In PowerShell, a function can accept multiple parameters by defining them in the parentheses after the function's name, allowing for flexible input when executing tasks.
Here's a simple example:
function Greet-User {
param (
[string]$Name,
[int]$Age
)
Write-Host "Hello, $Name! You are $Age years old."
}
Understanding PowerShell Functions
What is a PowerShell Function?
A PowerShell function is a block of code designed to perform a particular task. Functions are essential in scripting as they promote code reusability and simplify complex operations. By encapsulating logic into functions, you can call them as needed, reducing redundancy and errors in your scripts.
Why Use Multiple Parameters?
Incorporating multiple parameters in a PowerShell function adds flexibility and allows users to customize function behavior based on their needs. With the ability to accept various inputs, functions can handle a broader range of tasks and complexities without requiring you to write multiple versions of the same function.
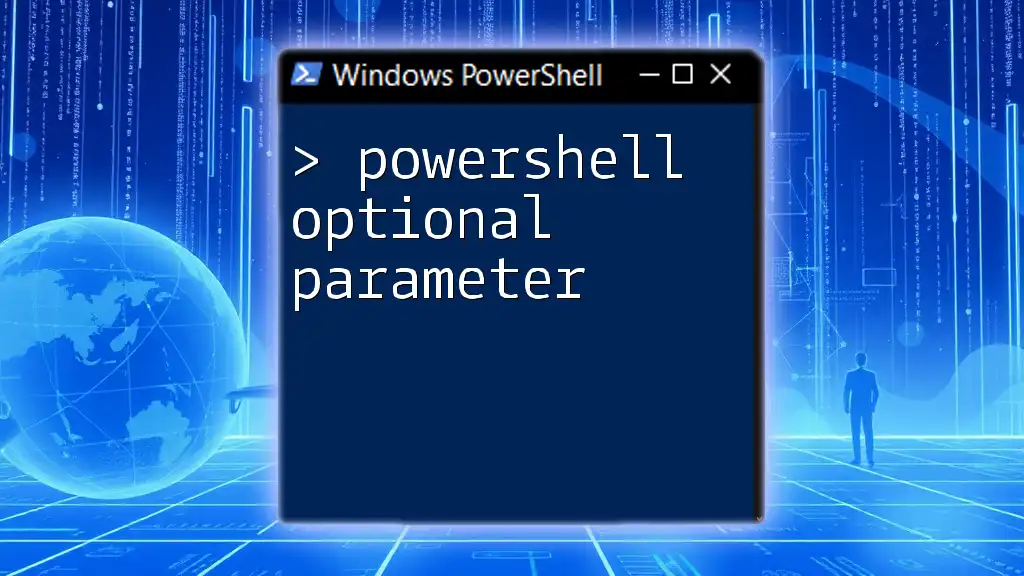
Understanding PowerShell Parameters
What are Parameters?
Parameters are variables passed to functions to provide the necessary values for execution. They enable functions to perform operations based on user input, making scripts dynamic. By defining parameters, users can customize how the function behaves when invoked.
Types of Parameters
Understanding the types of parameters is crucial for creating effective functions:
- Mandatory Parameters: These must be provided by the user when calling the function, or PowerShell will throw an error.
- Optional Parameters: These can be omitted; if not provided, they have default values.
- Named Parameters: Allow users to specify which argument corresponds to which parameter, enhancing clarity.
- Positional Parameters: Users can pass arguments in the order defined in the function.
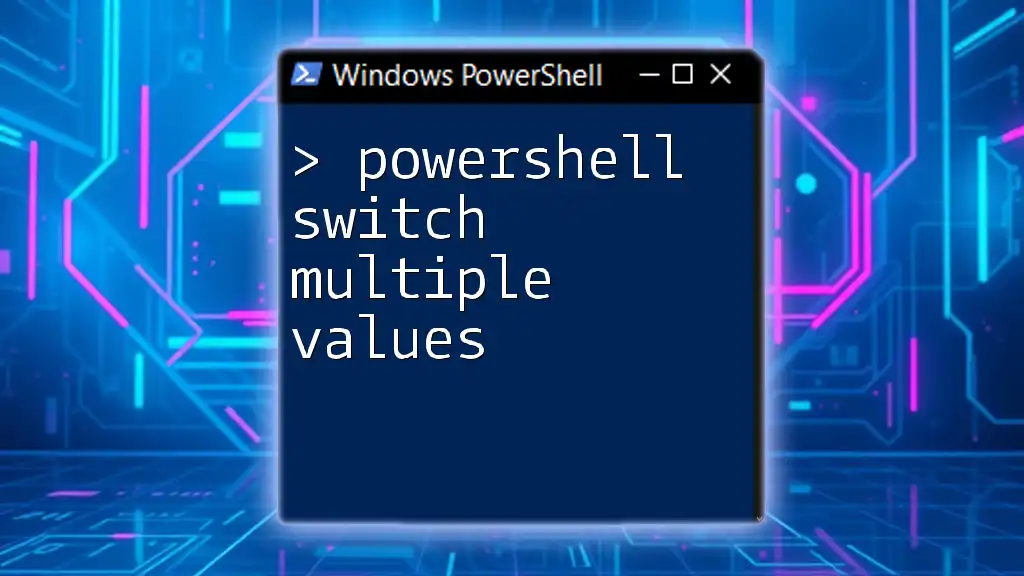
Creating PowerShell Functions with Multiple Parameters
Defining a Function with Multiple Parameters
Creating a function with multiple parameters involves defining parameters within the `param` block at the start of the function. Here’s a basic example:
function Get-UserInfo {
param(
[string]$FirstName,
[string]$LastName,
[int]$Age
)
Write-Host "User Info: $FirstName $LastName, Age: $Age"
}
Example of a Basic Function
To utilize the `Get-UserInfo` function, you can pass parameters using named arguments:
Get-UserInfo -FirstName "John" -LastName "Doe" -Age 30
This call would output: `User Info: John Doe, Age: 30`, confirming the function's capability to accept multiple parameters and display formatted output.
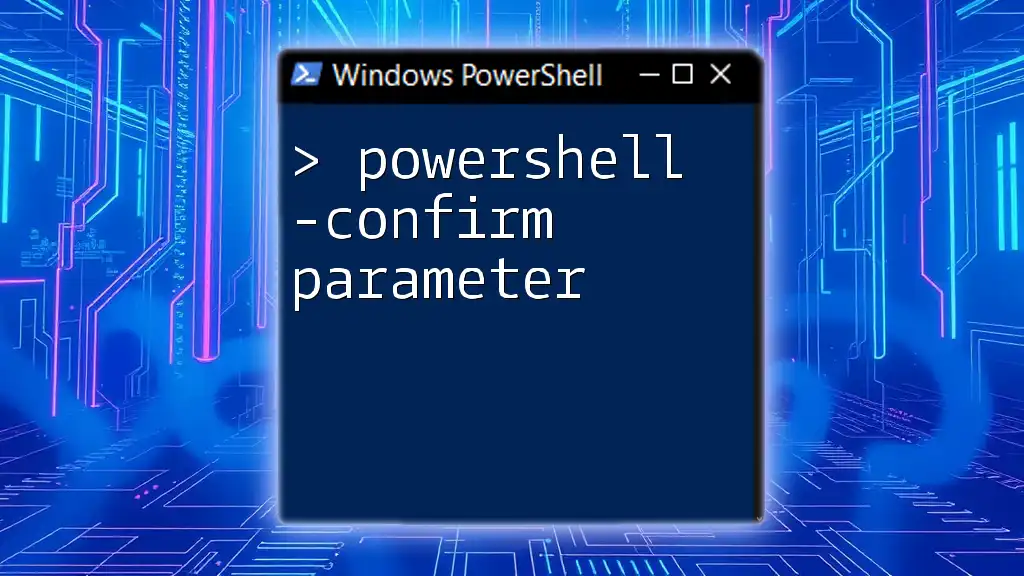
Handling Parameter Validation
Why Validate Parameters?
Parameter validation is vital to safeguard against unexpected inputs that could cause errors or undesired behavior. By ensuring that only appropriate values are accepted, you can maintain the integrity and functionality of your scripts.
Implementing Parameter Validation
You can implement validation using attributes directly in your parameter definitions. Here’s an effective approach using type constraints:
function Set-User {
param(
[string]$Username,
[ValidateRange(1, 120)]
[int]$Age
)
Write-Host "Creating user $Username with age $Age"
}
If the age is outside the defined range, PowerShell will display an error, promoting proper data handling.
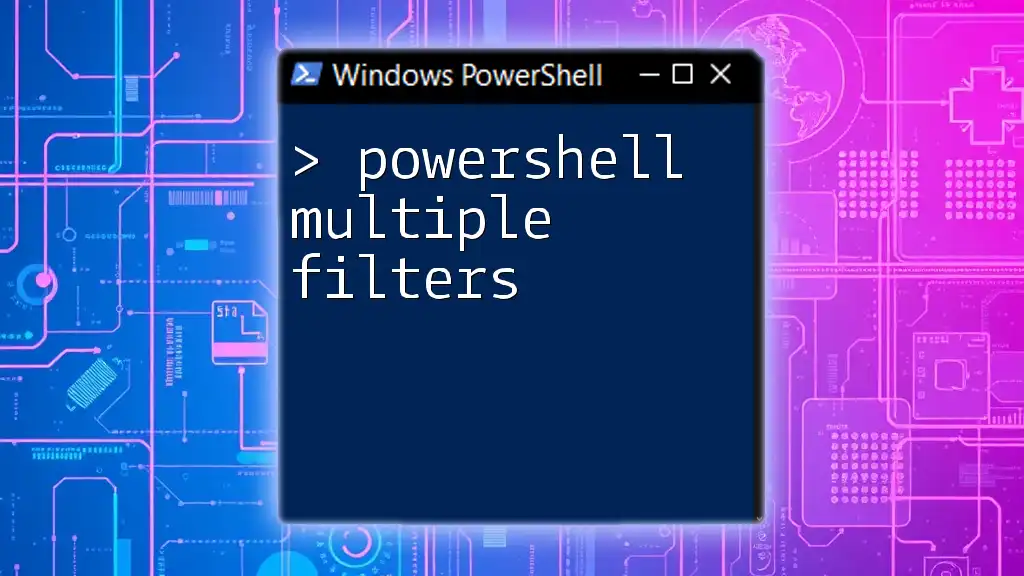
Tips for Managing Multiple Parameters
Using Parameter Sets
Parameter sets allow you to define different groups of parameters that must be used together. This can simplify complexity when functions have optional or exclusive parameters. Here’s how you can define a function with parameter sets:
function Invoke-Action {
[CmdletBinding(DefaultParameterSetName = 'Set1')]
param(
[Parameter(ParameterSetName = 'Set1')]
[string]$Option1,
[Parameter(ParameterSetName = 'Set2')]
[string]$Option2
)
# Function logic based on the parameter set
}
Using Default Values
Assigning default values to parameters can enhance user experience by providing sensible defaults while still allowing customization. Here’s an example:
function Connect-Service {
param(
[string]$ServiceName = "DefaultService",
[int]$Timeout = 30
)
Write-Host "Connecting to $ServiceName with a timeout of $Timeout seconds."
}
Users can call `Connect-Service` without specifying parameters to utilize the defaults, making the function adaptable.
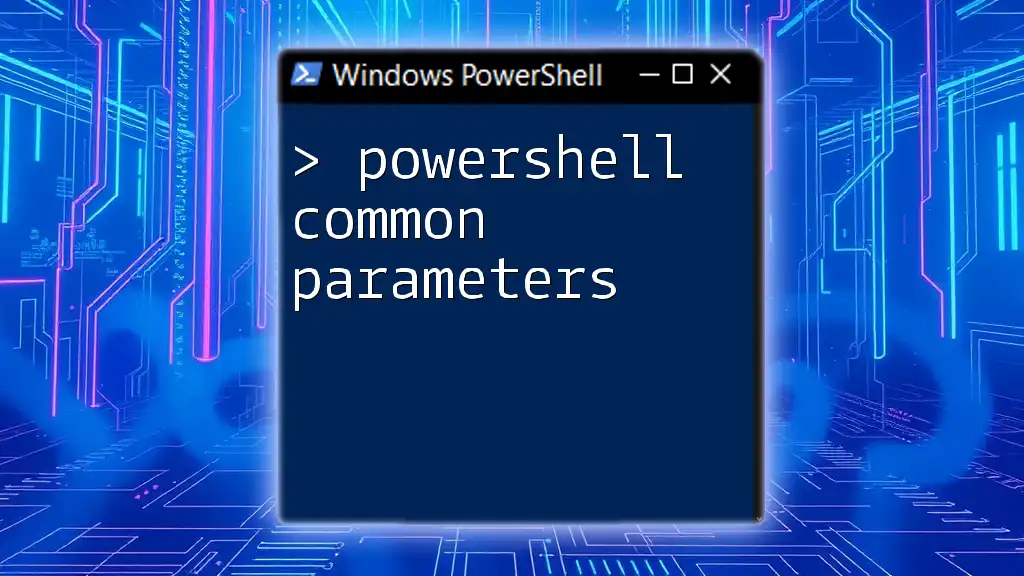
Advanced Techniques for Handling Multiple Parameters
Array Parameters for Dynamic Inputs
Sometimes, functions need to accept a variable number of arguments. This can be achieved using array parameters, allowing users to pass an array of values. Here’s an example:
function Add-Numbers {
param(
[int[]]$Numbers
)
$total = ($Numbers | Measure-Object -Sum).Sum
Write-Host "Total Sum: $total"
}
This function can be called with an array of integers:
Add-Numbers -Numbers @(1, 2, 3, 4, 5)
The output would be `Total Sum: 15`, demonstrating how array parameters add versatility.
Using Named Arguments
Using named arguments enhances function clarity, enabling users to understand what each input represents, especially when many parameters are involved. Here’s an example with a hypothetical function for sending emails:
Send-Email -To "example@example.com" -Subject "Hello" -Body "This is a test email."
This method promotes readability and minimizes the risk of errors when calling functions with multiple parameters.
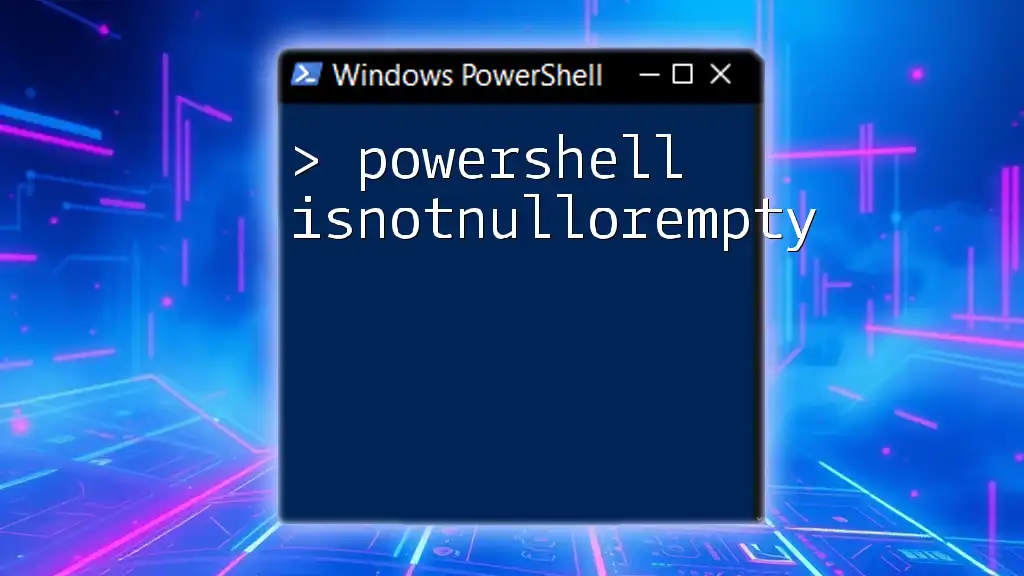
Practical Examples of PowerShell Functions with Multiple Parameters
Real-world Use Case #1: File Management
For practical applications, consider a function to copy files where multiple parameters define the source, destination, and overwrite behavior:
function Copy-FileWithOptions {
param(
[string]$Source,
[string]$Destination,
[bool]$Overwrite = $false
)
if (Test-Path -Path $Destination -and -not $Overwrite) {
Write-Host "Destination file exists. Use -Overwrite to proceed."
} else {
Copy-Item -Path $Source -Destination $Destination -Force
Write-Host "File copied from $Source to $Destination."
}
}
This function streamlines file operations by utilizing multiple parameters.
Real-world Use Case #2: User Creation
Another practical example involves a function designed for creating a user in an Active Directory setup, utilizing parameters for customization:
function New-ADUser {
param(
[string]$Name,
[string]$Title,
[string]$Department
)
# Code here to create AD user
Write-Host "Creating user $Name with title $Title in $Department department."
}
This function showcases how multiple parameters can facilitate user creation, allowing admins to specify details effectively.
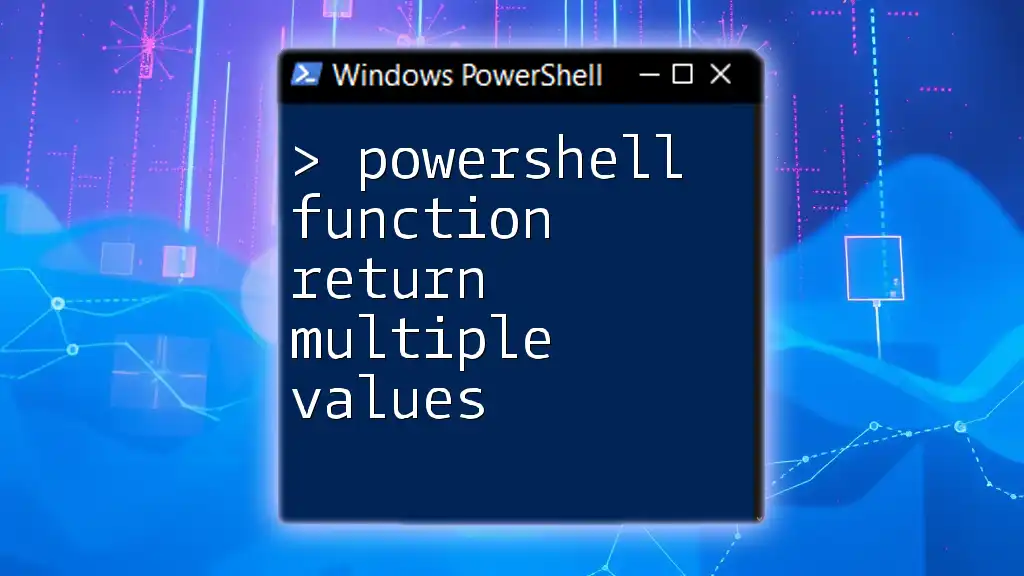
Conclusion
Functions in PowerShell become significantly more powerful and effective through the use of multiple parameters. By understanding various parameter types and validation techniques, you can create flexible, reusable, and reliable scripts that meet diverse user needs. Empowering users with these features enhances automation and ensures scripts perform as intended.
Further Learning Resources
To continue your mastery of PowerShell, explore official documentation and community forums. Engaging in hands-on practice and sharing your experiences can also foster deeper understanding.
Invite Readers to Share Their Experiences
As you implement what you've learned, share your experiences or examples of functions with multiple parameters. Engaging with a community can enhance your learning journey and inspire innovation in your scripts.
Promote Additional Resources and Courses
Stay tuned for more courses and tutorials that will guide you further into the depths of PowerShell scripting. Unlock the full potential of your coding skills with further knowledge and expertise.