In this post, we explore essential PowerShell interview questions designed to gauge a candidate's proficiency with command-line scripting and automation tasks.
Write-Host 'Hello, World!'
Understanding PowerShell
What is PowerShell?
PowerShell is a command-line shell and scripting language developed by Microsoft, designed for task automation and configuration management. Unlike traditional shells, it is built on the .NET framework, allowing users to work with objects rather than just text, which enhances the versatility and richness of the commands you can use.
Why are PowerShell Skills Valuable?
Mastering PowerShell is crucial for several reasons:
- Market Demand: Many organizations are looking for professionals who can automate repetitive tasks, manage server environments efficiently, and integrate multiple systems.
- Job Roles: Proficiency in PowerShell is often a prerequisite for roles in system administration, IT operations, and DevOps, among others.
- Automation and Efficiency: Knowing how to leverage PowerShell can lead to significant improvements in productivity, allowing more focus on strategic tasks instead of mundane manual processes.
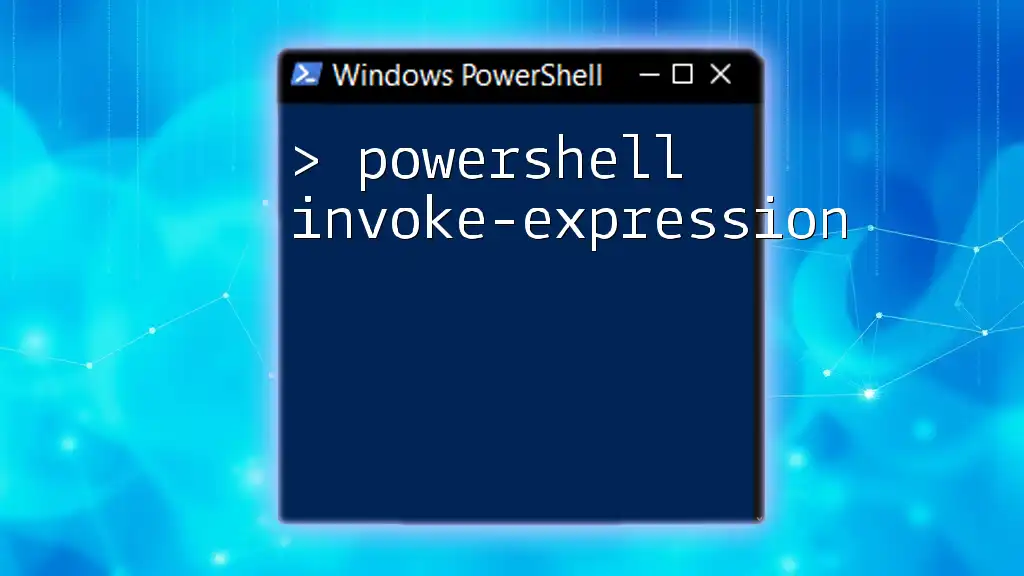
Common PowerShell Interview Questions
General PowerShell Questions
What is the difference between PowerShell and Command Prompt? PowerShell is a powerful scripting language that can execute complex scripts and automate processes, while Command Prompt primarily handles traditional command-line inputs. The key distinction is that PowerShell operates with objects, enabling users to manipulate data structures seamlessly—this is something Command Prompt cannot do.
How do PowerShell cmdlets differ from traditional commands? Cmdlets are built-in .NET classes specifically designed for PowerShell. They follow a verb-noun structure (e.g., `Get-Process`) and can return objects to the pipeline, contrasting with traditional commands which return text. For instance, running the command:
Get-Process
will return a collection of process objects rather than plain text.
Windows PowerShell Interview Questions
Can you explain what a PowerShell provider is? PowerShell providers enable access to various data stores such as the file system, registry, and certificates. They allow you to navigate and manipulate data similar to how you browse files. For example, the filesystem provider allows you to use commands like `cd`, `ls`, or `dir` to navigate through directories.
How do you perform error handling in PowerShell? Effective error handling is crucial in PowerShell scripts. You can utilize the `Try-Catch` block to handle exceptions gracefully:
Try {
# Code that might generate an error
Get-Content "C:\invalidPath.txt"
} Catch {
Write-Host "An error occurred: $_"
}
This will catch any errors while attempting to read a nonexistent file and provide a message rather than halting the script.
What are the key differences between PowerShell scripts and PowerShell commands? PowerShell scripts encapsulate multiple commands and logic in a `.ps1` file, allowing for complex automation. Meanwhile, individual commands can be executed directly in the console and often handle simple tasks. Scripts also support parameters, functions, and control-flow elements that commands do not.
Advanced PowerShell Questions
What are PowerShell modules and how do you create one? Modules are essential for organizing code. They allow you to group related functions, cmdlets, and variables. To create a module, you typically follow these steps:
- Create a `.psm1` file that contains your functions.
- Use `Export-ModuleMember` to export any cmdlets or functions you want to make public.
A simple module file `MyModule.psm1` could look like this:
function Get-Greeting {
param([string]$Name)
"Hello, $Name!"
}
Export-ModuleMember -Function Get-Greeting
Use `Import-Module MyModule` to load this module.
How can you retrieve data from a CSV file using PowerShell? PowerShell excels at accessing data formats like CSV. You can use the `Import-Csv` cmdlet to convert CSV data into objects:
$users = Import-Csv "C:\path\to\users.csv"
$users | ForEach-Object {
Write-Host "User: $_.Name, Email: $_.Email"
}
This code imports users and echoes back their names and emails.
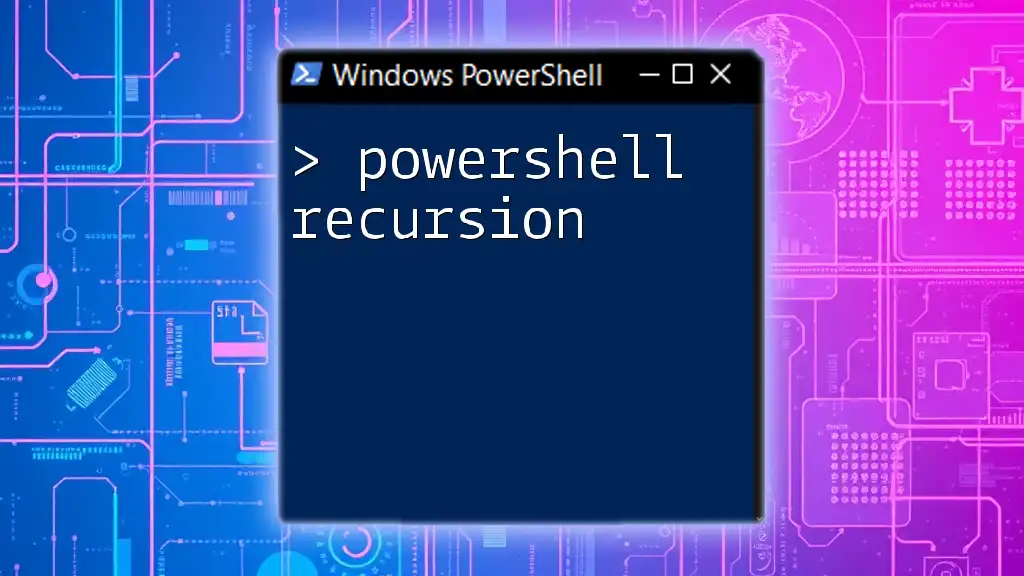
Coding and Scripting Questions
Basic Scripting Questions
Can you explain the syntax of a ForEach loop in PowerShell? The `ForEach` loop is essential for iterating over collections. Its syntax is straightforward:
$numbers = 1..5
ForEach ($number in $numbers) {
Write-Host "Current number is $number"
}
This loop will print each number in the specified range.
Describe how to use variables in PowerShell. Variables in PowerShell are prefixed with a `$` symbol. They can hold various data types, from strings to arrays. Here’s an example of declaring and using variables:
$name = "John Doe"
$age = 30
Write-Host "$name is $age years old."
Intermediate Scripting Questions
How would you schedule a PowerShell script to run automatically? You can use Windows Task Scheduler to schedule PowerShell scripts. Create a new task and set the action to run PowerShell with the script's path:
powershell -File "C:\path\to\your_script.ps1"
This allows the script to execute at specified times or triggers.
What are the different ways to pass parameters to a PowerShell script? Parameters can be passed in several ways:
- Positional: Parameters are added in order when calling the script.
- Named: Parameters are specified explicitly by name, enhancing readability.
Example of parameter passing in a script:
param([string]$Name, [int]$Age)
Write-Host "$Name is $Age years old."
You would call this script as follows:
.\YourScript.ps1 -Name "Alice" -Age 25
Advanced Scripting Questions
What are the benefits of using PowerShell Workflows? PowerShell Workflows offer advanced automation capabilities, particularly for long-running or parallel tasks, allowing for better resource management. They also provide built-in support for checkpoints, enabling you to resume workflows from a known state.
How do you manage PowerShell remoting? PowerShell remoting enables you to run commands on remote machines via `Invoke-Command`, simplifying administration across multiple servers. Ensure remoting is enabled on the target machine using:
Enable-PSRemoting -Force
You can then execute commands like this:
Invoke-Command -ComputerName "Server01" -ScriptBlock { Get-Process }
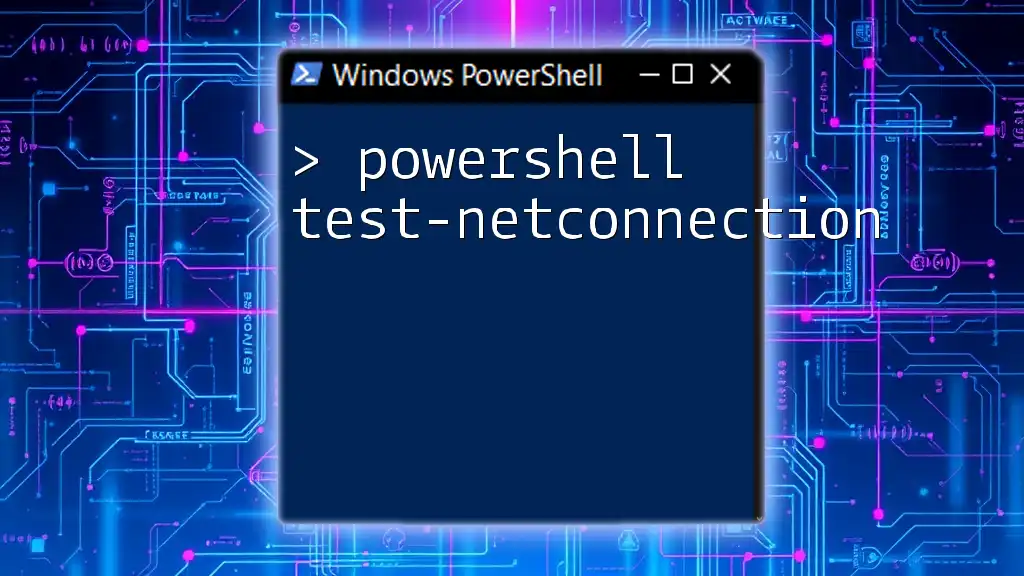
Troubleshooting and Testing
PowerShell Debugging Techniques
Debugging is an essential skill when working with scripts. PowerShell provides the `Set-PSDebug` command for tracing execution. To enable debugging, use:
Set-PSDebug -Trace 1
This will display the execution flow of your script, helping you identify issues effectively.
Testing Your Scripts
To ensure your scripts are reliable, you should implement testing principles. The Pester testing framework is popular among PowerShell users, allowing you to write unit tests for your scripts.
Example Pester test for a function:
Describe "MyFunction Tests" {
It "should return the correct greeting" {
Get-Greeting "World" | Should -Be "Hello, World!"
}
}
This test checks if the `Get-Greeting` function behaves correctly, ensuring quality and reliability.
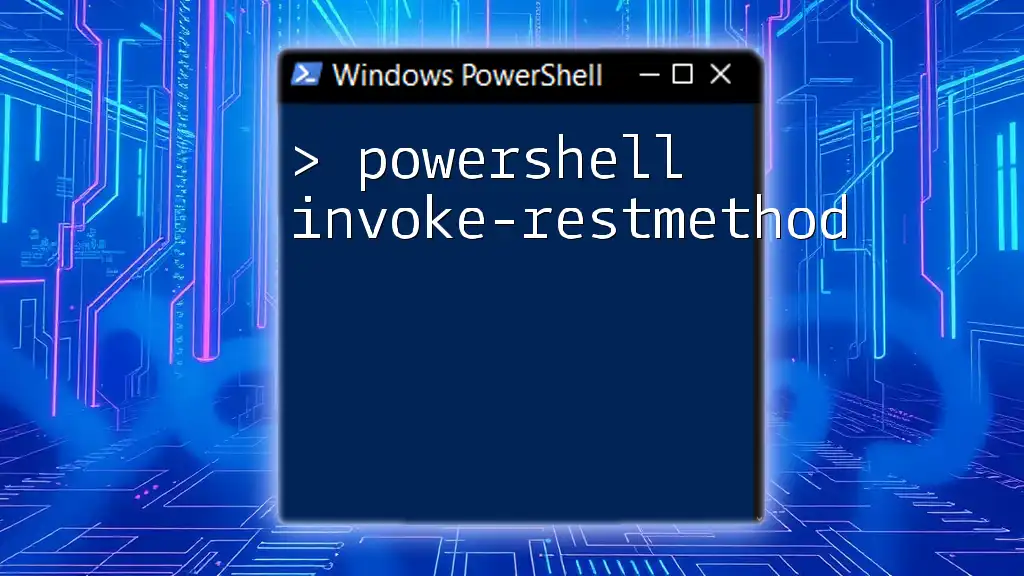
Soft Skill and Scenario-Based Questions
Behavioral Questions
Describe a challenging scenario you faced using PowerShell and how you resolved it. In an interview, you may be asked about a specific issue you've encountered. A good response would follow the STAR method (Situation, Task, Action, Result) for clarity, demonstrating your problem-solving skills and the impact of your solution.
Scenario-Based Problem Solving
How would you approach optimizing a slow-running PowerShell script? First, identify bottlenecks using profiling tools and measure execution time for various script segments. Then, implement optimizations such as reducing unnecessary loops, avoiding unnecessary calls to external systems, and leveraging built-in cmdlets that utilize .NET collections for better performance.
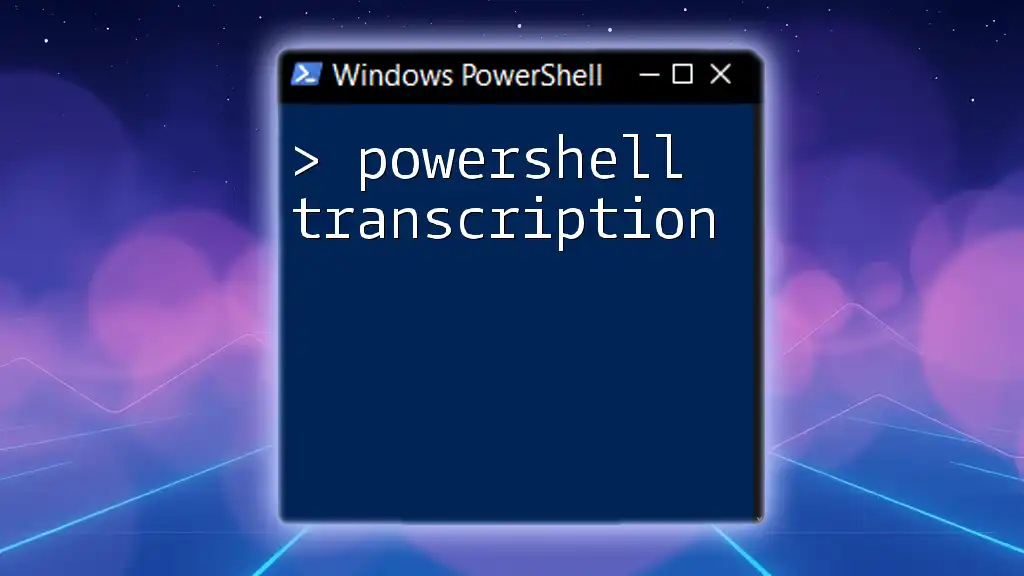
Conclusion
Preparing for Your PowerShell Interview
To prepare for interviews focusing on PowerShell interview questions, prioritize practical experience. Regularly practicing scripts, building modules, and participating in community forums will enhance your confidence and capabilities.
Additional Resources
Leverage resources such as books, online courses, and community forums to further your understanding of PowerShell. Engaging in discussions with fellow enthusiasts can deepen your knowledge and expose you to various use cases and tricks.
By implementing the techniques and knowledge discussed above, you'll present yourself as a strong candidate well-versed in PowerShell during interviews.