PowerShell conditionals allow users to execute commands based on specified criteria, enabling dynamic and flexible automation scripts.
if ($a -gt $b) { Write-Host "$a is greater than $b" } else { Write-Host "$a is not greater than $b" }
What are Conditions in PowerShell?
In PowerShell, conditions play a crucial role in determining how scripts behave and make decisions based on specific input. A condition allows you to branch your script's logic, enabling the script to execute different blocks of code depending on the truth value of the conditions evaluated. This helps create dynamic and responsive scripts that can adapt to the data they process.
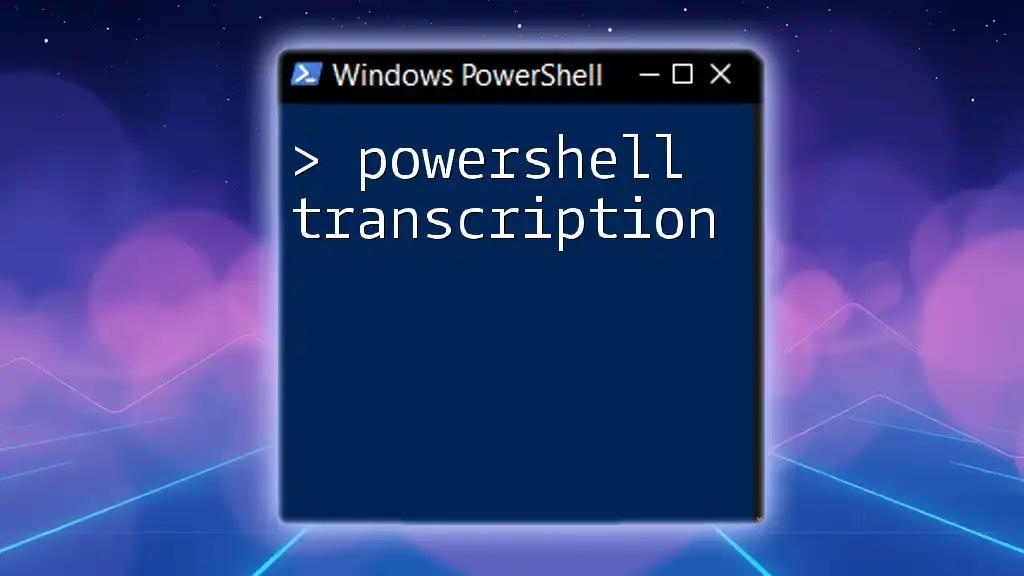
Understanding Conditional Operators in PowerShell
What are Conditional Operators?
Conditional operators are symbols that perform a comparison between values. They allow you to create expressions that return a Boolean result (`$true` or `$false`). Understanding these operators is essential for implementing effective decision-making in your PowerShell scripts.
Common Conditional Operators
PowerShell provides several conditional operators that you can use to control the flow of your scripts:
- Equal (`-eq`): Tests if two values are equal.
- Not Equal (`-ne`): Tests if two values are not equal.
- Greater Than (`-gt`): Tests if one value is greater than another.
- Less Than (`-lt`): Tests if one value is less than another.
- Greater Than or Equal (`-ge`): Tests if one value is greater than or equal to another.
- Less Than or Equal (`-le`): Tests if one value is less than or equal to another.
Examples of Conditional Operators
To see how conditional operators work, consider the following examples:
Using Equal Operator:
$a = 5
$b = 5
if ($a -eq $b) {
"Both values are equal."
}
In this example, since both `$a` and `$b` are equal, the output will be "Both values are equal."
Using Not Equal Operator:
$a = 3
$b = 4
if ($a -ne $b) {
"The values are not equal."
}
Here, the result will be "The values are not equal" because `$a` is not equal to `$b`.
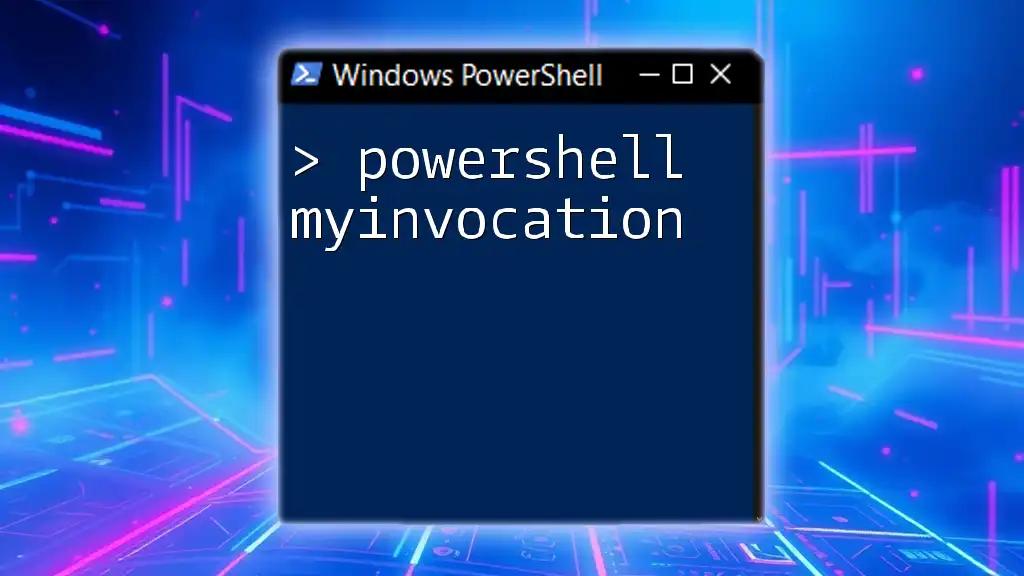
The Power of PowerShell If Statement
Understanding the If Statement
The `if` statement is fundamental in PowerShell for executing code based on whether a condition evaluates to `$true`. The basic syntax follows:
if (condition) {
# statements to execute if condition is true
}
Structure of an If Statement
Simple If Example
A straightforward implementation of an `if` statement looks like this:
$age = 18
if ($age -ge 18) {
"You are an adult."
}
In this case, since `$age` is 18, the output will be "You are an adult."
Elaborating with ElseIf and Else
The `elseif` and `else` constructs allow for further extensions of logical paths in your scripts.
Complex Example: Multi-Condition Logic
$temperature = 30
if ($temperature -lt 0) {
"It's freezing!"
} elseif ($temperature -lt 20) {
"It's cold."
} else {
"It's warm."
}
This example demonstrates how different outputs can be generated based on multiple conditions being evaluated.
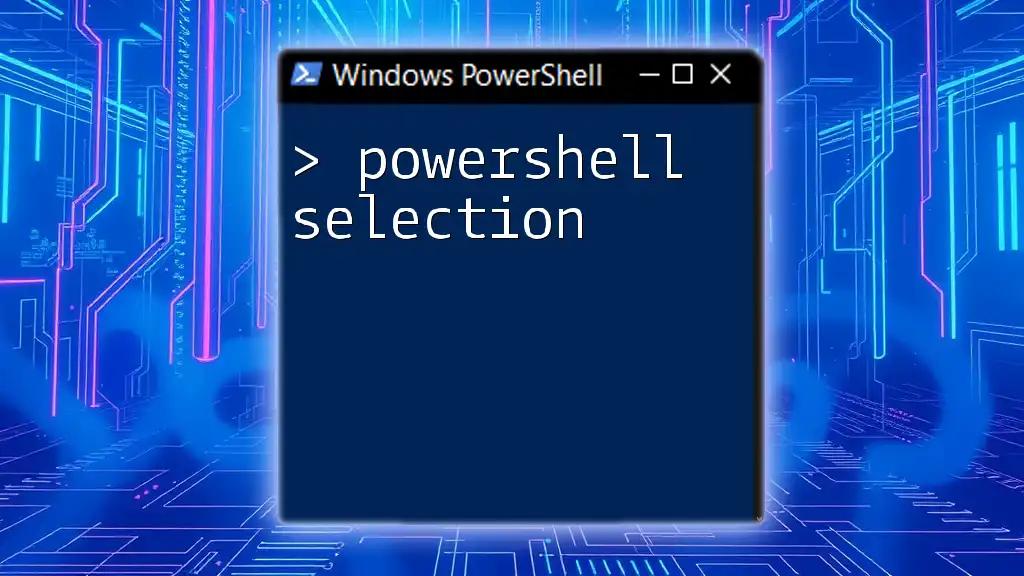
Using the AND Operator in PowerShell
What is the And Operator?
The `-and` operator is used to combine two or more conditions. If all conditions combined with `-and` evaluate to `$true`, then the entire expression returns `$true`.
Examples of the AND Operator
Here's an example that highlights how to use the `-and` operator effectively:
Basic Use of AND:
$a = 5
$b = 10
if ($a -lt 10 -and $b -gt 5) {
"Both conditions are true."
}
In this snippet, since both conditions are satisfied (`$a` is less than 10 and `$b` is greater than 5), the output will be "Both conditions are true."
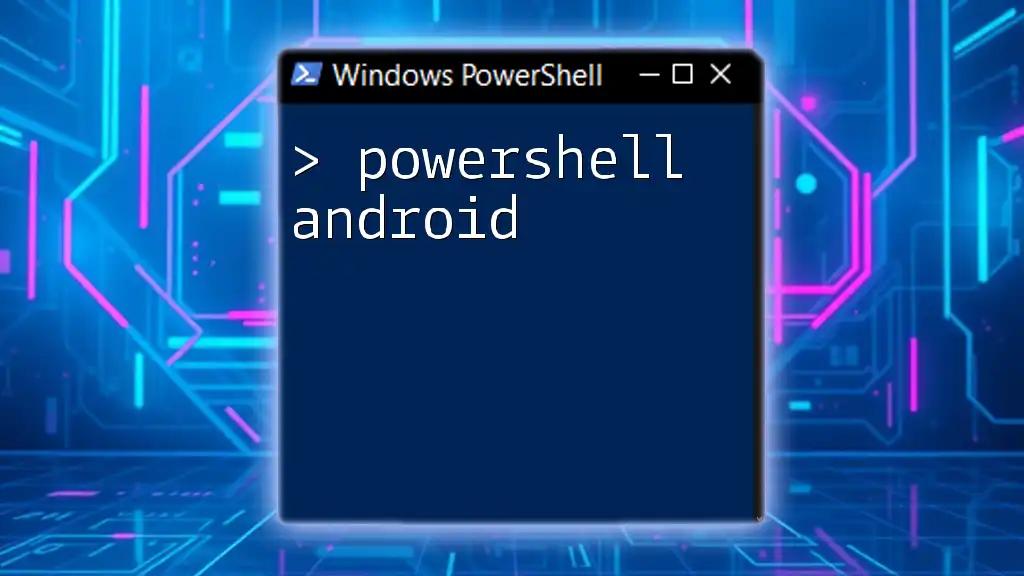
Harnessing the Power of Switch Statement
Introduction to Switch Statement
The `switch` statement in PowerShell is an alternative way to evaluate multiple conditions against a single variable. It simplifies the logic and improves readability, especially when dealing with numerous possible values.
Example of a Switch Statement
Here's an example of using a `switch` statement:
$fruit = 'Apple'
switch ($fruit) {
'Apple' { "Red fruit" }
'Banana' { "Yellow fruit" }
'Grapes' { "Purple fruit" }
default { "Unknown fruit" }
}
In this example, the output will be "Red fruit" since the `$fruit` variable matches the case for 'Apple'.
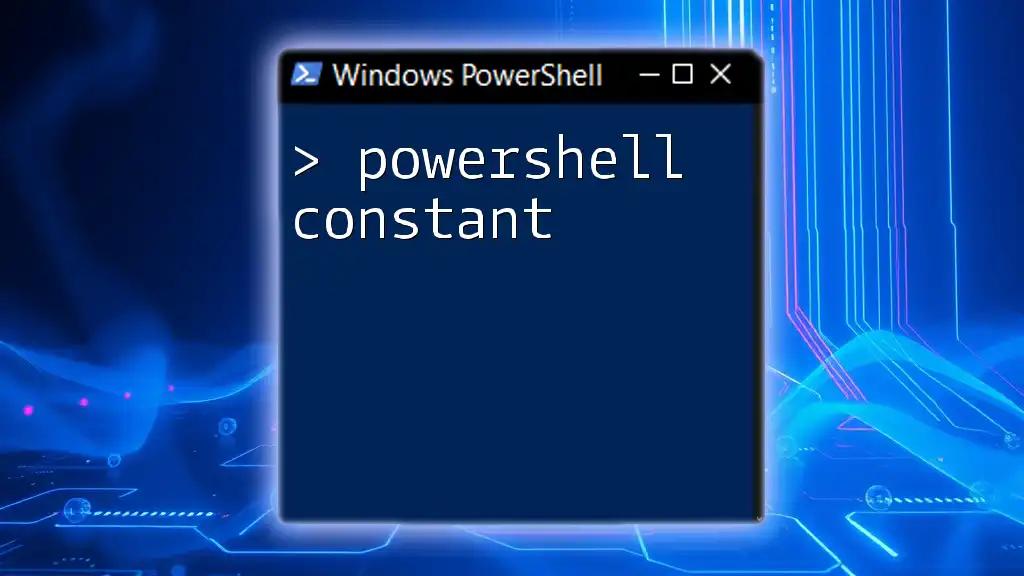
Combining Conditional Logic in PowerShell Scripts
Complex Condition Scenarios
Combining multiple conditions can lead to more sophisticated logic in scripts. You can nest conditional statements or use logical operators to create complex decision structures.
Example: Nested Conditions
$score = 85
if ($score -ge 90) {
"Grade: A"
} elseif ($score -ge 80) {
if ($score -ge 85) {
"Grade: A-"
} else {
"Grade: B+"
}
}
This nested condition provides detailed grading based on the value of `$score`, showcasing the flexibility of conditional logic.

Best Practices for Using Conditions in PowerShell
- Clear and Concise Code: Always strive for clarity. Complex conditions can be split into smaller parts for better readability.
- Use Meaningful Variable Names: Choose variable names that convey their purpose, making your code self-documenting.
- Limit Nesting Depth: Too much nesting can lead to confusion. If possible, look for ways to simplify conditions or break them into functions.
- Test Conditions Thoroughly: Always test edge cases to ensure your conditions handle all potential input scenarios.
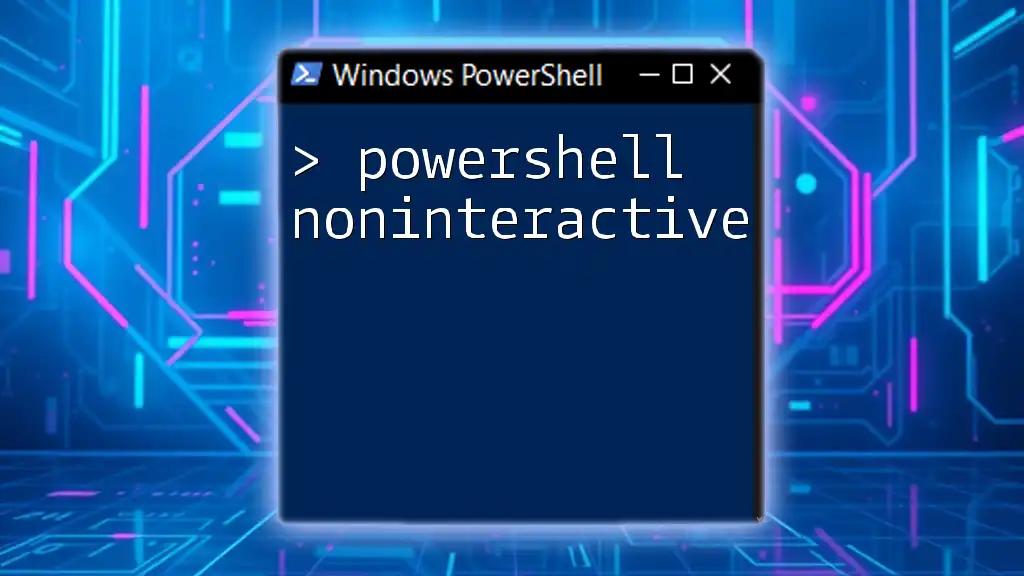
Conclusion
Understanding how to use conditions in PowerShell is essential for effective scripting. By mastering conditional operators, `if` statements, and combining logic, you empower yourself to write robust scripts that can handle a variety of scenarios dynamically.
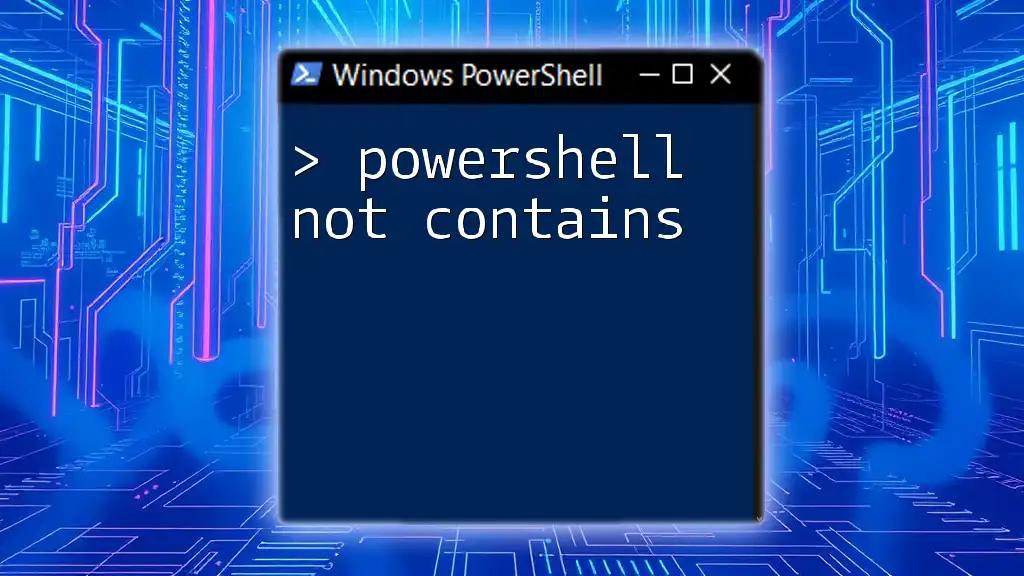
Additional Resources
For further exploration of PowerShell's powerful capabilities, consult the official PowerShell documentation or consider enrolling in PowerShell programming tutorials that delve deeper into scripting techniques and best practices.