Powershell games are interactive scripts that utilize the PowerShell command-line interface to create fun and educational experiences while enhancing users' scripting skills.
Write-Host 'Welcome to the PowerShell Game! Type your answer:'
# Simple guessing game
$secretNumber = Get-Random -Minimum 1 -Maximum 10
$userGuess = Read-Host 'Guess a number between 1 and 10'
if ($userGuess -eq $secretNumber) {
Write-Host 'Congratulations! You guessed it right!'
} else {
Write-Host "Sorry, the correct number was $secretNumber."
}
What are PowerShell Games?
PowerShell games are an innovative way to combine programming with entertainment. They leverage the capabilities of PowerShell as a scripting language to create engaging and interactive experiences. By implementing game mechanics, developers can enhance their understanding of coding, logic, and problem-solving skills. This unique approach allows users to learn while having fun, making programming concepts more accessible and enjoyable.
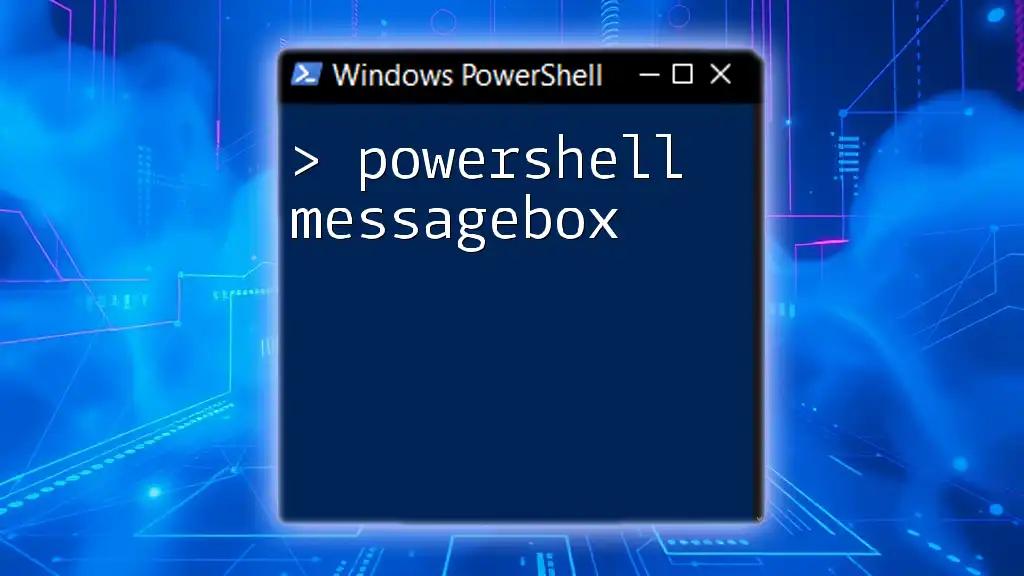
Benefits of Creating Games in PowerShell
Creating games in PowerShell not only sharpens your scripting skills but also encourages critical thinking. When you design a game, you are required to break down complex problems into manageable parts. This process enhances your ability to use syntax, logic, and computing basics effectively. As you work on these projects, you will gain deeper insights into variables, loops, conditions, and functions, making you a more adept programmer.
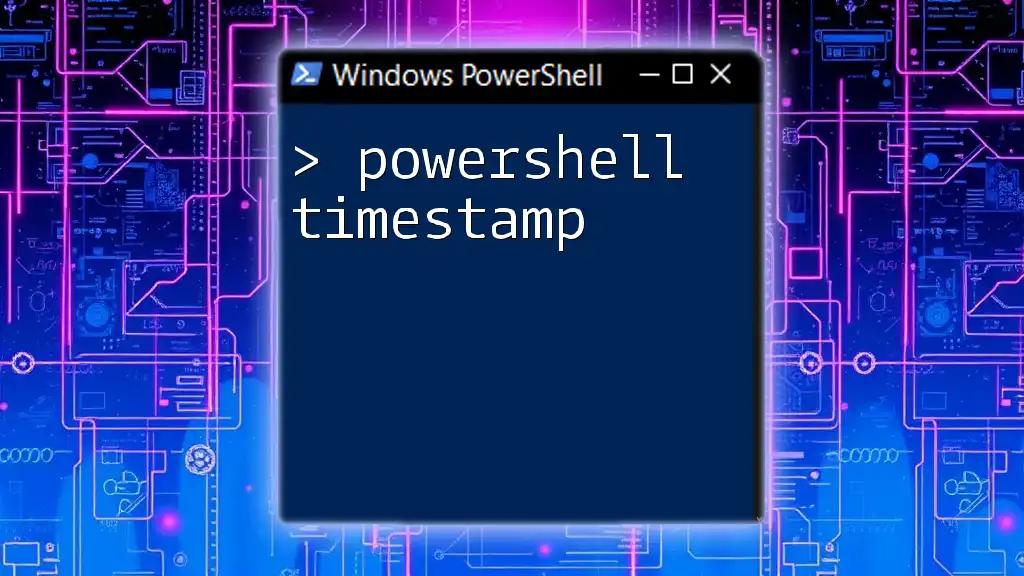
Getting Started with PowerShell
Installing PowerShell
To dive into PowerShell games, you first need to have PowerShell installed on your machine. Historically, you could access Windows PowerShell, but now PowerShell Core offers cross-platform capability, allowing you to work on both Windows and non-Windows systems. Installation is straightforward and can be done via Microsoft's official website.
Basic PowerShell Commands
Understanding the fundamental commands of PowerShell is crucial. Cmdlets are the building blocks of PowerShell. Here are some essential commands you should know:
- Get-Command: Lists all available cmdlets.
- Get-Help: Provides help information about cmdlets.
- Set-Location: Changes the current directory.
Getting comfortable with these commands allows you to manipulate and navigate your environment effectively.
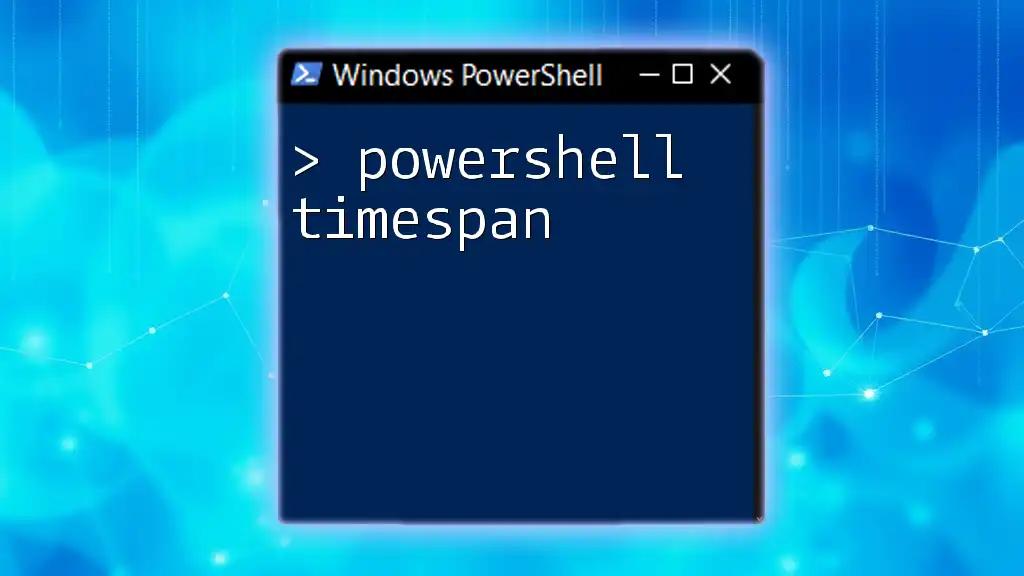
Creating Simple PowerShell Games
Conceptualizing Game Ideas
Before coding, you should brainstorm potential game concepts. Simple game ideas such as Tic-Tac-Toe, Hangman, and a Number Guessing game can serve as great starting points, especially for those new to PowerShell.
Tic-Tac-Toe
Setting Up the Game
Tic-Tac-Toe is a straightforward two-player game that can be played on a 3x3 grid. The goal is to get three marks in a row.
Code Example
Here’s a basic implementation of a Tic-Tac-Toe game in PowerShell:
function Start-TicTacToe {
$board = @(' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ')
$currentPlayer = 'X'
while ($true) {
Display-Board $board
$move = Read-Host "Player $currentPlayer, enter your move (1-9)"
if ($board[$move - 1] -eq ' ') {
$board[$move - 1] = $currentPlayer
if (Check-Winner $board $currentPlayer) {
Display-Board $board
Write-Host "Player $currentPlayer wins!" -ForegroundColor Green
break
}
$currentPlayer = if ($currentPlayer -eq 'X') { 'O' } else { 'X' }
} else {
Write-Host "Invalid move, try again." -ForegroundColor Red
}
}
}
Explanation of the Code
This code initializes a game board using an array, sets the current player, and enters a loop to take player moves. The `Display-Board` function outputs the current state of the game, and `Check-Winner` evaluates whether the current player has won. If the move is valid, the game alternates turns until a winner is found.
Hangman
Setting Up the Game
Hangman is a classic word-guessing game where players try to guess a hidden word one letter at a time. The objective is to reveal the word before too many incorrect guesses are made.
Code Example
Here’s a sample of how to code a Hangman game:
function Start-Hangman {
$word = "POWER"
$guessedLetters = @()
$wrongGuesses = 0
while ($wrongGuesses -lt 6) {
$currentWordState = $word -replace "[^$($guessedLetters -join '')]", '_'
Write-Host "Current word: $currentWordState"
$guess = Read-Host "Enter your guess (a single letter)"
if ($word -contains $guess) {
$guessedLetters += $guess
} else {
$wrongGuesses++
Write-Host "Wrong guess! Attempts left: $((6 - $wrongGuesses))" -ForegroundColor Red
}
if ($currentWordState -eq $word) {
Write-Host "Congratulations, you've guessed the word!" -ForegroundColor Green
break
}
}
}
Explanation of the Code
In this code snippet, the hidden word is stored in a variable. The game enters a loop where the player guesses letters. If the guess is correct, it is added to the list of guessed letters; if not, the wrong guess counter increases. The game continues until the word is fully revealed or six wrong guesses are made.
Number Guessing Game
Setting Up the Game
The Number Guessing game involves players attempting to guess a randomly selected number within a specific range. It is simple yet effective for learning about loops and conditional structures.
Code Example
Here’s an example of a Number Guessing game coded in PowerShell:
function Start-NumberGuessingGame {
$targetNumber = Get-Random -Minimum 1 -Maximum 100
$guess = 0
while ($guess -ne $targetNumber) {
$guess = [int](Read-Host "Guess a number between 1 and 100")
if ($guess -lt $targetNumber) {
Write-Host "Too low!" -ForegroundColor Yellow
} elseif ($guess -gt $targetNumber) {
Write-Host "Too high!" -ForegroundColor Yellow
} else {
Write-Host "Congratulations! You've guessed the number." -ForegroundColor Green
}
}
}
Explanation of the Code
This game generates a random number and prompts the user to guess. It uses conditional statements to provide feedback on whether the guess is too high or too low. The loop continues until the user guesses the correct number.

Enhancing Your PowerShell Games
Adding Difficulty Levels
One way to make your games more engaging is by introducing different difficulty levels. For example, you could let players choose a range for the Number Guessing game that determines potential maximum numbers, or you could limit the number of guesses allowed based on the difficulty.
Creating a Scoring System
A scoring system helps players track their performance over multiple rounds. You could keep a tally of correct answers or the number of attempts taken per game, providing players with insights into their improvement.
User Input Validation
Validation is essential to ensure the game runs smoothly and avoids potential errors. Here’s an example of input validation practice:
do {
$input = Read-Host "Enter your move (1-9)"
} while (-not ($input -match '^[1-9]$'))
This code snippet ensures that the user inputs only valid numerical choices, enhancing the user experience and reducing errors during gameplay.

Advanced PowerShell Gaming Concepts
Using Arrays and Hash Tables
Arrays and hash tables are powerful tools in PowerShell that help manage game states. In Tic-Tac-Toe, for example, you might utilize an array to represent the game board. This allows you to easily access and manipulate each cell within the grid:
$board = @(' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ')
Object-Oriented Programming in PowerShell
PowerShell also supports object-oriented programming (OOP), allowing you to encapsulate game logic within classes. This approach helps to organize your code better. Here’s a brief example of how you could define a class for a Tic-Tac-Toe game:
class TicTacToe {
[string[]]$board = @(' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ')
[void]Display() {
# Code to display the board
}
[bool]CheckWin() {
# Code to check for a winner
}
}
Using classes makes your code modular and reusable, which is a best practice in software development.

Community and Resources
To further your PowerShell gaming projects, explore various community resources. Websites like GitHub harbor a wealth of PowerShell game projects, where you can see real-world applications of concepts learned. Additionally, engaging in forums can spur inspiration and provide support from fellow enthusiasts.
PowerShell Resources for Continuous Learning
Dive deeper into PowerShell with books and online courses, including those offered by your company. As you progress, consider tackling more complex game mechanics or even exploring game development frameworks that could integrate with PowerShell.

Conclusion
Creating PowerShell games is a unique and enjoyable way to enhance programming skills. Whether you’re developing simple games or venturing into object-oriented techniques, the skills obtained through these projects will serve you well in your coding journey. The ability to learn through play offers a robust framework for mastering PowerShell and coding principles. Keep experimenting, keep learning, and most importantly, have fun with your coding projects!
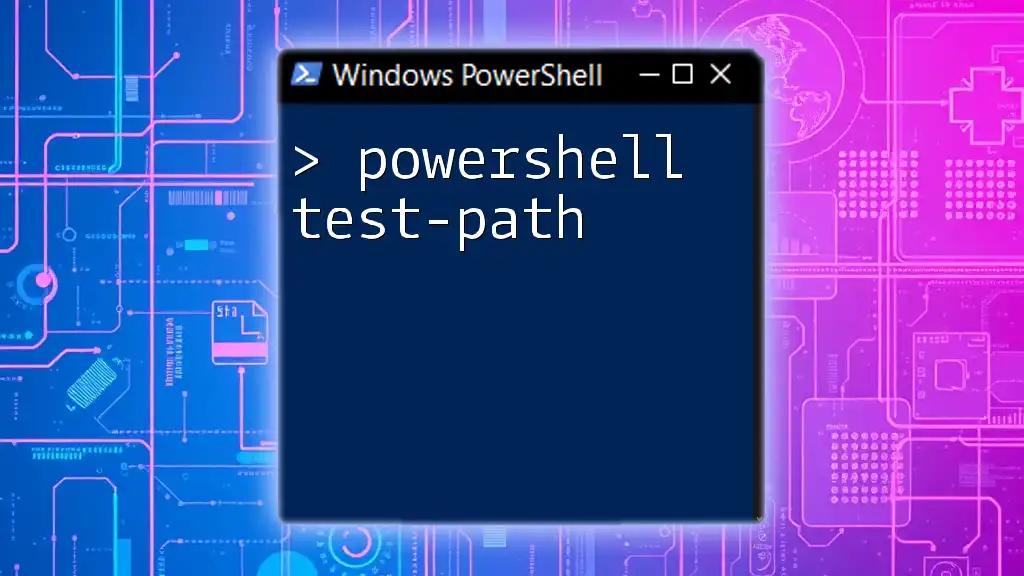
Additional Resources
Here’s a compilation of useful links to kickstart your exploration into PowerShell games:
- GitHub repositories featuring PowerShell games.
- Recommended books on PowerShell and game development tutorials.
By employing this guide, you are well on your way to mastering PowerShell gaming both as a development practice and a fun learning tool.