PowerShell named arguments allow you to specify parameters by name rather than by position, making your code more readable and less prone to errors.
Here’s an example:
Write-Host -Message 'Hello, World!' -ForegroundColor 'Cyan'
Understanding Named Parameters in PowerShell
What are Named Parameters?
Named parameters in PowerShell are specifically designed to improve readability and provide clarity in your scripts and functions. Instead of relying solely on the order of arguments (as with positional parameters), named parameters allow you to specify which argument corresponds to which parameter by using the parameter name directly. This enhances both the usability of scripts and their maintainability over time.
Benefits of Using Named Parameters
Named parameters help make scripts more descriptive and easier to follow. They reduce the chances of mixing up parameter values and improve the overall context when reading or maintaining the code. This is especially useful in scripts or functions that take multiple arguments, as it clarifies the purpose of each argument without requiring the user to remember their order.

Implementing Named Arguments in PowerShell
Basic Syntax and Usage
To use named parameters in a PowerShell function, you will define them within a `param` block. Here’s a simple example:
function Get-Greeting {
param(
[string]$Name,
[int]$Age
)
"Hello, my name is $Name and I am $Age years old."
}
In this example, the function takes two parameters: `$Name` and `$Age`. When calling this function, you would use the named parameters to provide values:
Get-Greeting -Name "Alice" -Age 30
In this case, each parameter is explicitly named, making it clear what values are being passed in.
Combining Named and Positional Parameters
You can also mix named and positional parameters. This can be particularly useful when some parameters are easier to remember or more frequently used than others. Here’s how you can implement mixed parameters:
function Set-User {
param(
[string]$Username,
[string]$Role = "User"
)
"Setting role of $Username to $Role."
}
# Using Positional and Named Parameters
Set-User "Bob" -Role "Admin"
In this example, `Username` is a positional parameter, while `Role` is a named parameter with a default value. When calling `Set-User`, you can provide `Bob` as a positional argument, while explicitly specifying the role.
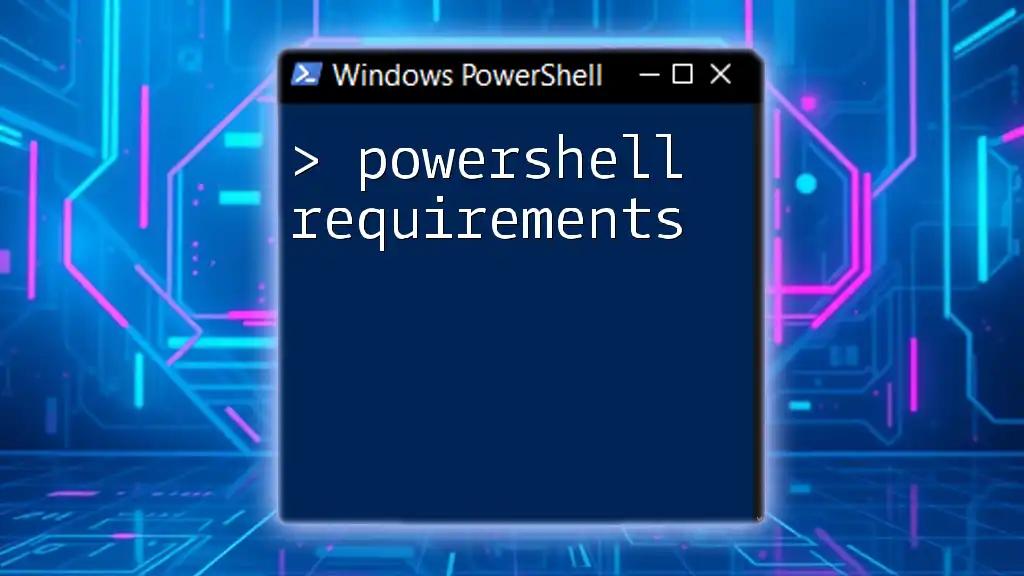
Advanced Usage of Named Parameters
Default Values for Named Parameters
Setting default values for named parameters can save time and streamline function usage. This allows the function to be called without specifying every single parameter. Consider the following function:
function Set-Config {
param(
[string]$ConfigName,
[string]$ConfigValue = "Default"
)
"Config $ConfigName is set to $ConfigValue."
}
If you call the function as follows:
Set-Config -ConfigName "Theme"
The output will say "Config Theme is set to Default." This shows how a default value can simplify function calls and still provide functional flexibility.
Handling Optional Versus Required Parameters
You can also enforce whether parameters are mandatory. This is done by utilizing the `Mandatory` attribute. Consider this example:
function Add-Item {
param(
[Parameter(Mandatory=$true)]
[string]$ItemName,
[string]$ItemType = "General"
)
"Adding $ItemType item: $ItemName."
}
If you run `Add-Item -ItemType "Book"` without specifying `$ItemName`, PowerShell will prompt you for this mandatory parameter. This ensures that critical data is always provided, which enhances the robustness of your functions and scripts.
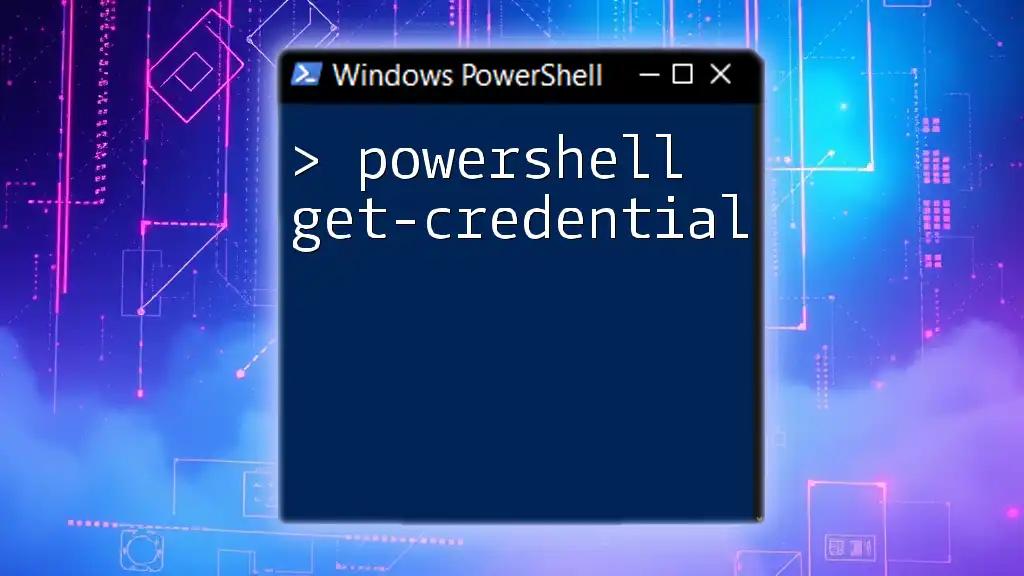
Best Practices for Using Named Arguments
Clarity and Intent
Using named parameters enhances the clarity of your scripts. When others (or future you) come back to the code, they will quickly understand what each parameter does without needing to refer to the function definition. This decreases debugging time and increases overall productivity.
Consistency Across Scripts
Maintaining a consistent approach when using named parameters is crucial, especially in team environments. Standardizing naming conventions and approaches can minimize confusion and mistakes, helping everyone on the team to align with the expected coding standards.
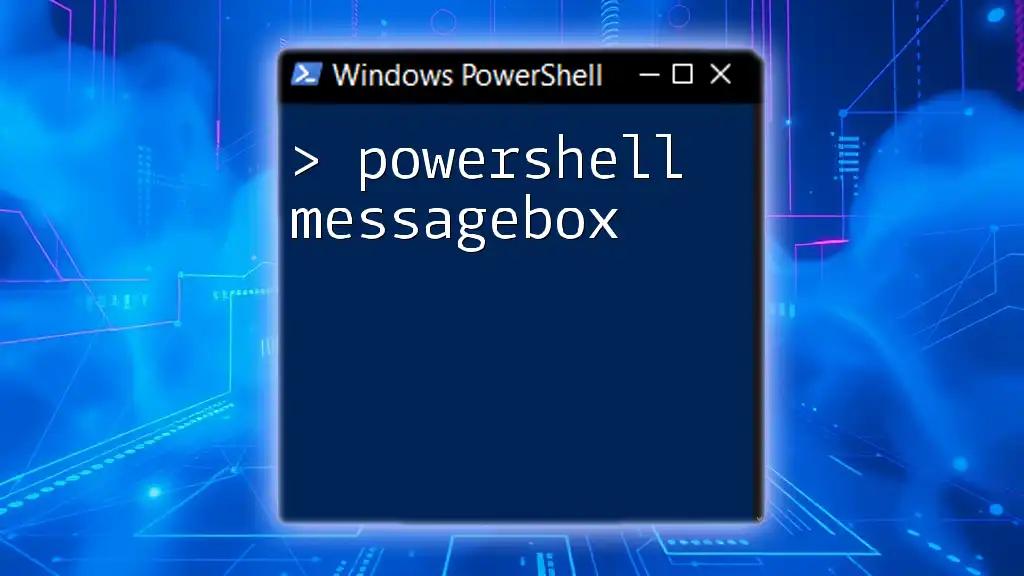
Common Pitfalls to Avoid
Overusing Named Parameters
While named parameters are often helpful, overusing them can lead to clutter. It’s vital to strike a balance. Clarity is essential, but over-complicating function calls with too many named parameters can lead to unnecessary confusion. For instance, consider this bad practice:
Get-Greeting -Name "Alice" -Age 30 -UnusedParam "Value"
Here, the extra `-UnusedParam` serves no purpose, potentially causing distraction and confusion.
Misleading Parameter Names
Parameter names should be meaningful and descriptive. Avoid short, cryptic names that don't reflect the parameter's purpose. For instance, instead of naming a parameter simply `-X`, use `-ExportFormat` to convey its role clearly.
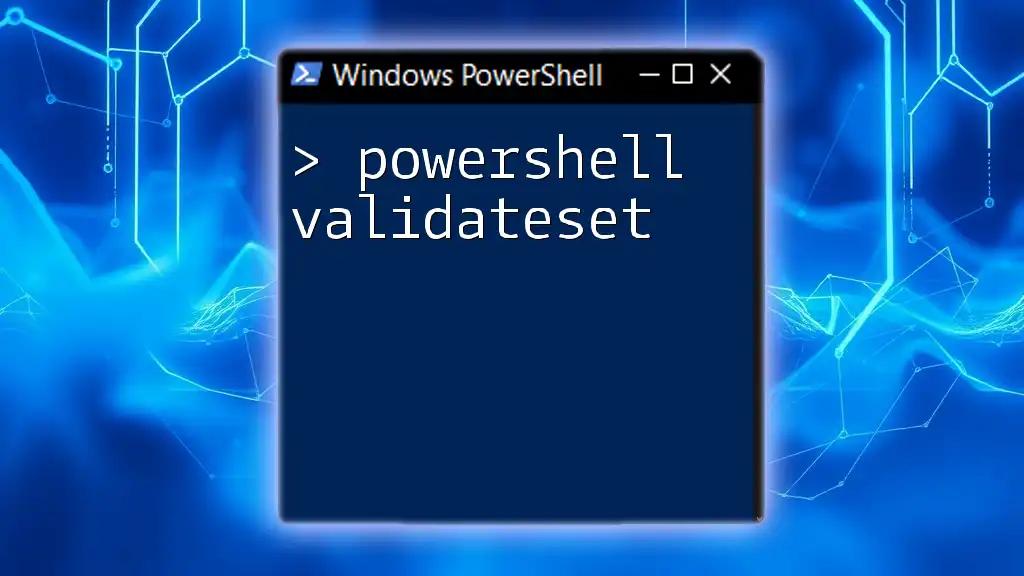
Conclusion
Using PowerShell named arguments is a powerful technique that can significantly improve the readability and maintainability of your scripts. By taking advantage of named parameters, default values, and enforcing mandatory parameters, you can create robust and user-friendly PowerShell functions. Always remember the principles of clarity and consistency, avoiding common pitfalls such as confusing names or unnecessary parameters. Incorporating these practices will not only enhance the quality of your PowerShell scripts but also empower you to write cleaner, more effective code.
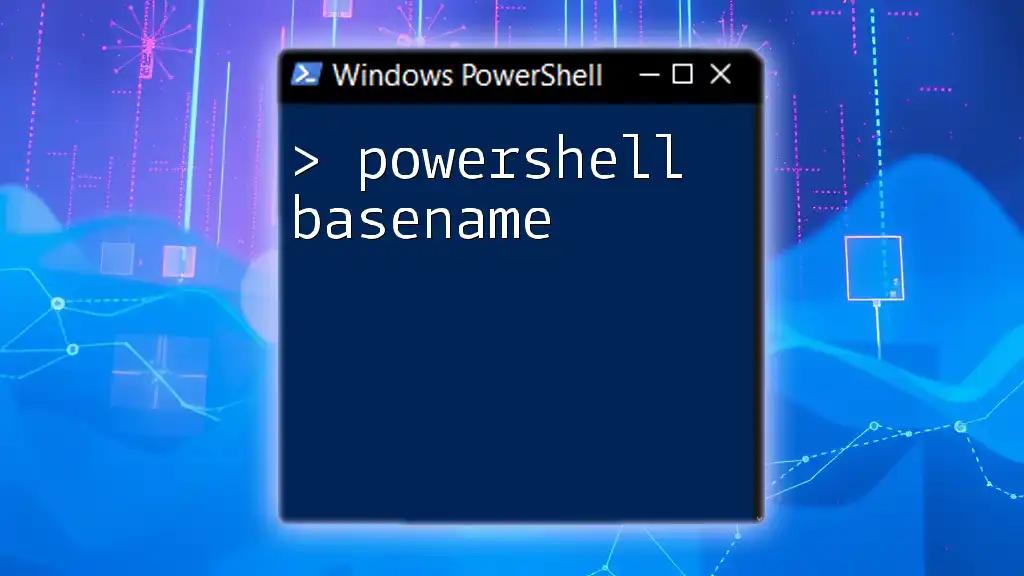
Additional Resources
For more advanced information, it's highly recommended to refer to the official PowerShell documentation and consider additional learning resources that can deepen your understanding of PowerShell concepts and functions.