The "Not In" operator in PowerShell allows you to check if a value is not present within a collection, effectively filtering out unwanted items.
Here's a code snippet demonstrating its use:
$fruits = 'Apple', 'Banana', 'Cherry'
$myFruit = 'Grape'
if ($myFruit -notin $fruits) {
Write-Host "$myFruit is not in the list of fruits."
}
Understanding the Concept of "Not In"
The "Not In" operator in PowerShell is a comparison operator used to determine if a specific value does not exist within a collection of values. Understanding how it operates is key to mastering conditional logic in PowerShell scripts.
What Does "Not In" Mean?
The operator is different from common comparison operators such as `-eq` (equal), `-ne` (not equal), `-gt` (greater than), or `-lt` (less than). While those operators compare numerical or string values, `-notin` emphasizes membership in a collection. When you use "Not In", you are asking, "Is this item not in this set?"
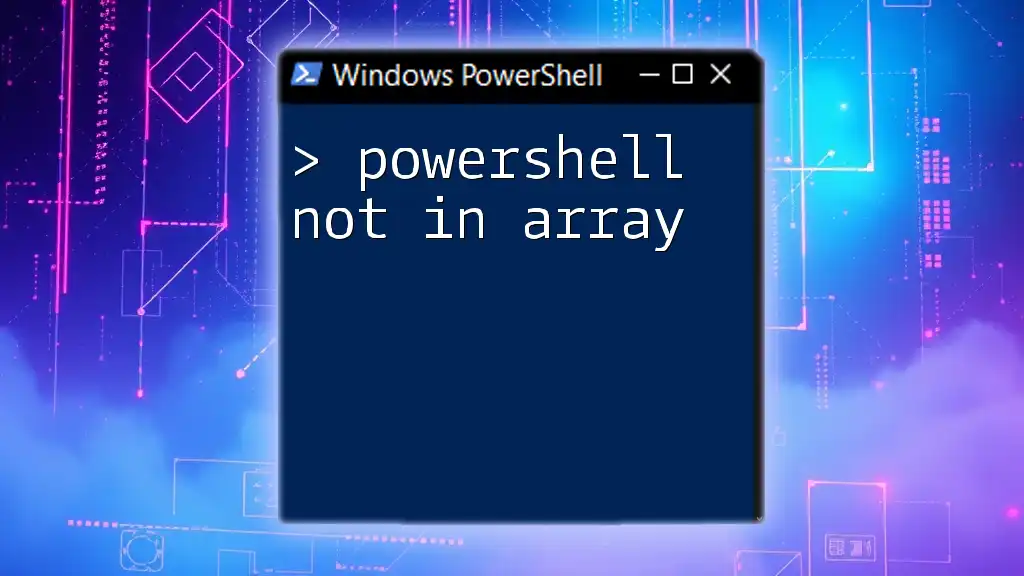
Using the "Not In" Operator
Basic Syntax of "Not In"
The syntax for using the "Not In" operator is quite straightforward. You typically have an item on the left side of the `-notin` operator and a collection (often an array) on the right side. Here's a basic example:
$item = "apple"
$fruits = "banana", "orange", "grape"
if ($item -notin $fruits) {
Write-Output "$item is not in $fruits."
}
In this snippet, we check if `"apple"` is not in the array of fruits. Since it's not present, the output will be: "apple is not in banana, orange, grape."
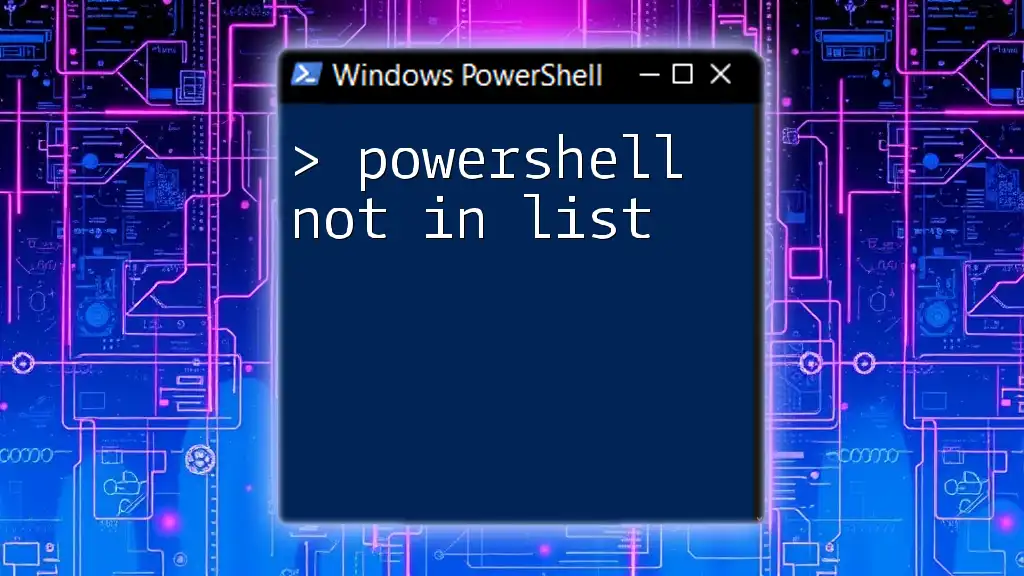
Practical Applications of "Not In"
Filtering Items from an Array
One of the most common applications of "Not In" is to exclude certain items from an array based on criteria. For example, you might want to filter out unwanted items:
$items = 1..10
$excluded = 2, 4, 6
$result = $items | Where-Object { $_ -notin $excluded }
Write-Output $result
In this code, we create an array of numbers from 1 to 10 and wish to exclude 2, 4, and 6. The command will output 1, 3, 5, 7, 8, 9, 10, demonstrating the effective filtering capability of "Not In."
Output Explanation
The output shows how you can easily filter data sets. This capability is especially useful for data management tasks where certain entries need to be excluded based on specific criteria, such as filtering error logs or managing list memberships.
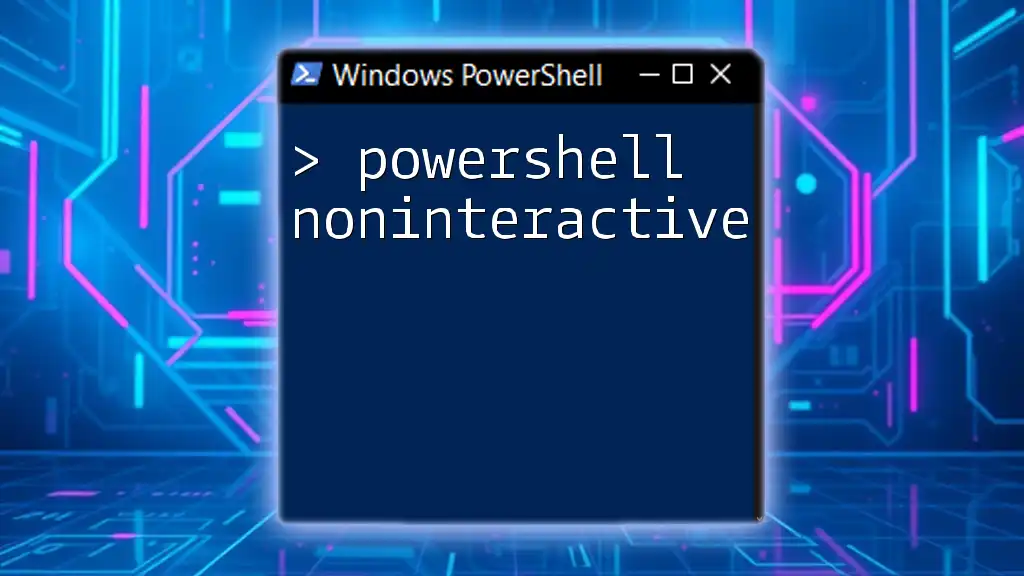
Combining "Not In" with Other Cmdlets
Using "Not In" with `Where-Object`
You can significantly enhance your script's functionality by combining "Not In" with the `Where-Object` cmdlet. This allows you to create more refined data selections.
For instance, if you want to filter processes running on your machine but exclude System and Idle, you can do the following:
$processes = Get-Process
$exclude = "System", "Idle"
$filteredProcesses = $processes | Where-Object { $_.ProcessName -notin $exclude }
Explanation of Results
Here, the expression retrieves all running processes and filters them, excluding any that match "System" or "Idle." This is particularly beneficial in management tasks when you need to focus only on actively utilized resources.
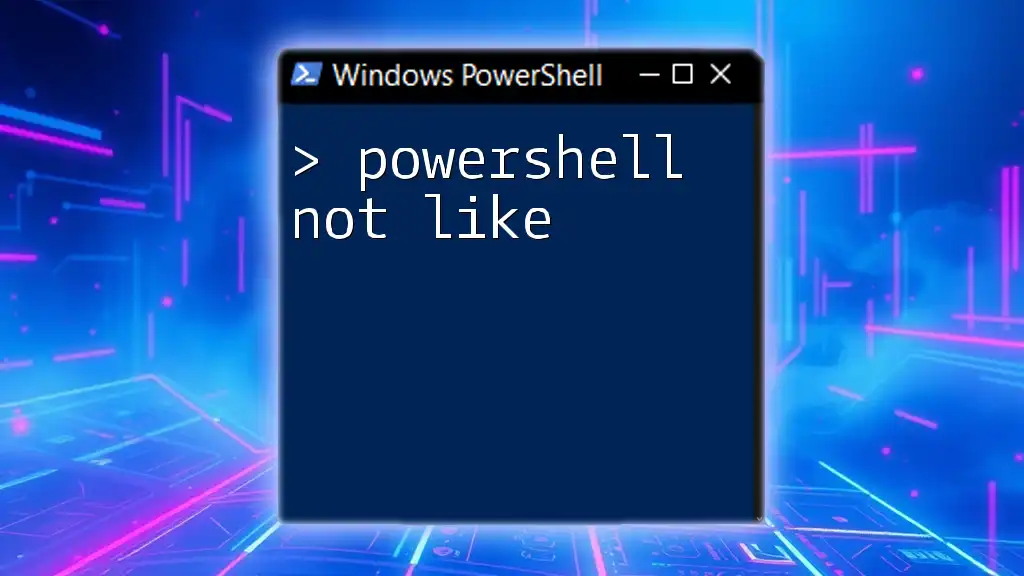
Practical Scenarios for Using "Not In"
Removing Unwanted Data in Scripts
Imagine you are working on a report script that gathers data from multiple sources. You might need to exclude specific categories not relevant to your report. Utilizing "Not In" can simplify this task and ensure your reports are precise and relevant.
Scripting for Reporting
For those responsible for generating regular reports, "Not In" can be used to identify anomalies or exceptions by excluding certain data points. For example, if you want to report on sales data while excluding products that were returned, "Not In" gives you the power to quickly filter out unwanted entries.
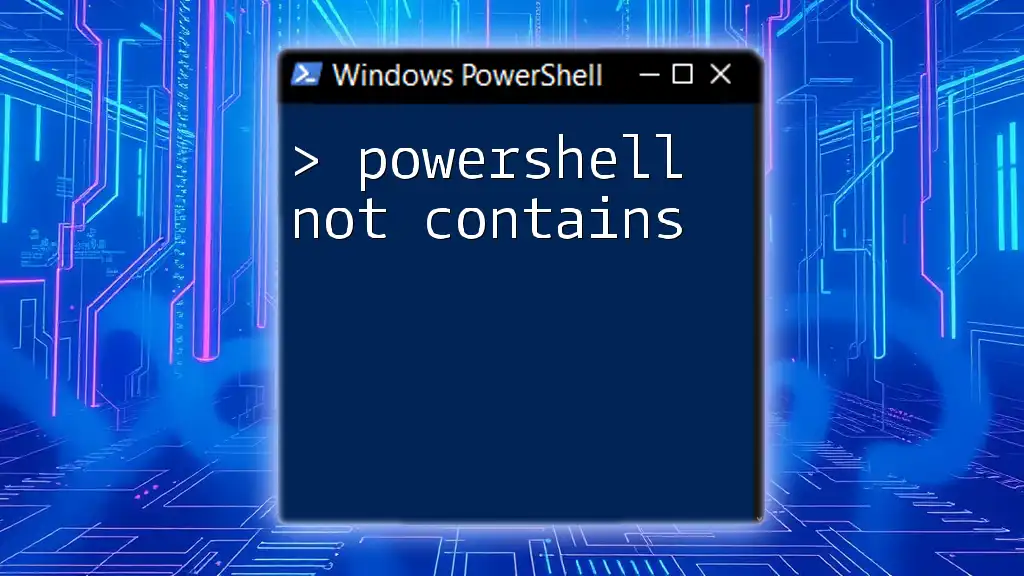
Avoiding Common Pitfalls
Typographical Errors
Typographical errors often lead to common mistakes when using "Not In." A frequent mistake is using `not-in` instead of the correct `-notin`. Such errors will result in unexpected behaviors or script failures.
Misunderstanding Operator Precedence
Operator precedence can also confuse new users. Be explicit in your expression grouping to avoid unintentional results. Always test your scripts in smaller chunks to ensure each part functions as expected.
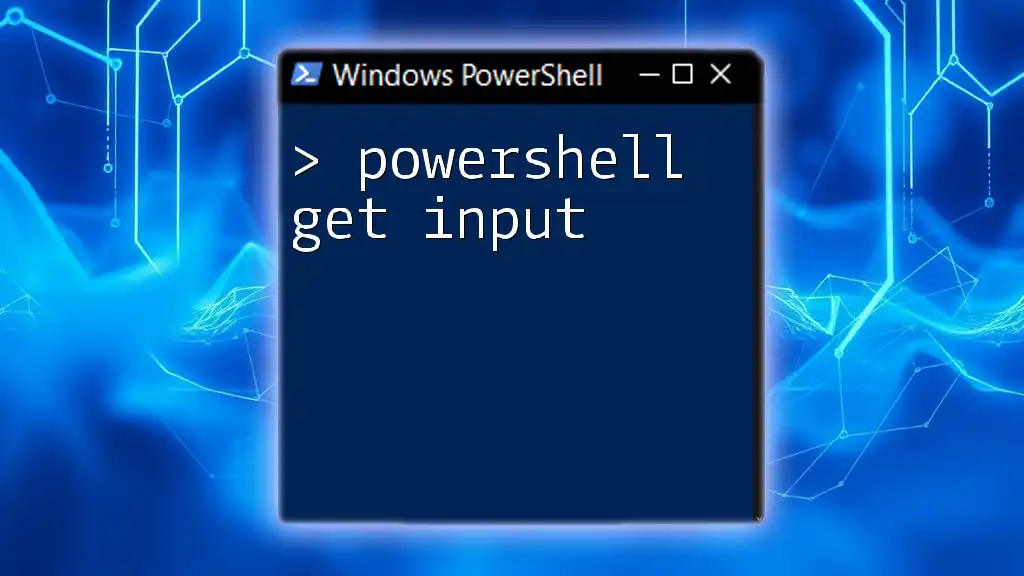
Best Practices for Using "Not In"
Readability and Code Maintenance
A significant aspect of coding is maintainability. When using "Not In," keep your code readable. Place your conditions clearly and, where necessary, provide comments detailing complex logic.
Performance Considerations
When working with large datasets, understand that using "Not In" could impact performance if the datasets are extremely large. Always evaluate your data size and consider possible optimizations, such as using hash sets for quicker membership testing.
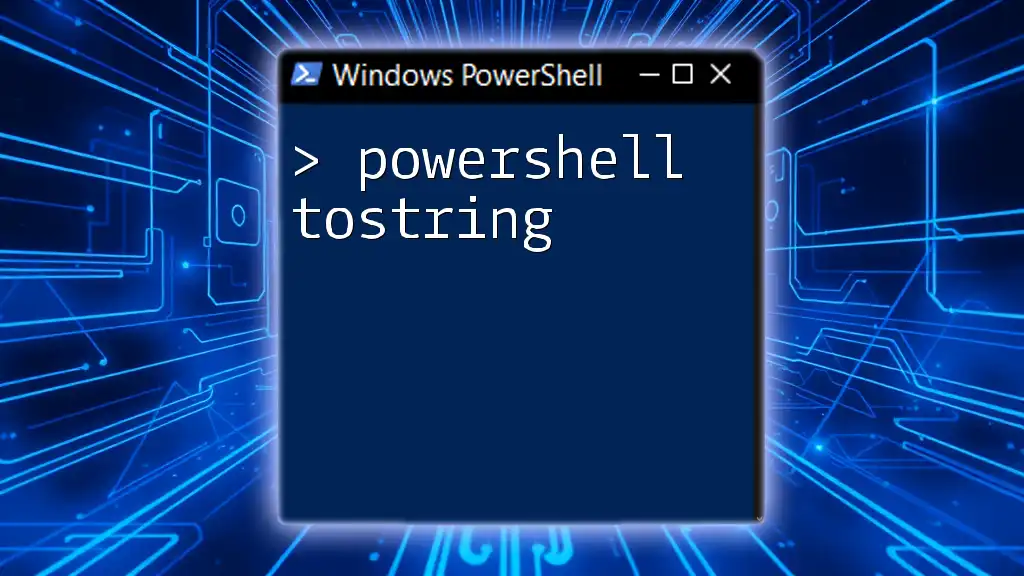
Conclusion
In conclusion, the "Not In" operator is a powerful tool available in PowerShell for performing conditional checks against collections. Its ability to filter data, coupled with other cmdlets, facilitates effective script writing and data management.
As you deepen your understanding and practical application of PowerShell, leveraging "Not In" effectively will become second nature. Always seek to improve your skills in PowerShell, exploring advanced topics and practices to elevate your scripting capabilities. Exploring official documentation and community support can provide further insights to refine your PowerShell expertise.
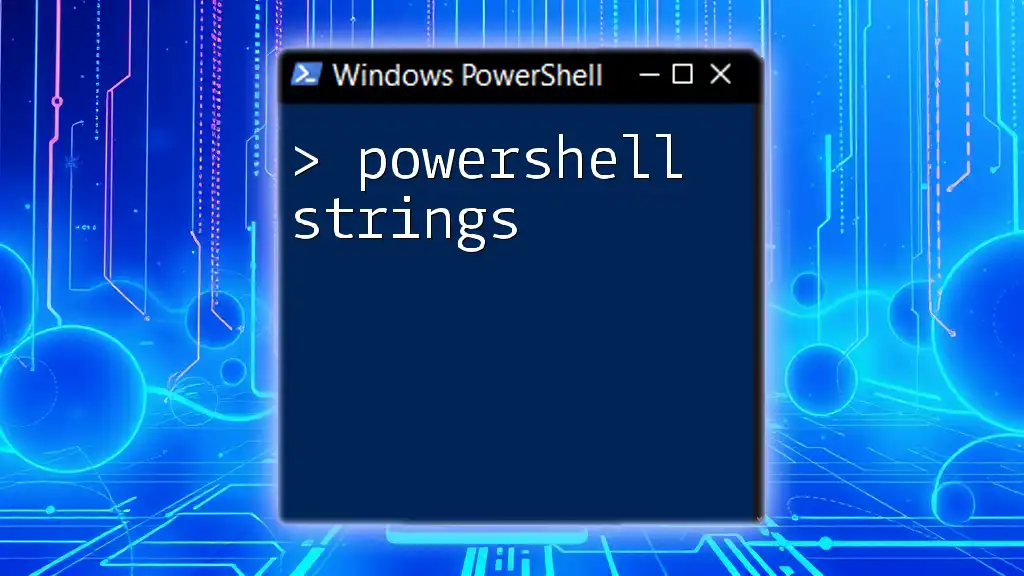
Additional Resources
To enhance your PowerShell journey, immerse yourself in official PowerShell documentation, and consider engaging with online courses tailored to expand your scripting skills. Don’t forget to be active in community forums where you can ask questions and share experiences, allowing continual growth in your understanding of PowerShell.