The `Exit` function in PowerShell is used to terminate the current session or script, returning an optional exit code that can indicate success or failure.
Here's a simple code snippet demonstrating its usage:
# Terminate the script with an exit code of 0 (success)
Exit 0
Understanding Function Exit Mechanisms
What is the PowerShell Exit Function?
The PowerShell exit function is crucial for managing the behavior of scripts and functions. It enables you to terminate the execution of a function immediately, returning control to the calling script or environment. Understanding this can save you from running into infinite loops or unexpected script behavior, enhancing script reliability.
The Role of Return Values
In PowerShell, every function can return a value. Return values are essential for providing feedback to the script owner about the function's execution status. For instance, when exiting a function after performing calculations, you might want to return the result, indicating whether it succeeded or failed.
Here's a code snippet that shows how return values work with the exit function:
function Calculate {
param (
[int]$a,
[int]$b
)
if ($b -eq 0) {
exit 1 # Return a non-zero value if there's an error (division by zero)
}
return $a / $b
}
In this example, if the second parameter is zero, the function uses `exit` to indicate an error, which communicates to the caller that something went wrong.
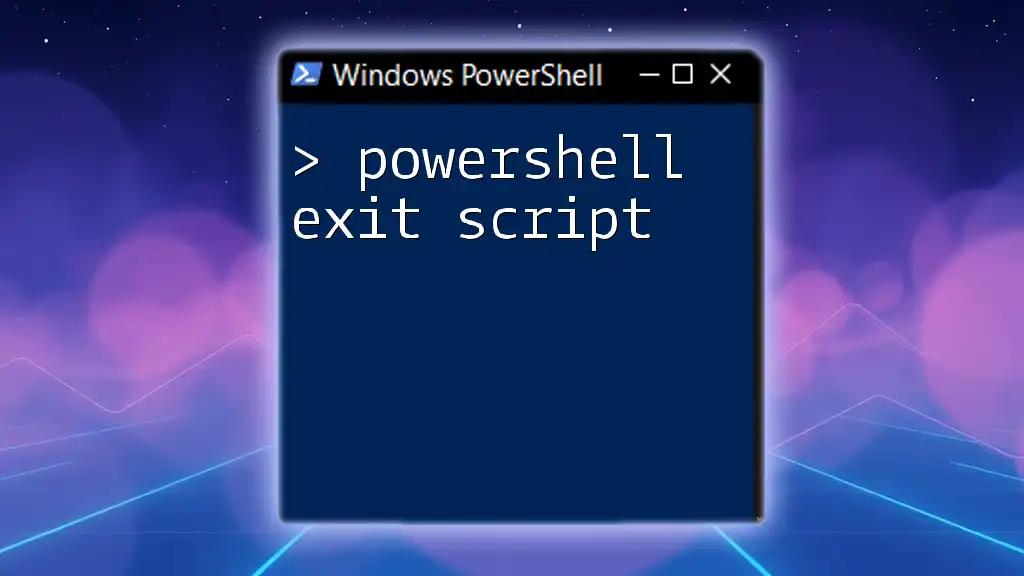
Using the PowerShell Exit Function
Basic Syntax of the Exit Function
The basic syntax for the exit function is simple. It is invoked as follows:
exit <exitCode>
If no exit code is provided, it defaults to `0`, indicating successful execution. A non-zero exit code typically signifies an error or abnormal termination.
Here’s a basic usage example:
function Test-Exit {
exit 0 # Successful exit
}
Exiting from a Function
Using the exit function within a function allows you to halt further script execution gracefully. This is particularly useful when certain conditions aren't met.
Consider the following example, where the function exits if a specific condition is true:
function Validate-UserInput {
param (
[string]$input
)
if (-not $input) {
Write-Host "No input provided. Exiting function."
exit 1 # Exits with an error code if input is empty
}
Write-Host "Input is valid!"
}
In this case, if no input is supplied, the function will exit before any further processing.
PowerShell Quit Function vs. PowerShell Exit Function
Quit vs. Exit: Understanding the Differences
While the exit command terminates a function and optionally returns a code, the quit command is generally used to terminate the entire PowerShell session. Understanding when to use which is essential for effective scripting.
Example for Quit Function
Here’s a scenario using the quit function:
function Stop-All {
Write-Host "Terminating all processes."
quit # Exits the entire PowerShell session
}
Example for Exit Function
By contrast, if you only want to terminate a function, use the exit command.
function Abort-Process {
Write-Host "Aborting process."
exit 1 # Only exits the function
}
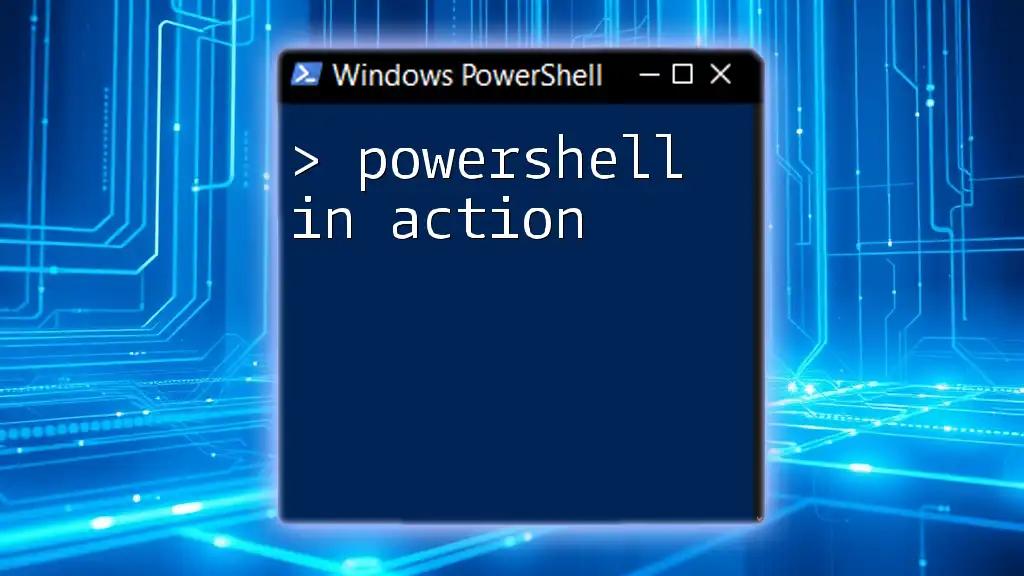
The PowerShell Break Function
What is the PowerShell Break Function?
The break function is a control statement used primarily within loops to interrupt the flow of control. It allows you to exit loops prematurely, which can be particularly useful when certain conditions are met.
Examples of Break Function Use
Consider a scenario where you search through a list and want to stop once you've found the target item. Using the break function makes this straightforward:
foreach ($item in $items) {
if ($item -eq "target") {
Write-Host "Target found!"
break # Exits the loop once the target is found
}
}
In this example, once the target is identified, control exits the loop effectively, removing the need to check subsequent items.
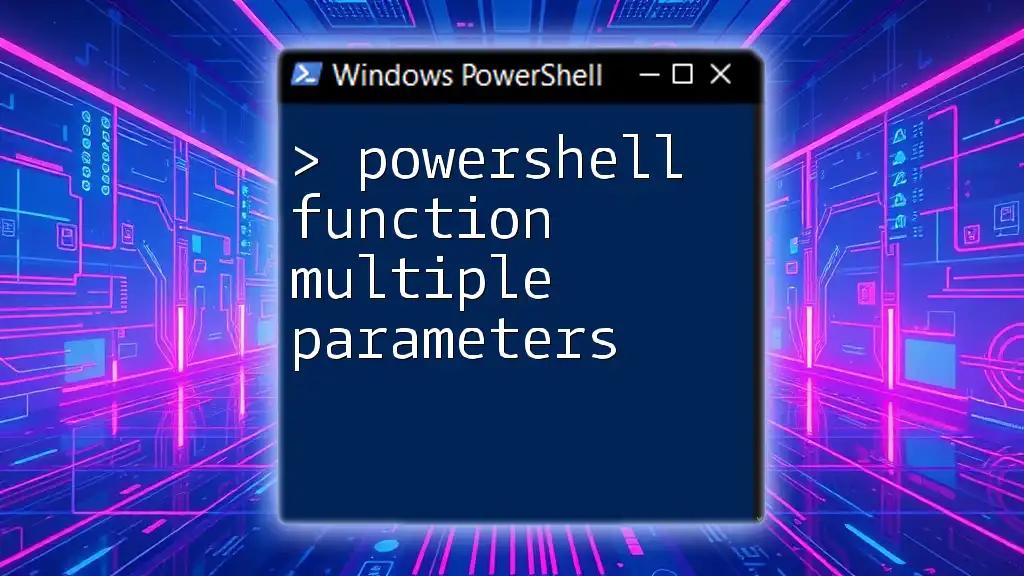
Practical Applications of Exit, Quit, and Break
Use Cases for Exiting Functions
Exiting functions is crucial in various real-world scenarios. For instance, consider a script that processes user data. If a required field is missing, exiting the function early prevents processing invalid data:
function Process-UserData {
param (
[string]$username
)
if (-not $username) {
Write-Host "Username is required. Exiting function."
exit 1 # Exit if username not provided
}
Write-Host "Processing user: $username"
}
Error Handling with Exit Function
Using the exit function can also aid in robust error handling. By returning specific codes, you inform the caller of what went wrong. A typical practice involves using the `$?` automatic variable to check for success:
function Sample-Operation {
if (-not (Some-Command)) {
exit 2 # Exits if the command fails
}
}
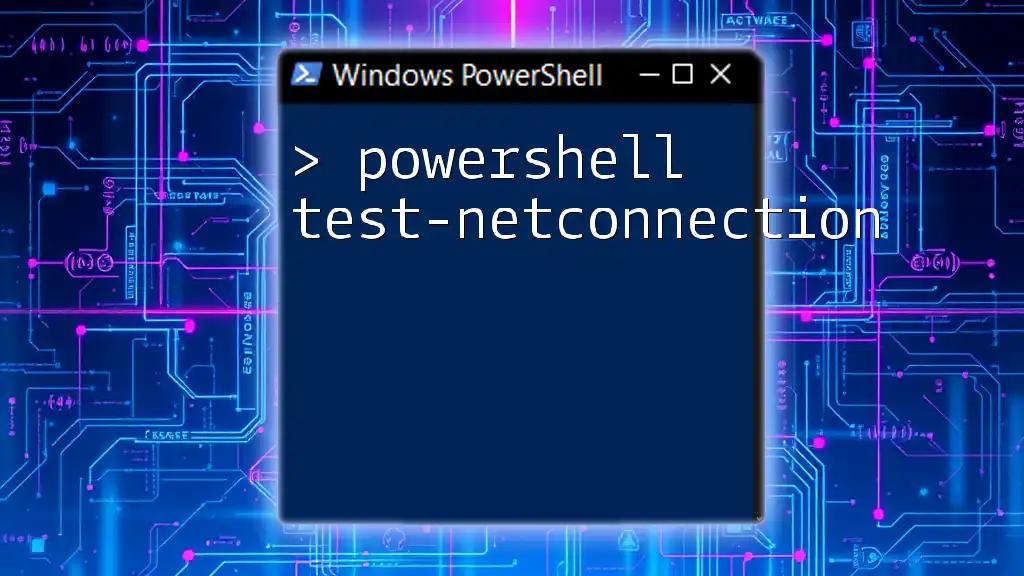
Best Practices for Exiting Functions in PowerShell
When to Use Each Function
Choosing between exit, quit, and break depends on your goals. Use exit when you want to leave a function abruptly with feedback. Use quit to terminate the entire session and break to exit loops when a condition is met.
Common Pitfalls to Avoid
Some common mistakes include:
- Using exit when you need to return a value: remember that exit doesn't return values, it just exits.
- Not handling exit codes properly: always check and handle non-zero exit codes from functions for better error management.
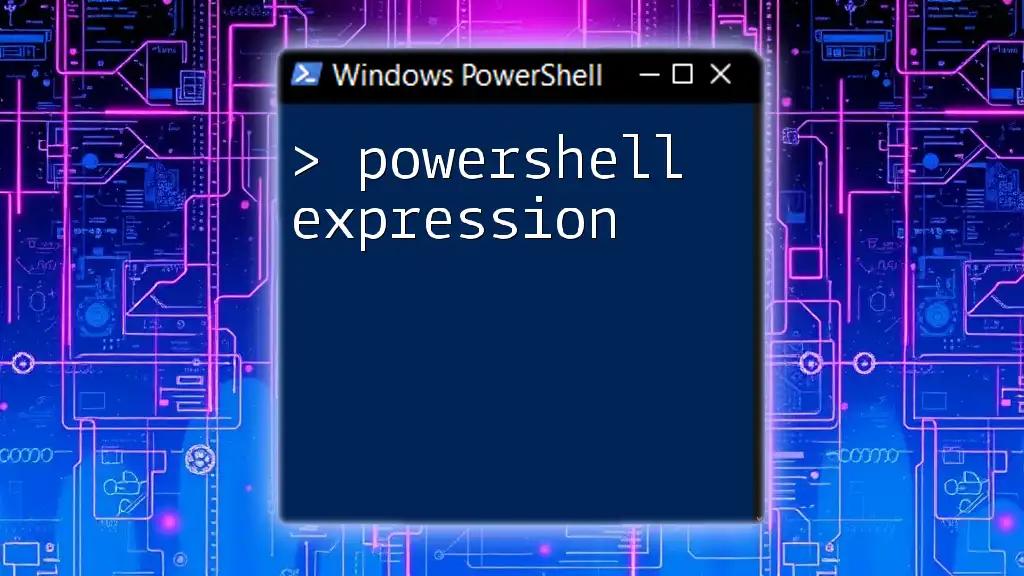
Conclusion
Mastering the PowerShell exit function is vital for efficient script management and execution. Understanding how to control function execution and effectively employ the quit and break commands can greatly enhance the robustness of your scripts. As you develop your PowerShell skills, experimenting with these functions will enable you to write cleaner, more efficient code. Don't hesitate to dive deeper into the documentation and further resources for continuous learning.