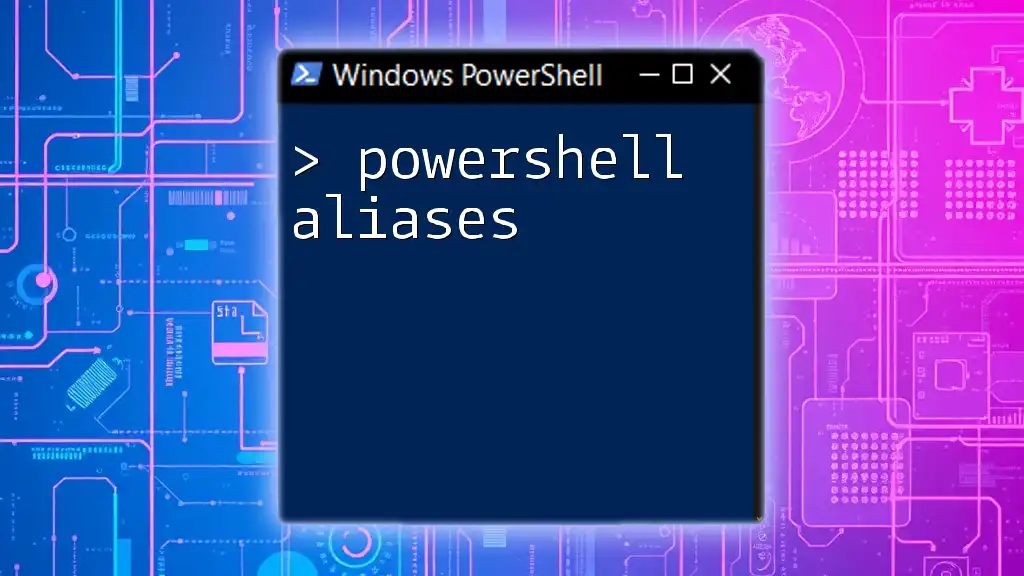
Additional Resources
For further exploration of the `FullName` property and other PowerShell functionalities, consider checking the official PowerShell documentation and following tutorials that dive deeper into practical applications. Engaging with community resources can also provide insights into real-world applications of PowerShell scripts and commands.