In PowerShell, the `-notcontains` operator is used to determine if a collection does not include a specified value, returning `True` if the value is absent.
Here's a code snippet demonstrating its use:
$fruits = @('apple', 'banana', 'cherry')
if ('grape' -notcontains $fruits) {
Write-Host 'Grape is not in the fruit list.'
}
Understanding the Basics: PowerShell Contains and Not Contains
What Does "Contains" Mean in PowerShell?
In PowerShell, the `-contains` operator is used to determine whether a collection (like an array) includes a specific item. This operator is particularly handy when you want to assess membership within a collection. For instance, if you have an array of fruits and wish to see if "orange" exists among them, you would use the `-contains` operator.
The Concept of "Not Contains" in PowerShell
Complementing the `-contains` operator is the `-notcontains` operator, which checks if a specified item is not present in a collection. This operator is crucial for scenarios where you need to filter out items or verify absence, supporting more robust decision-making in scripts.

Syntax and Usage of PowerShell Not Contains
Basic Syntax of the Not Contains Operator
The syntax for using `-notcontains` is straightforward. Here’s how it looks:
$array -notcontains $value
In this syntax:
- `$array` represents the collection you are checking against.
- `$value` is the item you want to verify is not in the array.
Common Use Cases of Not Contains
Filtering Elements in Arrays
A common use of the `-notcontains` operator is to filter elements in an array. For example, if you have a list of fruits:
$fruits = @('apple', 'banana', 'cherry')
$exists = $fruits -notcontains 'orange' # Returns true
Here, `$exists` evaluates to `true` because "orange" is not part of the `$fruits` array. This functionality is fundamental for checks in scripts that need to ensure specific conditions before proceeding.
Checking String Values
The `-notcontains` operator can also be applied to strings, validating if a substring is absent. For instance:
$string = "Hello, PowerShell"
$exists = $string -notcontains "World" # Returns true
In this case, `$exists` is `true` as "World" does not appear in the `$string`. This operator can be useful for condition checks within your PowerShell scripts, allowing customized responses based on string content.

Advanced Examples of Not Contains
Nested Arrays
The complexity of data structures in PowerShell can make using `-notcontains` particularly interesting. Consider an example with nested arrays:
$nestedArray = @(@('apple', 'banana'), @('cherry', 'date'))
$result = $nestedArray[0] -notcontains 'pear' # Returns true
In this scenario, we're checking the first nested array to see if "pear" is present. The result is `true`, demonstrating the utility of `-notcontains` even in more complex data structures like nested arrays.
Using Not Contains with Collections and Custom Objects
When working with collections of custom objects, `-notcontains` remains powerful. Let's assume you have a collection of people represented as custom objects:
$people = @(
[PSCustomObject]@{Name = 'John'; Age = 30},
[PSCustomObject]@{Name = 'Jane'; Age = 25}
)
$exists = $people -notcontains 'John' # Returns true
In this case, `$exists` returns `true`, as 'John' is not being assessed as an entire object. To accurately check against properties, you'd need to filter based on those properties, showcasing how `-notcontains` can still facilitate effective checks against complex data structures.

Debugging and Troubleshooting Not Contains in PowerShell
Common Mistakes and Misunderstandings
Despite its utility, using `-notcontains` can lead to mistakes. Here are some common pitfalls to watch for:
- Comparing Incompatible Types: Ensure that the types you are comparing using `-notcontains` are compatible.
- Confusing Strings and Arrays: Strings are treated differently from arrays. The `-notcontains` operator works with arrays, and attempting to use it on strings may lead to unexpected results.
Best Practices for Effective Use of Not Contains
To maximize the functionality of `-notcontains`, consider these best practices:
- Validate Data Types: Before performing a `-notcontains` check, ensure that the data types of the items being compared are aligned.
- Use Clear Statements: Avoid convoluted conditions for clarity. Make your code easy to read for future reference.
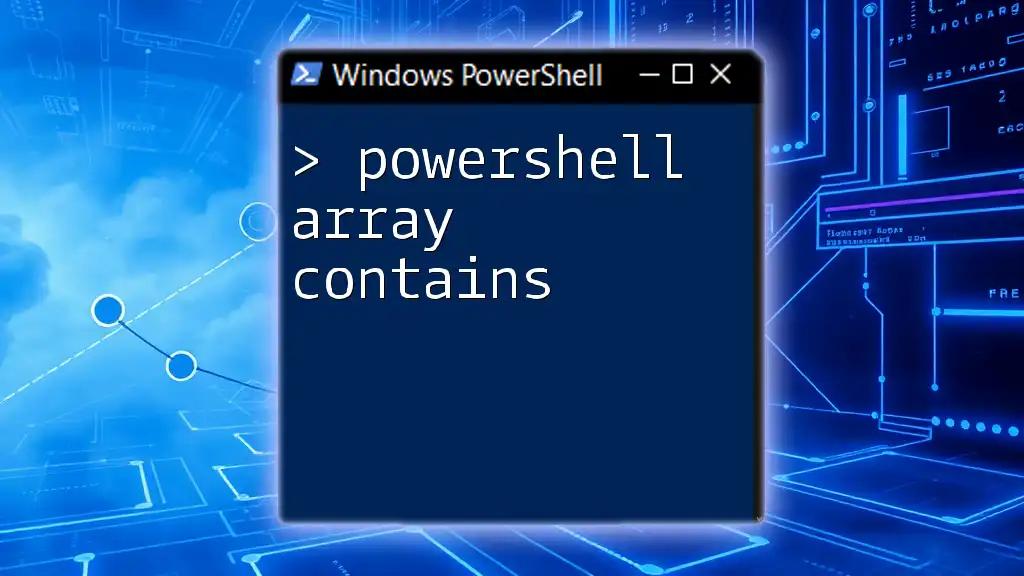
Real-World Applications of Not Contains in PowerShell Scripts
Sample Script: Identifying Missing Users in an Array
One of the most practical applications of `-notcontains` is identifying missing values from a dataset. Here’s a complete script exhibiting this use case:
$expectedUsers = @('Alice', 'Bob', 'Charlie')
$currentUsers = @('Alice', 'Charlie')
$missingUsers = $expectedUsers | Where-Object { $_ -notcontains $currentUsers }
In this example, we want to find out which users are missing from the current list compared to the expected list. The `$missingUsers` variable will yield users who are not found in the `$currentUsers`, helping in user management and administration tasks.
Performance Considerations
When utilizing `-notcontains`, especially with large datasets, it's crucial to consider performance. The complexity increases as data size grows, as PowerShell has to evaluate each item for presence or absence. Testing and timing these checks in production environments will provide insight into performance impacts and allow for optimization when necessary.
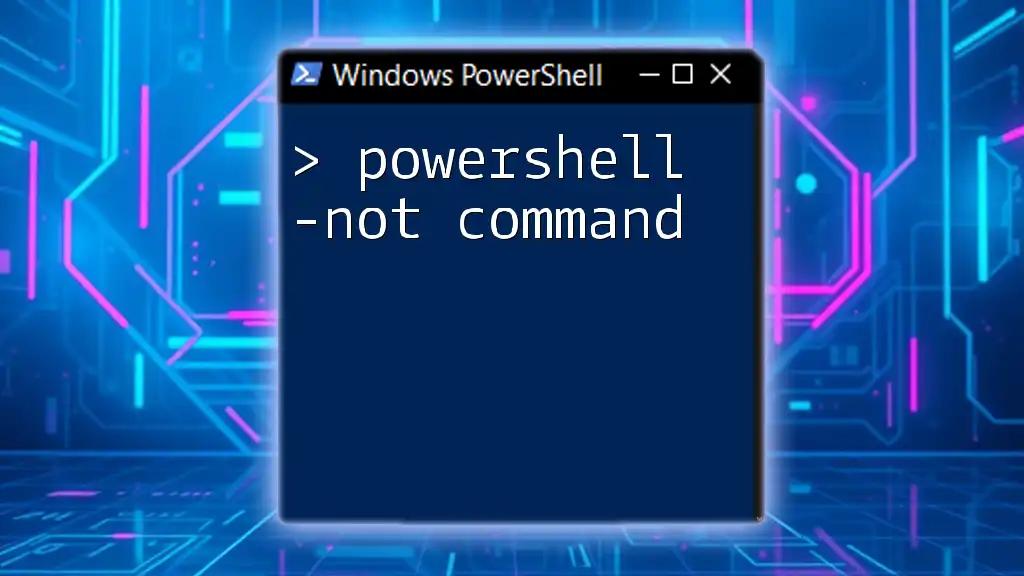
Conclusion
Understanding the PowerShell not contains operator is pivotal for effective scripting and automation. It empowers a wide range of decision-making processes within PowerShell, allowing for sophisticated data manipulation. Mastering the `-notcontains` functionality enhances your ability to control program flow and manage data efficiently.
With practice and application, you'll be well-equipped to leverage PowerShell’s capabilities, enabling you to tackle automation tasks with confidence and precision.

Additional Resources
For further exploration of PowerShell features, consider checking the official Microsoft documentation and other resources dedicated to PowerShell scripting. Engaging in community forums or workshops can also accelerate your learning journey, providing practical insights and tips for mastering PowerShell.