The `-In` parameter in PowerShell is used with the `Where-Object` cmdlet to filter objects based on whether they are contained within a specified collection.
Here’s a code snippet to demonstrate its usage:
$numbers = 1..10
$filterNumbers = 5..8
$filtered = $numbers | Where-Object {$_ -in $filterNumbers}
$filtered
Understanding the Basics of the -in Operator
What Does -in Do?
The `-in` operator in PowerShell is a filtering operator that checks if a specified value exists within a collection, such as an array or a hash table. It returns a Boolean value, meaning it will yield either `True` or `False`. This operator is particularly useful for simplifying membership tests and enhances code readability.
Syntax of the -in Operator
The basic syntax of the `-in` operator is structured like this:
$value -in $collection
Where `$value` is the item you want to check, and `$collection` is the array or group you are checking against. The `-in` operator provides an intuitive way of querying membership, eliminating the need for verbose looping constructs.

How to Use the -in Operator
Checking for Membership in a Collection
Using the `-in` operator is straightforward when checking for membership. For instance, suppose you have a collection of fruits:
$fruits = "Apple", "Banana", "Cherry"
"Banana" -in $fruits
In this example, the output will be `True` since "Banana" is indeed one of the fruits in the `$fruits` array. If you checked for "Orange", the outcome would be `False`.
How -in Works with Strings
The `-in` operator can also be applied to string arrays, which is useful when you want to check the existence of certain strings within a predefined list. For example:
$cities = "New York", "London", "Tokyo"
"Tokyo" -in $cities
Here, the output would again be `True`, confirming that "Tokyo" is included in the `$cities` array.

Combining -in with Other PowerShell Features
Using -in with Conditional Statements
Combining the `-in` operator with conditional statements can help you enforce rules based on user input or predefined conditions. For instance, if you wanted to allow access only to a list of authorized users, you could use the following approach:
$allowedUsers = "Alice", "Bob", "Charlie"
$username = Read-Host "Enter your username"
if ($username -in $allowedUsers) {
"Access Granted"
} else {
"Access Denied"
}
In this code snippet, the script checks if the entered `$username` exists in the `$allowedUsers` list. This enhances security by ensuring only specific users can gain access.
Using -in with Filtering Cmdlets
A powerful way to utilize the `-in` operator is in conjunction with cmdlets like `Where-Object`, which allows you to filter objects based on specific criteria. Consider the following example:
$users = Get-ADUser -Filter * | Select-Object Name, Department
$departments = "HR", "IT"
$users | Where-Object { $_.Department -in $departments }
This script fetches a list of users from Active Directory and filters them down to only those in the departments specified in the `$departments` array. In this case, it returns users who work in either "HR" or "IT".
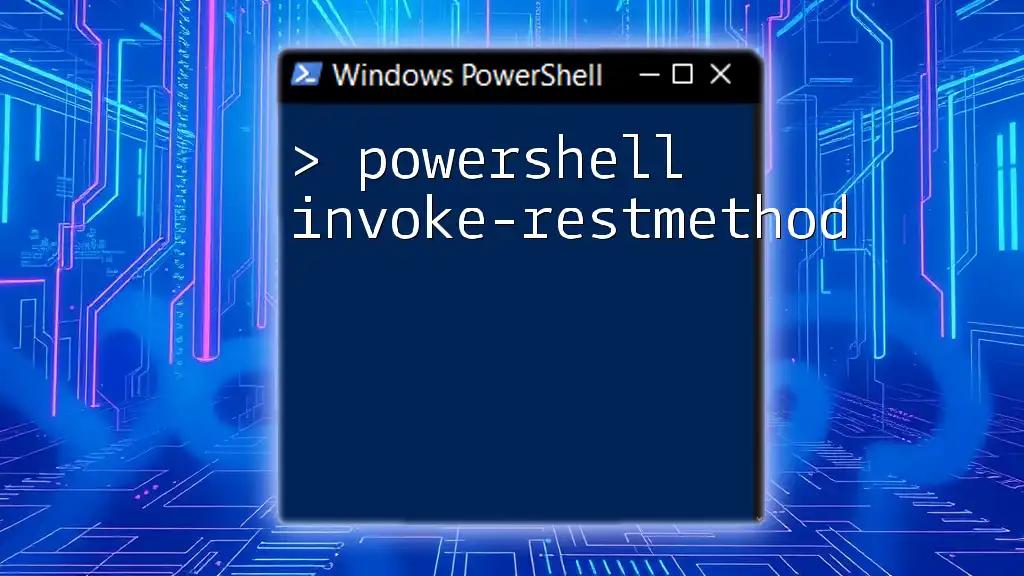
Practical Scenarios to Implement -in
Scenario 1: Data Validation
Implementing input validation is another excellent application of the `-in` operator. Suppose you have various options you want to validate against user input:
$validOptions = "Production", "Testing", "Development"
$inputOption = "Testing"
if ($inputOption -in $validOptions) {
"Valid option selected."
} else {
"Invalid option!"
}
In this example, the user-provided `$inputOption` is checked against valid options. This ensures that only acceptable values are processed, contributing to data integrity.
Scenario 2: User Management
The `-in` operator is also useful for managing user memberships effectively. You might need to check if a user belongs to certain groups, as shown in this example:
$groups = (Get-ADUser -Identity "Alice").MemberOf
$requiredGroups = "Admins", "Users"
if ($groups -in $requiredGroups) {
"User is part of required groups."
} else {
"User is not part of required groups."
}
This script retrieves the groups that user "Alice" is a member of and checks against the `$requiredGroups`. The resulting message will indicate whether "Alice" is in either "Admins" or "Users".

Common Pitfalls and Best Practices
Misusing the -in Operator
One common mistake when using the `-in` operator is mismatching data types. The operator expects both the value and the collection to align in type. For instance, trying to check a string against an array of integers will yield `False` even if they seem conceptually similar.
Best Practices for Using -in
To use the `-in` operator effectively, keep these best practices in mind:
- Type Consistency: Always ensure that the type of the checked value matches the items in the collection.
- Readability: Use the `-in` operator to simplify your conditions, which can make the code more readable and maintainable.
- Combination with String Manipulation: For complex checks, you can chain operators or combine with string functions. For example:
$names = "John", "Doe", "Jane"
"Jane" -in $names -and ("Doe" -in $names)
This checks multiple conditions simultaneously, ensuring that both names are in the list.

Conclusion
In summary, the PowerShell `-in` operator is a powerful and flexible tool for checking membership in collections. Its ability to return straightforward Boolean values simplifies conditional statements and makes scripts more readable. Experimenting with the examples provided will enhance your understanding and allow you to leverage the `-in` operator effectively in various scenarios.
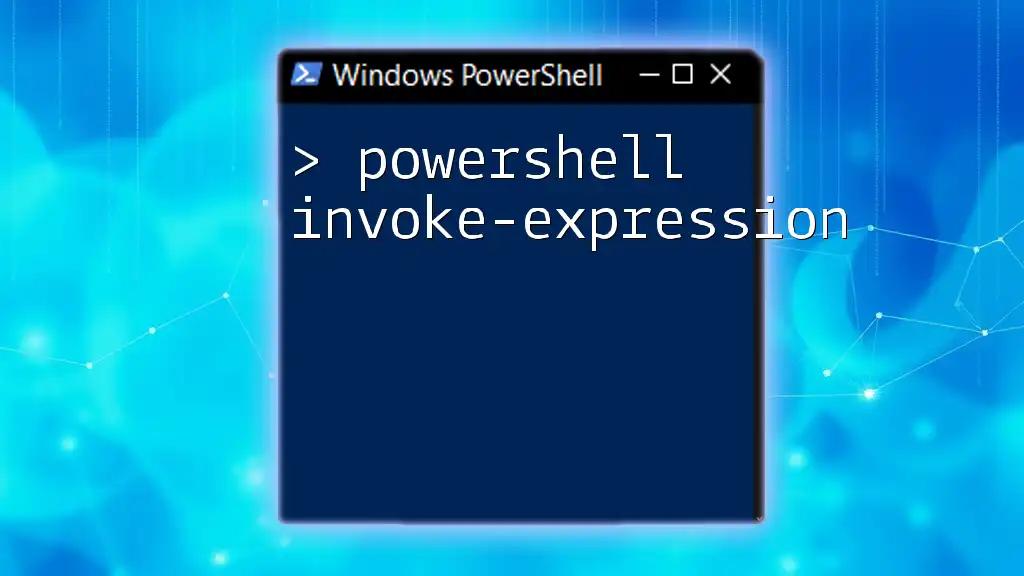
Additional Resources
Documentation and References
For further insights, consider exploring the [official PowerShell documentation](https://docs.microsoft.com/en-us/powershell/) and community forums dedicated to PowerShell users. These resources can greatly support your learning journey.
Recommended Reading
Look for books and blogs that focus on PowerShell best practices and advanced techniques to deepen your knowledge and skills in this versatile scripting language. Tutorials featuring real-world applications will also enrich your learning experience.