In PowerShell, naming conventions help improve code readability and maintainability by following specific guidelines for naming variables, functions, and scripts, typically using CamelCase or hyphenated formats.
function Get-UserInfo {
param (
[string]$UserName
)
Write-Host "Fetching information for $UserName"
}
What is a Naming Convention?
A naming convention refers to a set of rules and guidelines that dictate the names of variables, functions, parameters, and other entities in programming, particularly within PowerShell scripts. The primary purpose of these conventions is to promote clarity and consistency in code, making it easier for developers to understand, maintain, and collaborate on scripts.
When followed meticulously, naming conventions facilitate code sharing, allowing teams to work together without ambiguity. They improve code readability, which is critical when revisiting scripts after a long interval or when handed off to another developer.
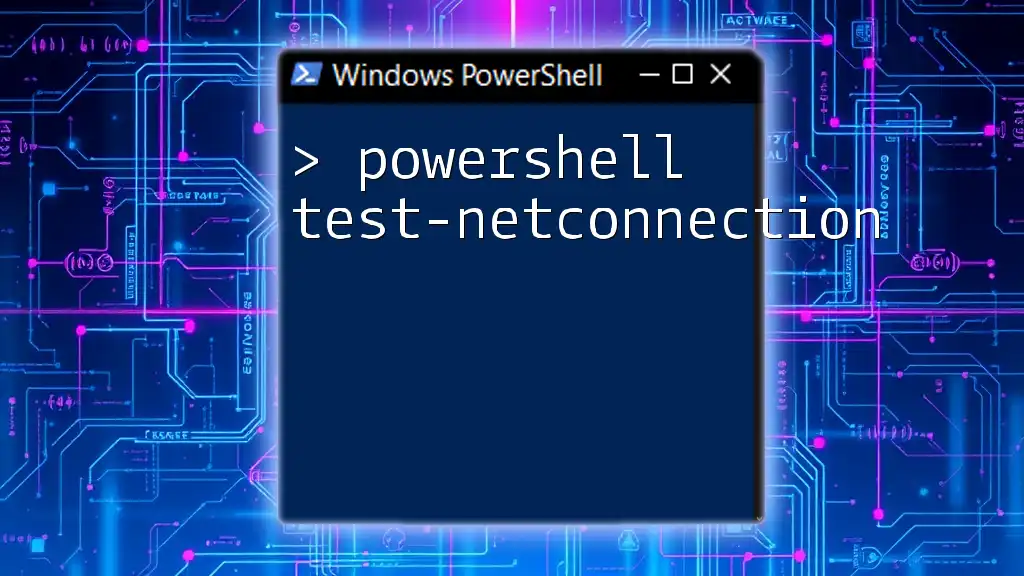
General Guidelines for Naming Conventions
Consistency is Key
One of the fundamental principles is consistency. Consistently employing a naming convention throughout your scripts ensures that every developer on your team can quickly comprehend the code without needing to decode various naming styles.
For example, consider a scenario where variables are inconsistently named:
$username = "Alice"
$userAge = 25
userType = "Admin"
In the snippet above, we see a mix of naming styles. Instead, using a consistent nomenclature enhances readability:
$userName = "Alice"
$userAge = 25
$userType = "Admin"
Meaningfulness in Names
Names should be meaningful and descriptive, providing clarity about the purpose or content they represent. Unambiguous names enhance understanding, while vague names can lead to confusion and ambiguity in code behavior.
Consider the difference between these two variable names:
- Vague: `$x`
- Descriptive: `$userCount`
The second example explicitly indicates what the variable holds, increasing the comprehension of the code even without context.
Differentiating Between Case Styles
PowerShell supports various case styles, including CamelCase, snake_case, and PascalCase. Each serves different purposes:
- CamelCase: Initial lowercase and then uppercase (e.g., `userAccount`)
- snake_case: Lowercase letters separated by underscores (e.g., `user_account`)
- PascalCase: Each word starts with an uppercase letter (e.g., `UserAccount`)
In PowerShell, PascalCase is predominantly used for function names and cmdlets, while CamelCase is often applied for variable names.
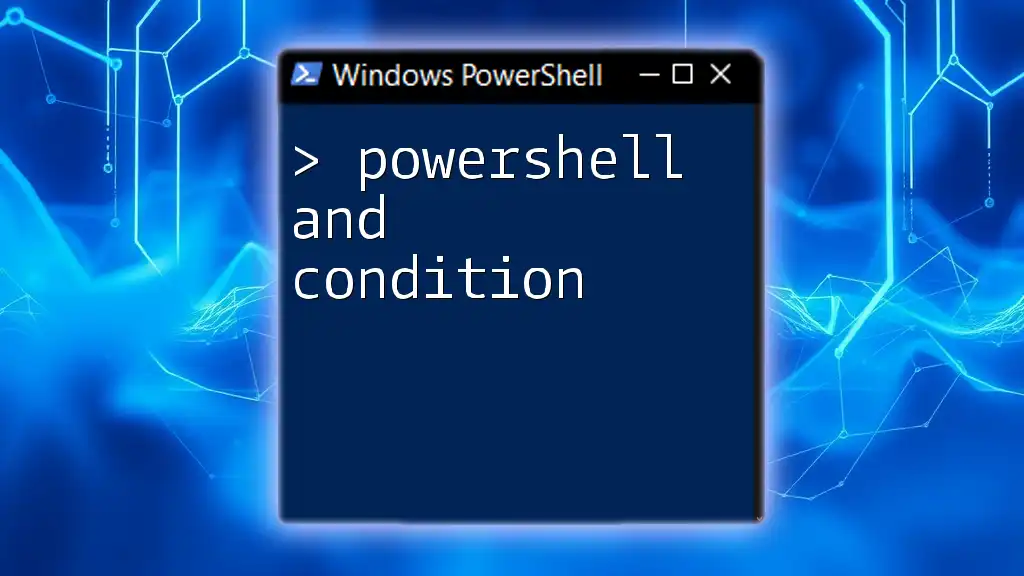
Naming Variables
Guidelines for Variable Names
When naming variables, follow these guidelines for optimal clarity:
- Use meaningful prefixes: This aids in understanding the nature of the variable. For example, a variable that holds user information might be prefixed with `$user`, resulting in names like `$userFirstName`, `$userEmail`, and `$userID`.
- Singular vs. plural: Use singular nouns for variables that hold a single value, and plural for collections. This helps in understanding the data type at a glance.
Example:
$userList = @("Alice", "Bob", "Charlie")
$userCount = $userList.Count
Avoiding Common Pitfalls
Avoid using single-character names like `$x` and `$y`, which provide no context for what they are intended to represent. These names offer little to no clarity, leading to confusion over their purpose when revisiting the code later.
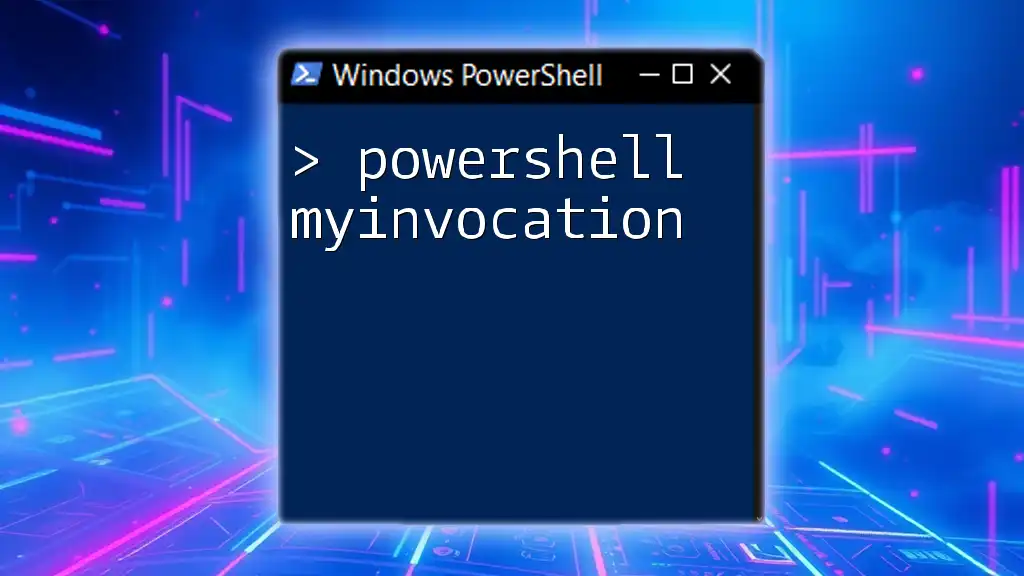
Naming Functions and Cmdlets
Crafting Descriptive Function Names
PowerShell encourages using a Verb-Noun pairing for naming functions. This structure clarifies the purpose of the function while adhering to PowerShell's inherent command structure.
For example:
Function Get-UserData {
# Function logic here
}
Understanding PowerShell Verb-Noun Pairs
PowerShell has a predefined list of approved verbs you should use while naming your functions. Adhering to these verbs not only ensures consistency but also improves the discoverability of your functions.
For instance, typical verbs like `Get`, `Set`, `Remove`, and `New` denote specific operations. So instead of naming a function `FetchUser`, rename it to `Get-User` to align with PowerShell standards.
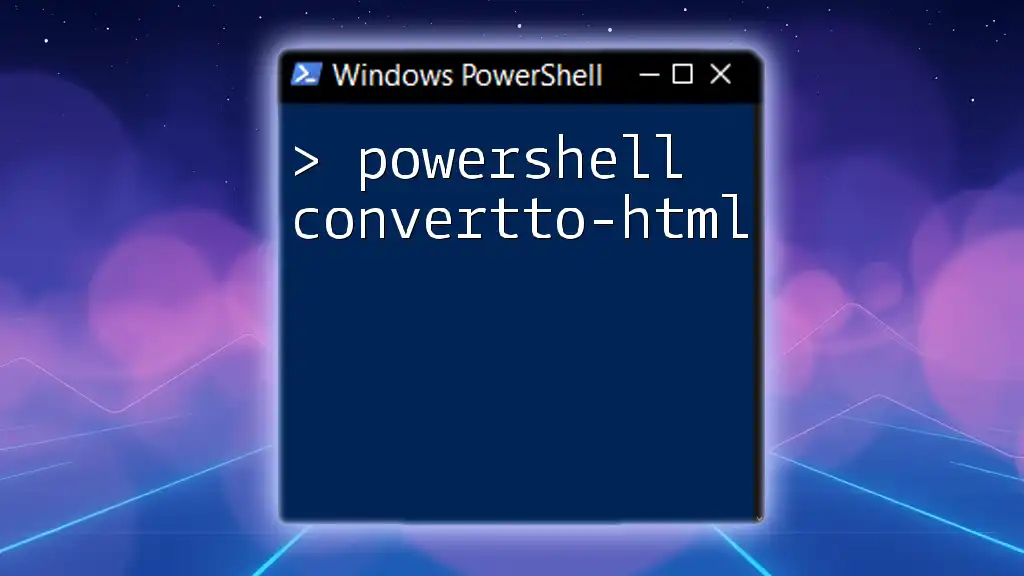
Modules and Script Naming
Naming Files and Modules
When naming your files and modules, clarity is essential. You should aim for names that succinctly represent the module's functionality. This makes it easy for others to pick up and understand the module’s purpose immediately.
Structure of Module Names
Consider structuring your module names in a way that reflects both its functionality and type. A recommended pattern is `ModuleName.ModuleType - Functionality`.
Example:
Module names such as `Networking.Utilities - NetworkConfig.psm1` provide insight into their content and type, aiding both you and other developers.
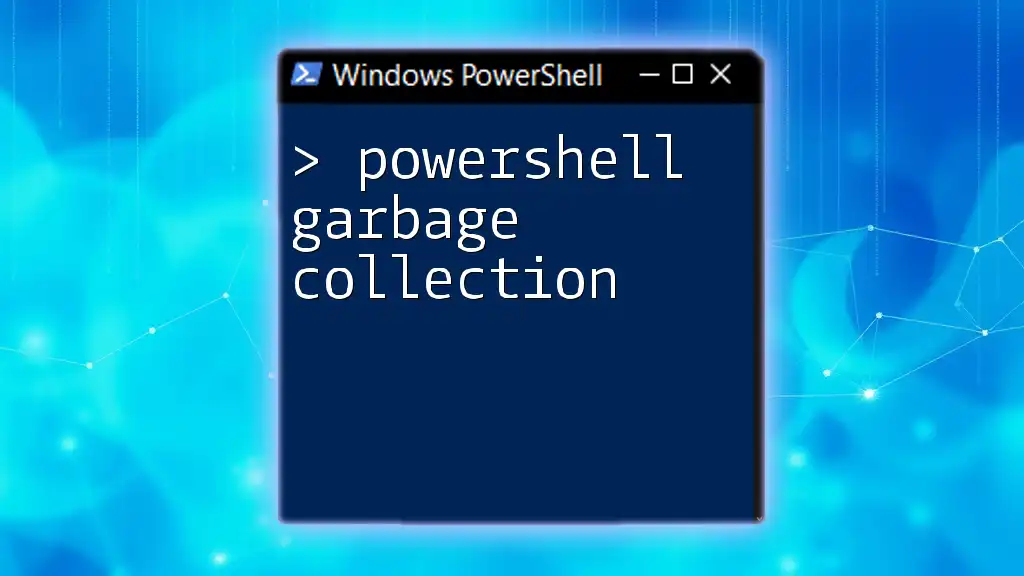
Naming Parameters
Crafting Clear Parameter Names
Parameter names should be self-explanatory. They should clarify their purpose, helping other developers understand what type of input is expected without further documentation.
For example:
Param (
[string]$ServerName,
[int]$Port
)
In this case, both `$ServerName` and `$Port` are clear and indicate exactly what the function expects.
Using Attributes for Enhanced Parameter Clarity
In PowerShell, you have the ability to enhance parameter definitions using attributes. This improves clarity and usability by specifying constraints and requirements.
Example:
Param (
[Parameter(Mandatory)]
[string]$UserName,
[ValidateSet("Admin", "User", "Guest")]
[string]$UserType
)
Here, using the `[Parameter(Mandatory)]` attribute indicates that `$UserName` is required, while `[ValidateSet()]` limits the values for `$UserType` to a defined set, improving both usability and robustness.
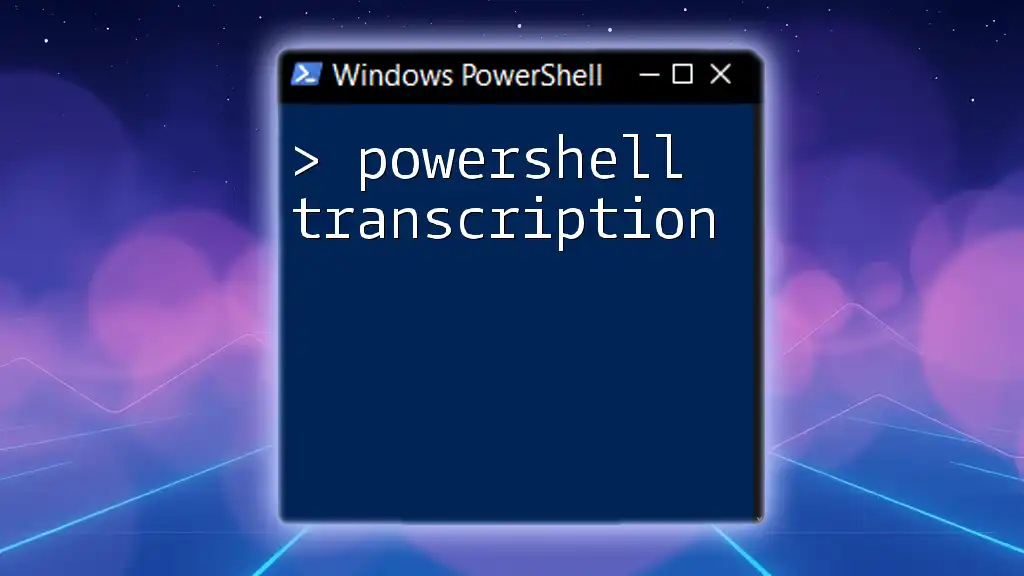
Commenting and Documentation
Importance of Comments
Commenting is essential for documenting your code. Thoughtful comments clarify your intentions, making it easier for others (and future you) to understand the logic and flow. Good comments bridge the gap between code and human understanding.
Use comments to describe complex logic or your thought process, especially in areas that might not be immediately clear.
Using Comment-Based Help
PowerShell supports comment-based help, which allows you to document your functions directly in the code. This is a valuable practice, especially for scripts that others will use. Using this method ensures that help documentation is always up-to-date and relevant.
Example:
<#
.SYNOPSIS
This function retrieves user data.
.PARAMETER UserName
The name of the user to retrieve data for.
#>
Function Get-UserData {
param (
[string]$UserName
)
# Logic to retrieve user data
}
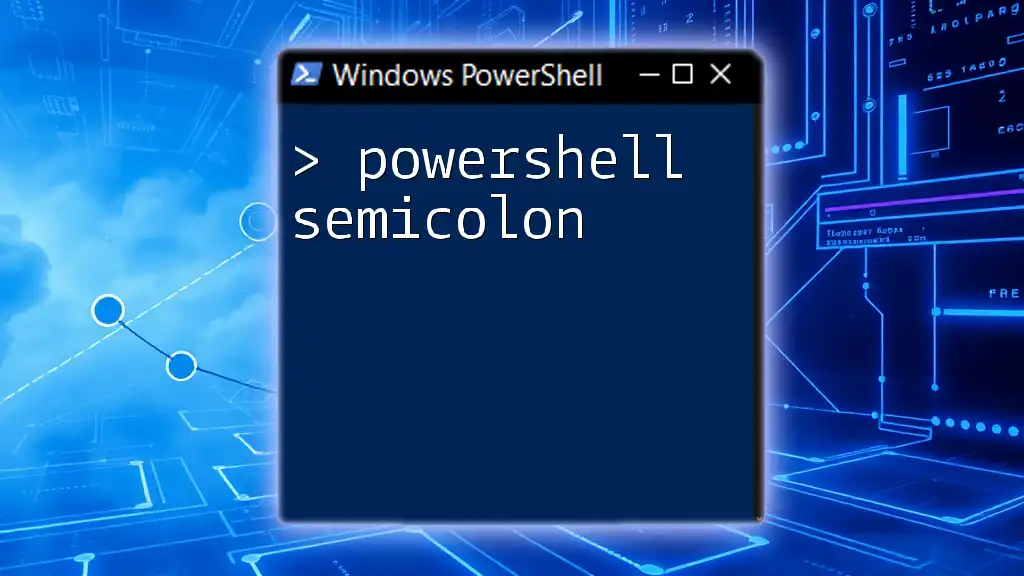
Conclusion
In summary, PowerShell naming conventions are a crucial aspect of writing clear, maintainable scripts. By adhering to a consistent style, using meaningful names, and following established guidelines for naming variables, functions, parameters, and modules, you can significantly improve the readability and usability of your scripts.
Adopting these naming practices not only benefits your own coding experience but also enhances collaboration within teams. Clear code leads to better understanding, easier maintenance, and overall improved software development processes.
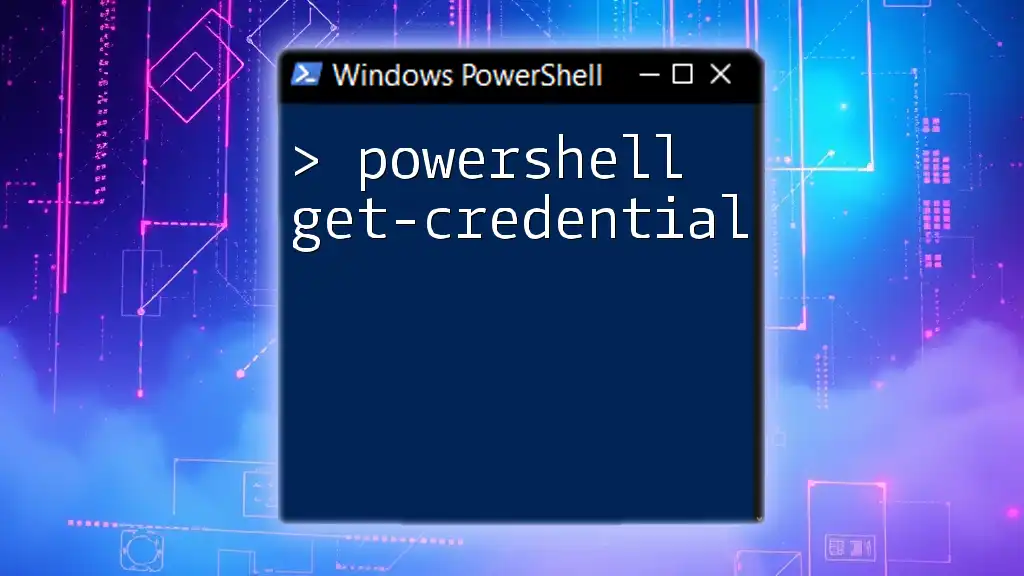
Additional Resources
For further learning, consider checking out official PowerShell documentation regarding naming conventions. There are also countless online courses and books available that delve deeper into PowerShell scripting best practices. Embracing these resources will aid you on your journey to becoming a more proficient PowerShell developer.