The `Select-String` cmdlet in PowerShell allows you to search for multiple patterns within a file or string using regular expressions, enabling efficient text pattern matching.
Here’s a code snippet that demonstrates selecting multiple patterns:
Select-String -Path "C:\example\file.txt" -Pattern "pattern1", "pattern2"
Understanding Patterns in Select-String
What are String Patterns?
String patterns are sequences of characters that you can search for in text using commands or scripts. They can be simple sequences like the word “error,” or more complex expressions defined by regular expressions (regex), which offer powerful mechanisms to match various text forms.
Regex patterns can include:
- Character classes: For example, `[aeiou]` matches any vowel.
- Anchors: Such as `^` for the start of a line and `$` for the end.
- Quantifiers: Like `*`, `+`, and `?`, which define how many instances of the preceding element must appear for a match.
Understanding string patterns is crucial when using Select-String as it processes your patterns to find matching text efficiently.
How Select-String Processes Patterns
The Select-String cmdlet interprets your patterns and searches through lines of text to find matches. Depending on the complexity of your pattern, it may return all occurrences in a single line or highlight lines in your target file with matches.
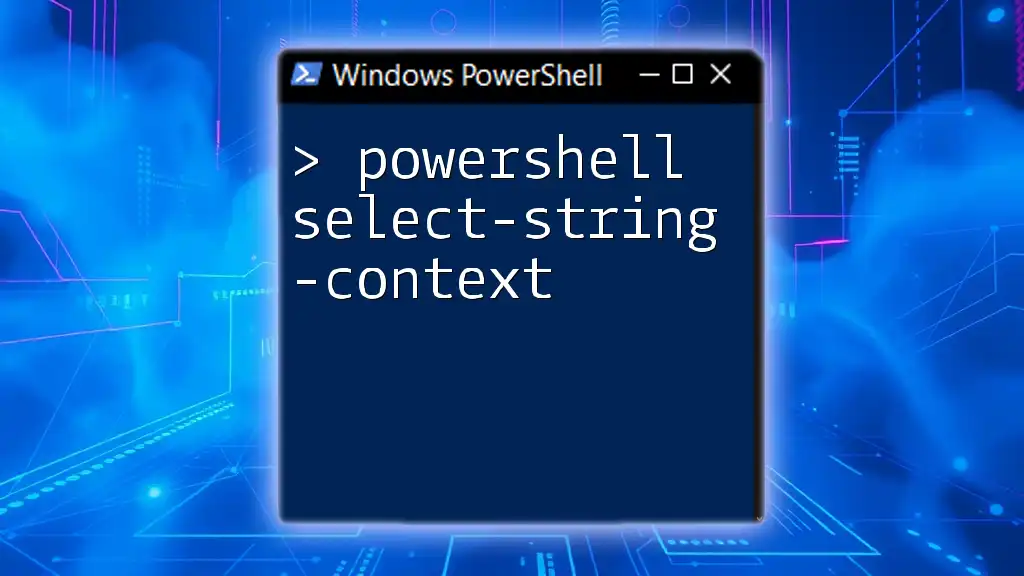
Utilizing Select-String for Multiple Patterns
The Need for Multiple Pattern Searches
In many real-world scenarios, you may need to search for more than one specific term within text. For instance, when analyzing logs for errors and warnings, using multiple patterns allows you to quickly identify critical issues without needing to execute multiple searches separately.
Advantages of combining search patterns include:
- Time efficiency: It reduces the number of searches required, streamlining the process.
- Comprehensive results: You capture all relevant occurrences in one go.
Syntax for Setting Up Select-String
Basic Syntax
The basic command structure of Select-String is straightforward. You need to specify the pattern you intend to search for along with the path of the file you are examining. Here’s the basic syntax:
Select-String -Pattern 'pattern1' -Path 'file.txt'
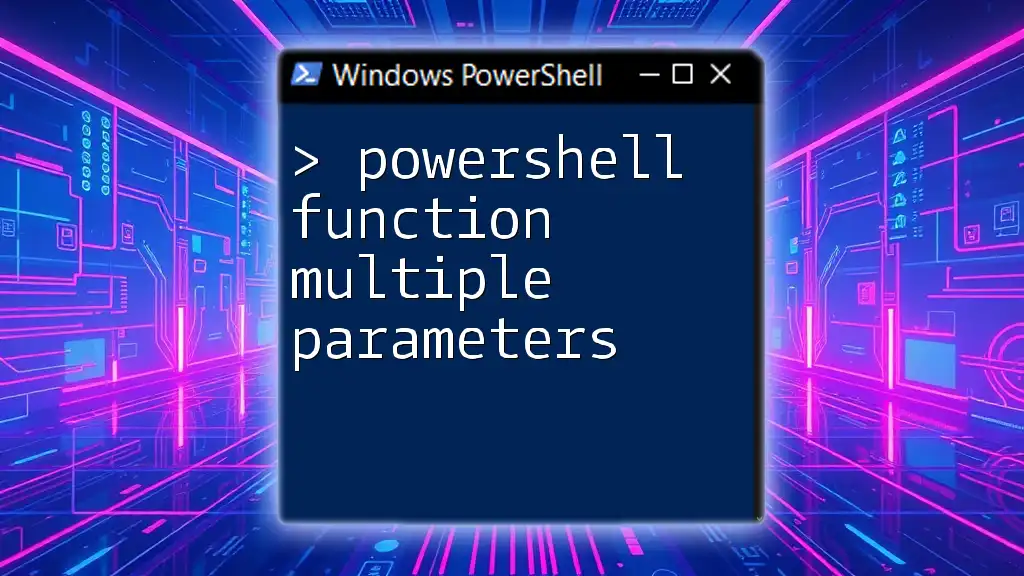
Combining Multiple Patterns
Using Comma-Separated Values
A simple method to search with multiple patterns is to use a comma between each pattern you want to include. For instance:
Select-String -Pattern 'pattern1', 'pattern2' -Path 'file.txt'
By employing this method, Select-String will return matches for both specified patterns, enhancing your search results.
Using Regular Expressions
For more advanced needs, utilizing regular expressions gives you greater flexibility. You can combine patterns using the pipe (`|`) operator, which represents "or" in regex. The following command searches for both `pattern1` and `pattern2`:
Select-String -Pattern 'pattern1|pattern2' -Path 'file.txt'
Understanding the Pipe (|) Operator
The pipe operator is pivotal in regex as it allows you to define alternative patterns to search for. This means that if either `pattern1` or `pattern2` is found in the text, that line will be returned in the results. This functionality is highly beneficial when dealing with complex search requirements.
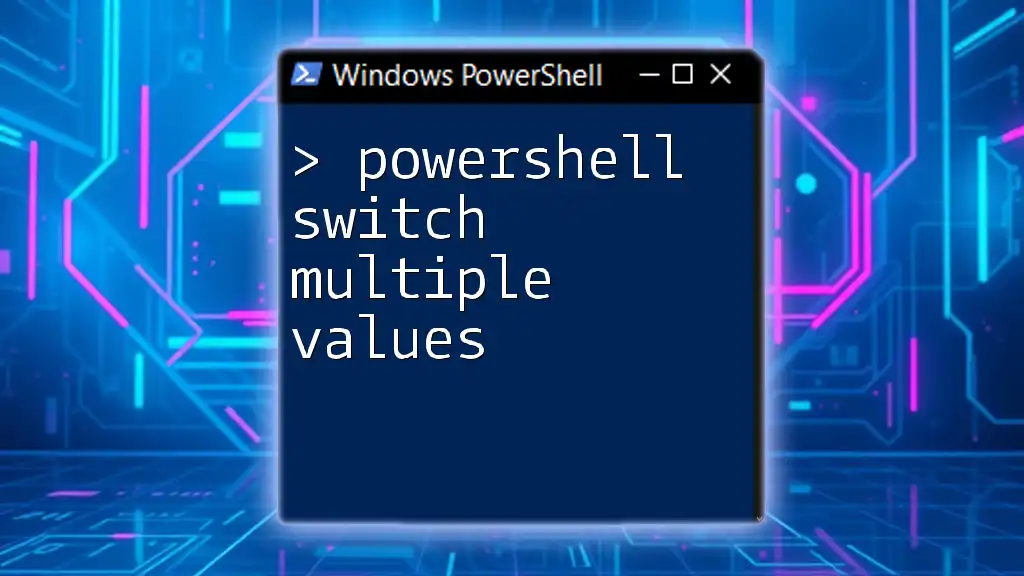
Practical Examples of Select-String with Multiple Patterns
Example 1: Searching Text Files
Imagine you want to identify both errors and warnings in a configuration file. You can use Select-String as follows:
Get-Content 'documents.txt' | Select-String -Pattern 'error|warning'
This command reads the content of `documents.txt` and searches for lines containing either "error" or "warning."
Example 2: Searching Logs
If you're analyzing a log file for critical issues, you can easily expand your search to include multiple levels of severity:
Get-Content 'system.log' | Select-String -Pattern 'failed|critical|warning'
This will return all lines from `system.log` that match the specified patterns, helping you quickly assess potential system problems.
Example 3: Using Select-String with Additional Parameters
To refine your search further, you can use additional parameters like `-CaseSensitive` and `-Context`. For instance:
Select-String -Pattern 'pattern1|pattern2' -Path 'file.txt' -CaseSensitive -Context 0,2
This command performs a case-sensitive search and returns the two lines of context before and after each match, providing a broader understanding of the content surrounding your patterns.
Example 4: Recursively Searching Directories
When working within directory structures, you might want to search not just in a single file but throughout nested folders. You can do this using:
Get-ChildItem -Recurse | Select-String -Pattern 'pattern1|pattern2'
This command searches through all files and subdirectories, returning matches for the specified patterns across the entire directory tree.
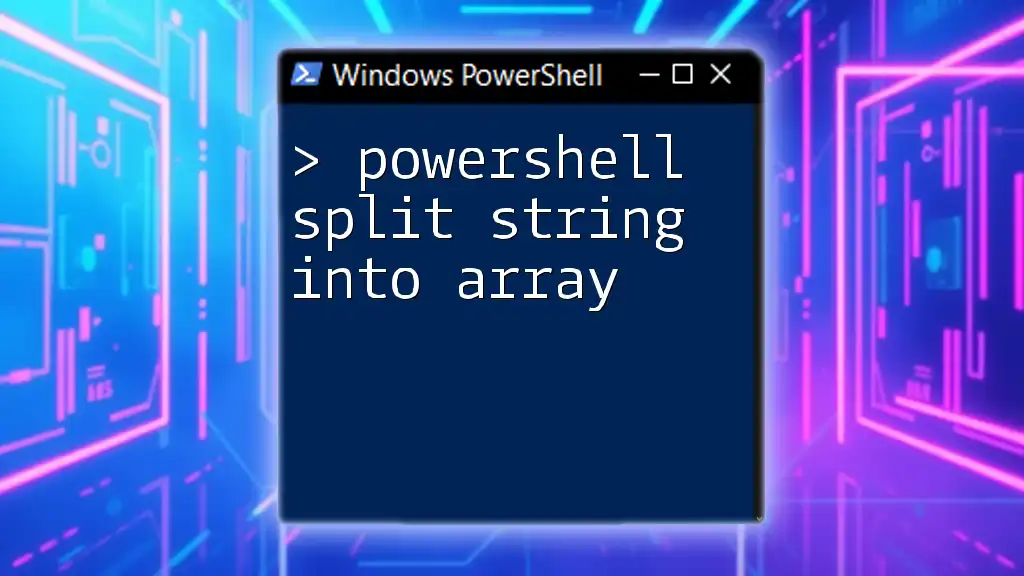
Best Practices for Using Select-String with Multiple Patterns
Efficiency Tips
When utilizing Select-String with multiple patterns, it’s crucial to maintain performance, especially with large datasets. Here are a few tips:
- Avoid overly complex regex patterns, as they may slow down your search.
- Implement Select-String in a way that narrows down the dataset before executing pattern searches if possible.
Error Handling in Pattern Searches
It’s essential to account for potential errors during pattern searches. Using Try-Catch blocks not only helps manage exceptions but also enhances the overall reliability of your PowerShell scripts.
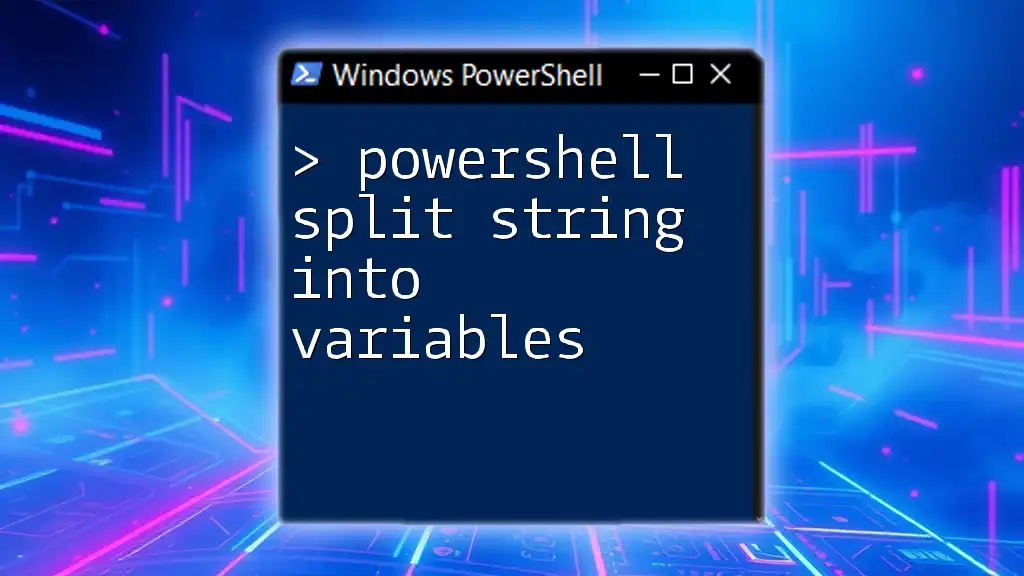
Conclusion
The PowerShell cmdlet Select-String offers robust capabilities for searching through text, especially when dealing with multiple patterns. Understanding how to leverage both simple and advanced regex techniques allows for more efficient text analysis. As you practice searching with real-world patterns, you'll become more proficient in extracting valuable insights from your data effectively.
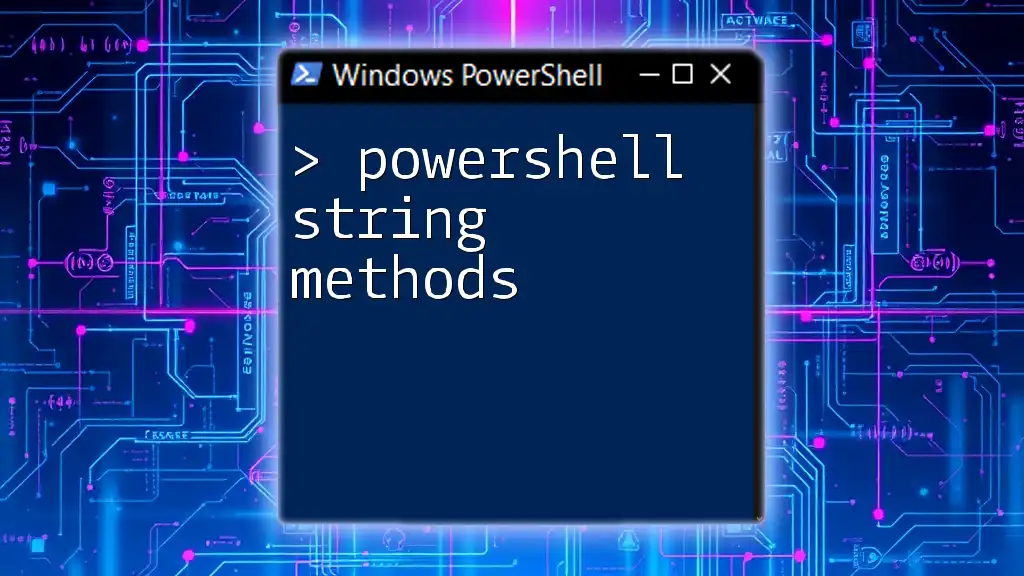
Additional Resources
For further learning, explore official PowerShell documentation, or consider investing time in recommended books and online courses focusing on regular expressions and PowerShell scripting. There is always more to learn, and expanding your skills will empower you to utilize Select-String like an expert.
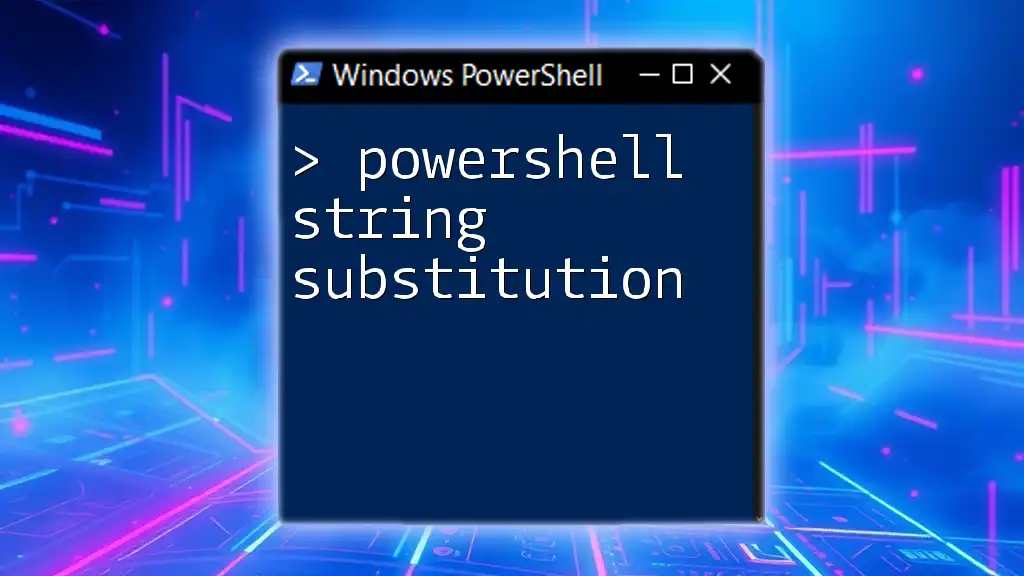
FAQ Section
How can I ignore case when searching? You can use the `-CaseSensitive` switch to control this feature. By default, searches are case-insensitive unless specified otherwise.
Can Select-String search multiple file types? Yes, you can specify the file types with wildcards. For example, you can search all `.log` files in a directory by using `Get-ChildItem *.log | Select-String -Pattern 'pattern'`.
By mastering the use of Select-String with multiple patterns, you open a world of possibilities for efficient and effective text searching in your PowerShell projects. Happy scripting!